上传文件到 Sharepoint 的文档库中和下载 Sharepoint 的文档库的文件到客户端
文件操作应用场景:
如果你的.NET项目是运行在SharePoint服务器上的,你可以直接使用SharePoint服务器端对象模型,用SPFileCollection.Add方法
http://msdn.microsoft.com/zh-cn/library/ms454491%28office.12%29.aspx
如果不在同一台机器上,并且你的SharePoint是2010,你可以使用.NET客户端对象模型,用FileCollection.Add方法
http://msdn.microsoft.com/zh-cn/library/microsoft.sharepoint.client.filecollection.add(en-us).aspx
如果是SharePoint 2007,你可以用SharePoint Web Service里面的copy.asmx
http://msdn.microsoft.com/zh-cn/library/copy.copy_members%28en-us,office.12%29.aspx
============帮助文档说明===========
Create Author: Kenmu
Create Date: 2014-05-22
Function: 支持上传文件到Sharepoint的文档库中;下载Sharepoint的文档库的文件到客户端
Support three ways as following:
1)通过调用SharePoint的Copy.asmx服务实现;只适用于SharePoint站点
IFileOperation fileOperation = new SPServiceOperation();
bool isSuccess = fileOperation.UploadFileToSPSite(domain, userAccount, pwd, documentLibraryUrl, localFilePath, ref statusInfo);
bool isSuccess = fileOperation.DownloadFileFromSPSite(domain, userAccount, pwd, documentUrl, downloadToLocalFilePath, ref statusInfo);
2)通过WebClient实现;适用于普通站点
IFileOperation fileOperation = new WebClientOperation();
3)通过WebRequest实现;适用于普通站点
IFileOperation fileOperation = new WebRequestOperation();
关键代码:
IFileOperation.cs
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Text; 5 6 namespace FileOperationAboutSharePoint.FileOperation 7 { 8 interface IFileOperation 9 { 10 bool UploadFileToSPSite(string domain, string userAccount, string pwd, string documentLibraryUrl, string localFilePath,ref string statusInfo); 11 bool DownloadFileFromSPSite(string domain, string userAccount, string pwd, string documentUrl, string localFilePath, ref string statusInfo); 12 } 13 }
SPServiceOperation.cs
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Web; 5 using System.IO; 6 using System.Net; 7 using FileOperationAboutSharePoint.SPCopyService; 8 9 namespace FileOperationAboutSharePoint.FileOperation 10 { 11 public class SPServiceOperation : IFileOperation 12 { 13 public bool UploadFileToSPSite(string domain, string userAccount, string pwd, string documentLibraryUrl, string localFilePath, ref string statusInfo) 14 { 15 bool isSuccess = false; 16 try 17 { 18 string fileName = Path.GetFileName(localFilePath); 19 string tempFilePath = string.Format("{0}{1}", Path.GetTempPath(), fileName); 20 File.Copy(localFilePath, tempFilePath, true); 21 FileStream fs = new FileStream(tempFilePath, FileMode.Open, FileAccess.Read); 22 BinaryReader br = new BinaryReader(fs); 23 byte[] fileContent = br.ReadBytes((int)fs.Length); 24 br.Close(); 25 fs.Close(); 26 Copy service = CreateCopy(domain, userAccount, pwd); 27 service.Timeout = System.Threading.Timeout.Infinite; 28 FieldInformation fieldInfo = new FieldInformation(); 29 FieldInformation[] fieldInfoArr = { fieldInfo }; 30 CopyResult[] resultArr; 31 service.CopyIntoItems( 32 tempFilePath, 33 new string[] { string.Format("{0}{1}", documentLibraryUrl, fileName) }, 34 fieldInfoArr, 35 fileContent, 36 out resultArr); 37 isSuccess = resultArr[0].ErrorCode == CopyErrorCode.Success; 38 if (!isSuccess) 39 { 40 statusInfo = string.Format("Failed Info: {0}", resultArr[0].ErrorMessage); 41 } 42 43 } 44 catch (Exception ex) 45 { 46 statusInfo = string.Format("Failed Info: {0}", ex.Message); 47 isSuccess = false; 48 } 49 return isSuccess; 50 } 51 52 public bool DownloadFileFromSPSite(string domain, string userAccount, string pwd, string documentUrl, string localFilePath, ref string statusInfo) 53 { 54 bool isSuccess = false; 55 try 56 { 57 Copy service = CreateCopy(domain, userAccount, pwd); 58 service.Timeout = System.Threading.Timeout.Infinite; 59 FieldInformation[] fieldInfoArr; 60 byte[] fileContent; 61 service.GetItem(documentUrl,out fieldInfoArr,out fileContent); 62 if (fileContent != null) 63 { 64 FileStream fs = new FileStream(localFilePath, FileMode.Create, FileAccess.Write); 65 fs.Write(fileContent, 0, fileContent.Length); 66 fs.Close(); 67 isSuccess = true; 68 } 69 else 70 { 71 statusInfo = string.Format("Failed Info: {0}不存在", documentUrl); 72 isSuccess = false; 73 } 74 } 75 catch (Exception ex) 76 { 77 statusInfo = string.Format("Failed Info: {0}", ex.Message); 78 isSuccess = false; 79 } 80 return isSuccess; 81 } 82 83 private Copy CreateCopy(string domain, string userAccount, string pwd) 84 { 85 Copy service = new Copy(); 86 if (String.IsNullOrEmpty(userAccount)) 87 { 88 service.UseDefaultCredentials = true; 89 } 90 else 91 { 92 service.Credentials = new NetworkCredential(userAccount, pwd, domain); 93 } 94 return service; 95 } 96 } 97 }
WebClientOperation.cs
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Web; 5 using System.IO; 6 using System.Net; 7 8 namespace FileOperationAboutSharePoint.FileOperation 9 { 10 public class WebClientOperation : IFileOperation 11 { 12 public bool UploadFileToSPSite(string domain, string userAccount, string pwd, string documentLibraryUrl, string localFilePath, ref string statusInfo) 13 { 14 bool isSuccess = false; 15 try 16 { 17 string fileName = Path.GetFileName(localFilePath); 18 string tempFilePath = string.Format("{0}{1}", Path.GetTempPath(), fileName); 19 File.Copy(localFilePath, tempFilePath, true); 20 FileStream fs = new FileStream(tempFilePath, FileMode.Open, FileAccess.Read); 21 BinaryReader br = new BinaryReader(fs); 22 byte[] fileContent = br.ReadBytes((int)fs.Length); 23 br.Close(); 24 fs.Close(); 25 WebClient webClient = CreateWebClient(domain, userAccount, pwd); 26 webClient.UploadData(string.Format("{0}{1}", documentLibraryUrl, fileName), "PUT", fileContent); 27 isSuccess = true; 28 } 29 catch (Exception ex) 30 { 31 statusInfo = string.Format("Failed Info: {0}", ex.Message); 32 isSuccess = false; 33 } 34 return isSuccess; 35 } 36 37 public bool DownloadFileFromSPSite(string domain, string userAccount, string pwd, string documentUrl, string localFilePath, ref string statusInfo) 38 { 39 bool isSuccess = false; 40 try 41 { 42 WebClient webClient = CreateWebClient(domain, userAccount, pwd); 43 byte[] fileContent = webClient.DownloadData(documentUrl); 44 FileStream fs = new FileStream(localFilePath, FileMode.Create, FileAccess.Write); 45 fs.Write(fileContent, 0, fileContent.Length); 46 fs.Close(); 47 } 48 catch (Exception ex) 49 { 50 statusInfo = string.Format("Failed Info: {0}", ex.Message); 51 isSuccess = false; 52 } 53 return isSuccess; 54 } 55 56 private WebClient CreateWebClient(string domain, string userAccount, string pwd) 57 { 58 WebClient webClient = new WebClient(); 59 if (String.IsNullOrEmpty(userAccount)) 60 { 61 webClient.UseDefaultCredentials = true; 62 } 63 else 64 { 65 webClient.Credentials = new NetworkCredential(userAccount, pwd, domain); 66 } 67 return webClient; 68 } 69 } 70 }
WebRequestOperation.cs
