基于.NET平台的分层架构实战(四)——实体类的设计与实现
2008-06-18 15:15 T2噬菌体 阅读(15544) 评论(47) 编辑 收藏 举报实体类是现实实体在计算机中的表示。它贯穿于整个架构,负担着在各层次及模块间传递数据的职责。一般来说,实体类可以分为“贫血实体类”和“充血实体类”,前者仅仅保存实体的属性,而后者还包含一些实体间的关系与逻辑。我们在这个Demo中用的实体类将是“贫血实体类”。
大多情况下,实体类和数据库中的表(这里指实体表,不包括表示多对多对应的关系表)是一一对应的,但这并不是一个限制,在复杂的数据库设计中,有可能出现一个实体类对应多个表,或者交叉对应的情况。在本文的Demo中,实体类和表是一一对应的,并且实体类中的属性和表中的字段也是对应的。
在看实体类的代码前,先看一下系统的工程结构。
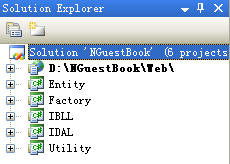
如上图所示,在初始阶段,整个系统包括6个工程,它们的职责是这样的:
Web——表示层
Entity——存放实体类
Factory——存放和依赖注入及IoC相关的类
IBLL——存放业务逻辑层接口族
IDAL——存放数据访问层接口族
Utility——存放各种工具类及辅助类
这只是一个初期架构,主要是将整个系统搭一个框架,在后续开发中,将会有其他工程被陆陆续续添加进来。
我们的实体类将放在Entity工程下,这里包括三个文件:AdminInfo.cs,MessageInfo.cs,CommentInfo.cs,分别是管理员实体类、留言实体类和评论实体类。具体代码如下:
AdminInfo.cs:
namespace NGuestBook.Entity
{
/// <summary>
/// 实体类-管理员
/// </summary>
[Serializable]
public class AdminInfo
{
private int id;
private string name;
private string password;
public int ID
{
get { return this.id; }
set { this.id = value; }
}
public string Name
{
get { return this.name; }
set { this.name = value; }
}
public string Password
{
get { return this.password; }
set { this.password = value; }
}
}
}
MessageInfo.cs:
namespace NGuestBook.Entity
{
/// <summary>
/// 实体类-留言
/// </summary>
[Serializable]
public class MessageInfo
{
private int id;
private string guestName;
private string guestEmail;
private string content;
private DateTime time;
private string reply;
private string isPass;
public int ID
{
get { return this.id; }
set { this.id = value; }
}
public string GuestName
{
get { return this.guestName; }
set { this.guestName = value; }
}
public string GuestEmail
{
get { return this.guestEmail; }
set { this.guestEmail = value; }
}
public string Content
{
get { return this.content; }
set { this.content = value; }
}
public DateTime Time
{
get { return this.time; }
set { this.time = value; }
}
public string Reply
{
get { return this.reply; }
set { this.reply = value; }
}
public string IsPass
{
get { return this.isPass; }
set { this.isPass = value; }
}
}
}
CommentInfo.cs:
namespace NGuestBook.Entity
{
/// <summary>
/// 实体类-评论
/// </summary>
[Serializable]
public class CommentInfo
{
private int id;
private string content;
private DateTime time;
private int message;
public int ID
{
get { return this.id; }
set { this.id = value; }
}
public string Content
{
get { return this.content; }
set { this.content = value; }
}
public DateTime Time
{
get { return this.time; }
set { this.time = value; }
}
public int Message
{
get { return this.message; }
set { this.message = value; }
}
}
}
大家可以看出,实体类的代码很简单,仅仅是负责实体的表示和数据的传递,不包含任何逻辑性内容。下篇将介绍接口的设计。

本文基于署名-非商业性使用 3.0许可协议发布,欢迎转载,演绎,但是必须保留本文的署名张洋(包含链接),且不得用于商业目的。如您有任何疑问或者授权方面的协商,请与我联系。