javascript原生技巧篇
有条件给对象添加属性
const bool = true;
const obj1 = {
id: 1,
name: 'JOho me',
...(bool && {sex: 12})
};
reduce 和find的巧妙用法
统计total并且删除对象数组中重复项
const data = [
{tam: "S", color: "blue", total: 5},
{tam: "S", color: "blue", total: 10},
{tam: "S", color: "blue", total: 20},
{tam: "M", color: "blue", total: 5},
{tam: "L", color: "blue", total: 5},
{tam: "S", color: "blue", total: 20},
];
const result = data.reduce((acc, curr) => {
const objInAcc = acc.find((o) => o.tam === curr.tam && o.color === curr.color);
if (objInAcc) objInAcc.total += curr.total;
else acc.push(curr);
return acc;
}, []);
属性hidden
显示隐藏, boolean
<!--隐藏啦-->
<h1 [hidden]="true">我是一个div</h1>
<!--显示啦-->
<h1 [hidden]="false">我是一个div-false</h1>
从别人的分支拉别人的组件到自己分支上
先切换到别人的分支(相当于把别人分支的代码下载到本地啦)
然后回到自己的分支
git checkout 别人的分支名 src/文件路径
Date的小问题
new Date(2019,10,18) //月份是索引0
Mon Nov 18 2019 00:00:00 GMT+0800 (中国标准时间)
new Date('2019-10-18')
Fri Oct 18 2019 08:00:00 GMT+0800 (中国标准时间)
new Date('2019-11-18')
Mon Nov 18 2019 08:00:00 GMT+0800 (中国标准时间)
获取焦点的样式问题
js
.focus();
css
.xxx:focus{
outline:0
}
不让浏览器翻译当前文字
<h1 translate="no">你是个孩子吗?</h1>
angular 判断可以else
*ngIf="bool;else Template"
cowsay 一直会说话的牛
npm install -g cowsay
控制台输入
cowthink 我爱js
SVG 线条的技巧
<path d="M66.039,133.545 ... " pathLength="1" />
然后我们设置stroke-dasharray
为1
.path {
stroke-dasharray: 1;
stroke-dashoffset: 1;
animation: dash 5s linear alternate infinite;
}
@keyframes dash {
from {
stroke-dashoffset: 1;
}
to {
stroke-dashoffset: 0;
}
}
完整的代码
for /await
const add1 = (res1, time) => new Promise(res => setTimeout(() => res(res1), time));
const add2 = [add1('111', 1000), add1('222', 700), add1('333', 500)];
async function add3(arr) {
for await(const item of arr){
console.log(item);
}
}
add3(add2);
// 111 222 333
Array.prototype.at()
采用整数值并返回该索引处的项目,允许正整数和负整数。负整数从数组中的最后一项开始计数。
at(index)
兼容性问题堪忧
在任意的github项目按下 . 键
会出现不一样的东西
或者把github.com
把com
改成dev
数字输入框 valueAsNumber
<input type="number" id="aaa" onChange="clickMethods()">
const a = document.querySelector("#aaa");
function clickMethods() {
console.log(typeof a.value);// string
