Python_oldboy_自动化运维之路(二)
本节内容:
1.pycharm工具的使用
2.进制运算
3.表达式if ...else语句
4.表达式for 循环
5.break and continue
6.表达式while 循环
1.pycharm工具的使用
【下载安装】
下载:http://pan.baidu.com/s/1jHPbo5g 密码:yhhf
破解:pycharm有两个版本,社区版和专业版,建议购买专业版~~~~
安装:下一步下一步,比较简单,就不废话了。
视频:http://list.youku.com/albumlist/show?id=28961509&ascending=1&page=1&qq-pf-to=pcqq.group
【使用】
1. 养成一个好习惯,一个项目里面建立相关的文件夹和脚本
2.优化设置
- 更改脚本里面内容的字体大小:File>Settings>Ediror>Colors & Fonts>Font
- 设置脚本的抬头注释:File>Settings>Editor>File and Code Templates>Python Script
- 设置显示脚本的行数:File>Setting>Editor>General>Apperance>勾选“Show line numbers”。
- pycharm Tab键设置成4个空格:File>Setting>Editor>Code Style>python
3.效果演示
2.进制运算
【补充知识:变量】
python中的变量是指向内存单元的值。
1 name = "alex" 2 name2 = name 3 4 print(name,name2) 5 6 name = "jack" 7 8 print(name,name2)
alex alex
jack alex
【%s,%d,%f,%r】
%s 任意字符
%d 必须是整形数字
%f 浮点型
%r raw string 原生字符,不转译
1 msg = "my name is %s and age is %s" %("lijun","22") 2 print(msg) 3 msg = "my name is %s and age is %d" %("lijun",22) 4 print(msg) 5 msg = "my name is %s and age is %f" %("lijun",22) 6 print(msg) 7 msg = "my name is %s and age is %r" %("lijun","aaa\n\tbbb") 8 print(msg) 9 msg = "my name is %s and age is %s" %("lijun","aaa\n\tbbb") 10 print(msg)
my name is lijun and age is 22 my name is lijun and age is 22 my name is lijun and age is 22.000000 my name is lijun and age is 'aaa\n\tbbb' my name is lijun and age is aaa bbb
【算数运算】
【比较运算】
【赋值运算】
【逻辑运算】
【成员运算】
【位运算】
a = 22
o = 56
128 64 32 16 8 4 2 1
a = 0 0 0 1 0 1 1 0
o = 0 0 1 1 1 0 0 0
a&o = 0 0 0 1 0 0 0 0 = 32
a|o = 0 0 1 1 1 1 1 0 = 62
a<<1 = 0 0 1 0 1 1 0 0 = 44
o>>1 = 0 0 0 1 1 1 0 0 = 28
【运算符优先级】
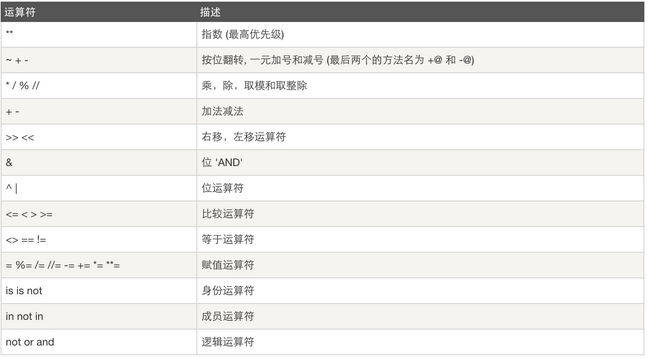
3.表达式if ...else语句
案例1:用户登录验证
1 # 提示输入用户名和密码 2 3 # 验证用户名和密码 4 # 如果错误,则输出用户名或密码错误 5 # 如果输入的用户名是chenlijun,就显示禁止登陆 6 # 如果成功,则输出 成功 7 # 最多允许输错三次 8 9 login = "root" 10 passwd = "root123" 11 12 for i in range(3): 13 newlogin = input("please input your user:") 14 newpasswd = input("please input youer passwd:") 15 if login == newlogin and passwd == newpasswd: 16 print ("login successfyl") 17 break 18 elif newlogin == 'chenlijun': 19 print ("use %s is disable" % newlogin) 20 break 21 else: 22 print ("user or passwd error") 23 24 else: 25 print ("你的密码输错三次以上,被锁到海枯石烂,哈哈哈!You input the wrong password three times, locked to the end, ha ha ha!")
