C++标准库string
C++标准库string
定义和初始化
string s1 | 默认初始化,s1是一个空串 |
---|---|
string s2(s1) | s2是s1的副本 |
string s2 = s1 | 等价于s2(s1),s2是s1的副本 |
string s3("value") | s3是字面值"value"的副本,除了字面值最后的那个空字符外 |
string s3 = "value" | 等价于s3("value"),s3是字面值"value"的副本 |
string s4(n, 'c') | 把s4初始化为由连续n个字符c组成的串 |
直接初始化和拷贝初始化
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1; // 默认初始化,空字符串
string s2("haha"); // 直接初始化
string s3 = "haha"; // 拷贝初始化
string s4(10, 'a'); // 直接初始化
cout << s1 << endl
<< s2 << endl
<< s3 << endl
<< s4 << endl;
system("pause");
return 0;
}
程序输出结果
haha
haha
aaaaaaaaaa
请按任意键继续. . .
string对象上的操作
读写string对象
- 写入一个字符串。
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s;
cin >> s;
cout << s << endl;
system("pause");
return 0;
}
程序输出结果
hello world
hello
请按任意键继续. . .
- 写入两个字符串。
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1, s2;
cin >> s1 >> s2;
cout << s1 << s2 << endl;
system("pause");
return 0;
}
程序输出结果
hello world
helloworld
请按任意键继续. . .
- 读取未知数量的string对象
#include<iostream>
#include<string>
using namespace std;
int main()
{
string word;
while (cin >> word)
cout << word << endl;
system("pause");
return 0;
}
程序输出结果
hi
hi
visual
visual
studio
studio
^Z
请按任意键继续. . .
使用ctrl + Z结束输入。
使用getline读取一整行
保留字符串中的空白符,从给定的输入流中读入内容,直到遇到换行符位置,然后把所读的内容存入到string对象中。
#include<iostream>
#include<string>
using namespace std;
int main()
{
string line;
while (getline(cin, line))
cout << line;
system("pause");
return 0;
}
程序输出结果
hello hi !!!
hello hi !!!
wooohhh woc
wooohhh woc
^Z
请按任意键继续. . .
- 只输出非空的行
#include<iostream>
#include<string>
using namespace std;
int main()
{
string line;
while (getline(cin, line))
// 只输出非空的行
if(!line.empty())
cout << line;
system("pause");
return 0;
}
- 只输出超过10个字符的行
#include<iostream>
#include<string>
using namespace std;
int main()
{
string line;
while (getline(cin, line))
// 只输出超过10个字符的行
if (line.size()>10)
cout << line;
system("pause");
return 0;
}
程序输出结果
hello
hello world
hello world
^Z
请按任意键继续. . .
string对象相加
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello";
string s2 = " world\n";
//string s3 = "hello" + " world\n"; //错误
string s3 = s1 + s2;
string s4 = s1 + " haha\n"; //正确,字面值可以和string对象相加
cout << s3 << endl;
cout << s4 << endl;
system("pause");
return 0;
}
程序运行结果
hello world
hello haha
请按任意键继续. . .
处理string对象中的字符
-
cctype头文| isalnum(c) | 如果c是字母或数字,则返回True |
|--------------|------------------------------------------------------|
| isalpha(c) | 如果c是字母,则返回true |
| iscntrl(c) | 如果c是控制字符,则返回true |
| isdigit(c) | 如果c是数字,则返回true |
| isgraph(c) | 如果c不是空格,但可打印,则为true |
| islower(c) | 如果c是小写字母,则为true |
| isprint(c) | 如果c是可打印字符,则为true |
| ispunct(c) | 如果c是标点符号,则为true |
| isspace(c) | 如果c是空白是否,则为true |
| isupper(c) | 如果c是大写字母,则为true |
| isxdigit(c) | 如果c是十六进制的数,则为true |
| tolower(c) | 如果c是大写字母,返回其小写字母的形式,否则直接返回c |
| toupper(c) | 如果c是小写字母,返回其大写字母的形式,否则直接返回c |
件中的函数 -
把string对象中的字符每行打印出来
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "some string";
cout << "method a" << endl;
for (auto c : s1)
cout << c << endl;
//that equals to
cout << "method b" << endl;
for (int i = 0; i < s1.size(); ++i)
cout << s1[i] << endl; // 通过下标索引
system("pause");
return 0;
}
程序输出结果
method a
s
o
m
e
s
t
r
i
n
g
method b
s
o
m
e
s
t
r
i
n
g
请按任意键继续. . .
- 统计string对象中标点符号的个数
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello, world!!!";
decltype(s1.size()) count = 0;
for (auto c : s1)
if (ispunct(c))
++count;
cout << s1 + "中共有"
<<count
<<"个标点符号" << endl;
system("pause");
return 0;
}
程序输出结果
hello, world!!!中共有4个标点符号
请按任意键继续. . .
当然还可以实现其他很多功能,就不一一列举。
出处: http://www.cnblogs.com/liutongqing/
本文版权归作者和博客园共有,欢迎转载、交流,但未经作者同意必须保留此段声明,且在文章明显位置给出原文链接。
如果觉得本文对您有益,欢迎点赞、欢迎打赏。
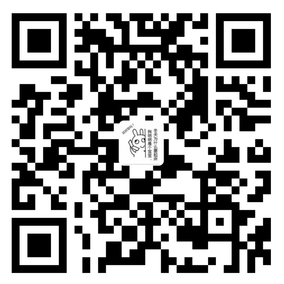
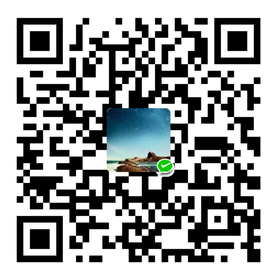
posted on 2017-08-07 22:46 tongqingliu 阅读(5791) 评论(1) 编辑 收藏 举报