poj1981 Circle and Points 单位圆覆盖问题
转载请注明出处: http://www.cnblogs.com/fraud/ ——by fraud
Circle and Points
Time Limit: 5000MS | Memory Limit: 30000K | |
Total Submissions: 6850 | Accepted: 2443 | |
Case Time Limit: 2000MS |
Description
You are given N points in the xy-plane. You have a circle of radius one and move it on the xy-plane, so as to enclose as many of the points as possible. Find how many points can be simultaneously enclosed at the maximum. A point is considered enclosed by a circle when it is inside or on the circle.
Fig 1. Circle and Points
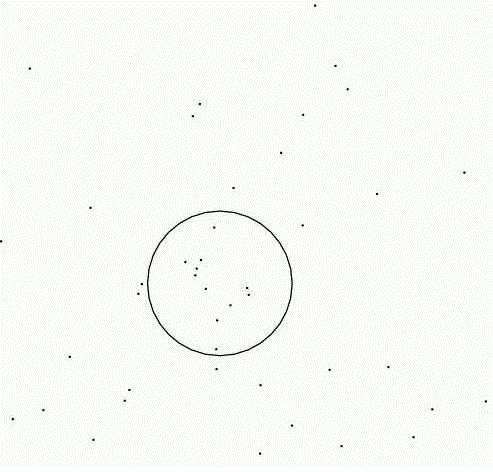
Fig 1. Circle and Points
Input
The input consists of a series of data sets, followed by a single line only containing a single character '0', which indicates the end of the input. Each data set begins with a line containing an integer N, which indicates the number of points in the data set. It is followed by N lines describing the coordinates of the points. Each of the N lines has two decimal fractions X and Y, describing the x- and y-coordinates of a point, respectively. They are given with five digits after the decimal point.
You may assume 1 <= N <= 300, 0.0 <= X <= 10.0, and 0.0 <= Y <= 10.0. No two points are closer than 0.0001. No two points in a data set are approximately at a distance of 2.0. More precisely, for any two points in a data set, the distance d between the two never satisfies 1.9999 <= d <= 2.0001. Finally, no three points in a data set are simultaneously very close to a single circle of radius one. More precisely, let P1, P2, and P3 be any three points in a data set, and d1, d2, and d3 the distances from an arbitrarily selected point in the xy-plane to each of them respectively. Then it never simultaneously holds that 0.9999 <= di <= 1.0001 (i = 1, 2, 3).
You may assume 1 <= N <= 300, 0.0 <= X <= 10.0, and 0.0 <= Y <= 10.0. No two points are closer than 0.0001. No two points in a data set are approximately at a distance of 2.0. More precisely, for any two points in a data set, the distance d between the two never satisfies 1.9999 <= d <= 2.0001. Finally, no three points in a data set are simultaneously very close to a single circle of radius one. More precisely, let P1, P2, and P3 be any three points in a data set, and d1, d2, and d3 the distances from an arbitrarily selected point in the xy-plane to each of them respectively. Then it never simultaneously holds that 0.9999 <= di <= 1.0001 (i = 1, 2, 3).
Output
For each data set, print a single line containing the maximum number of points in the data set that can be simultaneously enclosed by a circle of radius one. No other characters including leading and trailing spaces should be printed.
Sample Input
3 6.47634 7.69628 5.16828 4.79915 6.69533 6.20378 6 7.15296 4.08328 6.50827 2.69466 5.91219 3.86661 5.29853 4.16097 6.10838 3.46039 6.34060 2.41599 8 7.90650 4.01746 4.10998 4.18354 4.67289 4.01887 6.33885 4.28388 4.98106 3.82728 5.12379 5.16473 7.84664 4.67693 4.02776 3.87990 20 6.65128 5.47490 6.42743 6.26189 6.35864 4.61611 6.59020 4.54228 4.43967 5.70059 4.38226 5.70536 5.50755 6.18163 7.41971 6.13668 6.71936 3.04496 5.61832 4.23857 5.99424 4.29328 5.60961 4.32998 6.82242 5.79683 5.44693 3.82724 6.70906 3.65736 7.89087 5.68000 6.23300 4.59530 5.92401 4.92329 6.24168 3.81389 6.22671 3.62210 0
Sample Output
2 5 5 11
题目就是给你n个点,然后用一个单位圆去覆盖,问最多同时能够覆盖到几个点
