Spring Boot 教程:Rest 模板
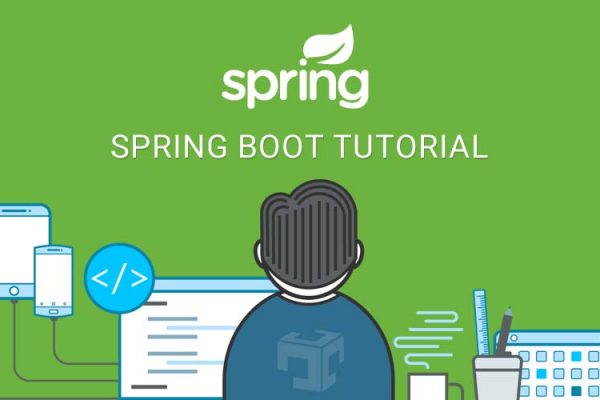
Rest 模板用于创建消费 RESTful Web 服务的应用。你可以使用 exchange() 方法来消费所有 HTTP 方法的 web 服务。下面的代码展示了如何创建 Rest 模板 Bean 来自动绑定 Rest 模板对象:
1 package com.tutorialspoint.demo; 2 3 import org.springframework.boot.SpringApplication; 4 import org.springframework.boot.autoconfigure.SpringBootApplication; 5 import org.springframework.context.annotation.Bean; 6 import org.springframework.web.client.RestTemplate; 7 8 @SpringBootApplication 9 public class DemoApplication { 10 public static void main(String[] args) { 11 SpringApplication.run(DemoApplication.class, args); 12 } 13 @Bean 14 public RestTemplate getRestTemplate() { 15 return new RestTemplate(); 16 }
GET
使用 RestTemplate - exchange() 方法消费 GET API
假设这个 URL http://localhost:8080/products 返回下面的 JSON ,我们要通过后面的代码使用 Rest 模板来消费这个 API 响应:
1 [ 2 { 3 "id": "1", 4 "name": "Honey" 5 }, 6 { 7 "id": "2", 8 "name": "Almond" 9 } 10 ]
你要通过以下几点来消费 API:
-
自动绑定 Rest 模板对象
-
使用 HttpHeaders 设置请求头
-
使用 HttpEntity 包装请求对象
-
为 Exchange() 方法提供 URL、HttpMethod 和返回值类型
1 @RestController 2 public class ConsumeWebService { 3 @Autowired 4 RestTemplate restTemplate; 5 6 @RequestMapping(value = "/template/products") 7 public String getProductList() { 8 HttpHeaders headers = new HttpHeaders(); 9 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 10 HttpEntity <String> entity = new HttpEntity<String>(headers); 11 12 return restTemplate.exchange(" 13 http://localhost:8080/products", HttpMethod.GET, entity, String.class).getBody(); 14 } 15 }
POST
使用 RestTemplate - exchange() 方法消费 POST API
假设 URL http://localhost:8080/products 返回如下响应,我们要使用 Rest 模板来消费这个 API 响应。
请求体代码如下:
1 { 2 "id":"3", 3 "name":"Ginger" 4 }
响应体代码如下:
Product is created successfully
你要通过以下几点来消费 API:
-
自动绑定 Rest 模板对象
-
使用 HttpHeaders 设置请求头
-
使用 HttpEntity 包装请求对象,这里我们包装了产品对象发送给请求体
-
为 Exchange() 方法提供 URL、HttpMethod 和返回值类型
1 @RestController 2 public class ConsumeWebService { 3 @Autowired 4 RestTemplate restTemplate; 5 6 @RequestMapping(value = "/template/products", method = RequestMethod.POST) 7 public String createProducts(@RequestBody Product product) { 8 HttpHeaders headers = new HttpHeaders(); 9 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 10 HttpEntity<Product> entity = new HttpEntity<Product>(product,headers); 11 12 return restTemplate.exchange( 13 "http://localhost:8080/products", HttpMethod.POST, entity, String.class).getBody(); 14 } 15 }
PUT
使用 RestTemplate - exchange() 方法消费 PUT API
假设 URL http://localhost:8080/products/3 返回下面的响应,我们要使用 Rest 模板来消费这个 API 响应。
请求体代码如下:
1 { 2 "name": "Indian Ginger" 3 }
响应体代码如下:
Product is updated successfully
你要通过以下几点来消费 API:
-
自动绑定 Rest 模板对象
-
使用 HttpHeaders 设置请求头
-
使用 HttpEntity 包装请求对象,这里我们包装了产品对象发送给请求体
-
为 Exchange() 方法提供 URL、HttpMethod 和返回值类型
1 @RestController 2 public class ConsumeWebService { 3 @Autowired 4 RestTemplate restTemplate; 5 6 @RequestMapping(value = "/template/products/{id}", method = RequestMethod.PUT) 7 public String updateProduct(@PathVariable("id") String id, @RequestBody Product product) { 8 HttpHeaders headers = new HttpHeaders(); 9 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 10 HttpEntity<Product> entity = new HttpEntity<Product>(product,headers); 11 12 return restTemplate.exchange( 13 "http://localhost:8080/products/"+id, HttpMethod.PUT, entity, String.class).getBody(); 14 } 15 }
