今日内容概要
- 取消转义
- python内置模块 re
- re 实战练习
- collections模块(python其他数据类型)
- time与datetime模块
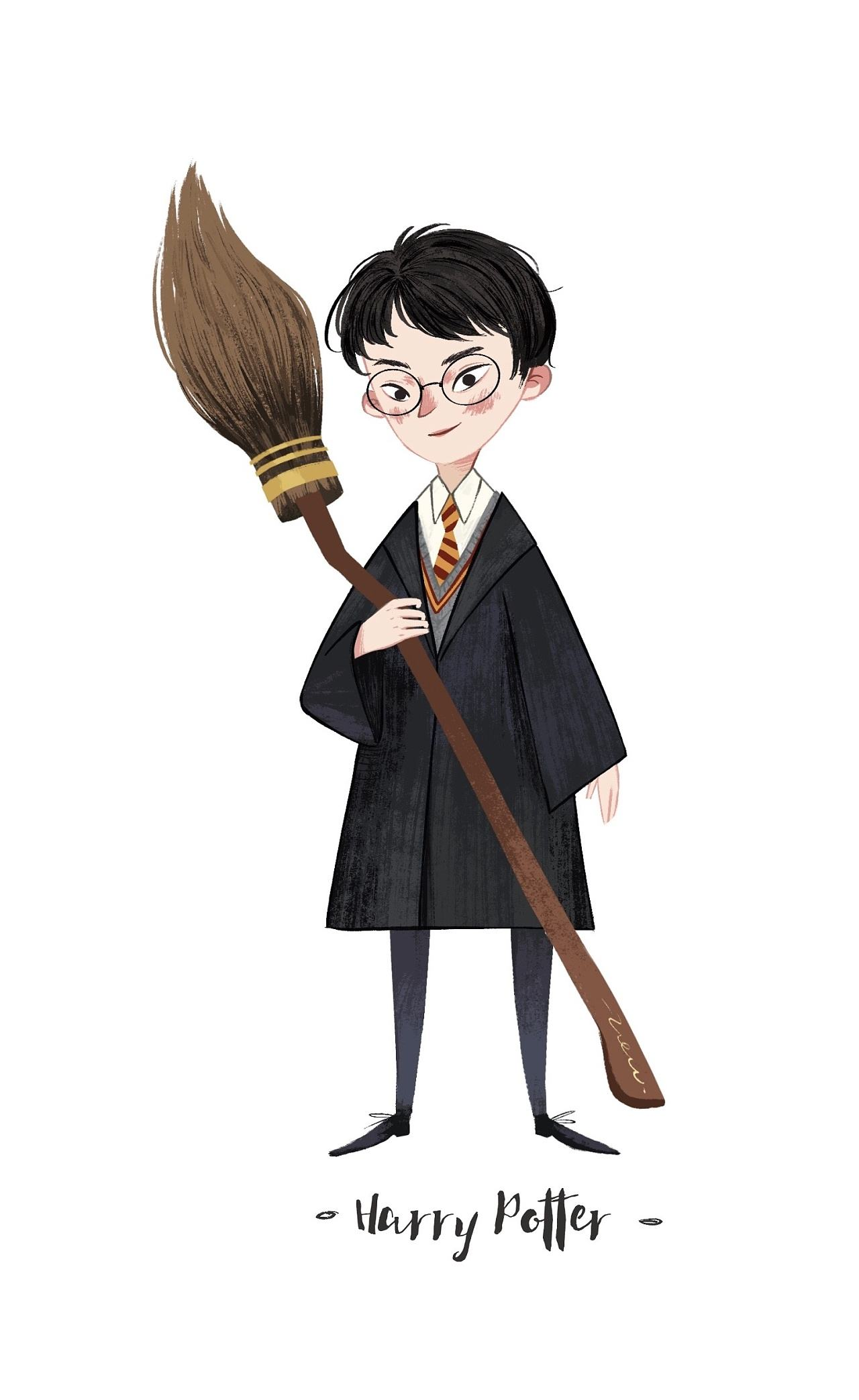
内容详细
正则表达式小练习
^[1-9]\d{13,16}[0-9x]$
# 开头一位1到9数字+13位或16位数字+0到9数字或X结尾
^[1-9]\d{14}(\d{2}[0-9x])?$
# 开头一位1到9数字+14位数字+(两位数字+0到9数字或X结尾)括号内组合可以出现一次或者不出现
^([1-9]\d{16}[0-9x]|[1-9]\d{14})$
# 开头一位1到9数字+16位数字+0到9数字或X 或者 开头一位1到9数字+14位数字
'''常见的正则表达式直接百度搜索即可'''
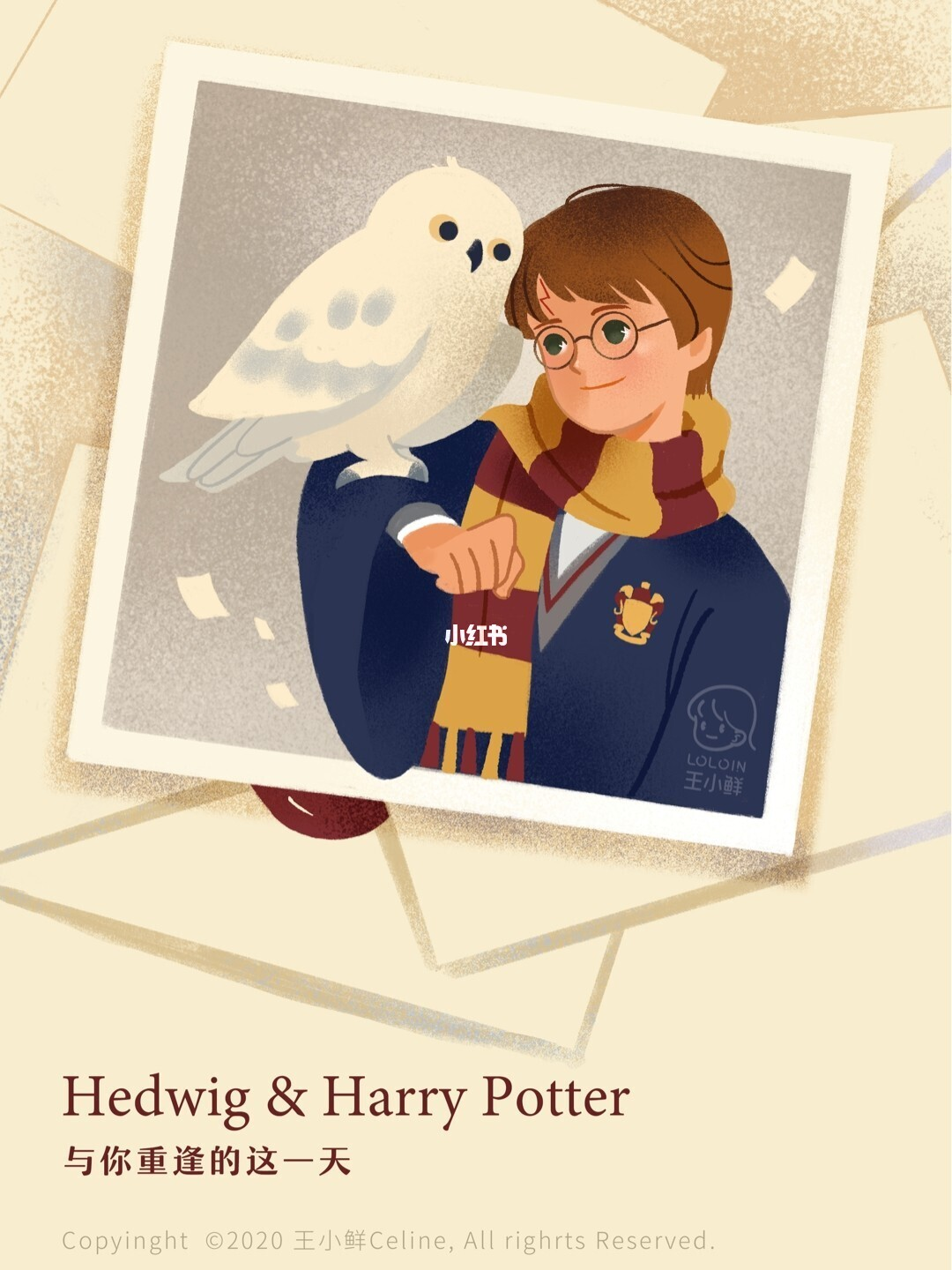
取消转义
在原生的正则表达式中取消转义 推荐使用\(每个\只能取消一个字符的转义)
在python中取消转义 推荐使用r'pass'(也可以使用\)
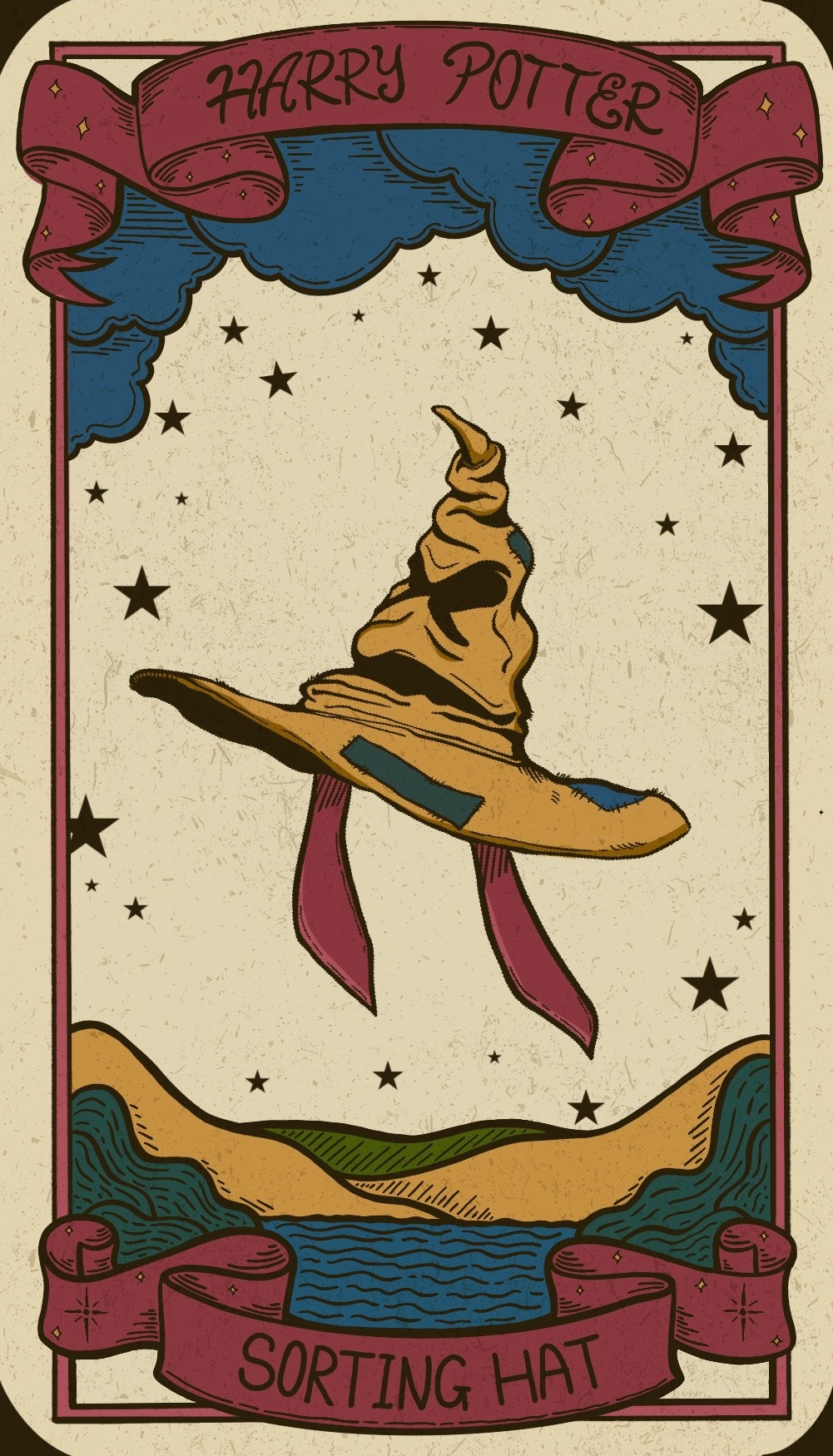
python内置模块之re模块
'''在python中想要使用正则表达式必须借助于 re 模块'''
import re
re.findall('正则表达式','带匹配的文本') # 根据正则匹配出所有符合条件的数据
res = re.findall('a','eva jason jackson') # 接收匹配的结果
print(res) # ['a', 'a', 'a'] 结果是一个列表(如果没有匹配到元素则位 空列表)
res = re.search('正则表达式','带匹配的文本') # 根据正则匹配到一个符合条件的就结束
