java中数组和字符串
数组
数组的声明方式: 类型[] 变量;
数组的创建方式: new 类型[数组长度]
数组的简单声明并且赋值
// 声明一个数组,它的长度是3
String[] arrs= new String[3];
arrs[0]="张三";
arrs[1]="李四";
// 访问数组的值
System.out.println(arrs[0]); 输出的是张三
// 获取当前数组的长度 使用length方法
arrs.length
字符串
字符串里面就是一个一个的字符;
字符又是一个一个的字节
字符串里面就是一个一个的字符;
// 字符串是由一个一个的字节组成的
char[] c= {'a','中','国'};
String str = new String(c);
System.out.println(str); // 输出的是:a中国
byte[] b = {-28,-72,-83,-27,-101,-67};
String str1 = new String(b,"UTF-8");
System.out.println(str1); // 输出的是:中国
如何表示双引号 -需要使用转义 \
String str1 = "\""
System.out.println(str1); // 输出的是:"
字符串拼接
字符串拼接有两种方式
第一种是:使用 + 表示连接或者相加; 左右两侧都是数字就是相加
第二中是: s.concat(s2)
判断字符串是否相等 equals
// 判断两个字符串内容是否相等
String s1 = "a";
String s2="a";
System.out.println(s1.equals(s2)); // 输出true
判断两个字符串内容是否相等忽略大小写
// 判断两个字符串内容是否相等忽略大小写
String s1 = "a";
String s2="A";
System.out.println(s1.equalsIgnoreCase(s2)); // 输出true
字符串的比较
i = 正数; a>b
i = 负数; a<b
i=0; a=b
字符串比较大小实际上是比较:ASCII编码
String a= "A";
String b = "B";
int c = a.compareTo(b);
System.out.println(c); // -1 说明 a < b
String a= "2";
String b = "3";
int c = a.compareTo(b);
System.out.println(c); // -1 说明 a < b
字符串是怎么比较的
byte b1 = (byte)'A'; // 将字符串类型强制转化为byte类型
byte b2 =(byte)'B';
System.out.println("A:"+ b1); // 65
System.out.println("B:"+ b2); // 66
int i = b1.compareTo(b2); // 这个结果整好是他们之间的差值 65-66
System.out.println(i); // 输出-1
字符串的截取 - substring[)
String str="hello";
// [)包含起始位,不包含结束位
System.out.println(str.substring(0,3));
如果只传递一个参数,表示从指定的位置开始,一直到字符串结束
// 如果只有一个参数,表示从指定位置一直到字符串末结束
System.out.println(str.substring(3)); // lo
将字符串变为数组 - split
String str="hello Word";
// 将字符串变为数组;以空格的形式切割
String[] arrs = str.split(" ");
System.out.println(arrs.length); // 2
System.out.println(arrs[0]); // hello
System.out.println(arrs[1]); // Word
循环数组的另外一种方式 for(String 每一项:被循环的数组){}
String[] arrs = new String[2];
arrs[0]="hello";
arrs[1]="Word";
for(String cont:arrs){
System.out.println(cont);
}
去除字符串首尾的空格 trim
// 去除字符串首尾的空格
String str=" helloWord ";
System.out.println(str.trim());
字符串替换
String str="hello python";
System.out.println(str.replace("python","PHP")); // hello PHP
如果一个字符串中有两处python;也会将第二处替换
String str="hello python python";
System.out.println(str.replace("python","PHP")); // hello PHP PHP
按照指定的规则进行替换
String str="hello python 世界";
System.out.println(str.replaceAll("hello|python","你好"));
会将hello或者python都替换成为 你好; 最后输出的是:你好 你好 世界
将大写转化为小写
String str="HELLO";
System.out.println(str.toLowerCase()); // hello
将小写转化为大写
String str="hello";
System.out.println(str.toUpperCase());
字符串查找- charAt
// charAt可以根据索引,查找字符串中指定位置的字符
String str="hello";
System.out.println(str.charAt(1)); // 输出e
字符串查找- indexOf
用于获取数据在字符串中第1次出现的位置
String str="hello World";
System.out.println(str.indexOf("hello")); // 0
String str1="World hello World";
System.out.println(str1.indexOf("World")); // 0
String str2="hello World";
System.out.println(str2.indexOf("World")); // 6
字符串查找 - lastIndexOf
lastIndexOf:用于获取数据在字符串最后一次出现的位置
String str1="henihe";
System.out.println(str1.lastIndexOf("e"));
字符串包含 - contains是否包含指定的字符
String str1="hello World";
System.out.println(str1.contains("World")); // true
String str2="hello World";
System.out.println(str2.contains("World-")); // false
字符串-startsWith是否以指定字符开头
String str1="hello World";
System.out.println(str1.startsWith("hello")); //true
String str1="hello World";
System.out.println(str1.startsWith("hello2")); // false
字符串-endsWith是否以指定字符结尾
String str1="hello World";
System.out.println(str1.endsWith("hello2")); // false
String str1="hello World";
System.out.println(str1.endsWith("ld")); // true
判断字符串是否是空字符
String str1="hello World";
System.out.println(str1.isEmpty()); // false
String str1=" ";
System.out.println(str1.isEmpty()); // false
ps:空格其实是一个特殊的字符。不为空。
String str1="";
System.out.println(str1.isEmpty()); // true
这样的拼接效率是非常的低的
String s="";
for(int i=0;i<100;i++){
s=s+i;
}
System.out.println(s);
ps:这样字符串的拼接效率是非常的低的;
java作为服务端的语言;这样肯定是不行的;
那么我们怎么来处理这个问题呢?
StringBuilder 提升效率
StringBuilder s = new StringBuilder();
for(int i=0;i<1000;i++){
// 添加到这个s变量中;不会做加法运算的哈
s.append(i);
}
// 转化为字符串
System.out.println(s.toString());
StringBuilder 的几个常见方法
StringBuilder s = new StringBuilder();
s.append("abc"); // 添加
System.out.println(s.toString()); // 输出字符串:abc
System.out.println(s.length()); // 长度3
System.out.println(s.reverse()); // 翻转操作:输出cba
System.out.println(s.insert(1, "1")); // 插入:在字符串cba下标为1的位置插入1;输出 c1ba
作者:流年少年
出处:https://www.cnblogs.com/ishoulgodo/
本文版权归作者所有,欢迎转载,未经作者同意须保留此段声明,在文章页面明显位置给出原文连接
如果文中有什么错误,欢迎指出。以免更多的人被误导。
出处:https://www.cnblogs.com/ishoulgodo/
想问问题,打赏了卑微的博主,求求你备注一下的扣扣或者微信;这样我好联系你;(っ•̀ω•́)っ✎⁾⁾!
如果觉得这篇文章对你有小小的帮助的话,记得在右下角点个“推荐”哦,或者关注博主,在此感谢!
万水千山总是情,打赏5毛买辣条行不行,所以如果你心情还比较高兴,也是可以扫码打赏博主(っ•̀ω•́)っ✎⁾⁾!
想问问题,打赏了卑微的博主,求求你备注一下的扣扣或者微信;这样我好联系你;(っ•̀ω•́)っ✎⁾⁾!
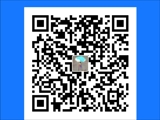
支付宝
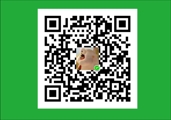
微信
如果文中有什么错误,欢迎指出。以免更多的人被误导。