DELETE
使用 RestTemplate - exchange() 方法消费 DELETE API
假设 URL http://localhost:8080/products/3 返回下面的响应,我们要使用 Rest 模板来消费这个 API 响应。
响应体代码如下:
Product is deleted successfully
你要通过以下几点来消费 API:
-
自动绑定 Rest 模板对象
-
使用 HttpHeaders 设置请求头
-
使用 HttpEntity 包装请求对象
-
为 Exchange() 方法提供 URL、HttpMethod 和返回值类型
1 @RestController 2 public class ConsumeWebService { 3 @Autowired 4 RestTemplate restTemplate; 5 6 @RequestMapping(value = "/template/products/{id}", method = RequestMethod.DELETE) 7 public String deleteProduct(@PathVariable("id") String id) { 8 HttpHeaders headers = new HttpHeaders(); 9 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 10 HttpEntity<Product> entity = new HttpEntity<Product>(headers); 11 12 return restTemplate.exchange( 13 "http://localhost:8080/products/"+id, HttpMethod.DELETE, entity, String.class).getBody(); 14 } 15 }
完整的 Rest 模板控制器类代码文件如下:
1 package com.tutorialspoint.demo.controller; 2 3 import java.util.Arrays; 4 5 import org.springframework.beans.factory.annotation.Autowired; 6 import org.springframework.http.HttpEntity; 7 import org.springframework.http.HttpHeaders; 8 import org.springframework.http.HttpMethod; 9 import org.springframework.http.MediaType; 10 11 import org.springframework.web.bind.annotation.PathVariable; 12 import org.springframework.web.bind.annotation.RequestBody; 13 import org.springframework.web.bind.annotation.RequestMapping; 14 import org.springframework.web.bind.annotation.RequestMethod; 15 import org.springframework.web.bind.annotation.RestController; 16 import org.springframework.web.client.RestTemplate; 17 18 import com.tutorialspoint.demo.model.Product; 19 20 @RestController 21 public class ConsumeWebService { 22 @Autowired 23 RestTemplate restTemplate; 24 25 @RequestMapping(value = "/template/products") 26 public String getProductList() { 27 HttpHeaders headers = new HttpHeaders(); 28 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 29 HttpEntity<String> entity = new HttpEntity<String>(headers); 30 31 return restTemplate.exchange( 32 "http://localhost:8080/products", HttpMethod.GET, entity, String.class).getBody(); 33 } 34 @RequestMapping(value = "/template/products", method = RequestMethod.POST) 35 public String createProducts(@RequestBody Product product) { 36 HttpHeaders headers = new HttpHeaders(); 37 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 38 HttpEntity<Product> entity = new HttpEntity<Product>(product,headers); 39 40 return restTemplate.exchange( 41 "http://localhost:8080/products", HttpMethod.POST, entity, String.class).getBody(); 42 } 43 @RequestMapping(value = "/template/products/{id}", method = RequestMethod.PUT) 44 public String updateProduct(@PathVariable("id") String id, @RequestBody Product product) { 45 HttpHeaders headers = new HttpHeaders(); 46 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 47 HttpEntity<Product> entity = new HttpEntity<Product>(product,headers); 48 49 return restTemplate.exchange( 50 "http://localhost:8080/products/"+id, HttpMethod.PUT, entity, String.class).getBody(); 51 } 52 @RequestMapping(value = "/template/products/{id}", method = RequestMethod.DELETE) 53 public String deleteProduct(@PathVariable("id") String id) { 54 HttpHeaders headers = new HttpHeaders(); 55 headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON)); 56 HttpEntity<Product> entity = new HttpEntity<Product>(headers); 57 58 return restTemplate.exchange( 59 "http://localhost:8080/products/"+id, HttpMethod.DELETE, entity, String.class).getBody(); 60 } 61 }