res = re.search('a','eva jason jackson') # 接收匹配的结果
print(res) # 结果对象 <_sre.SRE_Match object; span=(2, 3), match='a'>
print(res.group()) # .group才输出结果 a
res = re.search('b','eva jason jackson')
print(res) # None 如果没有符合条件的数据 那么search返回None
print(res.group()) # 使用.group会直接报错
优化:
if res:
print(res.group())
else:
print('不好意思 没有找到')
res = re.match('a','abac') # 根据正则从头开始匹配(文本内容必须在开头匹配上)
print(res) # 结果对象 <_sre.SRE_Match object; span=(0, 1), match='a'>
print(res.group()) # .group才输出结果 a
res = re.search('b','eva jason jackson')
print(res) # None 如果没有符合条件的数据 那么match返回None
print(res.group()) # 使用.group会直接报错
优化:
if res:
print(res.group())
else:
print('不好意思 没有找到')
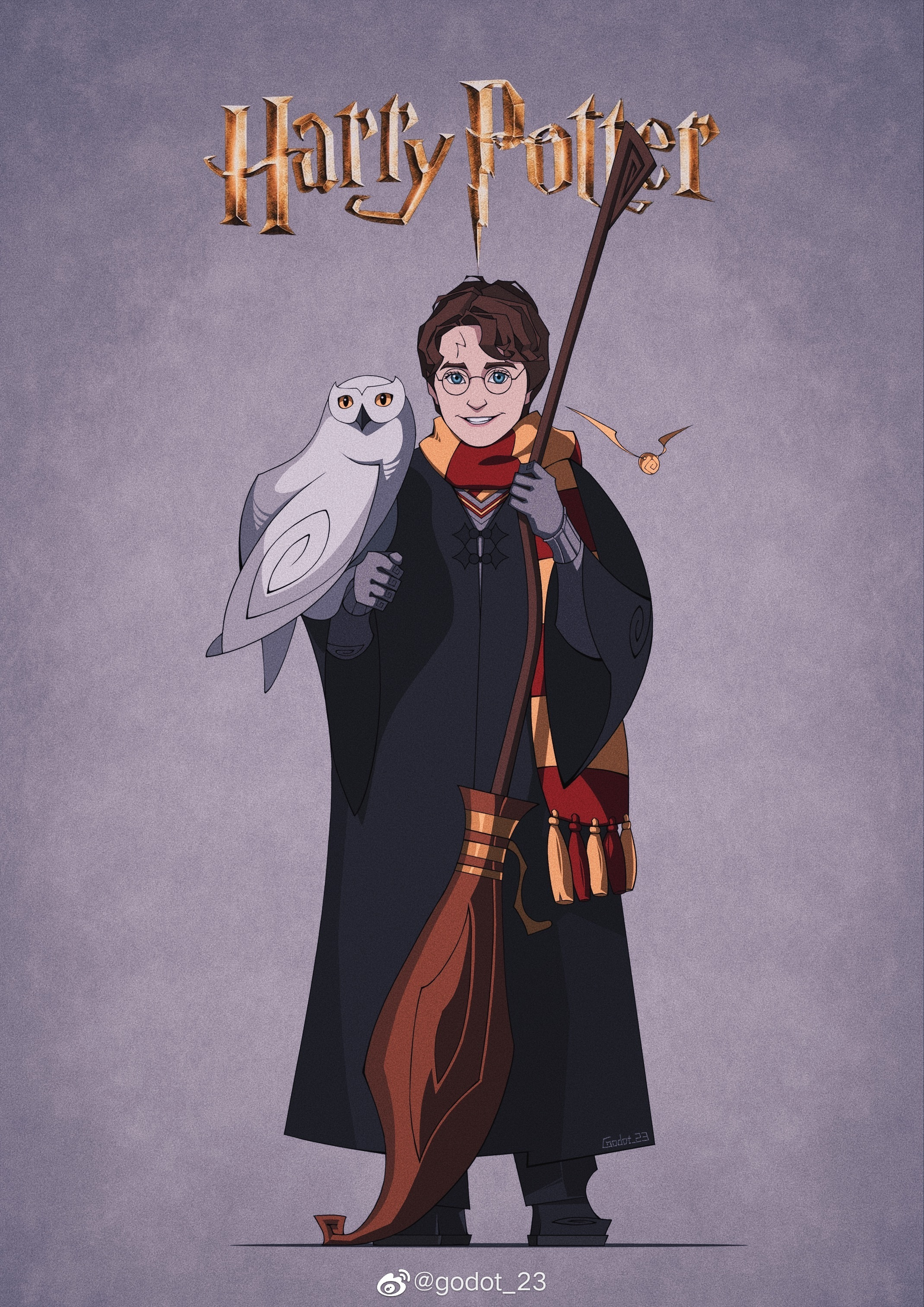
re模块其他方法
import re
res = re.split('[ab]','abcd') # 先按'a'分割得到''和'bcd',在对''和'bcd'分别按'b'分割
print(res) # ['', '', 'cd']
