饿了吗开源组件库Element模拟购物车系统
传统的用html+jquery来实现购物车系统要非常的复杂,但是购物车系统完全是一个数据驱动的系统,因此采用诸如Vue.js、angular.js这些框架要简单的多。饿了吗开源的组件库Element是基于Vue.js 2.0实现的,该组件库封装了开发中需要的各种组件,并且提供了友好的API文档供开发者查看,下面就是我用Element实现的一个简单的购物车系统。(https://github.com/iwideal/MyGit/tree/master/HTML/shopping-cart)
首先,我们用Vue.js推荐的命令行工具来搭建项目骨架。
# 创建一个基于 webpack 模板的新项目
$ vue init webpack shopping-cart
# 安装依赖,走你
$ cd shopping-cart
$ npm install
$ npm run dev
这时候,生成的项目骨架如图:

这时候,我们要像maven一样,给项目添加依赖,在package.json文件中添加Element依赖:
"dependencies": {
"element-ui": "^1.1.6",
"vue": "^2.1.0"
},
添加完依赖之后,这是我们可以在项目中使用依赖了,在main.js文件中,导入ElementUI:
// The Vue build version to load with the `import` command // (runtime-only or standalone) has been set in webpack.base.conf with an alias. import Vue from 'vue' import ElementUI from 'element-ui' import 'element-ui/lib/theme-default/index.css' import App from './App.vue' Vue.use(ElementUI) /* eslint-disable no-new */ new Vue({ el: '#app', template: '<App/>', components: { App } })
重新安装依赖,走你:
$ cd shopping-cart
$ npm install
$ npm run dev
这时,我们在App.vue中,写入我们的购物车代码。
预览一下,整体效果如下:
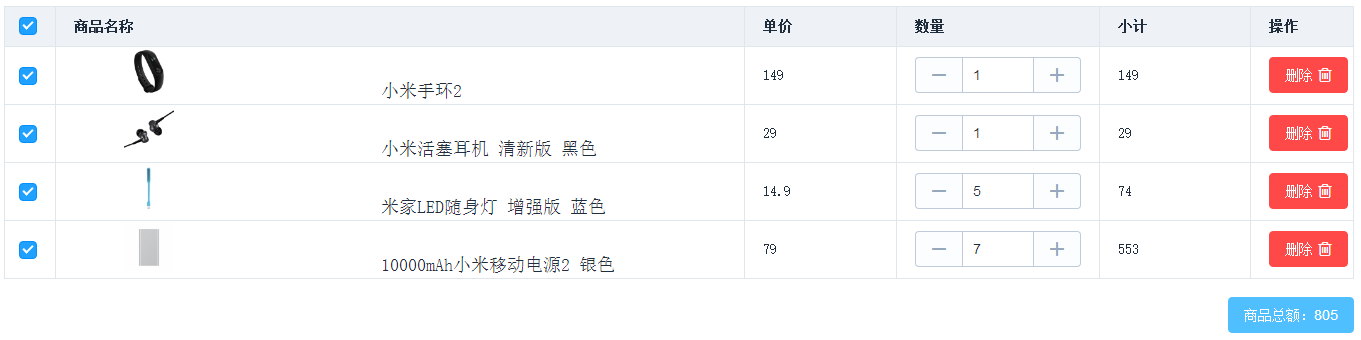
一、创建购物车骨架,即复杂型的表格。我们这时可以从Element的官网(http://element.eleme.io/#/zh-CN/component/table)copy过来模板,我们选择带有checkbox的表格:
<template> <div> <el-table :data="tableData" border style="width: 100%" @selection-change="selected"> <el-table-column type="selection" width="50"> </el-table-column> <el-table-column label="商品名称" width="680"> <template scope="scope"> <div style="margin-left: 50px"> <img :src="scope.row.goods.img" style="height: 50px;width: 50px"> <span style="font-size: 18px;padding-left: 200px;">{{scope.row.goods.descript}}</span> </div> </template> </el-table-column> <el-table-column label="单价" width="150" prop="price"> </el-table-column> <el-table-column label="数量" width="200"> <template scope="scope"> <div> <el-input v-model="scope.row.number" @change="handleInput(scope.row)"> <el-button slot="prepend" @click="del(scope.row)"><i class="el-icon-minus"></i></el-button> <el-button slot="append" @click="add(scope.row)"><i class="el-icon-plus"></i></el-button> </el-input> </div> </template> </el-table-column> <el-table-column label="小计" width="150" prop="goodTotal"> </el-table-column> <el-table-column label="操作"> <template scope="scope"> <el-button type="danger" @click="handleDelete(scope.$index, scope.row)"> 删除<i class="el-icon-delete2 el-icon--right"></i> </el-button> </template> </el-table-column> </el-table> <br> <el-button type="info" style="float: right">{{"商品总额:" + moneyTotal}}</el-button> </div> </template>
购物车骨架搭建好之后,我们就可以向里面插入数据了:
data() { return { tableData: [{ goods:{ img:'http://i1.mifile.cn/a1/pms_1474859997.10825620!80x80.jpg', descript:'小米手环2', }, price:149, number:1, goodTotal:149, },{ goods:{ img:'http://i1.mifile.cn/a1/pms_1482321199.12589253!80x80.jpg', descript:'小米活塞耳机 清新版 黑色', }, price:29, number:1, goodTotal:29, },{ goods:{ img:'http://i1.mifile.cn/a1/pms_1468288696.74437986!80x80.jpg', descript:'米家LED随身灯 增强版 蓝色', }, price:14.9, number:1, goodTotal:14.9, },{ goods:{ img:'http://i1.mifile.cn/a1/pms_1476688193.46995320.jpg?width=140&height=140', descript:'10000mAh小米移动电源2 银色', }, price:79, number:1, goodTotal:79, }], moneyTotal:0, multipleSelection:[], } }
这时候,我们需要对购物车中的各种事件做处理,包括删除商品、增加(减少)商品数量、选中商品等:
methods: { handleDelete(index, row) { this.$confirm('确定删除该商品?', '提示', { confirmButtonText: '确定', cancelButtonText: '取消', type: 'warning' }).then(() => { //删除数组中指定的元素 this.tableData.splice(index,1); this.$message({ type: 'success', message: '删除成功!' }); }).catch(() => { this.$message({ type: 'info', message: '已取消删除' }); }); }, handleInput:function(value){ if(null==value.number || value.number==""){ value.number=1; } value.goodTotal=(value.number*value.price).toFixed(2);//保留两位小数 //增加商品数量也需要重新计算商品总价 this.selected(this.multipleSelection); }, add:function(addGood){ //输入框输入值变化时会变为字符串格式返回到js //此处要用v-model,实现双向数据绑定 if(typeof addGood.number=='string'){ addGood.number=parseInt(addGood.number); }; addGood.number+=1; }, del:function(delGood){ if(typeof delGood.number=='string'){ delGood.number=parseInt(delGood.number); }; if(delGood.number>1){ delGood.number-=1; } }, //返回的参数为选中行对应的对象 selected:function(selection){ this.multipleSelection=selection; this.moneyTotal=0; //此处不支持forEach循环,只能用原始方法了 for(var i=0;i<selection.length;i++){ //判断返回的值是否是字符串 if(typeof selection[i].goodTotal=='string'){ selection[i].goodTotal=parseInt(selection[i].goodTotal); }; this.moneyTotal+=selection[i].goodTotal; } },