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Web; 5 using System.IO; 6 using System.Net; 7 8 namespace FileOperationAboutSharePoint.FileOperation 9 { 10 public class WebRequestOperation : IFileOperation 11 { 12 public bool UploadFileToSPSite(string domain, string userAccount, string pwd, string documentLibraryUrl, string localFilePath, ref string statusInfo) 13 { 14 bool isSuccess = false; 15 try 16 { 17 string fileName = Path.GetFileName(localFilePath); 18 string tempFilePath = string.Format("{0}{1}", Path.GetTempPath(), fileName); 19 File.Copy(localFilePath, tempFilePath, true); 20 FileStream fs = new FileStream(tempFilePath, FileMode.Open, FileAccess.Read); 21 BinaryReader br = new BinaryReader(fs); 22 byte[] fileContent = br.ReadBytes((int)fs.Length); 23 br.Close(); 24 fs.Close(); 25 MemoryStream ms = new MemoryStream(fileContent); 26 WebRequest webRequest = CreateWebRequest(domain,userAccount,pwd,string.Format("{0}{1}", documentLibraryUrl, fileName)); 27 webRequest.Method = "PUT"; 28 webRequest.Headers.Add("Overwrite", "T"); 29 webRequest.Timeout = System.Threading.Timeout.Infinite; 30 Stream outStream = webRequest.GetRequestStream(); 31 byte[] buffer = new byte[1024]; 32 while (true) 33 { 34 int i = ms.Read(buffer, 0, buffer.Length); 35 if (i <= 0) 36 break; 37 outStream.Write(buffer, 0, i); 38 } 39 ms.Close(); 40 outStream.Close(); 41 webRequest.GetResponse(); 42 isSuccess = true; 43 } 44 catch (Exception ex) 45 { 46 statusInfo = string.Format("Failed Info: {0}", ex.Message); 47 isSuccess = false; 48 } 49 return isSuccess; 50 } 51 52 public bool DownloadFileFromSPSite(string domain, string userAccount, string pwd, string documentUrl, string localFilePath, ref string statusInfo) 53 { 54 bool isSuccess = false; 55 try 56 { 57 WebRequest webRequest = CreateWebRequest(domain, userAccount, pwd, documentUrl); 58 webRequest.Method = "GET"; 59 webRequest.Timeout = System.Threading.Timeout.Infinite; 60 using (Stream stream = webRequest.GetResponse().GetResponseStream()) 61 { 62 using (FileStream fs = new FileStream(localFilePath, FileMode.Create, FileAccess.Write)) 63 { 64 byte[] buffer = new byte[1024]; 65 int i = 1; 66 while (i > 0) 67 { 68 i = stream.Read(buffer, 0, buffer.Length); 69 fs.Write(buffer, 0, i); 70 } 71 } 72 } 73 isSuccess = true; 74 } 75 catch (Exception ex) 76 { 77 statusInfo = string.Format("Failed Info: {0}", ex.Message); 78 isSuccess = false; 79 } 80 return isSuccess; 81 } 82 83 private WebRequest CreateWebRequest(string domain, string userAccount, string pwd, string requestUriString) 84 { 85 WebRequest webRequest = WebRequest.Create(requestUriString); 86 if (String.IsNullOrEmpty(userAccount)) 87 { 88 webRequest.UseDefaultCredentials = true; 89 } 90 else 91 { 92 webRequest.Credentials = new NetworkCredential(userAccount, pwd, domain); 93 } 94 return webRequest; 95 } 96 } 97 }
Web.config
1 <?xml version="1.0"?> 2 <configuration> 3 <configSections> 4 <sectionGroup name="applicationSettings" type="System.Configuration.ApplicationSettingsGroup, System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" > 5 <section name="FileOperationAboutSharePoint.Properties.Settings" type="System.Configuration.ClientSettingsSection, System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false" /> 6 </sectionGroup> 7 </configSections> 8 9 <system.web> 10 <compilation debug="true" targetFramework="4.0" /> 11 <authentication mode="Windows"> 12 </authentication> 13 </system.web> 14 15 <system.webServer> 16 <modules runAllManagedModulesForAllRequests="true"/> 17 </system.webServer> 18 <system.serviceModel> 19 <bindings /> 20 <client /> 21 </system.serviceModel> 22 <applicationSettings> 23 <FileOperationAboutSharePoint.Properties.Settings> 24 <setting