lambda 匿名函数,map,filter,reduce,zip,介绍
sum_1=lambda x,y:x+y
print(sum_1(1,2))
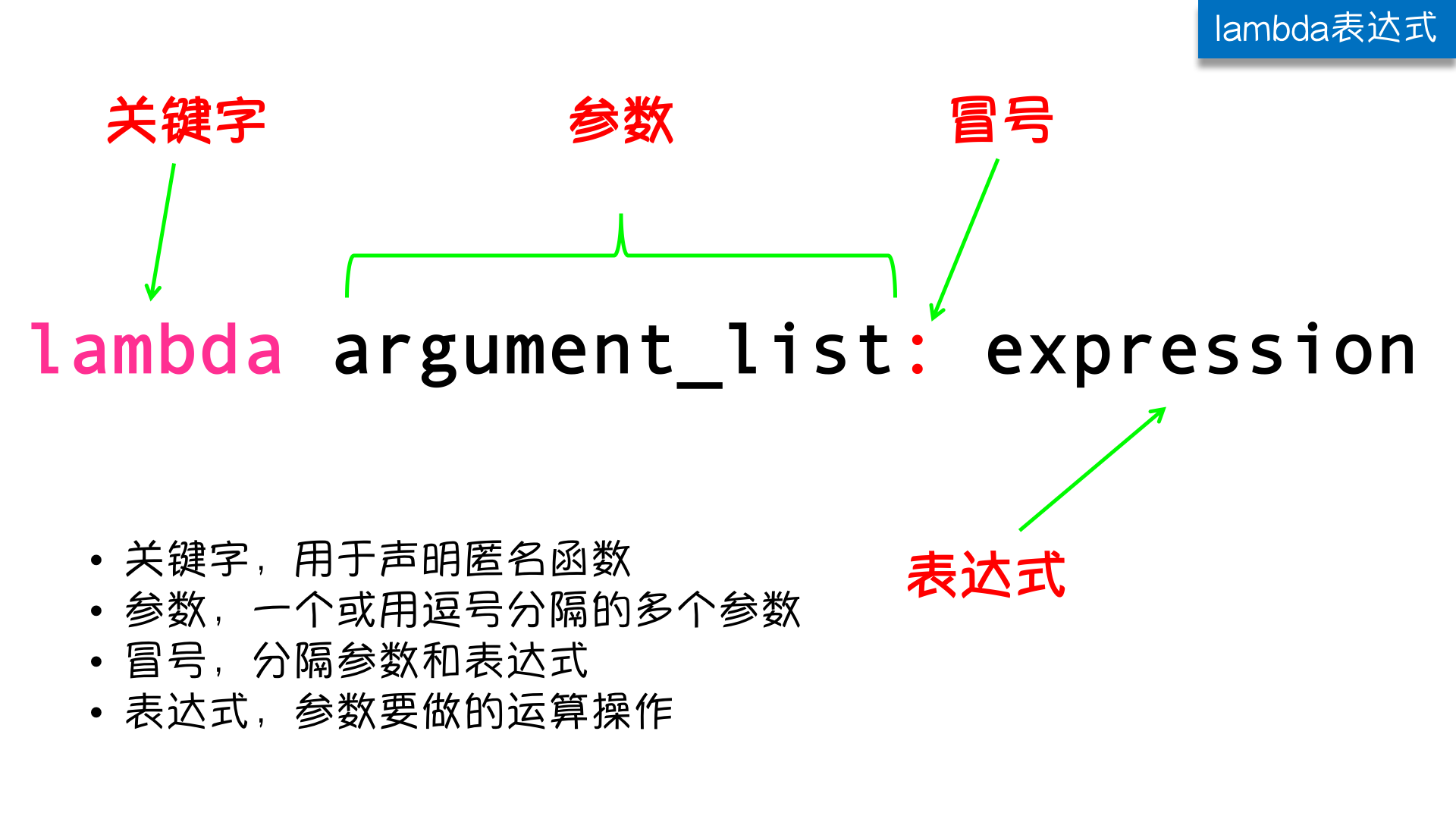
l=[1,2,3,4]
print(list(map(lambda x:x*4,l)))
#map()将函数func应用于序列seq中的所有元素。
# 在Python3之前,map()返回一个列表,
# 列表中的每个元素都是将列表或元组“seq”中的相应元素传入函数func返回的结果
#使用map就相当于使用了一个for循环
def my_map(func,seq):
result=[]
for i in seq:
result.append(func(i))
return result
l=[1,2,3,4]
print(list(my_map(lambda x:x+100,l)))
#########
def my_map(func,seq):
for i in seq:
yield func(i)
l=[1,2,3,4]
print(list(my_map(lambda x:x+100,l)))
#可以给map函数传入多个序列参数,它将并行的序列作为不同参数传入函数
print(list(map(pow,[2,3,4],[10,11,12])))
from operator import add
x=[1,2,3]
y=[4,5,6]
print(list(map(add,[1,2,3],[4,5,6]))) #注意格式
############
#filter函数实现一个过滤的功能
#判断大小
li=[30,11,77,8,25,65,4]
print(list(filter(lambda x:x>33,li)))
#判断两个列表的交集
x=[1,2,3,4,5]
y=[3,4,5,6,7]
print(list(filter(lambda a: a in x,y)))
#reduce同样是接收两个参数:func(函数)和seq(序列,如list)
#reduce最后返回的不是一个迭代器,它返回一个值
#reduce首先将序列中的前两个元素,传入func中,
# 再将得到的结果和第三个元素一起传入func,…,
# 这样一直计算到最后,得到一个值,把它作为reduce的结果返回
#reduce 求和
from functools import reduce
