C++实现安全队列
```cpp
#ifndef SAFEQUEUE
#define SAFEQUEUE
#include <iostream>
#include <queue>
#include <thread>
#include <mutex>
#include <condition_variable>
using namespace std;
template<typename T>
class SafeQueue {
public:
SafeQueue() {}
~SafeQueue() {}
void InitQueue()
{
work = 1;
}
void EnQueue(T value)
{
lock_guard<mutex> lock(mt);
if (work) {
q.push(value);
cv.notify_one();
}
}
void Print()
{
lock_guard<mutex> lock(mt);
queue<T> copy_queue = q;
while (!q.empty())
{
copy_queue.push(q.front());
q.pop();
}
while (!copy_queue.empty())
{
std::cout << copy_queue.front() << std::endl;
copy_queue.pop();
}
}
int DeQueue(T& value)
{
int ret = 0;
//占用空间相对lock_guard 更大一点且相对更慢一点,但是配合条件必须使用它,更灵活
unique_lock<mutex> lock(mt);
//第二个参数 lambda表达式:false则不阻塞 往下走
cv.wait(lock, [this] {return !work || !q.empty(); });
if (!q.empty()) {
value = q.front();
q.pop();
ret = 1;
}
return ret;
}
void setWork(int work)
{
lock_guard<mutex> lock(mt);
this->work = work;
}
void Clear()
{
lock_guard<mutex> lock(mt);
while (!q.empty())
{
q.pop();
}
}
int Find(T value)
{
return nullptr;
}
private:
mutex mt;
condition_variable cv;
queue<T> q;
//是否工作的标记 1 :工作 0:不接受数据 不工作
int work;
};
#endif //SAFEQUEUE
作者:
tongqingliu
出处: http://www.cnblogs.com/liutongqing/
本文版权归作者和博客园共有,欢迎转载、交流,但未经作者同意必须保留此段声明,且在文章明显位置给出原文链接。
如果觉得本文对您有益,欢迎点赞、欢迎打赏。
出处: http://www.cnblogs.com/liutongqing/
本文版权归作者和博客园共有,欢迎转载、交流,但未经作者同意必须保留此段声明,且在文章明显位置给出原文链接。
如果觉得本文对您有益,欢迎点赞、欢迎打赏。
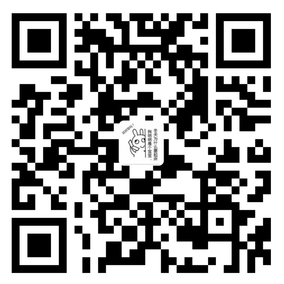
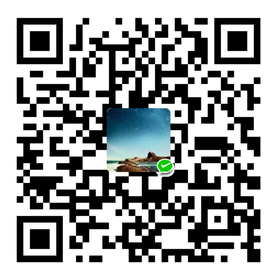
posted on 2020-09-01 18:00 tongqingliu 阅读(187) 评论(0) 编辑 收藏 举报