According to an old legeng, a long time ago Ankh-Morpork residents did something wrong to miss Fortune, and she cursed them. She said that at some time n snacks of distinct sizes will fall on the city, and the residents should build a Snacktower of them by placing snacks one on another. Of course, big snacks should be at the bottom of the tower, while small snacks should be at the top.
Years passed, and once different snacks started to fall onto the city, and the residents began to build the Snacktower.
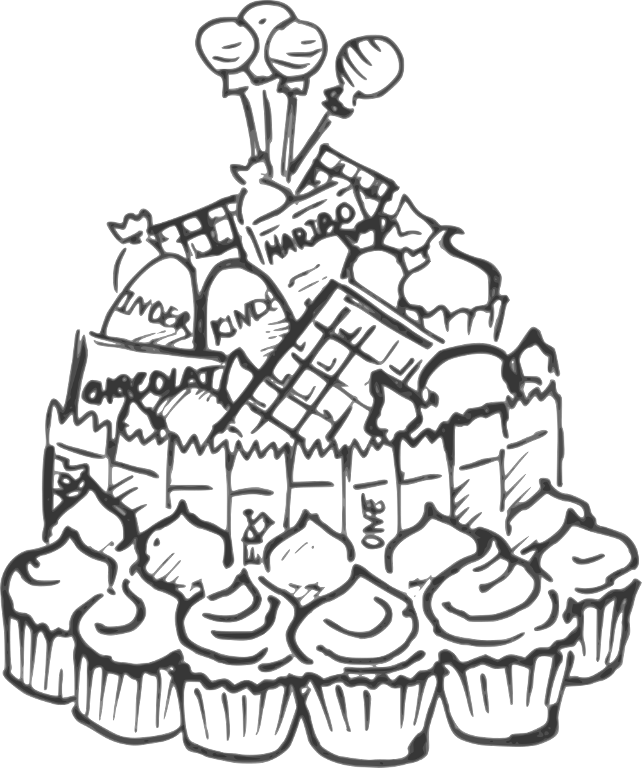
However, they faced some troubles. Each day exactly one snack fell onto the city, but their order was strange. So, at some days the residents weren't able to put the new stack on the top of the Snacktower: they had to wait until all the bigger snacks fell. Of course, in order to not to anger miss Fortune again, the residents placed each snack on the top of the tower immediately as they could do it.
Write a program that models the behavior of Ankh-Morpork residents.
The first line contains single integer n (1 ≤ n ≤ 100 000) — the total number of snacks.
The second line contains n integers, the i-th of them equals the size of the snack which fell on the i-th day. Sizes are distinct integers from 1 to n.
Print n lines. On the i-th of them print the sizes of the snacks which the residents placed on the top of the Snacktower on the i-th day in the order they will do that. If no snack is placed on some day, leave the corresponding line empty.
3
3 1 2
3
2 1
5
4 5 1 2 3
5 4
3 2 1
In the example a snack of size 3 fell on the first day, and the residents immediately placed it. On the second day a snack of size 1 fell, and the residents weren't able to place it because they were missing the snack of size 2. On the third day a snack of size 2 fell, and the residents immediately placed it. Right after that they placed the snack of size 1 which had fallen before.
题意:有一个1到n的全排列,按顺序每一秒会到达一个数,你要把它们从n到1叠起来,如果能叠,你就马上叠,叠起来的意思是逆序输出。不能叠就输出换行。
比如n=3到达顺序312 ,那么你第一秒就叠3,第三秒叠21。
题解:模拟
1 #include <bits/stdc++.h> 2 #include <iostream> 3 #include <cstdio> 4 #include <cstdlib> 5 #include <cstring> 6 #include <algorithm> 7 #include <stack> 8 #include <queue> 9 #include <cmath> 10 #include <map> 11 #define ll __int64 12 #define mod 1000000007 13 #define dazhi 2147483647 14 using namespace std; 15 int n; 16 int a[100005]; 17 map<int,int> mp; 18 int main() 19 { 20 scanf("%d",&n); 21 int maxn=0; 22 for(int i=1;i<=n;i++) 23 { 24 scanf("%d",&a[i]); 25 maxn=max(a[i],maxn); 26 } 27 mp[maxn]=1; 28 for(int i=1;i<=n;i++) 29 { 30 if(a[i]!=maxn) 31 { 32 printf("\n"); 33 mp[a[i]]=1; 34 } 35 else 36 { 37 mp[a[i]]=1; 38 while(mp[maxn]==1) 39 { 40 printf("%d ",maxn); 41 maxn--; 42 } 43 printf("\n"); 44 } 45 } 46 return 0; 47 }
Finally! Vasya have come of age and that means he can finally get a passport! To do it, he needs to visit the passport office, but it's not that simple. There's only one receptionist at the passport office and people can queue up long before it actually opens. Vasya wants to visit the passport office tomorrow.
