徒手撸设计模式-状态模式
2022-07-02 16:34 hikoukay 阅读(21) 评论(0) 编辑 收藏 举报概念
在状态模式(State Pattern)中,类的行为是基于它的状态改变的。这种类型的设计模式属于行为型模式。
在状态模式中,我们创建表示各种状态的对象和一个行为随着状态对象改变而改变的 context 对象。
参考链接: https://www.runoob.com/design-pattern/state-pattern.html
代码案例
设计生产状态接口
/** * 生产状态 */ public interface ProductionState { String changeState(ProductionContext context); }
上下文对象类 存储生产对象
/** * 生产上下文 */ @Setter @Getter public class ProductionContext { private ProductionState productionState; public ProductionContext() { this.productionState = null; } }
三个生产状态实现类
开始生产 状态改变后更新上下文
/** * 开始生产状态 */ @Slf4j @Service("startState") public class ProductionStartState implements ProductionState { @Override public String changeState(ProductionContext context) { context.setProductionState(this); log.info("start to product goods"); return toString(); } @Override public String toString(){ return "start state"; } }
停止生产 状态改变后更新上下文
/** * 停止生产状态 */ @Slf4j @Service("stopState") public class ProductionStopState implements ProductionState { @Override public String changeState(ProductionContext context) { context.setProductionState(this); log.info("stop to product goods"); return toString(); } @Override public String toString(){ return "stop state"; } }
结束生产 状态改变后更新上下文
/** * 开始生产状态 */ @Slf4j @Service("endState") public class ProductionEndState implements ProductionState { @Override public String changeState(ProductionContext context) { context.setProductionState(this); log.info("end to product goods"); return toString(); } @Override public String toString(){ return "end state"; } }
测试主类
/** * 设计模式控制器 */ @RestController @RequestMapping("/designPattern") @Slf4j public class DesignController { @Autowired private ApplicationContext applicationContext; @PostMapping("/state") public ResponseModel state(@RequestBody String[] status) throws Exception { log.info("state ---- start "); List list = new ArrayList(); //创建上下文 ProductionContext productionContext = new ProductionContext(); for (String state : status) { Object bean = applicationContext.getBean(state); if (bean instanceof ProductionStartState){ ProductionStartState startState= (ProductionStartState) bean; startState.changeState(productionContext); }else if (bean instanceof ProductionStopState){ ProductionStopState stopState= (ProductionStopState) bean; stopState.changeState(productionContext); }else if (bean instanceof ProductionEndState){ ProductionEndState endState= (ProductionEndState) bean; endState.changeState(productionContext); } String result = productionContext.getProductionState().toString(); log.info("current state is: {}",result); list.add(result); } /*//初始化开始状态 ProductionStartState startState = new ProductionStartState(); //上下文状态设置为初始状态 startState.changeState(productionContext); log.info(productionContext.getProductionState().toString()); //初始化停止状态 ProductionStopState stopState = new ProductionStopState(); //上下文状态设置为停止状态 stopState.changeState(productionContext); log.info(productionContext.getProductionState().toString());*/ log.info("state ---- end "); return new ResponseModel("状态模式完成", 200, list); } }
测试案例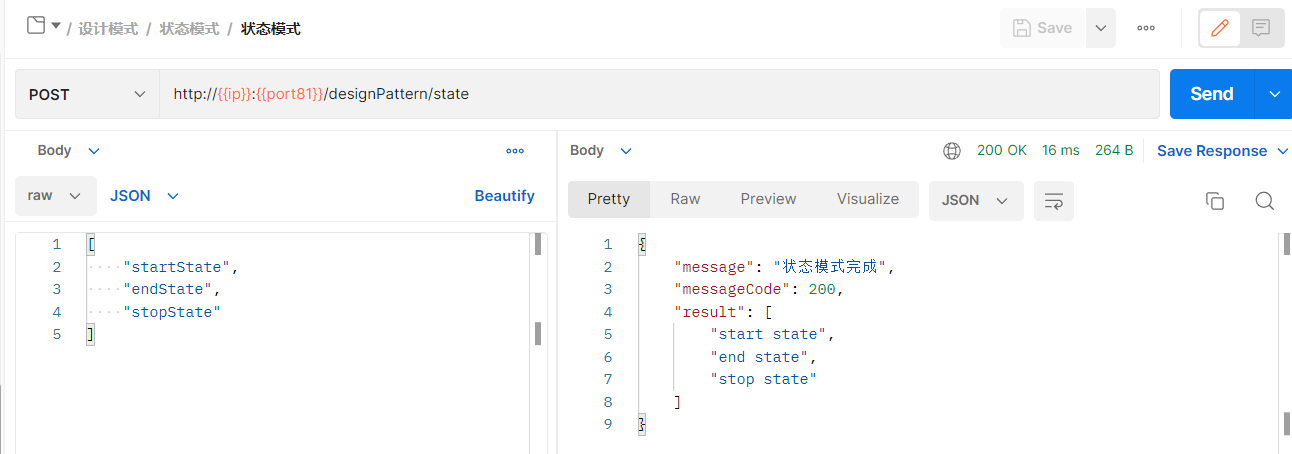
2022-07-02 16:20:33.329 INFO state ---- start 【http-nio-8081-exec-6】【DesignController:84】 2022-07-02 16:20:33.329 INFO start to product goods 【http-nio-8081-exec-6】【ProductionStartState:17】 2022-07-02 16:20:33.329 INFO current state is: start state 【http-nio-8081-exec-6】【DesignController:103】 2022-07-02 16:20:33.330 INFO end to product goods 【http-nio-8081-exec-6】【ProductionEndState:17】 2022-07-02 16:20:33.330 INFO current state is: end state 【http-nio-8081-exec-6】【DesignController:103】 2022-07-02 16:20:33.330 INFO stop to product goods 【http-nio-8081-exec-6】【ProductionStopState:17】 2022-07-02 16:20:33.330 INFO current state is: stop state 【http-nio-8081-exec-6】【DesignController:103】 2022-07-02 16:20:33.331 INFO state ---- end 【http-nio-8081-exec-6】【DesignController:118】