CSS基础-边框和圆角
边框和圆角
边框
CSS中用border 定义边框属性。
border语法:border:[宽度][样式][颜色]
其中:
-
宽度:边框的宽度,单位可以使px、em、rem 等单位,也可以使用thin、medium、thick 三种预设值
-
样式:边框的样式,值可以是 solid(实线),dashed(虚线),dotted(点线)等多种样式。
样式值 意思 solid 实线 dashed 虚线 dotted 点状线 -
颜色:边框的颜色,可以使用颜色的英文名称、十六进制值、RGB、RGBA等多种方式来指定
示例
创建一个宽度为1px,线型为实线, 颜色为红色边框的盒子。
<style>
.box {
width: 200px;
height: 200px;
margin: 0 auto;
border: 1px solid red;
}
</style>
<body>
<div class="box"></div>
</body>
单独表示 边框的宽度、线型、颜色
border
三要素可以拆分成小属性进行替换。
border-width
: 边框的宽度。border-style
: 边框的样式(线型)。border-color
: 边框的颜色。
示例
border-width: 2px;
border-style: dashed;
border-color: blue;
单独表示每个方向的边框
border 属性可以通过指定四个方向的值,来分别表示每个边框的样式。
属性 | 意义 |
---|---|
border-top | 上边框 |
border-right | 右边框 |
border-bottom | 下边框 |
border-left | 左边框 |
四个边框的属性演示
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>演示四个边框属性</title>
<style>
.box1 {
width: 100px;
height: 100px;
border-top: 2px solid red;
border-bottom: 3px dotted green;
border-left: 3px dashed yellowgreen;
border-right: 2px solid #89d8de;
}
</style>
</head>
<body>
<div class="box1"></div>
</body>
</html>
每个边框的样式、线型和颜色单独指定
CSS中关于边框的设置还有更细粒度的属性。
以下属性可以分别设置每个边框的样式、线型和颜色。
属性 | 意思 |
---|---|
border-top-width | 上边框宽度 |
border-top-style | 上边框线型 |
border-top-color | 上边框颜色 |
border-right-width | 右边框宽度 |
border-right-style | 右边框线型 |
border-right-color | 右边框颜色 |
border-bottom-width | 下边框宽度 |
border-bottom-style | 下边框线型 |
border-bottom-color | 下边框颜色 |
border-left-width | 左边框宽度 |
border-left-style | 左边框线型 |
border-left-color | 左边框颜色 |
举例
在所有div标签盒子的样式确定好后,我们想微调 class=“box” 属性的上边框样式。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>边框小属性演示</title>
<style>
div {
width: 200px;
height: 200px;
border: 2px solid green;
}
.box {
border-top-width: 2px;
border-top-color: red;
border-top-style: dotted;
}
</style>
</head>
<body>
<div class="box"></div>
</body>
</html>
去掉边框
- border:none; 去掉盒子所有的边框(默认值)。
- border-left:none: 去掉盒子左边的边框。
- border-top:none; 去掉盒子的上边框。
- border-right:none; 去掉盒子的右边框。
- border-bottom:none; 去掉盒子底部的边框。
举例
去掉盒子的左边框
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>去掉盒子的左边框</title>
<style>
div {
width: 200px;
height: 200px;
background-color: orange;
border: 3px solid red;
}
.box {
border-left: none;
}
</style>
</head>
<body>
<div class="box"></div>
</body>
</html>
圆角
CSS中用 border-radius 属性设置圆角 , 常用单位:px、百分比等。
语法:
# 如果只指定一个值,表示四个角都使用该值。
border-radius: 10px;
# 如果有两个值, 第一个值作用在左上角 和 右下角 , 第二个值作用在 右上角 和 左下角
border-radius: 20px 50px;
# 如果有三个值, 第一个值作用在左上角 ,第二个值作用在右上角 和 左下角 , 第三个值适用于右下角
border-radius: 15px 50px 30px;
# 如果有四个值, 第一个值 左上角, 第二个值 右上角, 第三个值 右下角 , 第四个值适用于左下角
border-radius: 15px 50px 30px 5px
在一个正方形盒子中,当我们使用如下的属性的时候
border-radius: 10px;
其实我们就构建除了一个半径为 10px 的圆形。
给一个正方形盒子设置圆角为百分比 50% ,就可以得到一个圆形。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>圆角百分比表示</title>
<style>
.box {
height: 100px;
width: 100px;
border: 1px solid red;
border-radius: 50%;
}
</style>
</head>
<body>
<div class="box"></div>
</body>
</html>
单独设置某个角
属性 | 意义 |
---|---|
border-top-left-radius | 左上角 |
border-top-right-radius | 右上角 |
border-bottom-left-radius | 左下角 |
border-bottom-right-radius | 右上角 |
请关于一下啦^_^
微信公众号
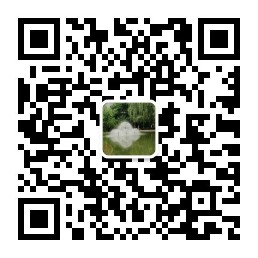