javascript权威指南第七版读书笔记(二)
Fucntion
function add(a) {
return this.name + this.age + a
}
console.log(add.call({name: 'xxx', age: 12}, 'm'))
console.log(add.apply({name: 'xxx', age: 12}, ['m']))
console.log(add.bind({name: 'nnn', age: 23})('m'))
const sum = (x, y) => x + y
console.log(sum.bind(null, 1)(2))
const fn = new Function('x', 'y', 'return x*y')
console.log(fn(1, 2))
模块
1.
export const str = 'abc'
export function log(a) {
return a
}
---
import {str,log} from './test6.js'
console.log(str,log(10))
2.
const str = 'bbbb'
const a = 122
export default {str, a}
---
import Home from './test6.js'
console.log(Home.str)
console.log(Home.a)
3.
import * as stas from './test.js'
sort
let arr=['page10','page9','page1'];
console.log(arr.slice().sort((a, b) => a.localeCompare(b)))
// [ 'page1', 'page10', 'page9' ]
let fuzz=new Intl.Collator(undefined,{numeric:true}).compare;
console.log(arr.sort(fuzz))
// [ 'page1', 'page9', 'page10' ]
URL
let url=new URL("https://www.sss.com?name=xxx&age=12")
console.log(url.href)
// https://www.sss.com/?name=xxx&age=12
console.log(url.origin)
// https://www.sss.com
console.log(url.search)
// ?name=xxx&age=12
跟url的api保持一致
===========
let url=new URL('https://www.bai.com')
console.log(url.search)
// '' 没有参数为空
url.searchParams.append('name','xxxx')
console.log(url.href)
// https://www.bai.com/?name=xxxx
url.searchParams.set('qqq', 'xxxx')
console.log(url.href)
// https://www.bai.com/?name=xxxx&qqq=xxxx
url.searchParams.append('opts','111')
console.log(url.href)
// https://www.bai.com/?name=xxxx&qqq=xxxx&opts=111
console.log(url.searchParams.has('name'))
// true
console.log(url.searchParams.get('name'))
// xxxx
url.searchParams.sort()
console.log(url.href)
// https://www.bai.com/?name=xxxx&opts=111&qqq=xxxx
console.log([...url.searchParams])
// [ [ 'name', 'xxxx' ], [ 'opts', '111' ], [ 'qqq', 'xxxx' ] ]
url.searchParams.delete('name')// 删除
======
let url = new URL('https://www.baidu.com');
let params=new URLSearchParams();
params.append('q', 'then')
params.append('opts', 'exact');
console.log(params.toString())
// q=then&opts=exact
url.search=params;
console.log(url.href)
