poj 1691 Painting A Board
Painting A Board
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 3018 | Accepted: 1441 |
Description
The CE digital company has built an Automatic Painting Machine (APM) to paint a flat board fully covered by adjacent non-overlapping rectangles of different sizes each with a predefined color.
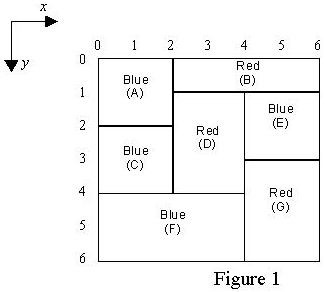
To color the board, the APM has access to a set of brushes. Each brush has a distinct color C. The APM picks one brush with color C and paints all possible rectangles having predefined color C with the following restrictions:
To avoid leaking the paints and mixing colors, a rectangle can only be painted if all rectangles immediately above it have already been painted. For example rectangle labeled F in Figure 1 is painted only after rectangles C and D are painted. Note that each rectangle must be painted at once, i.e. partial painting of one rectangle is not allowed.
You are to write a program for APM to paint a given board so that the number of brush pick-ups is minimum. Notice that if one brush is picked up more than once, all pick-ups are counted.
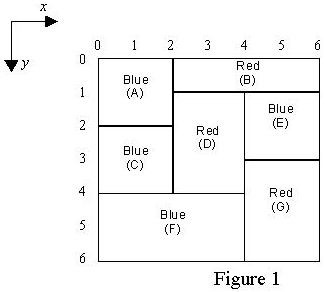
To color the board, the APM has access to a set of brushes. Each brush has a distinct color C. The APM picks one brush with color C and paints all possible rectangles having predefined color C with the following restrictions:
To avoid leaking the paints and mixing colors, a rectangle can only be painted if all rectangles immediately above it have already been painted. For example rectangle labeled F in Figure 1 is painted only after rectangles C and D are painted. Note that each rectangle must be painted at once, i.e. partial painting of one rectangle is not allowed.
You are to write a program for APM to paint a given board so that the number of brush pick-ups is minimum. Notice that if one brush is picked up more than once, all pick-ups are counted.
Input
The first line of the input file contains an integer M which is the number of test cases to solve (1 <= M <= 10). For each test case, the first line contains an integer N, the number of rectangles, followed by N lines describing the rectangles. Each rectangle R is specified by 5 integers in one line: the y and x coordinates of the upper left corner of R, the y and x coordinates of the lower right corner of R, followed by the color-code of R.
Note that:
Note that:
- Color-code is an integer in the range of 1 .. 20.
- Upper left corner of the board coordinates is always (0,0).
- Coordinates are in the range of 0 .. 99.
- N is in the range of 1..15.
Output
One line for each test case showing the minimum number of brush pick-ups.
Sample Input
1 7 0 0 2 2 1 0 2 1 6 2 2 0 4 2 1 1 2 4 4 2 1 4 3 6 1 4 0 6 4 1 3 4 6 6 2
Sample Output
3
题目大概意思就是有n个矩形需要涂上不同的颜色,问换尽量少的刷子时,将所有的矩形涂上颜色, 一个矩形可以上颜色当且仅当在它上面的矩形都涂上了颜色

1 #include <stdio.h> 2 #include <string.h> 3 #include <iostream> 4 using namespace std ; 5 #define N 16 6 int dp[1<<N][N] ; // dp[s][i] 表示在状态s下第i个矩形上颜色的换最少的刷子 7 int state[N]; // state[i] 表示第i个矩形要涂上颜色时必须满足它上面的矩形也要涂上矩形 8 9 struct Point{ 10 int x, y ; 11 } ; 12 13 struct Rec{ 14 Point p1, p2 ; 15 int c ; 16 } r[N]; 17 18 void prework(int n){ 19 for(int i=0; i<n; i++){ 20 for(int j=0; j<n; j++){ 21 if(r[j].p2.x <= r[i].p1.x && (!(r[j].p1.y > r[i].p2.y || r[j].p2.y < r[i].p1.y))) 22 state[i] |= (1<<j) ; 23 } 24 } 25 } 26 int main(){ 27 //freopen("input.txt", "r", stdin) ; 28 int T ; 29 scanf("%d", &T) ; 30 while(T--){ 31 memset(state, 0, sizeof(state)) ; 32 int n ; 33 scanf("%d", &n) ; 34 for(int i=0; i<n; i++) scanf("%d %d %d %d %d", &r[i].p1.x, &r[i].p1.y, &r[i].p2.x, &r[i].p2.y, &r[i].c) ; 35 prework(n) ; 36 for(int i=0; i<n; i++) 37 for(int j=1; j<(1<<n); j++) 38 dp[j][i] = 20 ; 39 for(int s=1; s<(1<<n); s++){ 40 for(int i=0; i<n; i++){ 41 if(s & (1<<i)){ 42 if((s & state[i]) == state[i]){ 43 if(s == (1<<i)) dp[s][i] = 1 ; 44 else{ 45 for(int j=0; j<n; j++){ 46 if(j == i || !(s & (1<<j))) continue ; 47 dp[s][i] = min(dp[s][i], dp[s^(1<<i)][j] + (r[j].c != r[i].c)) ; 48 } 49 } 50 } 51 } 52 } 53 } 54 int ans = 20 ; 55 for(int i=0; i<n; i++) ans = min(ans, dp[(1<<n)-1][i]) ; 56 printf("%d\n", ans) ; 57 } 58 return 0 ; 59 }