WPF-RaisePropertyChanged
在MVVM架构中,界面View的数据源来自ViewModel,如果想实现 从ViewModel到View 和 从View到ViewModel的双向交互,这里有一种基于MVVM框架下的方式,即使用RaisePropertyChanged,当然还有一种基于INotifyPropertyChanged接口的方式实现,这里我们主要讲下RaisePropertyChanged 实现双向交互的方式。----姜彦20180117
1.View

<Window x:Class="MVVM_Binding.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:c="clr-namespace:MVVM_Binding.ViewModel" Title="MainWindow" Height="350" Width="525"> <Grid x:Name="grid1" > <TextBox Height="23" HorizontalAlignment="Left" Margin="71,42,0,0" Name="textBox1" VerticalAlignment="Top" Width="120" Text="{Binding Path= Name,Mode=TwoWay}" /> <TextBox Height="23" HorizontalAlignment="Left" Margin="71,81,0,0" Name="textBox2" VerticalAlignment="Top" Width="120" Text="{Binding Path= Char,Mode=TwoWay}" /> <TextBox Height="23" HorizontalAlignment="Left" Margin="71,123,0,0" Name="textBox3" VerticalAlignment="Top" Width="120" Text="{Binding Path= Len}" /> <Button Content="测试" Height="23" HorizontalAlignment="Left" Margin="221,42,0,0" Name="button1" VerticalAlignment="Top" Width="75" Click="button1_Click" /> <Button Content="测试2" Height="23" HorizontalAlignment="Left" Margin="221,78,0,0" Name="button2" VerticalAlignment="Top" Width="75" Click="button2_Click" /> </Grid> </Window>
2.Model

using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace MVVM_Binding.Model { /// <summary> /// 原始数据类对象 可以不只有这三个字段 提供给ViewModel数据支持 /// </summary> public class Student { /// <summary> /// 构造函数 /// </summary> /// <param name="name"></param> public Student(string name) { this.Name = name; } /// <summary> /// 姓名 /// </summary> public string Name { get; set; } /// <summary> /// 字符 /// </summary> public string Char { get { return this.Name.Substring(2, 1); } set { Name = value; } } /// <summary> /// 长度 /// </summary> public int Len { get { return this.Name.Length; } set { Name = value.ToString(); } } } }
3.ViewModel

using GalaSoft.MvvmLight; using System.ComponentModel; using MVVM_Binding.Model; namespace MVVM_Binding.ViewModel { /// <summary> /// 交互 /// ViewModel 为界面提供数据对象 /// 界面需要什么字段,就配置什么字段 /// </summary> public class MainViewModel : ViewModelBase { private Student _student;// = new Student("Jim"); /// <summary> /// Initializes a new instance of the MainViewModel class. /// </summary> public MainViewModel(Student stu) { this._student = stu; } /// <summary> /// 原始对象数据 /// </summary> public Student Model { get { return this._student; } } /// <summary> /// 姓名 /// </summary> public string Name { get { return this._student.Name; } set { if (this._student.Name != value) { this._student.Name = value; RaisePropertyChanged(() => this._student.Name); } } } /// <summary> /// 字符 /// </summary> public string Char { get { return this._student.Char; } set { if (this._student.Char != value) { this._student.Char = value; RaisePropertyChanged(() => this._student.Char); } } } /// <summary> /// 长度 /// </summary> public int Len { get { return this._student.Len; } set { if (this._student.Len != value) { this._student.Len = value; RaisePropertyChanged(() => this._student.Len); } } } } }
4.View Controller

using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Navigation; using System.Windows.Shapes; using MVVM_Binding.ViewModel; using MVVM_Binding.Model; namespace MVVM_Binding { /// <summary> /// MainWindow.xaml 的交互逻辑 /// </summary> public partial class MainWindow : Window { private MainViewModel student = new MainViewModel(new Student("Hello")); public MainWindow() { InitializeComponent(); this.grid1.DataContext = student; } private void button1_Click(object sender, RoutedEventArgs e) { this.student.Name = "JiangYan"; } private void button2_Click(object sender, RoutedEventArgs e) { //MainViewModel stu = this.student;//你可以取消这两行的注释,修改下textbox的内容,在int i=0 这里打个断点,看看textbox更改后的数据是否传递进来了 姜彦20180117 //int i = 0; this.student = new MainViewModel(new Student("JiangYan")); this.grid1.DataContext = student; } } }
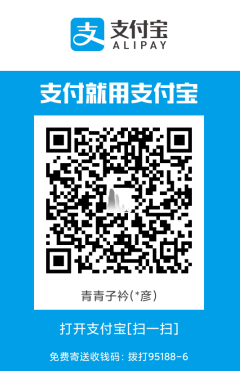
您的资助是我最大的动力!
金额随意,欢迎来赏!
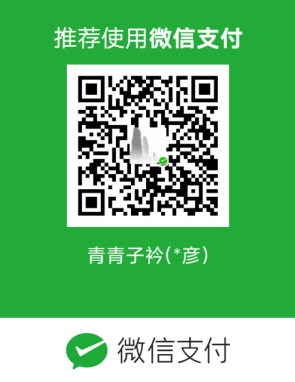
我写的东西能让你能懂,那是义务
毕竟占用了你生命中的宝贵的时间和注意力
要是你还能喜欢我的作品,那就是缘分了
如果,您希望更容易地发现我的新博客,不妨点击一下绿色通道的因为,我的写作热情也离不开您的肯定支持,感谢您的阅读,我是【青青子衿】!