c++ 栈(顺序表)
栈可以用顺序表(数组)也可以用链表来储存内容,本文采用顺序表(数组)来保存内部元素。代码如下:
1 #include <iostream>
2 using namespace std; 3 4 class MyStack 5 { 6 private: 7 int l; 8 int count; 9 int * array; //这里要注意,不要用array[l],成员变量不能用成员变量初始化。 10 public: 11 MyStack(int len) 12 { 13 l=len; 14 count=0; 15 array=new int[l]; 16 }; 17 MyStack() //默认构造函数,最大容量为50. 18 { 19 l=50; 20 count=0; 21 array=new int[l]; 22 } 23 24 void push(int n) 25 { 26 if(count>l-1) 27 { 28 cout<<"out of boundary"<<endl; 29 return ; 30 }else 31 { 32 array[count]=n; 33 count++; 34 } 35 } 36 void pop() 37 { 38 if(count<0) 39 { 40 cout<<"empty!"<<endl; 41 }else 42 { 43 array[count--]=0; 44 } 45 } 46 47 int top() 48 { 49 return array[count]; 50 } 51 52 bool isEmpty() 53 { 54 return (count==0); 55 } 56 int stackSize() 57 { 58 return count+1; 59 } 60 }; 61 62 int main() 63 { 64 MyStack ms(10); 65 ms.push(10); 66 ms.push(4); 67 ms.push(3); 68 if(ms.isEmpty()) 69 cout<<"为空"<<endl; 70 cout<<"不为空"<<endl; 71 cout<<"长度为:"<<ms.stackSize()<<endl; 72 ms.pop(); 73 cout<<"出栈后顶部元素为:"<<ms.top()<<endl; 74 }
运行结果:
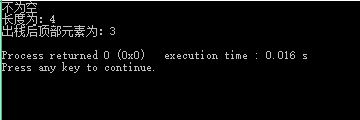