ActiveRecord学习笔记(五):处理继承关系
本文主要介绍了如何使用Castle.ActiveRecord来处理继承关系。
本文涉及两个实体类:基类(User)、子类(Employee)。以下是类图:
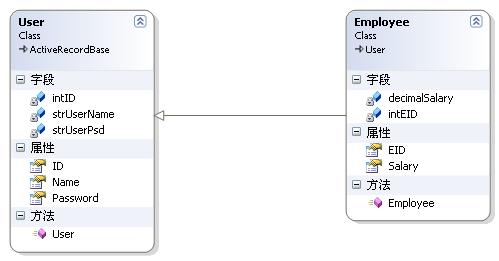
本文主要内容:
1.编写数据库脚本
2.JoinedBase和JoinedKey属性说明
3.编写实体类
4.编写调用代码
一、编写数据库脚本
其实本文涉及的数据表在前面的笔记中都出现过!
Create Table Users
(
ID int identity(1,1) primary key,
LoginName Varchar(50) not null,
Password Varchar(20) not null
)

Create Table Employees
(
ID int primary key,
Salary money
)
二、JoinedBase和JoinedKey属性说明
JoinedBase属性:该属性是ActiveRecord属性子属性,用于基类中;
JoinedKey性性:该属性用在子类中,用于代替PrimaryKey的位置;
三、编写实体类
基类:User.cs
///jailusd@hotmail.com
///2006-09-24

using System;
using System.Collections.Generic;
using System.Text;

using Castle.ActiveRecord;

namespace Inherit
{
[ActiveRecord("Users"),JoinedBase] //JoinedBase
public class User : ActiveRecordBase
{
private int intID;
private string strUserName;
private string strUserPsd;

public User()
{
intID = 0;
strUserName = string.Empty;
strUserPsd = string.Empty;
}
[PrimaryKey(PrimaryKeyType.Identity,"ID")]
public int ID
{
get
{
return intID;
}
set
{
intID = value;
}
}

/// <summary>
/// 用户名
/// </summary>
[Property("LoginName")]
public string Name
{
get
{
return strUserName;
}
set
{
strUserName = value;
}
}

/// <summary>
/// 用户密码
/// </summary>
[Property("Password")]
public string Password
{
get
{
return strUserPsd;
}
set
{
strUserPsd = value;
}
}
}
}
子类:Employee.cs
///jailusd@hotmail.com
///2006-09-24

using System;
using System.Collections.Generic;
using System.Text;

using Castle.ActiveRecord;

namespace Inherit
{
[ActiveRecord("Employees")]
public class Employee : User
{
private int intEID;
private decimal decimalSalary;

public Employee()
{
intEID = 0;
decimalSalary = 0;
}

[JoinedKey("ID")] //JoinedKey代替PrimaryKey的位置
public int EID
{
get
{
return intEID;
}
set
{
intEID = value;
}
}
/// <summary>
/// 薪水
/// </summary>
[Property]
public decimal Salary
{
get
{
return decimalSalary;
}
set
{
decimalSalary = value;
}
}
}
}
四、编写调用代码(只列出添加Employee的代码)
private void button2_Click(object sender, EventArgs e)
{
IConfigurationSource source = System.Configuration.ConfigurationManager.GetSection("activerecord") as IConfigurationSource;
ActiveRecordStarter.Initialize(source, typeof(Inherit.Employee), typeof(Inherit.User));

//使用事务处理
using (TransactionScope tran = new TransactionScope())
{
Inherit.Employee objEmployee = new Inherit.Employee();

objEmployee.Name = "jailu";
objEmployee.Password = "123456789";
objEmployee.Salary = 1000;

objEmployee.Save();
}
}
本文涉及两个实体类:基类(User)、子类(Employee)。以下是类图:
本文主要内容:
1.编写数据库脚本
2.JoinedBase和JoinedKey属性说明
3.编写实体类
4.编写调用代码
一、编写数据库脚本
其实本文涉及的数据表在前面的笔记中都出现过!












二、JoinedBase和JoinedKey属性说明
JoinedBase属性:该属性是ActiveRecord属性子属性,用于基类中;
JoinedKey性性:该属性用在子类中,用于代替PrimaryKey的位置;
三、编写实体类
基类:User.cs







































































子类:Employee.cs





















































四、编写调用代码(只列出添加Employee的代码)
