He knows that the receptionist starts working after ts minutes have passed after midnight and closes after tf minutes have passed after midnight (so that (tf - 1) is the last minute when the receptionist is still working). The receptionist spends exactly t minutes on each person in the queue. If the receptionist would stop working within t minutes, he stops serving visitors (other than the one he already serves).
Vasya also knows that exactly n visitors would come tomorrow. For each visitor Vasya knows the point of time when he would come to the passport office. Each visitor queues up and doesn't leave until he was served. If the receptionist is free when a visitor comes (in particular, if the previous visitor was just served and the queue is empty), the receptionist begins to serve the newcomer immediately.
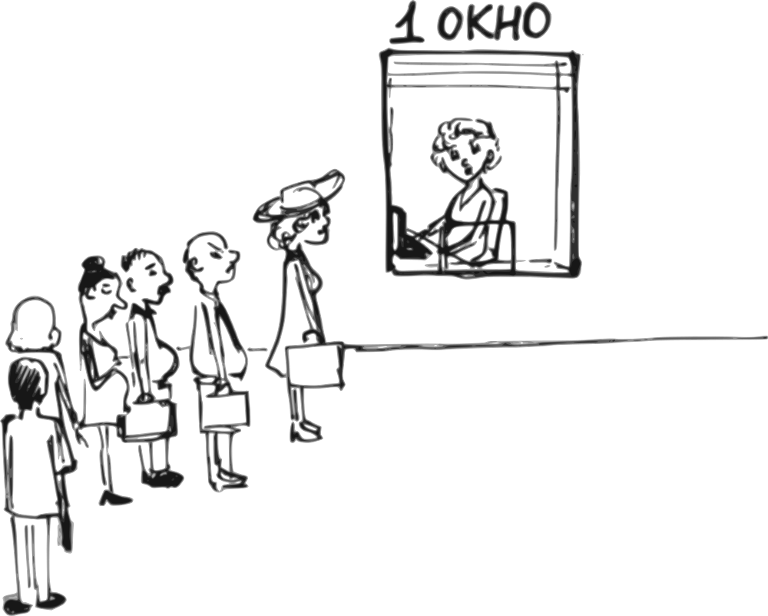
For each visitor, the point of time when he would come to the passport office is positive. Vasya can come to the office at the time zero (that is, at midnight) if he needs so, but he can come to the office only at integer points of time. If Vasya arrives at the passport office at the same time with several other visitors, he yields to them and stand in the queue after the last of them.
Vasya wants to come at such point of time that he will be served by the receptionist, and he would spend the minimum possible time in the queue. Help him!
The first line contains three integers: the point of time when the receptionist begins to work ts, the point of time when the receptionist stops working tf and the time the receptionist spends on each visitor t. The second line contains one integer n — the amount of visitors (0 ≤ n ≤ 100 000). The third line contains positive integers in non-decreasing order — the points of time when the visitors arrive to the passport office.
All times are set in minutes and do not exceed 1012; it is guaranteed that ts < tf. It is also guaranteed that Vasya can arrive at the passport office at such a point of time that he would be served by the receptionist.
Print single non-negative integer — the point of time when Vasya should arrive at the passport office. If Vasya arrives at the passport office at the same time with several other visitors, he yields to them and queues up the last. If there are many answers, you can print any of them.
10 15 2
2
10 13
12
8 17 3
4
3 4 5 8
2
In the first example the first visitor comes exactly at the point of time when the receptionist begins to work, and he is served for two minutes. At 12 minutes after the midnight the receptionist stops serving the first visitor, and if Vasya arrives at this moment, he will be served immediately, because the next visitor would only come at 13 minutes after midnight.
In the second example, Vasya has to come before anyone else to be served.
