142. 环形链表
给定一个链表,返回链表开始入环的第一个节点。 如果链表无环,则返回 null。
为了表示给定链表中的环,我们使用整数 pos 来表示链表尾连接到链表中的位置(索引从 0 开始)。 如果 pos 是 -1,则在该链表中没有环。
说明:不允许修改给定的链表。
示例 1:
输入:head = [3,2,0,-4], pos = 1
输出:tail connects to node index 1
解释:链表中有一个环,其尾部连接到第二个节点。
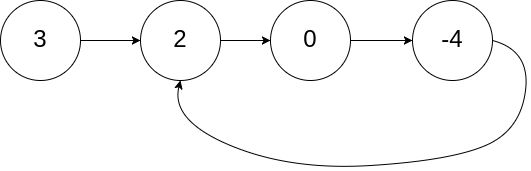
示例 2:
输入:head = [1,2], pos = 0
输出:tail connects to node index 0
解释:链表中有一个环,其尾部连接到第一个节点。
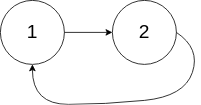
示例 3:
输入:head = [1], pos = -1
输出:no cycle
解释:链表中没有环。

进阶:
你是否可以不用额外空间解决此题?
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/linked-list-cycle-ii
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
思路 1
遍历链表,将链表中节点对应的指针插入 set
插入节点前,要查找 set 中是否存在这个节点,存在即起点
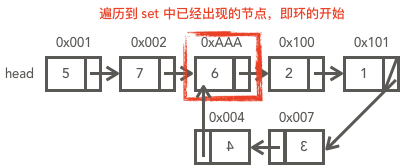
1 class Solution { 2 public: 3 ListNode *detectCycle(ListNode *head) { 4 set<ListNode *> node_set; 5 while (head) { 6 if (node_set.find(head) != node_set.end()) { 7 return head; 8 } 9 node_set.insert(head); 10 head = head->next; 11 } 12 return NULL; 13 } 14 };
思路 2
快慢指针
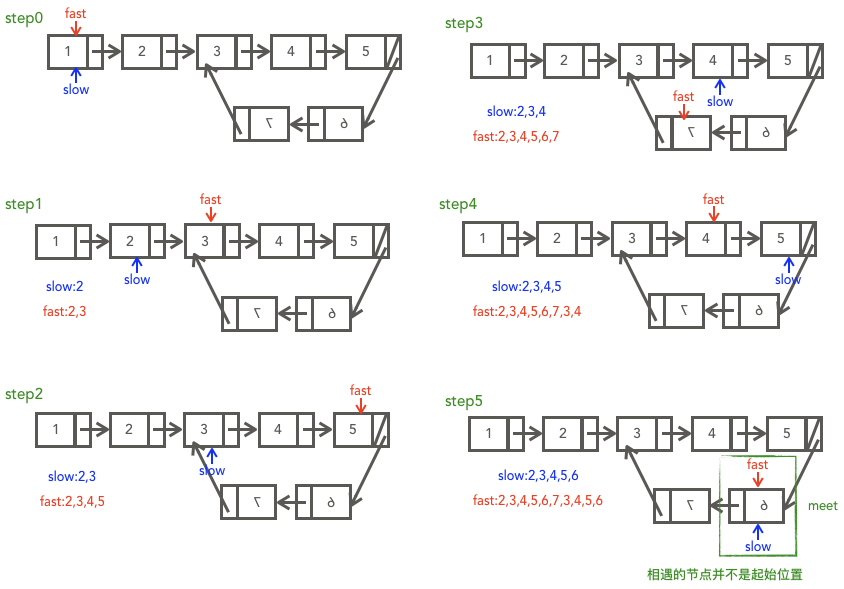
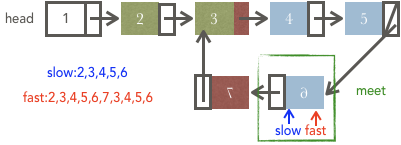
设:1)绿色 2,3 段为 a2)蓝色 4,5,6 段为 b3)红色 7,3 段为 cslow = a+bfast = a+b+c+bfast 的路程是 slow 的两倍:2*(a+b)= a+b+c+b>> a = c(有环,这两段是相等的)
从 head 与 meet 出发,两指针速度一样,相遇即环的起点
1 class Solution { 2 public: 3 ListNode *detectCycle(ListNode *head) { 4 ListNode *fast = head; 5 ListNode *slow = head; 6 ListNode *meet = NULL;//相遇的节点 7 8 while (fast) { 9 slow = slow->next;//各走一步 10 fast = fast->next; 11 if (!fast) {//fast到了尾部,说明不是环形 12 return NULL; 13 } 14 fast = fast->next; 15 if (fast == slow) { 16 meet = fast; 17 break; 18 } 19 } 20 if (meet == NULL) { 21 return NULL; 22 } 23 while (head && meet) {//能到这里说明一定有环,条件(true) 24 if (head == meet) { 25 return head;//相遇即环的起点 26 } 27 head = head->next; 28 meet = meet->next; 29 } 30 return NULL;// 31 } 32 };