'''类似于字符串类型的replace方法'''
res = re.sub('\d','H', 'eva3jason4yuan4', 1) # 替换正则匹配到的一位纯数字内容
print(res) # evaHjason4yuan4
res = re.sub('\d','H', 'eva3jason4yuan4') # 不写默认替换所有
print(res) # evaHjason4yuan4
'''返回元组 并提示替换了几处'''
res = re.subn('\d', 'H', 'eva3jason4yuan4', 1)
print(res) # ('evaHjason4yuan4', 1)
res = re.subn('\d', 'H', 'eva3jason4yuan4')
print(res) # ('evaHjasonHyuanH', 3)
'''compile'''
# 把正则先预编译好,重复使用时就不再需要编译,直接匹配
regexp_obj = re.compile('\d+')
res = regexp_obj.search('absd213j1hjj213jk')
res1 = regexp_obj.match('123hhkj2h1j3123')
res2 = regexp_obj.findall('1213k1j2jhj21j3123hh')
print(res.group()) # 213
print(res1.group()) # 123
print(res2) # ['1213', '1', '2', '21', '3123']
"""
根据正则匹配出所有符合条件的数据 类似于findall
但是在数据过大时 比findall更加节省空间
"""
res = re.finditer('\d+','ashdklah21h23kj12jk3klj112312121kl131')