案例2:猜年龄游戏
在程序里设定好你的年龄,然后启动程序让用户猜测,用户输入后,根据他的输入提示用户输入的是否正确,如果错误,提示是猜大了还是小了
1 #!/usr/bin/python 2 # -*- coding: UTF-8 -*- 3 age = 24 4 5 newage = int(input("youerage:")) 6 7 if age == newage: 8 print ("恭喜你,猜对啦~~") 9 10 elif age < newage: 11 print ("我有那么老吗?臭傻逼~~~") 12 13 else: 14 print ("我有那么小吗?臭傻逼~~~")
4.表达式for 循环
【循环原理其实就是一个列表】
案例1:年龄输错三次就退出,结束,猜小了提示年龄小,猜大了提示年龄大。
1 #!/usr/bin/python 2 # -*- coding: UTF-8 -*- 3 #blog:http://www.cnblogs.com/linux-chenyang/ 4 age = 24 5 6 for i in range(10): 7 8 if i == 3: 9 print("bay....") 10 break 11 newage = int(input("youerage:")) 12 13 if age == newage: 14 print ("恭喜你,猜对啦~~") 15 break 16 17 elif age < newage: 18 print ("我有那么老吗?臭傻逼~~~") 19 20 else: 21 print ("我有那么小吗?臭傻逼~~~")
youerage:23 我有那么小吗?臭傻逼~~~ youerage:47 我有那么老吗?臭傻逼~~~ youerage:100 我有那么老吗?臭傻逼~~~ bay....
案例2:增加提示,如果输入三次还想继续猜,输入Y,输入N退出。
1 #!/usr/bin/python 2 # -*- coding: UTF-8 -*- 3 #blog:http://www.cnblogs.com/linux-chenyang/ 4 5 age = 24 6 count = 0 7 8 for i in range(10): 9 10 if count == 3: 11 prompt = input("do you want to continue(Y/N):") 12 if prompt == "Y": 13 count = 0 14 else: 15 break 16 newage = int(input("youerage:")) 17 18 if age == newage: 19 print ("恭喜你,猜对啦~~") 20 break 21 22 elif age < newage: 23 print ("我有那么老吗?臭傻逼~~~") 24 25 else: 26 print ("我有那么小吗?臭傻逼~~~") 27 28 count += 1
youerage:22 我有那么小吗?臭傻逼~~~ youerage:29 我有那么老吗?臭傻逼~~~ youerage:30 我有那么老吗?臭傻逼~~~ do you want to continue(Y/N):Y youerage:22 我有那么小吗?臭傻逼~~~ youerage:22 我有那么小吗?臭傻逼~~~ youerage:22 我有那么小吗?臭傻逼~~~ do you want to continue(Y/N):N Process finished with exit code 0
5.break and continue
案例1:循环套循环
1 #!/usr/bin/python 2 # -*- coding: UTF-8 -*- 3 #blog:http://www.cnblogs.com/linux-chenyang/ 4 5 for i in range(10): 6 if i == 5: 7 for j in range(10): 8 print("inner forloop", j) 9 if j == 8: 10 break 11 continue 12 print("forloop", i)
forloop 0 forloop 1 forloop 2 forloop 3 forloop 4 inner forloop 0 inner forloop 1 inner forloop 2 inner forloop 3 inner forloop 4 inner forloop 5 inner forloop 6 inner forloop 7 inner forloop 8 forloop 6 forloop 7 forloop 8 forloop 9 Process finished with exit code 0
6.表达式while 循环
案例1:两种方法对比时间的快慢
1 #!/usr/bin/python 2 # -*- coding: UTF-8 -*- 3 #blog:http://www.cnblogs.com/linux-chenyang/ 4 5 import time #导入时间模块 6 t0_start = time.time() #time.time()表示当前时间 7 8 count=0 9 while True: 10 if count == 1000000: 11 break 12 # print ("计数开始:", count) 13 count += 1 14 print ("count:",time.time()-t0_start,count) 15 16 17 t_start = time.time() 18 count0=0 19 while count0 < 1000000: 20 count0 += 1 21 print ("count0:",time.time()-t_start,count0)
count: 0.488048791885376 1000000 count0: 0.4170417785644531 1000000 Process finished with exit code 0
案例2:还是猜年龄,猜错三次就提示笨蛋,并退出。
1 age = 24 2 count = 0 3 4 while count < 3: 5 6 7 newage = int(input("youerage:")) 8 9 if age == newage: 10 print ("恭喜你,猜对啦~~") 11 12 elif age < newage: 13 print ("我有那么老吗?臭傻逼~~~") 14 15 else: 16 print ("我有那么小吗?臭傻逼~~~") 17 18 count += 1 19 else: 20 print("笨死了,猜那么多次还是猜不对~")
youerage:1 我有那么小吗?臭傻逼~~~ youerage:2 我有那么小吗?臭傻逼~~~ youerage:3 我有那么小吗?臭傻逼~~~ 笨死了,猜那么多次还是猜不对~ Process finished with exit code 0
7.知识补充
【三元运算】
result
=
值
1
if
条件
else
值
2
如果条件为真:result = 值1
如果条件为假:result = 值2
如果条件为假:result = 值2