// https://www.baidu.com/?q=then&opts=exact
Iterators
let a = [1, 2, 3]
let b=a[Symbol.iterator]();
console.log(b.next())
//{ value: 1, done: false }
console.log(b.next())
// { value: 2, done: false }
console.log(b.next())
// { value: 3, done: false }
for(let item of b){
console.log(item.value)//1,2,3
}
=====
function *one(){
yield 2;
yield 3;
yield 5;
}
let a=one();
console.log(a.next().value)
console.log(a.next().value)
console.log(a.next().value)
----------------
function* add() {
yield 1
return 2
yield 3
yield 4
}
let a=add();
console.log(a.next())
// { value: 1, done: false }
console.log(a.next())
// { value: 2, done: true }
// 停止啦
let c=[...add()]
console.log(c)
// [1]
======
let obj = {
get [Symbol.toStringTag]() {
return 'Range'
}
}
const typeObj = x => {
return Object.prototype.toString.call(x)
}
console.log(typeObj(obj))
// [object Range]
console.log(typeObj([]))
// [object Array]
========
class EzArray extends Array {
get first() {
return this[0]
}
get last() {
return this[this.length - 1]
}
}
let e = new EzArray(1, 2, 3)
let f = e.map(x => x ** 2)
console.log(e.last) // 3
console.log(f.last) // 9
=========
let data = [10, 22]
let methods={
square:x=>x*x,
}
console.log(methods.square(10))
// 100
DOM操作
<p name="age">12211221</p>
console.log(document.querySelector("*[name='age']"))
console.log(document.querySelector("p[name='age']"))
=====
<p id="aaa">12211221</p>
<script>
let a = document.querySelector('#aaa')
let c = document.createElement('h1')
c.textContent = '前面';
a.before(c)
===
let d = document.createElement('h1')
d.textContent = '前面';
a.prepend(d) //插入前面
a.after(`<h1>后面</h1>`)
a.replaceWith(`<h1>我是替换后</h1>`)// 把#aaa替换了
a.remove();// 删除当前节点
</script>
className
textContent
classList.add('xx','xxx') //插入两个css
classList.remove('xxx')//删除一个xxx的css
Scroll
// 文档的高度
console.log(document.documentElement.offsetHeight)//可以直接滚动到最低位置
// 视口的高度
console.log(window.innerHeight)
// 滚动到指定坐标
scrollTo() //x轴,y轴
//偏移量滚动文档
setInterval(()=>{
scrollBy(0,50)// 会在当前基础上,慢慢向下50px慢慢滚动
},1000)
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
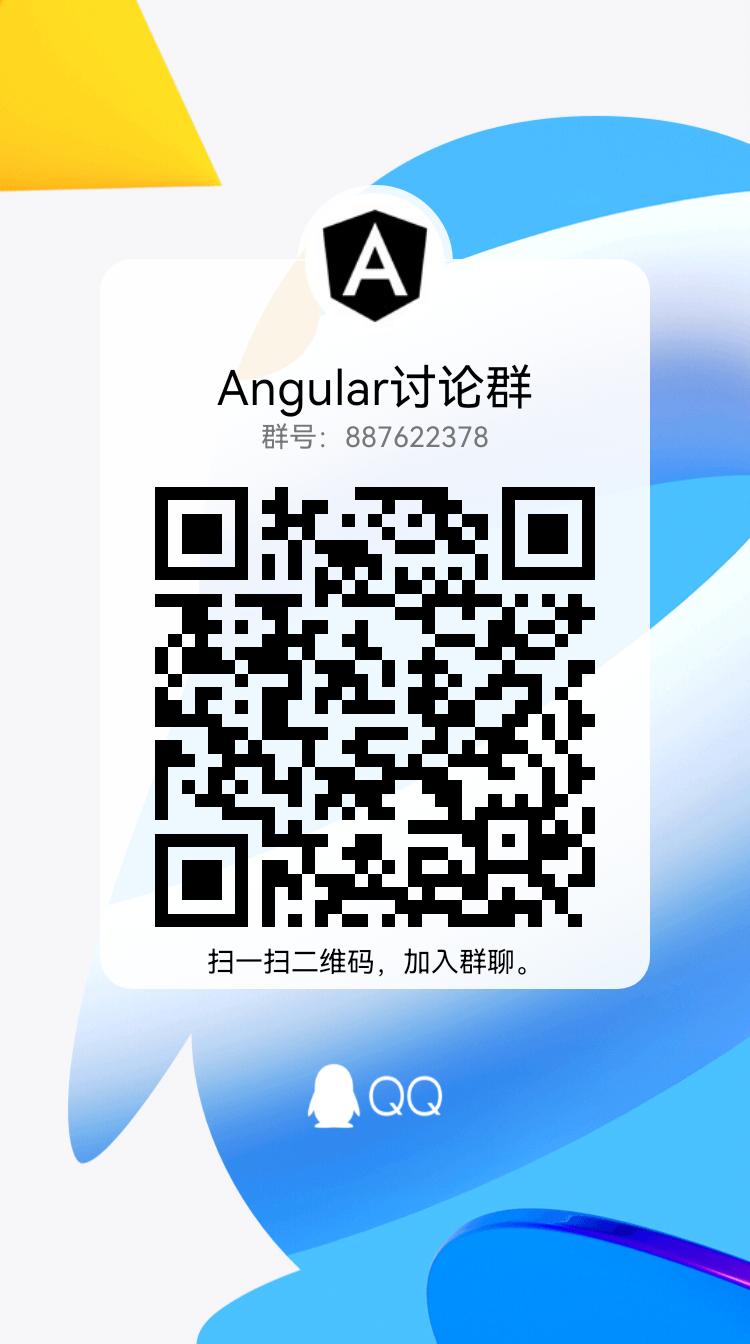