print([i.group() for i in res]) # ['21', '23', '12', '3', '112312121', '131']
'''分组优先展示'''
res = re.search('^[1-9](\d{14})(\d{2}[0-9x])?$','110105199812067023')
print(res)
print(res.group()) # 110105199812067023
print(res.group(1)) # 小括号代表分组 10105199812067
print(res.group(2)) # 小括号代表分组 023
# findall针对分组的优先展示(无命名分组)
res = re.findall("^[1-9]\d{14}(\d{2}[0-9x])?$",'110105199812067023')
print(res) # ['023'] 只会展示分组内的结果
# 通过添加?: 会取消优先展示分组 直接展示匹配的所有结果
res1 = re.findall("^[1-9]\d{14}(?:\d{2}[0-9x])?$",'110105199812067023')
print(res1) # ['110105199812067023']
# findall针对分组的优先展示(有名分组)
命名:
?P<name>
re.search('^[1-9](?P<xxx>\d{14})(?P<ooo>\d{2}[0-9x])?$','110105199812067023')
print(res.group()) # 110105199812067023
print(res.group(2)) # 023 无名分组的取值方式(索引取)
print(res.group('xxx')) # 10105199812067 命名 ?P<xxx>
print(res.group('ooo')) # 023 命名 ?P<ooo>
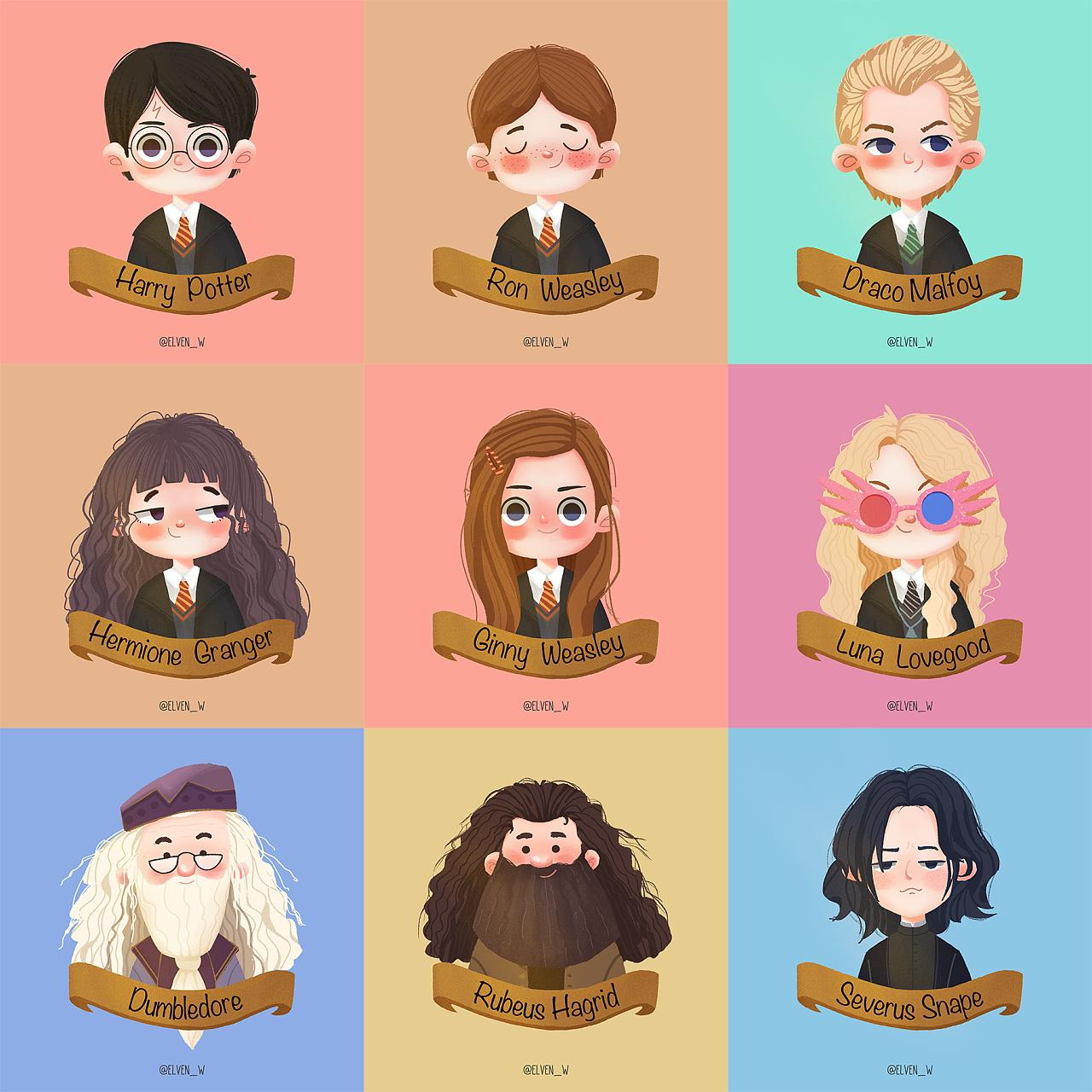
正则实战案例
# 读取红牛redbull官网所有分公司名称、地址、邮箱、电话
1.到官网公司信息页 通过源代码复制全部内容到文本
http://www.redbull.com.cn/about/branch
2.读取待匹配的数据
with open(r'a.txt', 'r', encoding='utf8') as f:
data = f.read()
# 利用正则匹配数据
# 分公司名称
title_list = re.findall('<h2>(.*?)</h2>', data)
# 分公司地址
address_list = re.findall("<p class='mapIco'>(.*?)</p>", data)
# 分公司邮箱
email_list = re.findall("<p class='mailIco'>(.*?)</p>", data)
# 分公司电话
phone_list = re.findall("<p class='telIco'>(.*?)</p>", data)
# 用zip拉链将结果组合成想要的类型
res = zip(title_list, address_list, email_list, phone_list)
for data_tuple in res:
print("""
公司名称:%s
公司地址:%s
公司邮箱:%s
公司电话:%s
""" % (data_tuple[0], data_tuple[1], data_tuple[2], data_tuple[3]))
结果:
公司名称:红牛杭州分公司
公司地址:杭州市上城区庆春路29号远洋大厦11楼A座
公司邮箱:310009
公司电话:0571-87045279/7792
...