console.log(typeof a.valueAsNumber);// number
}
数组互换位置
let a = [1, 2, 3];
[[a[2], a[0]] = [a[0], a[2]];
console.log(a);
// [ 3, 2, 1 ]
按钮的外轮廓
outline:4px solid darkorchid;
outline-offset:4px;
完整的
button{
width: 100px;
height: 50px;
font-size:18px;
color:#fff;
background-color:darkorchid;
border:2px solid darkorchid;
border-radius:20px;
outline:4px solid darkorchid;
outline-offset:4px;
}
console.count 打印的次数
console.log(1)
enum 的实际使用
常量枚举
const enum DownloadStatus {
Idle = "idle",
Loading = 'loading',
Done = 'done'
}
const currentStatus: DownloadStatus = DownloadStatus.Idle;
console.log(currentStatus);
排序
arr.sort((a, b) => {
升序
// return a - b
降序
// return b - a
不变
return 0
});
科学计算法的妙用
1.12e2 // 1.12
112e-2 // 1.12
const round = (n, decimals = 0) => {
let a = Math.round(`${n}e${decimals}`)
return +`${a}e-${decimals}`
};
console.log(round(1.005, 2));
有趣的正则
const str2='AAA111-222-333-444DDD'
str2.replace(/1{3}-2{3}-3{3}-4{3}/,'$`xxx-xxx-xxx')
// 'AAAAAAxxx-xxx-xxxDDD'
$` 好像是把前面的提出来啦
折叠动画
.yl-fb-hide {
transition: max-height .3s;
max-height: 0;
overflow: hidden;
}
.yl-fb-show {
overflow: auto;
max-height: 1000px;
animation: hide-scroll .3s backwards;
}
@keyframes hide-scroll {
from{
max-height: 0;
overflow:hidden;
}
to{
max-height: 1000px;
overflow:hidden;
}
}
字段自动输入的动画
<style>
body {
background: #fff7ee;
font-family: Fira Mono;
height: 100vh;
width: 100vw;
display: flex;
justify-content: center;
align-items: center;
}
h3 {
position: relative;
/*宽度总长度*/
width: 43ch;
font-weight: bold;
/*注意修改43这个字段*/
animation: type 5s steps(43), blink 0.5s step-end infinite alternate;
white-space: nowrap;
overflow: hidden;
border-right: 3px solid;
}
@keyframes type {
0% {
width: 0;
}
}
@keyframes blink {
50% {
border-color: transparent;
}
}
</style>
<h3>All work and no play makes Jack a dull boy.</h3>
进制转化
let a = "10100000100100110110010000010101111011011001101110111111111101000000101111001110001111100001101";
// 十进制
const d = BigInt('0b' + a);
// 二进制
const b = BigInt(a);
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
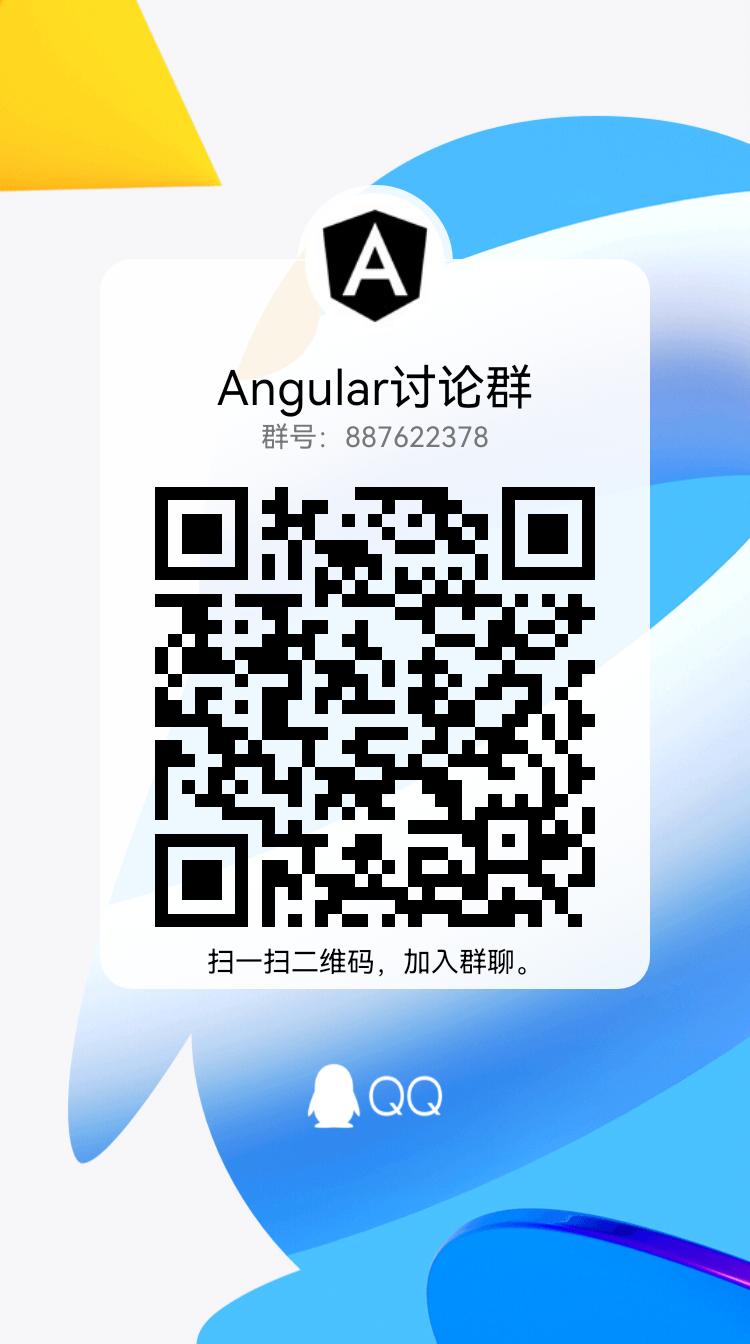