package text1;
import java.sql.*;
public class JDBCutils {
//获取连接对象的方法
public static Connection getcon() throws Exception {
Connection con=null;
Class.forName("com.mysql.jdbc.Driver");
con= DriverManager.getConnection("jdbc:mysql://localhost:3306/jdbc",
"root",
"root");
return con;
}
//关闭连接 释放资源
public static void realse(ResultSet rs,Statement stmt,Connection con) {
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
if (stmt != null) {
try {
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
if (con != null) {
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
}
}
package text1;
import java.util.Date;
public class user {
private int id;
private String username;
private String password;
private String email;
private String birthday;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
}
package text1;
import org.apache.tomcat.jni.User;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import java.sql.Connection;
import java.sql.Statement;
//完成对数据库的增删改查操作
public class usersdao {
//提供一个添加方法
public boolean insert(user user){
Connection con=null;
Statement stmt=null;
try{
//获取连接对象
con=JDBCutils.getcon();
//获取执行sql语句的对象
stmt=con.createStatement();
String sql="insert into yonghu "+"values('"
+user.getId()+"','"
+user.getUsername()+"','"
+user.getPassword()+"','"
+user.getEmail()+"','"
+user.getBirthday()+"'"
+")";
int a =stmt.executeUpdate(sql);
if(a==1){
return true;
}
}catch (Exception e){
/* throw new RuntimeException(e);*/
e.printStackTrace();
}finally {
JDBCutils.realse(null,stmt,con);
}
return false;
}
//提供查询所有的方法
public List<user> findalluser(){
Connection con=null;
ResultSet rs=null;
Statement stmt=null;
try {
con=JDBCutils.getcon();
stmt=con.createStatement();
String sql="select * from yonghu";
rs=stmt.executeQuery(sql);
/* while (rs.next()){
int num=rs.getInt("id");
String username=rs.getString("username");
}*/
List<user> list=new ArrayList<user>();
while (rs.next()){
user user=new user();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setEmail(rs.getString("email"));
user.setBirthday(rs.getString("birthday"));
list.add(user);
}
return list;
}catch (Exception e){
e.printStackTrace();
}finally {
JDBCutils.realse(rs,stmt,con);
}
return null;
}
//根据id来查询记录
public user finduserbyid(int id){
Connection con=null;
PreparedStatement stme=null;
ResultSet rs=null;
try{
con=JDBCutils.getcon();
String sql="select * from yonghu where id=?";
stme=con.prepareStatement(sql);
//给占位符赋值
stme.setInt(1,id);
rs=stme.executeQuery();
while (rs.next()){
user user=new user();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setEmail(rs.getString("email"));
user.setBirthday(rs.getString("birthday"));
return user;
}
}catch (Exception e){
e.printStackTrace();
}finally {
JDBCutils.realse(rs,stme,con);
}
return null;
}
//提供根基id修改数据方法
public boolean update(user user){
Connection con=null;
PreparedStatement stmt=null;
try {
con=JDBCutils.getcon();
String sql="update yonghu set username=?,password=? where id =?";
stmt=con.prepareStatement(sql);
stmt.setString(1,user.getUsername());
stmt.setString(2,user.getPassword());
stmt.setInt(3,user.getId());
int row=stmt.executeUpdate();
if(row==1){
return true;
}
}catch (Exception e){
e.printStackTrace();
}finally {
JDBCutils.realse(null,stmt,con);
}
return false;
}
//提供删除方法
public boolean delete(int id){
Connection con=null;
PreparedStatement stmt=null;
try{
con=JDBCutils.getcon();
String sql="delete from yonghu where id=?";
stmt=con.prepareStatement(sql);
stmt.setInt(1,id);
if(stmt.executeUpdate()==1){
return true;
}
}catch (Exception e){
e.printStackTrace();
}finally {
JDBCutils.realse(null,stmt,con);
}
return false;
}
}
package text1;
import java.util.List;
public class jdbcinserttext {
public static void main(String[] args) {
usersdao dao=new usersdao();
user user=new user();
user.setId(2);
user.setUsername("guoyang");
user.setPassword("4560");
user.setEmail("guo@qq.com");
user.setBirthday("2020-4-20");
dao.insert(user);
package text1;
public class deleteusertest {
public static void main(String[] args) {
usersdao dao=new usersdao();
user u=new user();
boolean f=dao.delete(1);
System.out.println(f);
}
}
package text1;
public class updateusertest {
public static void main(String[] args) {
usersdao dao=new usersdao();
user u=new user();
u.setId(18);
u.setUsername("guoyang");
u.setPassword("777777");
boolean f=dao.update(u);
System.out.println(f);
}
}
package text1;
import java.util.List;
public class findallusertest {
public static void main(String[] args) {
usersdao dao=new usersdao();
user u=dao.finduserbyid(18);
System.out.println(u.getUsername());
}
}
package text1;
public class finduserbyidtest {
public static void main(String[] args) {
usersdao dao=new usersdao();
user u=dao.finduserbyid(18);
System.out.println(u.getUsername());
}
}
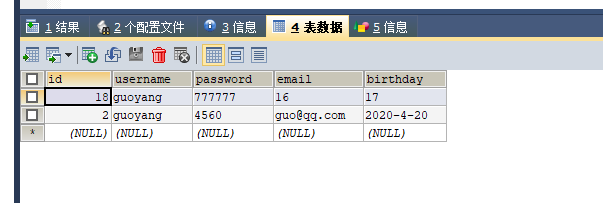
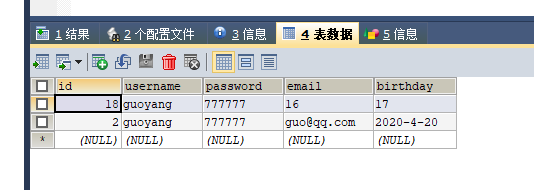
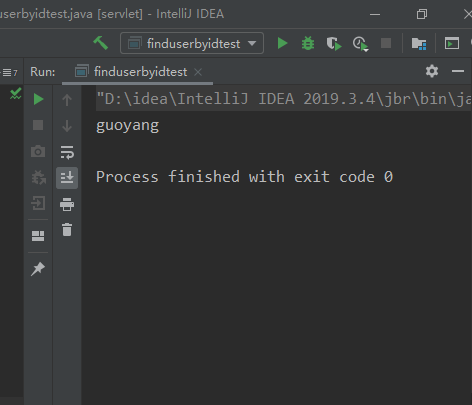
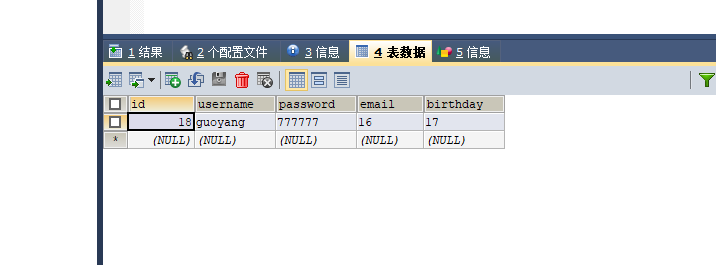