print(reduce(lambda x,y:x+y,[1,2,3,4]))
#求1-100 的和
from functools import reduce
print(reduce(lambda x,y:x+y,range(1,101)))
#三元运算
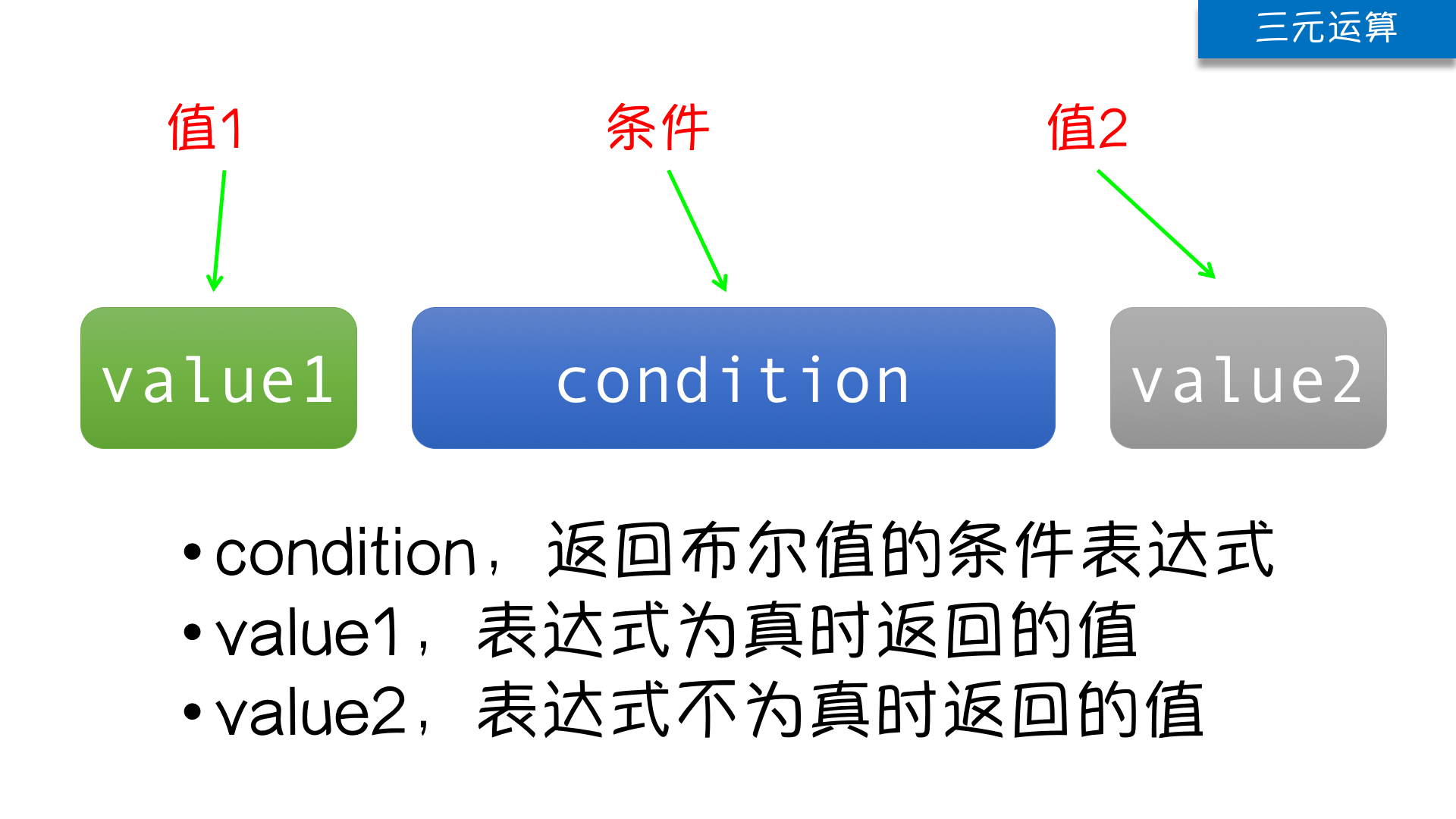
#三元运算(三目运算)在Python中也叫条件表达式。
# 三元运算的语法非常简单,主要是基于True/False的判断
#使用它就可以用简单的一行快速判断,# 而不再需要使用复杂的多行if语句
from functools import reduce
l=[30,11,33,44,66,232,6]
t=(2,3,5)
print(reduce(lambda x,y:x if x>y else y,l))
print(reduce(lambda x,y:x if x>y else y,t))
print(max(max(2,6),5))
#利用max函数求最大值#三元运算配合lambda表达式和map,
将一个列表里面的奇数加100
print(list(map(lambda x: x+100 if x%2 else x,l)))
#zip函数接收一个或多个可迭代对象作为参数,
最后返回一个迭代器
x=['a','b','c']
y=[1,2,3]
a=list(zip(x,y))
print(a)
b=list(zip(*a))
print(b)#
zip(x, y) 会生成一个可返回元组 (m, n) 的迭代器,
# 其中m来自x,n来自y。 一旦其中某个序列迭代结束,# 迭代就宣告结束。
因此迭代长度跟参数中最短的那个序列长度一致
x=[1,2,3,4,5]
y=[9,5,6]
for m,n in zip(x,y):
print(m,n)
# 1 9
# 2 5
# 3 6
itertools.zip_longest()
from itertools import zip_longestfor
m,n in zip_longest(x,y):
print(m,n)
#1 9# 2 5# 3 6# 4 None# 5 None#
其他应用
keys = ['name','age','salary']
values=['andy',12,1111]
d=dict(zip(keys,values))
print(d)