name="FileOperationAboutSharePoint_SPCopyService_Copy" 25 serializeAs="String"> 26 <value>http://ifca-psserver/_vti_bin/copy.asmx</value> 27 </setting> 28 </FileOperationAboutSharePoint.Properties.Settings> 29 </applicationSettings> 30 <appSettings> 31 <add key="domain" value="IFCA"/> 32 <add key="userAccount" value="huangjianwu"/> 33 <add key="pwd" value="ifca.123456"/> 34 <add key="documentLibraryUrl" value="http://ifca-psserver/KDIT/"/> 35 <add key="downloadFileName" value="提示信息Message说明文档.docx"/> 36 <add key="downloadToLocalFilePath" value="C:\\Users\Administrator\Desktop\download_提示信息Message说明文档.docx"/> 37 </appSettings> 38 </configuration>
Default.aspx
上传操作:<asp:FileUpload ID="fuSelectFile" runat="server" /> <asp:Button ID="btnUpload" Text="上传" runat="server" Style="margin: 0 10px;" OnClick="btnUpload_Click" /><br /> 下载操作:“<%= string.Format("{0}{1}",documentLibraryUrl, downloadFileName)%>”文件 <asp:Button ID="btnDownload" Text="下载" runat="server" Style="margin: 5px 10px;" OnClick="btnDownload_Click" /> <p /><strong>执行结果:</strong><br /> <asp:Literal ID="ltrInfo" runat="server"></asp:Literal>
Default.aspx.cs
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Web; 5 using System.Web.UI; 6 using System.Web.UI.WebControls; 7 using FileOperationAboutSharePoint.FileOperation; 8 using System.Web.Configuration; 9 10 namespace FileOperationAboutSharePoint 11 { 12 public partial class _Default : System.Web.UI.Page 13 { 14 private string domain = WebConfigurationManager.AppSettings["domain"]; 15 private string userAccount = WebConfigurationManager.AppSettings["userAccount"]; 16 private string pwd = WebConfigurationManager.AppSettings["pwd"]; 17 protected string documentLibraryUrl = WebConfigurationManager.AppSettings["documentLibraryUrl"]; 18 protected string downloadFileName = WebConfigurationManager.AppSettings["downloadFileName"]; 19 private string downloadToLocalFilePath = WebConfigurationManager.AppSettings["downloadToLocalFilePath"]; 20 21 protected void Page_Load(object sender, EventArgs e) 22 { 23 24 } 25 26 protected void btnUpload_Click(object sender, EventArgs e) 27 { 28 if (fuSelectFile.HasFile) 29 { 30 31 string localFilePath = string.Format("{0}\\{1}", Server.MapPath("Upload"), fuSelectFile.PostedFile.FileName); 32 fuSelectFile.PostedFile.SaveAs(localFilePath); 33 string statusInfo = ""; 34 //IFileOperation fileOperation = new WebClientOperation(); 35 //IFileOperation fileOperation = new WebRequestOperation(); 36 IFileOperation fileOperation = new SPServiceOperation(); 37 bool isSuccess = fileOperation.UploadFileToSPSite(domain, userAccount, pwd, documentLibraryUrl, localFilePath, ref statusInfo); 38 ltrInfo.Text = isSuccess ? "上传成功" : statusInfo; 39 } 40 else 41 { 42 ltrInfo.Text = "请选择需要上传的文件"; 43 } 44 } 45 46 protected void btnDownload_Click(object sender, EventArgs e) 47 { 48 string statusInfo = ""; 49 //IFileOperation fileOperation = new WebClientOperation(); 50 //IFileOperation fileOperation = new WebRequestOperation(); 51 IFileOperation fileOperation = new SPServiceOperation(); 52 bool isSuccess = fileOperation.DownloadFileFromSPSite(domain, userAccount, pwd, string.Format("{0}{1}", documentLibraryUrl, downloadFileName), downloadToLocalFilePath, ref statusInfo); 53 ltrInfo.Text = isSuccess ? string.Format("下载成功<br/>下载文件位置为:{0}", downloadToLocalFilePath) : statusInfo; 54 } 55 } 56 }
结果:

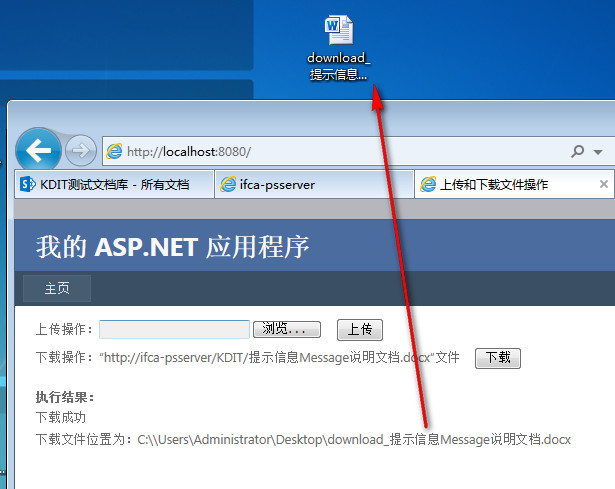
PS:如用第一种方式,就得在Visual Studio中添加Web Service,服务来源,例如:
http://ifca-psserver/_vti_bin/copy.asmx