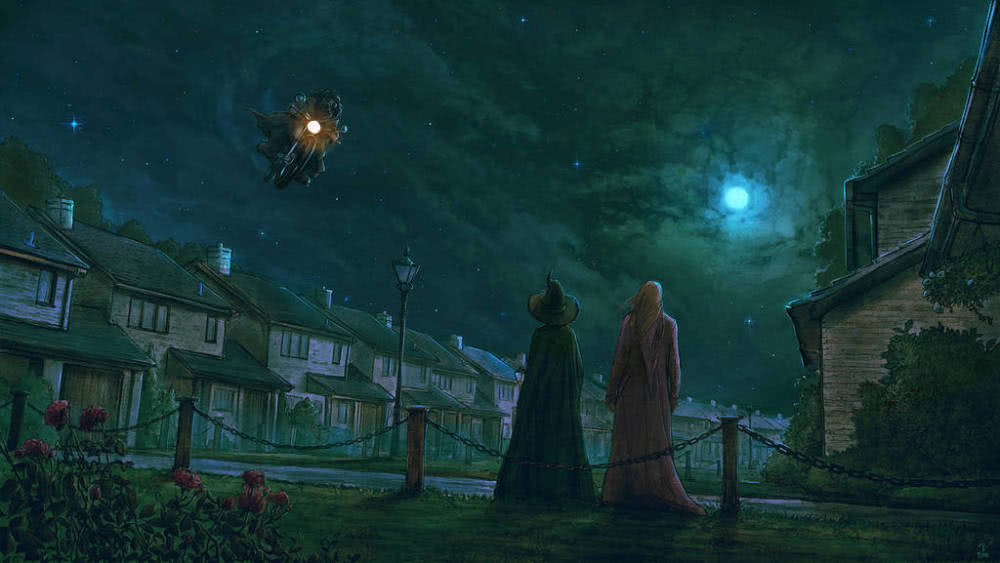
collections模块
'''该模块内部只要提供了一些高阶(进阶)的数据类型'''
1.namedtuple(具名元组)
from collections import namedtuple
"""
语法格式
namedtuple('名称',[名字1,名字2,...])
namedtyple('名称','名称1 名字2 ...')
"""
point = namedtuple('坐标', ['x', 'y']) # 先在具名元组内放条件
res = point(11, 22) # 传条件到具名元组中
print(res) # 坐标(x=11, y=22)
print(res.x) # 11
print(res.y) # 22
point = namedtuple('坐标', 'x y z')
res = point(11, 22, 33)
print(res) # 坐标(x=11, y=22, z=33)
print(res.x) # 11
print(res.y) # 22
print(res.z) # 33
card = namedtuple('扑克', '花色 点数')
card1 = card('♠', 'A')
card2 = card('♥', 'K')
print(card1) # 扑克(花色='♠', 点数='A')
print(card1.花色) # ♠
print(card1.点数) # A
2.队列
# 队列模块
import queue # 内置队列模块:FIFO
# 初始化队列
q = queue.Queue()
# 往队列中添加元素
q.put('first') # 先进
q.put('second')
q.put('third')
# 从队列中获取元素
print(q.get()) # first 先进先出
print(q.get()) # second
print(q.get()) # third
print(q.get()) # 值取完了会原地等待 一旦有新的元素进入立刻输出
3.双端队列
# 导入模块
from collections import deque
q = deque([11, 22, 33])
q.append(44) # 从右边添加
q.appendleft(55) # 从左边添加
print(q) # deque([55, 11, 22, 33, 44])
print(q.pop()) # 44 从右边取值
print(q.popleft()) # 55 从左边取值
4.有序字典
normal_dict = dict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])
print(normal_dict)
'''{'name': 'jason', 'pwd': 123, 'hobby': 'study'} pycharm会主动排序'''
"""
但是在python解释器中 字典实际上时无序的
结果就为:{'hobby': 'study', 'pwd': 123, 'name': 'jason'}
"""
from collections import OrderedDict
order_dict = OrderedDict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])
print(order_dict)
"""结果为:OrderedDict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])"""
order_dict['xxx'] = 111 # 新增键值对
print(order_dict)
"""
OrderedDict模块中
结果有序
OrderedDict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])
"""
normal_dict['yyy'] = 222 # 新增键值对
print(normal_dict)
"""
但是在python解释器中 字典实际上时无序的
{'hobby': 'study', 'pwd': 123, 'yyy': 222, 'name': 'jason'}
"""
5.默认值字典
from collections import defaultdict
values = [11, 22, 33,44,55,66,77,88,99,90]
# 列表中元素大于60放到K1 小于60放到K2
my_dict = defaultdict(list) # 同样是定义空列表
for value in values:
if value>60:
my_dict['k1'].append(value)
else:
my_dict['k2'].append(value)
print(my_dict)
'''defaultdict(<class 'list'>, {'k2': [11, 22, 33, 44, 55], 'k1': [66, 77, 88, 99, 90]})'''
6.计数器
res = 'abcdeabcdabcaba'
# 统计字符串中每个元素出现的次数
# 写法1
new_dict = {}
for i in res:
if i not in new_dict:
new_dict[i] = 1
else:
new_dict[i] += 1
print(new_dict) # {'a': 5, 'b': 4, 'c': 3, 'd': 2, 'e': 1}
# 写法2
from collections import Counter # 计数器
ret = Counter(res)
print(ret) # Counter({'a': 5, 'b': 4, 'c': 3, 'd': 2, 'e': 1})
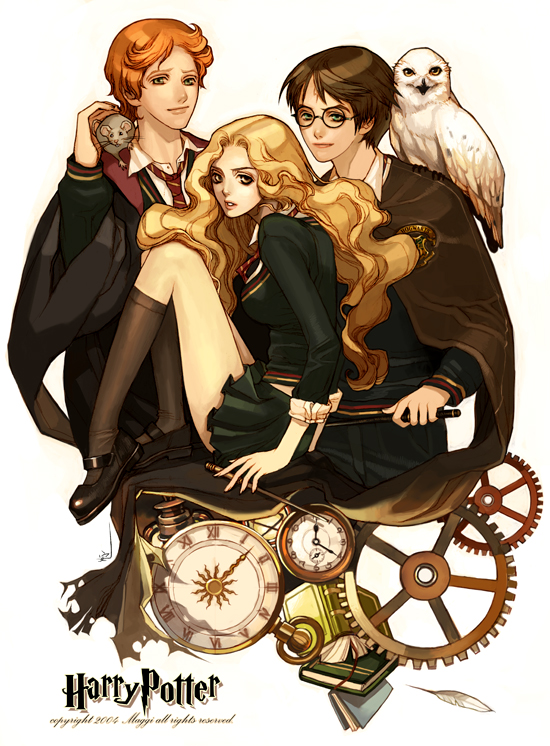
time模块
"""
时间三种表现形式
1.时间戳(秒数)
2.结构化时间(一般是给机器看的)
3.格式化时间(一般是给人看的)
三种时间可以相互转换的!!!
