Python监控Redis脚本
Python监控Redis脚本
一、Redis监控信息
介绍下监控redis那些信息:
Redis ping:检验ping
Redis alive:查看检查端口是否alive
Redis connections:查看连接数
Redis blockedClients:正在等待阻塞客户端数量
Redis connectionsUsage:redis的连接使用率
Redis memoryUsage:redis内存使用量
Redis memoryUsageRate:redis内存使用率
Redis evictedKeys:运行以来删除过的key的数量
Redis rejectedConnections:拒绝连接数
Redis ops:redis的OPS
Redis hitRate:redis命中率
二、Python脚本
需要安装的环境:
pip install redis
脚本如下:
#!/usr/bin/env python
# -*- coding: utf-8 -*-
__author__ = 'lawrence'
import sys
import subprocess
import json
try:
import redis
except Exception as e:
print('pip install redis')
sys.exit(1)
class Redis(object):
def __init__(self, host, port, password=None):
self.host = host
self.port = port
self.password = password
if self.password:
self.rds = redis.StrictRedis(host=host, port=port, password=self.password)
else:
self.rds = redis.StrictRedis(host=host, port=port)
try:
self.info = self.rds.info()
except Exception as e:
self.info = None
def redis_connections(self):
try:
return self.info['connected_clients']
except Exception as e:
return 0
def redis_connections_usage(self):
try:
curr_connections = self.redis_connections()
max_clients = self.parse_config('maxclients')
rate = float(curr_connections) / float(max_clients)
return "%.2f" % (rate * 100)
except Exception as e:
return 0
def redis_used_memory(self):
try:
return self.info['used_memory']
except Exception as e:
return 0
def redis_memory_usage(self):
try:
used_memory = self.info['used_memory']
max_memory = self.info['maxmemory']
system_memory = self.info['total_system_memory']
if max_memory:
rate = float(used_memory) / float(max_memory)
else:
rate = float(used_memory) / float(system_memory)
return "%.2f" % (rate * 100)
except Exception as e:
return 0
def redis_ping(self):
try:
return self.rds.ping()
except Exception as e:
return False
def rejected_connections(self):
try:
return self.info['rejected_connections']
except Exception as e:
return 999
def evicted_keys(self):
try:
return self.info['evicted_keys']
except Exception as e:
return 999
def blocked_clients(self):
try:
return self.info['blocked_clients']
except Exception as e:
return 0
def ops(self):
try:
return self.info['instantaneous_ops_per_sec']
except Exception as e:
return 0
def hitRate(self):
try:
misses = self.info['keyspace_misses']
hits = self.info['keyspace_hits']
rate = float(hits) / float(int(hits) + int(misses))
return "%.2f" % (rate * 100)
except Exception as e:
return 0
def parse_config(self, type):
try:
return self.rds.config_get(type)[type]
except Exception as e:
return None
def test(self):
print('Redis ping: %s' % self.redis_ping())
print('Redis alive: %s ' % check_alive(self.host, self.port))
print('Redis connections: %s' % self.redis_connections())
print('Redis blockedClients %s' % self.blocked_clients())
print('Redis connectionsUsage: %s%%' % self.redis_connections_usage())
print('Redis memoryUsage: %s' % self.redis_used_memory())
print('Redis memoryUsageRate: %s%%' % self.redis_memory_usage())
print('Redis evictedKeys: %s' % self.evicted_keys())
print('Redis rejectedConnections: %s' % self.rejected_connections())
print('Redis ops: %s' % self.ops())
print('Redis hitRate: %s%%' % self.hitRate())
def check_alive(host, port):
cmd = 'nc -z %s %s > /dev/null 2>&1' % (host, port)
return subprocess.call(cmd, shell=True)
def parse(type, host, port, password):
rds = Redis(host, port, password)
# print(rds.info) # 获取全部redis的info信息
if type == 'connections':
print(rds.redis_connections())
elif type == 'connectionsUsage':
print(rds.redis_connections_usage())
elif type == 'blockedClients':
print(rds.blocked_clients())
elif type == 'ping':
print(rds.redis_ping())
elif type == 'alive':
print(check_alive(host, port))
elif type == 'memoryUsage':
print(rds.redis_used_memory())
elif type == 'memoryUsageRate':
print(rds.redis_memory_usage())
elif type == 'rejectedConnections':
print(rds.rejected_connections())
elif type == 'evictedKeys':
print(rds.evicted_keys())
elif type == 'hitRate':
print(rds.hitRate())
elif type == 'ops':
print(rds.ops())
else:
rds.test()
if __name__ == '__main__':
try:
type = sys.argv[1]
host = sys.argv[2]
port = sys.argv[3]
if sys.argv.__len__() >= 5:
password = sys.argv[4]
else:
password = None
except Exception as e:
print("Usage: python %s type 127.0.0.1 6379" % sys.argv[0])
sys.exit(1)
parse(type, host, port, password)
三、执行方法
[root@Manager ~]# python redisMonitor.py test 127.0.0.1 6379 password
Redis ping: True
Redis alive: 0
Redis connections: 1
Redis blockedClients 0
Redis connectionsUsage: 0.00%
Redis memoryUsage: 1156403848
Redis memoryUsageRate: 0%
Redis evictedKeys: 0
Redis rejectedConnections: 0
Redis ops: 16
Redis hitRate: 48.86%
# 获取某一个单独值
[root@Manager ~]# python redisMonitor.py connections 127.0.0.1 6379 password
1
[root@Manager ~]# python redisMonitor.py alive 127.0.0.1 6379 password
0
[root@Manager ~]# python redisMonitor.py ping 127.0.0.1 6379 password
True
四、Zabbix接入
[root@Manager ~]# cat /etc/zabbix/zabbix_agentd.d/redis.conf
# Redis
UserParameter=redis.stats[*],/bin/python /home/python/redisMonitor.py $1 127.0.0.1 6379 password
作者:Lawrence
-------------------------------------------
个性签名:独学而无友,则孤陋而寡闻。做一个灵魂有趣的人!
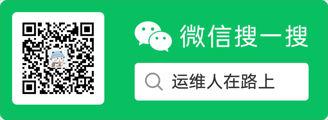
扫描上面二维码关注我
如果你真心觉得文章写得不错,而且对你有所帮助,那就不妨帮忙“推荐"一下,您的“推荐”和”打赏“将是我最大的写作动力!
本文版权归作者所有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接.