对于这个题目,首先很容易想到一种n^3的解法,那就是先枚举两个点,这两个点能够得到一个或者两个单位圆。然后在枚举所有点来统计一遍。
方法比较简单,我就不贴代码了。
对于此题,还有一种O(n^2logn)的解法。
首先以每个点为圆心 ,做一个单位圆,那么被同时覆盖到单位圆的数目最多的地方,就是我们需要求的圆心的地方。
于是,我们可以依次枚举所有圆,然后,对于每一个圆,我们在枚举其他的圆,如果有交点的话,求出两个交点,然后按照极角排序,求出被覆盖次数的最多的圆弧,因为被覆盖次数最多的圆弧也就是目标圆心的位置。
求极角的时候可以利用atan2函数,然后加上或者减去一个acos的角度即可
注意atan2使用方法

1 /** 2 * code generated by JHelper 3 * More info: https://github.com/AlexeyDmitriev/JHelper 4 * @author xyiyy @https://github.com/xyiyy 5 */ 6 7 #include <iostream> 8 #include <fstream> 9 10 //##################### 11 //Author:fraud 12 //Blog: http://www.cnblogs.com/fraud/ 13 //##################### 14 //#pragma comment(linker, "/STACK:102400000,102400000") 15 #include <iostream> 16 #include <sstream> 17 #include <ios> 18 #include <iomanip> 19 #include <functional> 20 #include <algorithm> 21 #include <vector> 22 #include <string> 23 #include <list> 24 #include <queue> 25 #include <deque> 26 #include <stack> 27 #include <set> 28 #include <map> 29 #include <cstdio> 30 #include <cstdlib> 31 #include <cmath> 32 #include <cstring> 33 #include <climits> 34 #include <cctype> 35 36 using namespace std; 37 #define mp(X, Y) make_pair(X,Y) 38 #define rep(X, N) for(int X=0;X<N;X++) 39 40 // 41 // Created by xyiyy on 2015/8/10. 42 // 43 44 #ifndef JHELPER_EXAMPLE_PROJECT_P_HPP 45 #define JHELPER_EXAMPLE_PROJECT_P_HPP 46 47 const double EPS = 1e-9; 48 49 double add(double a, double b) { 50 if (fabs(a + b) < EPS * (fabs(a) + fabs(b)))return 0; 51 return a + b; 52 } 53 54 class P { 55 public: 56 double x, y; 57 58 P() { } 59 60 P(double x, double y) : x(x), y(y) { } 61 62 P operator+(const P &p) { 63 return P(add(x, p.x), add(y, p.y)); 64 } 65 66 P operator-(const P &p) { 67 return P(add(x, -p.x), add(y, -p.y)); 68 } 69 70 P operator*(const double &d) { 71 return P(x * d, y * d); 72 } 73 74 P operator/(const double &d) { 75 return P(x / d, y / d); 76 } 77 78 double dot(P p) { 79 return add(x * p.x, y * p.y); 80 } 81 82 83 double abs() { 84 return sqrt(abs2()); 85 } 86 87 double abs2() { 88 return dot(*this); 89 } 90 91 }; 92 93 94 95 //直线和直线的交点 96 /*P isLL(P p1,P p2,P q1,P q2){ 97 double d = (q2 - q1).det(p2 - p1); 98 if(sig(d)==0)return NULL; 99 return intersection(p1,p2,q1,q2); 100 }*/ 101 102 103 //四点共圆判定 104 /*bool onC(P p1,P p2,P p3,P p4){ 105 P c = CCenter(p1,p2,p3); 106 if(c == NULL) return false; 107 return add((c - p1).abs2(), -(c - p4).abs2()) == 0; 108 }*/ 109 110 //三点共圆的圆心 111 112 113 #endif //JHELPER_EXAMPLE_PROJECT_P_HPP 114 115 const int MAXN = 710; 116 P ps[MAXN]; 117 pair<double, bool> arc[MAXN]; 118 119 class poj1981 { 120 public: 121 void solve(std::istream &in, std::ostream &out) { 122 int n; 123 P t; 124 while (in >> n && n) { 125 rep(i, n)in >> ps[i].x >> ps[i].y; 126 int ans = 1; 127 rep(i, n) { 128 int num = 0; 129 rep(j, n) { 130 if (i == j)continue; 131 double d; 132 if ((d = (ps[i] - ps[j]).abs()) <= 2) { 133 double a = acos(d / 2); 134 double b = atan2((ps[j].y - ps[i].y), (ps[j].x - ps[i].x)); 135 arc[num++] = mp(b - a, 1); 136 arc[num++] = mp(b + a, 0); 137 } 138 } 139 sort(arc, arc + num); 140 int res = 1; 141 rep(j, num) { 142 if (arc[j].second)res++; 143 else res--; 144 ans = max(ans, res); 145 } 146 } 147 out << ans << endl; 148 } 149 } 150 }; 151 152 int main() { 153 std::ios::sync_with_stdio(false); 154 std::cin.tie(0); 155 poj1981 solver; 156 std::istream &in(std::cin); 157 std::ostream &out(std::cout); 158 solver.solve(in, out); 159 return 0; 160 }