"""
语法:
1.time.sleep() # 原地阻塞(等待)指定的秒数
2.time.time() # 获取时间戳时间
import time
# 格式化时间
print(time.strftime('%Y-%m-%d')) # 2021-11-25 当时日期
print(time.strftime('%Y-%m-%d %H:%M:%S')) # 2021-11-25 18:55:54 当前日期时分秒
print(time.strftime('%Y-%m-%d %X')) # 2021-11-25 18:55:54 当前日期时分秒
print(time.localtime())
time.struct_time(
tm_year=2021,
tm_mon=11,
tm_mday=25,
tm_hour=11,
tm_min=51,
tm_sec=25,
tm_wday=3,
tm_yday=329,
tm_isdst=0)
print(time.time())
print(time.gmtime())
print(time.localtime())
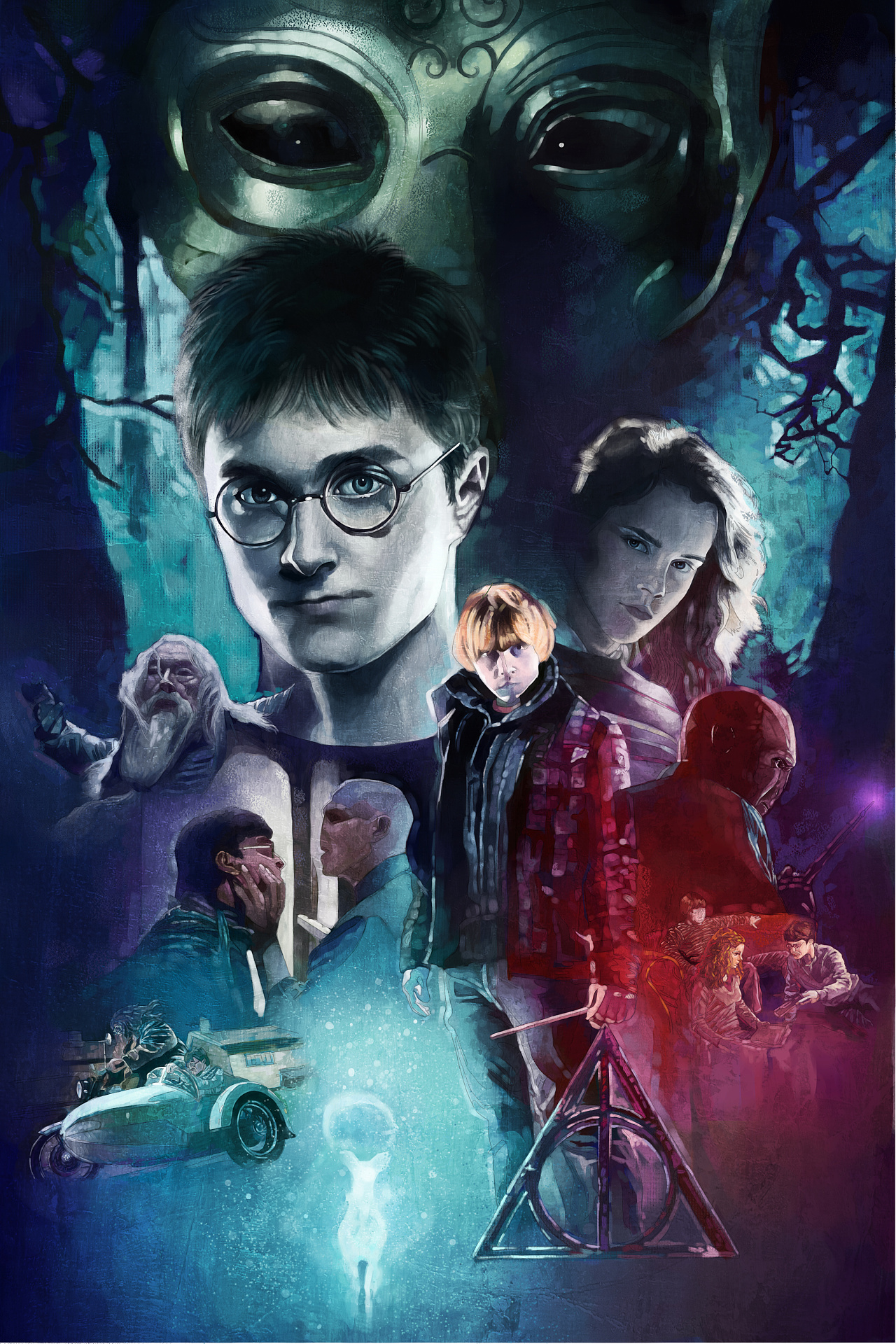
datetime模块
"""
date年月日
datetime年月日时分秒
time时分秒
"""
import datetime
print(datetime.date.today()) # 2021-11-25
print(datetime.datetime.today()) # 2021-11-25 19:09:40.341481
res = datetime.datetime.today()
print(res.year) # 2021
print(res.month) # 11
print(res.day) # 25
print(res.weekday()) # 获取星期(weekday星期是0-6) 0表示周一
