2.老生常谈:Strings and String Builders
书目:MCTS 70-536: TS: .NET Framework 2.0-Application Development Foundation
章节:Chapter 1: Framework Fundamentals -- Lesson 2: Using Common Reference Types -- Strings and String Builders
原文内容:
Strings of type System.String are immutable in .NET. That means any change to a string
causes the runtime to create a new string and abandon the old one. That happens
invisibly, and many programmers might be surprised to learn that the following code
allocates four new strings in memory:
// C#
string s;
s = "wombat"; // "wombat"
s += " kangaroo"; // "wombat kangaroo"
s += " wallaby"; // "wombat kangaroo wallaby"
s += " koala"; // "wombat kangaroo wallaby koala"
Console.WriteLine(s);
// C#
System.Text.StringBuilder sb = new System.Text.StringBuilder(30);
sb.Append("wombat"); // Build string.
sb.Append(" kangaroo");
sb.Append(" wallaby");
sb.Append(" koala");
string s = sb.ToString(); // Copy result to string.
Console.WriteLine(s);
Another subtle but important feature of the String class is that it overrides operators
from System.Object. Table 1-4 lists the operators the String class overrides.
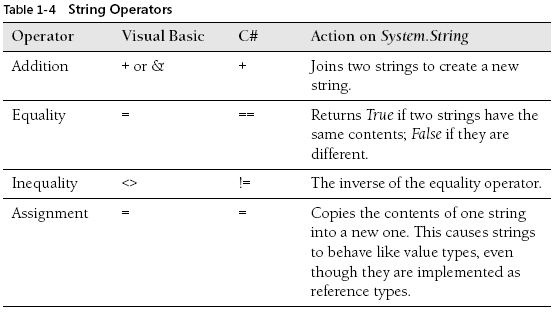
参考文章:
http://www.codeproject.com/Purgatory/string.asp
http://channel9.msdn.com/ShowPost.aspx?PostID=14932
章节:Chapter 1: Framework Fundamentals -- Lesson 2: Using Common Reference Types -- Strings and String Builders
原文内容:
Strings of type System.String are immutable in .NET. That means any change to a string
causes the runtime to create a new string and abandon the old one. That happens
invisibly, and many programmers might be surprised to learn that the following code
allocates four new strings in memory:







Only the last string has a reference; the other three will be disposed of during garbage
collection. Avoiding these types of temporary strings helps avoid unnecessary garbage
collection, which improves performance. There are several ways to avoid temporary
strings:
1.Use the String class’s Concat, Join, or Format methods to join multiple items in a
single statement.
2.Use the StringBuilder class to create dynamic (mutable) strings.
The StringBuilder solution is the most flexible because it can span multiple statements.
The default constructor creates a buffer 16 bytes long, which grows as needed.
You can specify an initial size and a maximum size if you like. The following code demonstrates
using StringBuilder:








Another subtle but important feature of the String class is that it overrides operators
from System.Object. Table 1-4 lists the operators the String class overrides.
参考文章:
http://www.codeproject.com/Purgatory/string.asp
http://channel9.msdn.com/ShowPost.aspx?PostID=14932