题意:给你起始时间 终止时间 n个人到来的时刻 每个人办事需要的时长 问你什么时刻来办事 等待的时间最短。
题解:细节题 枚举每个人到来时刻的前一秒 找到等待最小值的时刻。
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 using namespace std; 14 ll ts,tf,t,n; 15 ll a[100005]; 16 ll b[100005]; 17 map<ll,int> mp; 18 ll ans=10000000000000; 19 int main() 20 { 21 scanf("%I64d %I64d %I64d",&ts,&tf,&t); 22 scanf("%d",&n); 23 int jishu=1; 24 ll re; 25 for(int i=1; i<=n; i++) 26 { 27 scanf("%I64d",&a[i]); 28 mp[a[i]]++; 29 if(mp[a[i]]==1) 30 b[jishu++]=a[i]; 31 } 32 if(b[1]!=0) 33 { 34 if(b[1]<=ts) 35 { 36 ans=ts-(b[1]-1); 37 re=b[1]-1; 38 } 39 else 40 { 41 ans=0; 42 re=ts; 43 } 44 } 45 else 46 { 47 if(mp[b[1]]==n) 48 ans=mp[b[1]]*t; 49 else 50 { 51 if(ts+mp[b[1]]*t<b[2]) 52 ans=0; 53 else 54 ans=ts+mp[b[1]]*t-(b[2]-1); 55 } 56 re=ans+ts; 57 } 58 ll now=max(b[1],ts)+mp[b[1]]*t; 59 for(int i=2; i<jishu; i++) 60 { 61 if(now>=tf) 62 break; 63 ll exm=now-(b[i]-1); 64 if(exm<0) 65 exm=0; 66 if(ans>exm) 67 { 68 ans=min(ans,max((ll)0,exm)); 69 re=min(now,b[i]-1); 70 } 71 now=max(now,b[i])+mp[b[i]]*t; 72 } 73 if(now<=(tf-t)) 74 printf("%I64d\n",now); 75 else 76 printf("%I64d\n",re); 77 return 0; 78 } 79 /* 80 2 10 2 81 3 82 2 4 5 83 2 10 2 84 4 85 2 4 5 15 86 4 5 1 87 1 88 7 89 100 200 50 90 2 91 20 150 92 */ 93 /* 94 61 1000000000 13 95 55 96 29 72 85 94 103 123 125 144 147 153 154 184 189 192 212 234 247 265 292 296 299 304 309 365 378 379 393 401 414 417 421 427 439 441 480 500 509 515 522 539 571 582 623 630 634 635 643 649 654 679 680 686 747 748 775 97 */
Once at New Year Dima had a dream in which he was presented a fairy garland. A garland is a set of lamps, some pairs of which are connected by wires. Dima remembered that each two lamps in the garland were connected directly or indirectly via some wires. Furthermore, the number of wires was exactly one less than the number of lamps.
There was something unusual about the garland. Each lamp had its own brightness which depended on the temperature of the lamp. Temperatures could be positive, negative or zero. Dima has two friends, so he decided to share the garland with them. He wants to cut two different wires so that the garland breaks up into three parts. Each part of the garland should shine equally, i. e. the sums of lamps' temperatures should be equal in each of the parts. Of course, each of the parts should be non-empty, i. e. each part should contain at least one lamp.

Help Dima to find a suitable way to cut the garland, or determine that this is impossible.
While examining the garland, Dima lifted it up holding by one of the lamps. Thus, each of the lamps, except the one he is holding by, is now hanging on some wire. So, you should print two lamp ids as the answer which denote that Dima should cut the wires these lamps are hanging on. Of course, the lamp Dima is holding the garland by can't be included in the answer.
The first line contains single integer n (3 ≤ n ≤ 106) — the number of lamps in the garland.
Then n lines follow. The i-th of them contain the information about the i-th lamp: the number lamp ai, it is hanging on (and 0, if is there is no such lamp), and its temperature ti ( - 100 ≤ ti ≤ 100). The lamps are numbered from 1 to n.
If there is no solution, print -1.
Otherwise print two integers — the indexes of the lamps which mean Dima should cut the wires they are hanging on. If there are multiple answers, print any of them.
