Bear Limak wants to become the largest of bears, or at least to become larger than his brother Bob.
Right now, Limak and Bob weigh a and b respectively. It's guaranteed that Limak's weight is smaller than or equal to his brother's weight.
Limak eats a lot and his weight is tripled after every year, while Bob's weight is doubled after every year.
After how many full years will Limak become strictly larger (strictly heavier) than Bob?
The only line of the input contains two integers a and b (1 ≤ a ≤ b ≤ 10) — the weight of Limak and the weight of Bob respectively.
Print one integer, denoting the integer number of years after which Limak will become strictly larger than Bob.
4 7
2
4 9
3
1 1
1
In the first sample, Limak weighs 4 and Bob weighs 7 initially. After one year their weights are 4·3 = 12 and 7·2 = 14 respectively (one weight is tripled while the other one is doubled). Limak isn't larger than Bob yet. After the second year weights are 36 and 28, so the first weight is greater than the second one. Limak became larger than Bob after two years so you should print 2.
In the second sample, Limak's and Bob's weights in next years are: 12 and 18, then 36 and 36, and finally 108 and 72 (after three years). The answer is 3. Remember that Limak wants to be larger than Bob and he won't be satisfied with equal weights.
In the third sample, Limak becomes larger than Bob after the first year. Their weights will be 3 and 2 then.
题意:给你a,b a不断乘以3 b不断乘以2 问第几步的时候a>b
题解:水
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 #define bug() printf("!!!!!!!") 14 #define M 100005 15 using namespace std; 16 ll a,b; 17 int main() 18 { 19 scanf("%I64d %I64d",&a,&b); 20 ll ans=0; 21 while(a<=b) 22 { 23 a=a*3; 24 b=b*2; 25 ans++; 26 } 27 printf("%I64d\n",ans); 28 return 0; 29 }
Bear Limak examines a social network. Its main functionality is that two members can become friends (then they can talk with each other and share funny pictures).
There are n members, numbered 1 through n. m pairs of members are friends. Of course, a member can't be a friend with themselves.
Let A-B denote that members A and B are friends. Limak thinks that a network is reasonable if and only if the following condition is satisfied: For every three distinct members (X, Y, Z), if X-Y and Y-Z then also X-Z.
For example: if Alan and Bob are friends, and Bob and Ciri are friends, then Alan and Ciri should be friends as well.
Can you help Limak and check if the network is reasonable? Print "YES" or "NO" accordingly, without the quotes.
The first line of the input contain two integers n and m (3 ≤ n ≤ 150 000, ) — the number of members and the number of pairs of members that are friends.
The i-th of the next m lines contains two distinct integers ai and bi (1 ≤ ai, bi ≤ n, ai ≠ bi). Members ai and bi are friends with each other. No pair of members will appear more than once in the input.
If the given network is reasonable, print "YES" in a single line (without the quotes). Otherwise, print "NO" in a single line (without the quotes).
4 3
1 3
3 4
1 4
YES
4 4
3 1
2 3
3 4
1 2
NO
10 4
4 3
5 10
8 9
1 2
YES
3 2
1 2
2 3
NO
The drawings below show the situation in the first sample (on the left) and in the second sample (on the right). Each edge represents two members that are friends. The answer is "NO" in the second sample because members (2, 3) are friends and members (3, 4) are friends, while members (2, 4) are not.
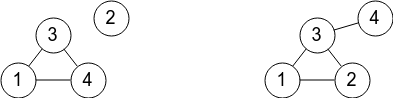
题意:给你n个点m条边 如果每个联通块都是完全图 则输出YES
题解:并查集处理出联通块。 点的度=当前联通块中的点的个数-1
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 #define bug() printf("!!!!!!!") 14 #define M 100005 15 using namespace std; 16 ll fa[150005]; 17 ll du[150005]; 18 ll n,m; 19 map<ll,ll>mp; 20 ll find(ll root) 21 { 22 if(fa[root]!=root) 23 return fa[root]=find(fa[root]); 24 else 25 return fa[root]; 26 } 27 void unio(ll a,ll b) 28 { 29 ll aa=find(a); 30 ll bb=find(b); 31 if(aa!=bb) 32 { 33 fa[aa]=bb; 34 } 35 } 36 int main() 37 { 38 memset(du,0,sizeof(du)); 39 scanf("%I64d %I64d",&n,&m); 40 for(int i=1;i<=n;i++) 41 fa[i]=i; 42 ll a,b; 43 for(int i=1;i<=m;i++) 44 { 45 scanf("%I64d %I64d",&a,&b); 46 du[a]++; 47 du[b]++; 48 unio(a,b); 49 } 50 mp.clear(); 51 for(int i=1;i<=n;i++) 52 { 53 ll x=find(i); 54 mp[fa[i]]++; 55 } 56 for(int i=1;i<=n;i++) 57 { 58 ll exm=mp[fa[i]]; 59 if((du[i]+1)!=(exm)) 60 { 61 printf("NO\n"); 62 return 0; 63 } 64 } 65 printf("YES\n"); 66 return 0; 67 }
In the army, it isn't easy to form a group of soldiers that will be effective on the battlefield. The communication is crucial and thus no two soldiers should share a name (what would happen if they got an order that Bob is a scouter, if there are two Bobs?).