Spring Boot 应用类 – DemoApplication.java 如下所示:
1 package com.tutorialspoint.demo; 2 3 import org.springframework.boot.SpringApplication; 4 import org.springframework.boot.autoconfigure.SpringBootApplication; 5 6 @SpringBootApplication 7 public class DemoApplication { 8 public static void main(String[] args) { 9 SpringApplication.run(DemoApplication.class, args); 10 } 11 }
Maven build – pom.xml 如下:
1 <?xml version = "1.0" encoding = "UTF-8"?> 2 <project xmlns = "http://maven.apache.org/POM/4.0.0" 3 xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" 4 xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 5 http://maven.apache.org/xsd/maven-4.0.0.xsd"> 6 7 <modelVersion>4.0.0</modelVersion> 8 <groupId>com.tutorialspoint</groupId> 9 <artifactId>demo</artifactId> 10 <version>0.0.1-SNAPSHOT</version> 11 <packaging>jar</packaging> 12 <name>demo</name> 13 <description>Demo project for Spring Boot</description> 14 15 <parent> 16 <groupId>org.springframework.boot</groupId> 17 <artifactId>spring-boot-starter-parent</artifactId> 18 <version>1.5.8.RELEASE</version> 19 <relativePath/> 20 </parent> 21 22 <properties> 23 <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> 24 <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> 25 <java.version>1.8</java.version> 26 </properties> 27 28 <dependencies> 29 <dependency> 30 <groupId>org.springframework.boot</groupId> 31 <artifactId>spring-boot-starter-web</artifactId> 32 </dependency> 33 34 <dependency> 35 <groupId>org.springframework.boot</groupId> 36 <artifactId>spring-boot-starter-test</artifactId> 37 <scope>test</scope> 38 </dependency> 39 </dependencies> 40 41 <build> 42 <plugins> 43 <plugin> 44 <groupId>org.springframework.boot</groupId> 45 <artifactId>spring-boot-maven-plugin</artifactId> 46 </plugin> 47 </plugins> 48 </build> 49 </project>
Gradle Build – build.gradle 如下:
1 buildscript { 2 ext { 3 springBootVersion = '1.5.8.RELEASE' 4 } 5 repositories { 6 mavenCentral() 7 } 8 dependencies { 9 classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") 10 } 11 } 12 13 apply plugin: 'java' 14 apply plugin: 'eclipse' 15 apply plugin: 'org.springframework.boot' 16 17 group = 'com.tutorialspoint' 18 version = '0.0.1-SNAPSHOT' 19 sourceCompatibility = 1.8 20 21 repositories { 22 mavenCentral() 23 } 24 dependencies { 25 compile('org.springframework.boot:spring-boot-starter-web') 26 testCompile('org.springframework.boot:spring-boot-starter-test') 27 }
你可以使用 Maven 或 Graven 命令 创建可执行 JAR 文件,并运行 Spring Boot 应用:
Maven 可以使用以下命令:
mvn clean install
在 “BUILD SUCCESS” 之后 ,你可以在 target 目录下找到 JAR 文件:
对于 Gradle,可以使用以下命令:
gradle clean build
在 “BUILD SUCCESSFUL” 之后,你可以在 build/libs 目录下找到 JAR 文件:
使用以下命令运行 JAR 文件:
java –jar <JARFILE>
现在应用已经在 Tomcat 上以 8080 端口上启动了:

在 POSTMAN 应用中输入 URL 可以看到下面的输出:
使用 Rest 模板 GET 产品:http://localhost:8080/template/products
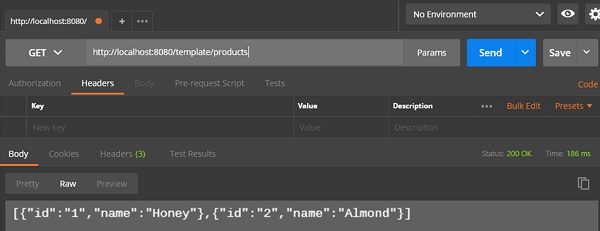
创建产品 POST:http://localhost:8080/template/products
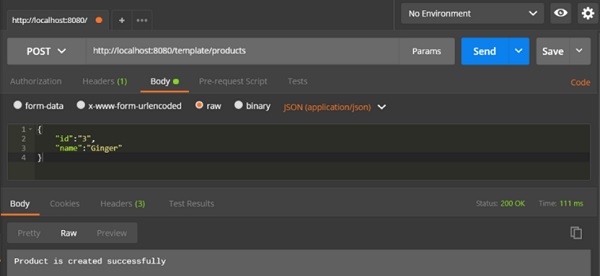
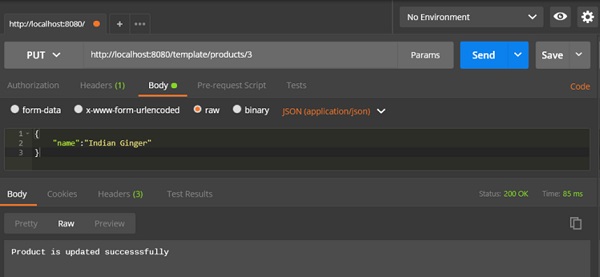
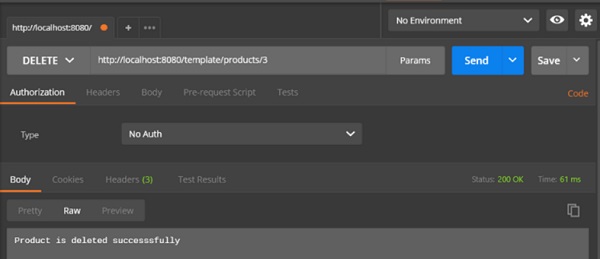