6
2 4
0 5
4 2
2 1
1 1
4 2
1 4
6
2 4
0 6
4 2
2 1
1 1
4 2
-1
The garland and cuts scheme for the first example:
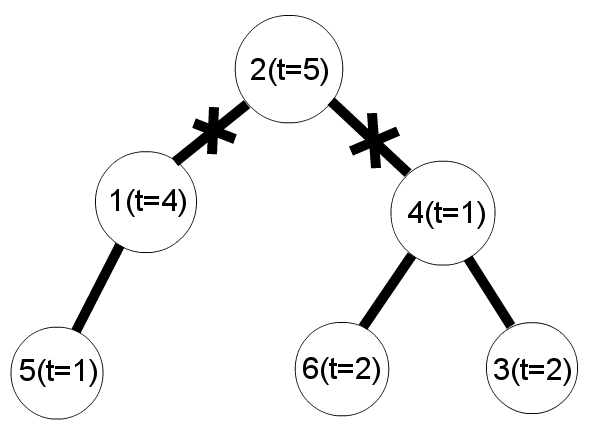
题意:有一棵给定根的树,有点权,要求剪掉两条边使得三个联通块权值和相等
题解:dfs处理 注意root
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 using namespace std; 14 int n; 15 int a[1000006]; 16 struct node 17 { 18 int ed; 19 int pre; 20 }N[1000006]; 21 int pre[1000006],he[1000006],mp[1000006]; 22 int nedge=0; 23 int to; 24 int sum=0; 25 int root; 26 vector<int>re; 27 void add(int st,int ed) 28 { 29 nedge++; 30 N[nedge].ed=ed; 31 N[nedge].pre=pre[st]; 32 pre[st]=nedge; 33 } 34 int getdfs(int x,int y) 35 { 36 he[x]=a[x]; 37 for(int i=pre[x];i;i=N[i].pre) 38 { 39 if(N[i].ed!=y) 40 { 41 he[x]+=getdfs(N[i].ed,x); 42 } 43 } 44 if(he[x]==sum&&x!=root){ 45 re.push_back(x); 46 he[x]=0; 47 } 48 return he[x]; 49 } 50 int main() 51 { 52 scanf("%d",&n); 53 54 for(int i=1;i<=n;i++) 55 { 56 scanf("%d %d",&to,&a[i]); 57 sum+=a[i]; 58 if(to==0) 59 root=i; 60 else{ 61 add(to,i); 62 } 63 } 64 if(sum%3!=0) 65 { 66 printf("-1\n"); 67 return 0; 68 } 69 sum=sum/3; 70 getdfs(root,root); 71 if(re.size()<2) 72 printf("-1\n"); 73 else 74 printf("%d %d\n",re[0],re[1]); 75 return 0; 76 }
Olya likes milk very much. She drinks k cartons of milk each day if she has at least k and drinks all of them if she doesn't. But there's an issue — expiration dates. Each carton has a date after which you can't drink it (you still can drink it exactly at the date written on the carton). Due to this, if Olya's fridge contains a carton past its expiry date, she throws it away.
Olya hates throwing out cartons, so when she drinks a carton, she chooses the one which expires the fastest. It's easy to understand that this strategy minimizes the amount of cartons thrown out and lets her avoid it if it's even possible.
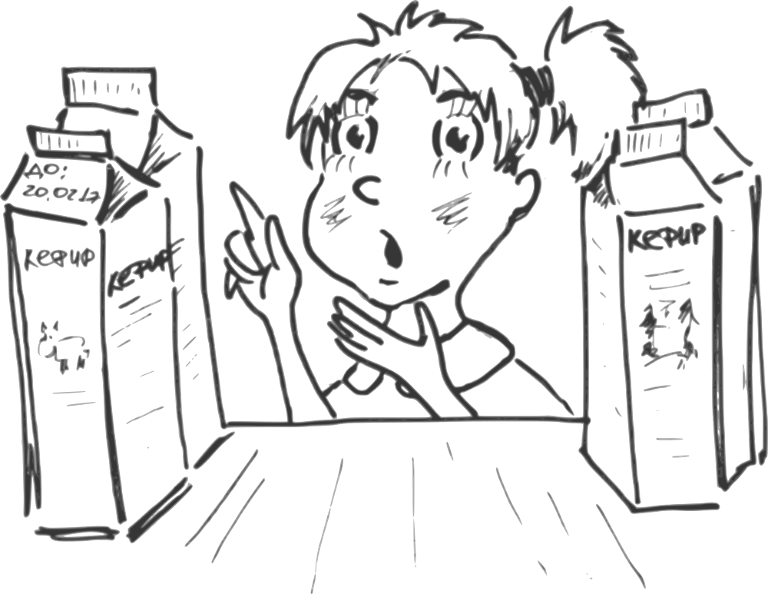
The main issue Olya has is the one of buying new cartons. Currently, there are n cartons of milk in Olya's fridge, for each one an expiration date is known (how soon does it expire, measured in days). In the shop that Olya visited there are m cartons, and the expiration date is known for each of those cartons as well.
