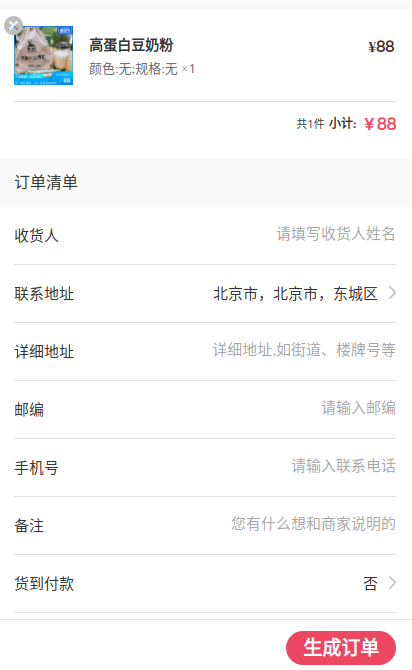
<view class="order_list">
<view class="order_top">商品清单</view>
<image class="img" src="/images/common/plus.png" bind:tap="addProduct" />
</view>
<view class="good_list">
<block wx:for="{{orderData.product_list}}" wx:key="">
<view class="good_info">
<image class="del_product" src="/images/common/del2.png" data-id="{{item.id}}" bind:tap="delProduct" />
<image class="good_img" src="{{item.ProSimg}}" />
<view class="good_detail">
<text class="good_name">{{item.ProTitle}}</text>
<text class="good_num">{{item.ProSizesName}} ×{{item.buyNumber}}</text>
</view>
<view class="price">
<view class="good_price">¥{{item.ProFxPrice}}</view>
</view>
</view>
</block>
<view class="total">
<view class="right">
<view class="right-count">共{{orderData.total_count}}件</view>
<view class="right-price-title">小计:</view>
<view class="right-price">¥{{orderData.total_price}}</view>
</view>
</view>
</view>
<view class="order_list">
<view class="order_top">订单清单</view>
</view>
<view class="form">
<view class="info_line">
<text class="info_text">收货人</text>
<input class="info_input" bindinput="input_uName" placeholder-class="info_input_holder" placeholder="请填写收货人姓名" />
</view>
<view class="info_line">
<text class="info_text">联系地址</text>
<picker class="info_picker" mode="region" bindchange="bindRegionChange" value="{{region}}" custom-item="{{customItem}}">
<text>{{region[0]}},{{region[1]}},{{region[2]}}</text>
<image class="info_picker_right" src="/images/common/more_gray.png" />
</picker>
</view>
<view class="info_line">
<text class="info_text">详细地址</text>
<input class="info_input" bindinput="input_address" placeholder-class="info_input_holder" placeholder="详细地址,如街道、楼牌号等" />
</view>
<view class="info_line">
<text class="info_text">邮编</text>
<input class="info_input" bindinput="input_postcode" placeholder-class="info_input_holder" placeholder="请输入邮编" />
</view>
<view class="info_line">
<text class="info_text">手机号</text>
<input class="info_input" bindinput="input_phone" placeholder-class="info_input_holder" placeholder="请输入联系电话" />
</view>
<view class="info_line">
<text class="info_text">备注</text>
<input class="info_input" bindinput="input_cRemark" placeholder-class="info_input_holder" placeholder="您有什么想和商家说明的" />
</view>
<view class="info_line">
<text class="info_text">货到付款</text>
<picker class="info_picker" range="{{expCodType}}" disabled="{{true}}" bindchange="expCodChange">
<text>{{expCodType[expCod]}}</text>
<image class="info_picker_right" src="/images/common/more_gray.png" />
</picker>
</view>
</view>
<view class="bottom-box">
<button class="quickpay" bind:tap="addOrder">生成订单</button>
</view>
import Storage from "../../../common/auth/Storage";
import request from "../../../common/request";
import tips from "../../../common/tips";
import { checkTel } from "../../../common/commonFunc";
Page({
getOrderData() {
request("getTempProduct", {
openid: this.openid,
}).then(({ data }) => {
this.setData({ orderData: data });
});
},
/**
* 页面的初始数据
*/
data: {
expCodType: ["否", "是"],
expCod: 0,
uName: '',
region: ['北京市', '北京市', '东城区'],
province: '北京市',
city: '北京市',
district: '东城区',
address: '',
postcode: '',
phone: '',
cRemark: '',
customItem: '',
clickFlag: true
},
bindRegionChange: function ({ detail: { value } }) {
this.setData({
region: value,
province: value[0],
city: value[1],
district: value[2]
});
},
input_uName({ detail: { value } }) {
this.setData({
uName: value
});
},
input_address({ detail: { value } }) {
this.setData({
address: value
});
},
input_postcode({ detail: { value } }) {
this.setData({
postcode: value
});
},
input_phone({ detail: { value } }) {
this.setData({
phone: value
});
},
input_cRemark({ detail: { value } }) {
this.setData({
cRemark: value
});
},
expCodChange({ detail: { value } }) {
this.setData({
expCod: value
});
},
addOrder() {
let that = this;
const {
expCod,
uName,
province,
city,
district,
address,
postcode,
phone,
cRemark,
clickFlag
} = this.data;
// console.log(this.data);
if (!clickFlag) {
return;
}
if (!uName) {
return tips.showMsg('请填写收货人姓名');
}
if (!address) {
return tips.showMsg('请填写详细地址');
}
if (!postcode) {
return tips.showMsg('请填写邮编');
}
if (!phone) {
return tips.showMsg('请填写电话号码');
}
if (!checkTel(phone)) {
return tips.showMsg('请填写正确的电话号码');
}
that.setData({
clickFlag: false
});
let openid = Storage.get().openid;
request("addOrder", {
openid,
expCod,
uName,
province,
city,
district,
address,
postcode,
phone,
cRemark: cRemark || ""
})
.then(({ data }) => {
tips.showMsg("生成成功");
// that.setData({
// clickFlag: true
// });
setTimeout(function () {
return wx.redirectTo({
url: "/pages/order/list/index"
});
}, 1500);
})
.catch(({ errdesc }) => {
that.setData({
clickFlag: true
});
return tips.showMsg(errdesc);
});
},
addProduct: function () {
wx.switchTab({
url: '/pages/product_type/index',
});
},
delProduct({
currentTarget: {
dataset: { id }
}
}) {
let openid = Storage.get().openid;
request("delTempProduct", {
openid,
id
})
.then(({ data }) => {
tips.showMsg("删除成功");
this.getOrderData();
})
.catch(({ errdesc }) => {
return tips.showMsg(errdesc);
});
},
/**
* 生命周期函数--监听页面加载
*/
onLoad: function ({ id }) {
this.orderId = id;
this.openid = Storage.get().openid;
},
/**
* 生命周期函数--监听页面显示
*/
onShow: function () {
this.getOrderData();
},
/**
* 用户点击右上角分享
*/
onShareAppMessage: function () { }
});