第十一周,十二周作业
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo9Activity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_demo9);
findViewById(R.id.btn1);
findViewById(R.id.btn2);
}
public void click1(View view){
EditText et1=findViewById(R.id.et1);
String fileName="data.txt";
String content=et1.getText().toString();
FileOutputStream fos=null;
try{
fos=openFileOutput(fileName,MODE_PRIVATE);
fos.write(content.getBytes());
}catch (Exception e){
e.printStackTrace();
}finally{
try{
if (fos!=null){
fos.close();
}
}catch (IOException e){
e.printStackTrace();
}
}
Toast.makeText(Demo9Activity.this,"储存成功",Toast.LENGTH_LONG).show();
}
public void click2(View v){
EditText et2=findViewById(R.id.et2);
String content="";
FileInputStream fis=null;
try {
fis=openFileInput("data.txt");
byte[]buffer=new byte[fis.available()];
fis.read(buffer);
content=new String(buffer);
} catch (Exception e) {
e.printStackTrace();
}finally {
try{
if (fis!=null){
fis.close();
}
}catch (IOException e){
e.printStackTrace();
}
}
et2.setText(content);
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Demo9Activity"
android:orientation="vertical">
<EditText
android:id="@+id/et1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入数据"/>
<Button
android:id="@+id/btn1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="储存"
android:onClick="click1"
/>
<EditText
android:id="@+id/et2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<Button
android:id="@+id/btn2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读取"
android:onClick="click2"/>
</LinearLayout>
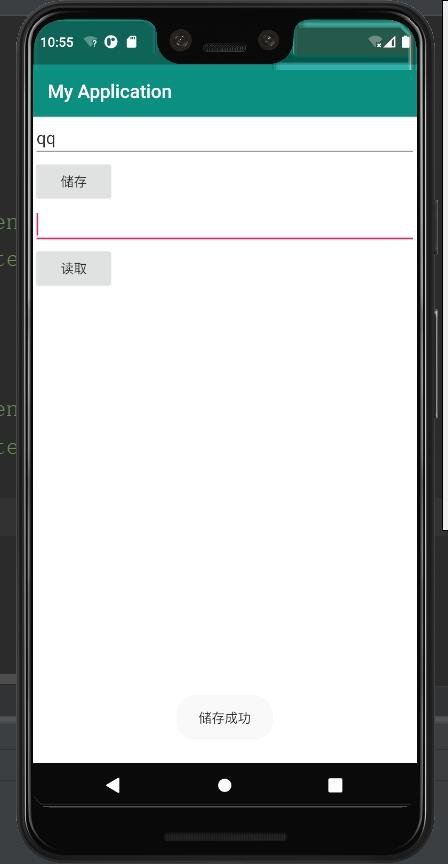
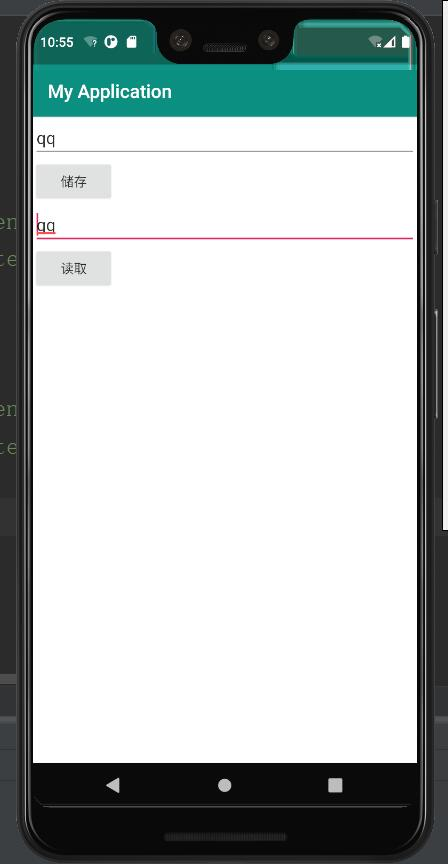
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="用户登录"
android:textColor="#FFC0CB"
android:textSize="30dp"/>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="账号"
android:textColor="#D8BFD8"
android:textSize="20dp"/>
<EditText
android:id="@+id/a1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入用户名" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="密码"
android:textColor="#D8BFD8"
android:textSize="20dp"/>
<EditText
android:id="@+id/a2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入密码" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<CheckBox
android:id="@+id/b1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="记住密码"
android:textSize="15dp" />
<CheckBox
android:id="@+id/b2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="自动登录"
android:textSize="15dp" />
<Button
android:id="@+id/c1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="登录"
android:textSize="20dp"
android:textColor="#9370DB"/>
</LinearLayout>
</LinearLayout>
package com.example.gc1;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.os.SharedMemory;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TableLayout;
import android.widget.Toast;
import java.io.FileInputStream;
import java.util.HashMap;
import java.util.Map;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private EditText a1;//账号输入框
private EditText a2;//密码输入框
private Button c1;//登录按钮
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
Map<String,String>userInfo = SPSaveQQ.getUserInfo(this);
if (userInfo!=null){
a1.setText(userInfo.get("account"));
a2.setText(userInfo.get("pssword"));
}
}
private void initView() {
a1=(EditText)findViewById(R.id.a1);
a2=(EditText)findViewById(R.id.a2);
c1=(Button)findViewById(R.id.c1);
c1.setOnClickListener(this);
}
public void onClick(View view){
switch (view.getId()){
case R.id.c1:
String account =a1.getText().toString().trim();
String password =a2.getText().toString();
if (TextUtils.isEmpty(account)){
Toast.makeText(this,"请输入账号",Toast.LENGTH_SHORT).show();
return;
}
if (TextUtils.isEmpty(password)){
Toast.makeText(this,"请输入密码",Toast.LENGTH_SHORT).show();
return;
}
Toast.makeText(this,"登陆成功",Toast.LENGTH_SHORT).show();
boolean isSaveSuccess =SPSaveQQ.saveUserInfo(this,account,password);
if (isSaveSuccess){
Toast.makeText(this,"保存成功", Toast.LENGTH_SHORT).show();
}else
{
Toast.makeText(this,"保存失败",Toast.LENGTH_SHORT).show();
}
break;
}
}
public static class SPSaveQQ{
public static boolean saveUserInfo(Context context,String account,String password){
SharedPreferences sp =context.getSharedPreferences("data",Context.MODE_PRIVATE);
SharedPreferences.Editor edit =sp.edit();
edit.putString("userName",account);
edit.putString("pwd",password);
edit.commit();
return true;
}
public static Map<String,String> getUserInfo(Context context){
SharedPreferences sp =context.getSharedPreferences("data",Context.MODE_PRIVATE);
String account =sp.getString("userName",null);
String password =sp.getString("pwd",null);
Map<String,String> userMap =new HashMap<String, String>();
userMap.put("account",account);
userMap.put("password",password);
return userMap;
}
}
}