Find the maximum number of cartons Olya can buy so that she wouldn't have to throw away any cartons. Assume that Olya drank no cartons today.
In the first line there are three integers n, m, k (1 ≤ n, m ≤ 106, 1 ≤ k ≤ n + m) — the amount of cartons in Olya's fridge, the amount of cartons in the shop and the number of cartons Olya drinks each day.
In the second line there are n integers f1, f2, ..., fn (0 ≤ fi ≤ 107) — expiration dates of the cartons in Olya's fridge. The expiration date is expressed by the number of days the drinking of this carton can be delayed. For example, a 0 expiration date means it must be drunk today, 1 — no later than tomorrow, etc.
In the third line there are m integers s1, s2, ..., sm (0 ≤ si ≤ 107) — expiration dates of the cartons in the shop in a similar format.
If there's no way for Olya to drink the cartons she already has in her fridge, print -1.
Otherwise, in the first line print the maximum number x of cartons which Olya can buy so that she wouldn't have to throw a carton away. The next line should contain exactly x integers — the numbers of the cartons that should be bought (cartons are numbered in an order in which they are written in the input, starting with 1). Numbers should not repeat, but can be in arbitrary order. If there are multiple correct answers, print any of them.
3 6 2
1 0 1
2 0 2 0 0 2
3
1 2 3
3 1 2
0 0 0
1
-1
2 1 2
0 1
0
1
1
In the first example k = 2 and Olya has three cartons with expiry dates 0, 1 and 1 (they expire today, tomorrow and tomorrow), and the shop has 3 cartons with expiry date 0 and 3 cartons with expiry date 2. Olya can buy three cartons, for example, one with the expiry date 0 and two with expiry date 2.
In the second example all three cartons Olya owns expire today and it means she would have to throw packets away regardless of whether she buys an extra one or not.
In the third example Olya would drink k = 2 cartons today (one she alreay has in her fridge and one from the shop) and the remaining one tomorrow.
题意:每瓶牛奶一个保质期Ai,然后必须在保质期前喝完它,每天只能喝k瓶牛奶。求最多可以从商店买几瓶。
题解:Ai范围很小,从后往前,让每一瓶冰箱里的牛奶尽可能晚喝掉,看看能否喝完冰箱里的。
如果能喝完,就把商店的牛奶排序一下,从后往前对每一个还能喝的天,都尽可能的买,即可。
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 using namespace std; 14 struct node 15 { 16 int w; 17 int pos; 18 }N[1000006]; 19 int f[10000007]; 20 int ans[1000006]; 21 int n,m,k; 22 bool cmp(struct node aa,struct node bb) 23 { 24 return aa.w<bb.w; 25 } 26 int main() 27 { 28 memset(f,0,sizeof(f)); 29 scanf("%d %d %d",&n,&m,&k); 30 int maxn=0; 31 int x; 32 for(int i=1;i<=n;i++) 33 { 34 scanf("%d",&x); 35 maxn=max(x,maxn); 36 f[x]++; 37 } 38 for(int i=1;i<=m;i++) 39 { 40 scanf("%d",&N[i].w); 41 N[i].pos=i; 42 } 43 sort(N+1,N+1+m,cmp); 44 for(int i=maxn;i>0;i--) 45 { 46 if(f[i]>=k) 47 { 48 f[i-1]+=f[i]-k; 49 f[i]=0; 50 } 51 else 52 { 53 f[i]=k-f[i]; 54 } 55 } 56 if(f[0]>k) 57 { 58 printf("-1\n"); 59 return 0; 60 } 61 else 62 f[0]=k-f[0]; 63 for(int i=maxn+1;i<=10000000;i++) f[i]=k; 64 int j=m; 65 int jishu=1; 66 for(int i=10000000;i>=0;i--) 67 { 68 while(j>=1&&N[j].w>=i&&f[i]>0) 69 { 70 f[i]--; 71 ans[jishu]=N[j].pos; 72 jishu++; 73 j--; 74 } 75 } 76 printf("%d\n",jishu-1); 77 for(int i=1;i<jishu;i++) 78 printf("%d ",ans[i]); 79 printf("\n"); 80 81 82 return 0; 83 }