一个表三级联动---WinFrom
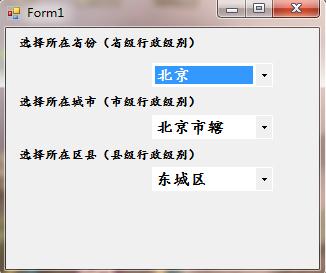

1 using System; 2 using System.Collections.Generic; 3 using System.ComponentModel; 4 using System.Data; 5 using System.Data.SqlClient; 6 using System.Drawing; 7 using System.Linq; 8 using System.Text; 9 using System.Threading.Tasks; 10 using System.Windows.Forms; 11 12 namespace rr 13 { 14 public partial class Form1 : Form 15 { 16 public Form1() 17 { 18 InitializeComponent(); 19 } 20 private void Fill1() 21 { 22 List<ChinaStates> list = new ChinaStatesDA().Select("0001"); 23 comboBox1.DataSource = list; 24 comboBox1.DisplayMember = "AreaName"; 25 comboBox1.ValueMember = "AreaCode"; 26 } 27 private void Fill2() 28 { 29 30 string parentcode=""; 31 if (comboBox1.SelectedItem!=null) 32 { 33 parentcode = (comboBox1.SelectedItem as ChinaStates).AreaCode; 34 35 } 36 37 List<ChinaStates> list = new ChinaStatesDA().Select(parentcode); 38 comboBox2.DataSource = list; 39 comboBox2.DisplayMember = "AreaName"; 40 comboBox2.ValueMember = "AreaCode"; 41 42 } 43 private void Fill3() 44 { 45 string parentcode = ""; 46 if (comboBox2.SelectedItem != null) 47 { 48 parentcode = (comboBox2.SelectedItem as ChinaStates).AreaCode; 49 50 } 51 List<ChinaStates> list = new ChinaStatesDA().Select(parentcode); 52 comboBox3.DataSource = list; 53 comboBox3.DisplayMember = "AreaName"; 54 comboBox3.ValueMember = "AreaCode"; 55 56 } 57 private void comboBox2_SelectedIndexChanged(object sender, EventArgs e) 58 { 59 60 Fill3(); 61 } 62 63 private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) 64 { 65 Fill2(); 66 Fill3(); 67 } 68 69 private void Form1_Load(object sender, EventArgs e) 70 { 71 Fill1(); 72 Fill2(); 73 Fill3(); 74 } 75 } 76 class DBcon 77 { 78 public const string CONSTR = "server=.;database=yy;uid=sa;pwd=111111"; 79 } 80 class ChinaStates 81 { 82 public string AreaCode { get; set; } 83 public string AreaName { get; set; } 84 public string ParentCode { get; set; } 85 } 86 class ChinaStatesDA 87 { 88 private SqlConnection Con; 89 private SqlCommand Cmd; 90 private SqlDataReader dr; 91 public ChinaStatesDA() 92 { 93 Con = new SqlConnection(DBcon.CONSTR); 94 Cmd = Con.CreateCommand(); 95 } 96 public List<ChinaStates> Select(string code) 97 { 98 List<ChinaStates> list = new List<ChinaStates>(); 99 Cmd.CommandText = "select * from ChinaStates where ParentAreaCode=@code"; 100 Cmd.Parameters.Clear(); 101 Cmd.Parameters.AddWithValue("@code", code); 102 try 103 { 104 Con.Open(); 105 dr = Cmd.ExecuteReader(); 106 while (dr.Read()) 107 { 108 ChinaStates data = new ChinaStates(); 109 data.AreaCode = dr["AreaCode"].ToString(); 110 data.AreaName = dr["AreaName"].ToString(); 111 data.ParentCode = dr["ParentAreaCode"].ToString(); 112 list.Add(data); 113 } 114 } 115 finally 116 { 117 Con.Close(); 118 } 119 120 121 return list; 122 } 123 } 124 }