print(res.isoweekday()) # 获取星期(weekday星期是1-7) 1表示周一
"""时间差(timedelta)"""
ctime = datetime.datetime.today()
time_tel = datetime.timedelta(days=3)
print(ctime) # 2021-11-25 19:11:41.507849
print(ctime - time_tel) # 2021-11-22 19:11:41.507849
print(ctime + time_tel) # 2021-11-28 19:11:41.507849
"""
日期对象 = 日期对象 +/- timedelta对象
timedelta对象 = 日期对象 +/- 日期对象
"""
ret = ctime + time_tel
print(ret - ctime) # 3 days, 0:00:00
print(ctime - ret) # -3 days, 0:00:00
# 练习 计算出生有多少天
birthday = datetime.date(2000, 11, 11)
now_date = datetime.date.today()
days = birthday - now_date
print('距离出生已经{}天'.format(days))
'''距离出生已经-7684 days, 0:00:00天'''
# UTC时间与我们国家的东八区时间差 八个小时
print(datetime.datetime.now()) # 2021-11-25 19:23:12.833902
print(datetime.datetime.utcnow()) # 2021-11-25 11:23:12.833902
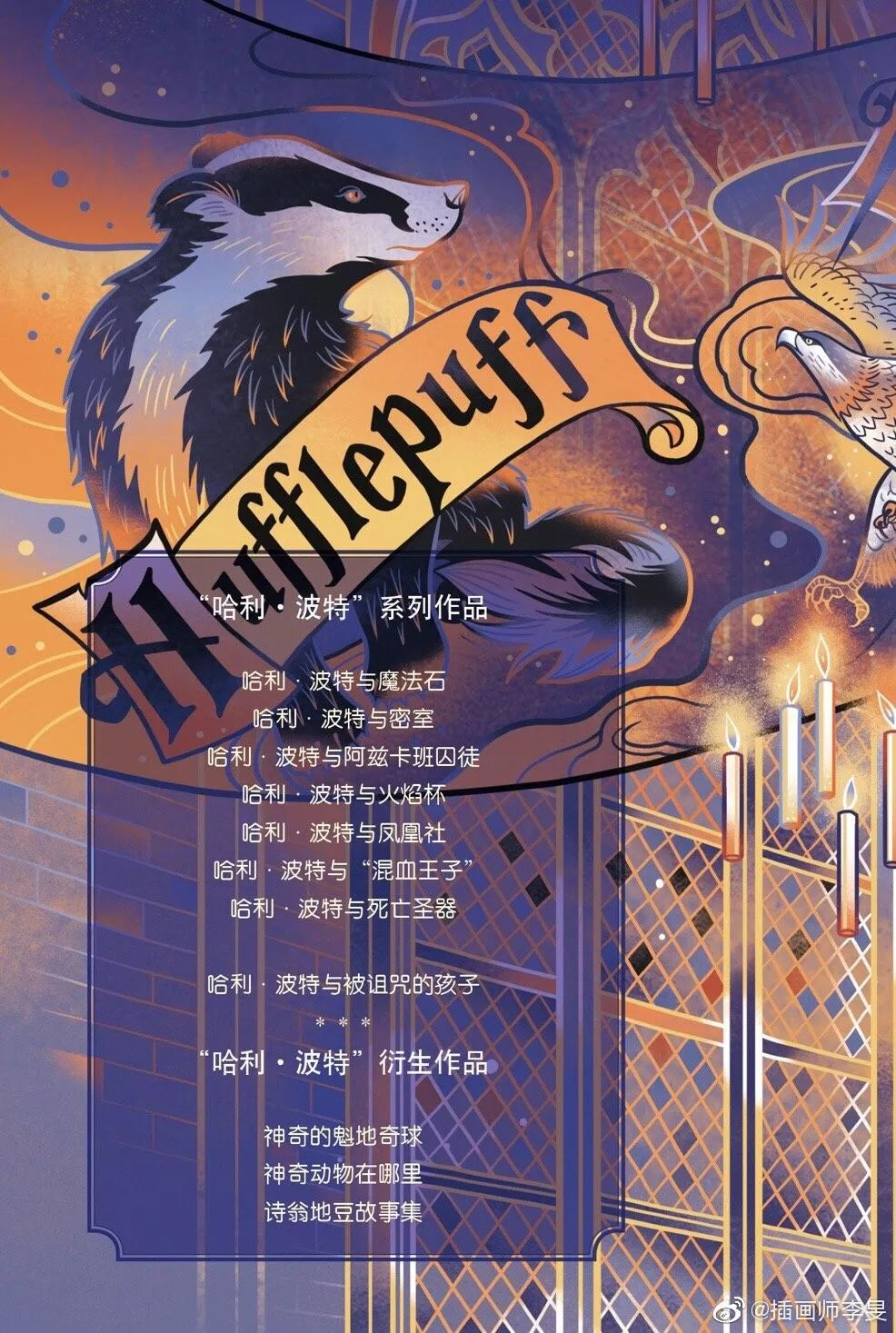