A group of soldiers is effective if and only if their names are different. For example, a group (John, Bob, Limak) would be effective, while groups (Gary, Bob, Gary) and (Alice, Alice) wouldn't.
You are a spy in the enemy's camp. You noticed n soldiers standing in a row, numbered 1 through n. The general wants to choose a group of k consecutive soldiers. For every k consecutive soldiers, the general wrote down whether they would be an effective group or not.
You managed to steal the general's notes, with n - k + 1 strings s1, s2, ..., sn - k + 1, each either "YES" or "NO".
- The string s1 describes a group of soldiers 1 through k ("YES" if the group is effective, and "NO" otherwise).
- The string s2 describes a group of soldiers 2 through k + 1.
- And so on, till the string sn - k + 1 that describes a group of soldiers n - k + 1 through n.
Your task is to find possible names of n soldiers. Names should match the stolen notes. Each name should be a string that consists of between 1 and 10 English letters, inclusive. The first letter should be uppercase, and all other letters should be lowercase. Names don't have to be existing names — it's allowed to print "Xyzzzdj" or "T" for example.
Find and print any solution. It can be proved that there always exists at least one solution.
The first line of the input contains two integers n and k (2 ≤ k ≤ n ≤ 50) — the number of soldiers and the size of a group respectively.
The second line contains n - k + 1 strings s1, s2, ..., sn - k + 1. The string si is "YES" if the group of soldiers i through i + k - 1 is effective, and "NO" otherwise.
Find any solution satisfying all given conditions. In one line print n space-separated strings, denoting possible names of soldiers in the order. The first letter of each name should be uppercase, while the other letters should be lowercase. Each name should contain English letters only and has length from 1 to 10.
If there are multiple valid solutions, print any of them.
8 3
NO NO YES YES YES NO
Adam Bob Bob Cpqepqwer Limak Adam Bob Adam
9 8
YES NO
R Q Ccccccccc Ccocc Ccc So Strong Samples Ccc
3 2
NO NO
Na Na Na
In the first sample, there are 8 soldiers. For every 3 consecutive ones we know whether they would be an effective group. Let's analyze the provided sample output:
- First three soldiers (i.e. Adam, Bob, Bob) wouldn't be an effective group because there are two Bobs. Indeed, the string s1 is "NO".
- Soldiers 2 through 4 (Bob, Bob, Cpqepqwer) wouldn't be effective either, and the string s2 is "NO".
- Soldiers 3 through 5 (Bob, Cpqepqwer, Limak) would be effective, and the string s3 is "YES".
- ...,
- Soldiers 6 through 8 (Adam, Bob, Adam) wouldn't be effective, and the string s6 is "NO".
题意:构造n个首字母大写长度不大于10的字符串 满足要求
YES 代表 从当前点开始的m个字符串不相同
题解:思维 初始n个字符串都不同
当遇到NO 只需要更改第m个为第一个
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 #define bug() printf("!!!!!!!") 14 #define M 100005 15 using namespace std; 16 int n,k; 17 char a[15]; 18 map<int,int> mp; 19 map<int,string> mpp; 20 int main() 21 { 22 mpp[1]="A"; 23 for(int i=2;i<=8;i++) 24 { 25 mpp[i]=mpp[i-1]+"a"; 26 } 27 mpp[9]="B"; 28 for(int i=10;i<=18;i++) 29 { 30 mpp[i]=mpp[i-1]+"a"; 31 } 32 mpp[19]="C"; 33 for(int i=20;i<=28;i++) 34 { 35 mpp[i]=mpp[i-1]+"a"; 36 } 37 mpp[29]="D"; 38 for(int i=30;i<=38;i++) 39 { 40 mpp[i]=mpp[i-1]+"a"; 41 } 42 mpp[39]="E"; 43 for(int i=40;i<=48;i++) 44 { 45 mpp[i]=mpp[i-1]+"a"; 46 } 47 mpp[49]="F"; 48 for(int i=50;i<=58;i++) 49 { 50 mpp[i]=mpp[i-1]+"a"; 51 } 52 scanf("%d %d",&n,&k); 53 int now=1; 54 for(int i=1;i<=n;i++) 55 mp[i]=i; 56 for(int i=1;i<=n-k+1;i++) 57 { 58 scanf("%s",a); 59 if(strcmp(a,"NO")==0) 60 { 61 mp[i+k-1]=mp[i]; 62 } 63 } 64 for(int i=1;i<=n;i++) 65 cout<<mpp[mp[i]]<<" "; 66 cout<<endl; 67 return 0; 68 }