本周学习总结
include和indexOf底层实现
const toInteger = (index) => isNaN(index = +index) ? 0 :
(index > 0 ? Math.floor : Math.ceil)(index);
const absolute = (index, length) => {
let integer = toInteger(index);
return integer < 0 ? Math.max(integer + length, 0) : Math.min(integer, length);
};
let obj = {
includes: createMethod(true),
indexOf: createMethod(false)
};
function createMethod(booleans) {
return (array, el, index) => {
index = absolute(index, array.length);
let value, length = array.length;
if (booleans && el != el) { //这个是用来计算includes的NaN
while (index < length) {
value = array[index++];
if (value != value) return true;
}
} else {
for (; index < length; index++) {
if ((booleans || index in array) && array[index] === el) {
// ||0 的意思是当index为0的时候回执行后面
return booleans || index || 0;
}
}
return !booleans && -1;
}
}
}
在 JavaScript 中, (a 1 && a 2 && a==3) 是否有可能为 true?
- 创建一个带有自定义
toString
(或者valueOf
) 函数的对象let a = { i: 1, toString: function () { return a.i++ } }; console.log(a == 1 && a == 2 && a == 3);//true
- unicode空格字符,但是ECMAScript不将其解释为一个空格,因为是三个完全不同的变量,一个a,一个前空格+a,一个a+后空格
var aᅠ = 1; var a = 2; var ᅠa = 3; console.log(aᅠ == 1 && a == 2 && ᅠa == 3);//true 写成易读的就是 var a_ = 1; var a = 2; var _a = 3; console.log(a_==1 && a== 2 &&_a==3)//true
- Object.defineProperty 修改对象的属性,劫持 JS 对象的
getter
,不过这种方式对于严格相等===
同样有效;let b = 0; Object.defineProperty(window, 'a', { get: function () { return ++b; } }); console.log(a == 1 && a == 2 && a == 3);
求小于1000的含1的正整数的个数
let i=0;
let res=0;
while (++i < 1000) {
String(i).includes('1')?res++:res;
}
console.log(res);
lastIndexOf 底层实现
const toInteger = (index) => isNaN(index = +index) ? 0 :
(index > 0 ? Math.floor : Math.ceil)(index);
const absolute = (index, length) => {
let integer = toInteger(index);
return integer < 0 ? Math.max(integer + length, 0) : Math.min(integer, length);
};
const lastIndexOf = (array, target, index) => {
let length = array.length;
// 如果第二个参数存在
if (index != null) index = absolute(index, length);
for (; index >= 0; index--) {
if (index in array && array[index] === target) {
return index ||0;
}
}
return -1
};
try...catch程序的输出结果
(function () {
try {
throw new Error();
} catch (x) {
var x = 1, y = 2;
console.log(x);
}
console.log(x);
console.log(y);
})();
输出结果:
1
undefined
2
函数的名字是不可变的
function add() {}
let oldName = add.name;
add.name='bar';
console.log([oldName, add.name]); // [ 'add', 'add' ]
reduce+reduceRight源码实现
const createMethod = type => {
return (array, fn, memo) => {
let length = array.length;
let index = type ? length - 1 : 0;
let i = type ? -1 : 1;
if (memo == null) {
while (true) {
// console.log([1,2].reduce(acc => acc)); //1
//为了确定初始化的第一个值
if (index in array) {
memo = array[index];
index += i;
break
}
index += i;
if (type ? index < 0 : length <= index) {
// 没有初始值的问题
// console.log([].reduce(acc => acc)); 报下面的错
throw TypeError('Reduce of empty array with no initial value')
}
}
}
for (; type ? index >= 0 : length > index; index += i) {
if (index in array) {
memo = fn(memo, array[index], index, array)
}
}
return memo
}
};
const obj = {
left: createMethod(false),
right: createMethod(true)
};
let reduces = obj.left;
console.log(reduces([1, 2, 3, 4, 5], (acc, val) => acc+val,''));
CSS github动画查询
css-animations
自己喜欢的是什么,真正想要的是什么,最适合自己的是什么,并且思考这些东西在现实生活中会不会存在
Array.of
function of(){
let length=arguments.length;
var A = new Array(length);
while(length--) A[length] = arguments[length];
return A
}
console.log(of(1, 2, 3, 4));
// [ 1, 2, 3, 4 ]
Object.keys
const has = (it, key) => ({}).hasOwnProperty.call(it, key);
const keys = (obj) => {
let arr = [
'constructor',
'hasOwnProperty',
'isPrototypeOf',
'propertyIsEnumerable',
'toLocaleString',
'toString',
'valueOf'
];
let i=0;
let result=[];
for (let key in obj)
!has(arr, key) && has(obj, key) && result.push(key);
// 不能被枚举的隐藏的属性
while(arr.length>i) if (has(obj, key = arr[i++])) {
~result.indexOf(key) || result.push(key);
}
return result
};
toString
const isType = target => ({}).toString.call(target).slice(8, -1);
console.log(isType({}));// Object
判断是否是arguments
const isArguments = type =>typeof type.callee === 'function';
console.log(isArguments(arguments));// true
Array.from
const isFunction = type => ({}).toString.call(type) === '[object Function]';
const from = (items, mapFn) => {
if (items == null) {
throw new TypeError('报错了')
}
if (typeof mapFn !== "undefined") {
if (!isFunction(mapFn)) {
throw new TypeError('mapFn 只能是函数')
}
}
let {length} = items;
let array = new Array(length);
let i = -1;
while (++i < length) {
array[i] =mapFn? mapFn(items[i], i, array):items[i];
}
return array
};
Object.is
const is = (a, b) => a === b ? a !== 0 || 1 / a === 1 / b : a != a && b != b;
console.log(is(NaN, NaN));//true
console.log(is(0, -0));// false
parseInt(string,radix)
将一个字符串string转换为radix 进制的整数,radix为介于
2-36
之间的数radix为0 返回本身,如果不是就返回NaN
console.log(parseInt('12.122', 0));//12 console.log(parseInt('12.122'));//12 console.log(parseInt('12ssss'));//12 console.log(parseInt('ssss'));//NaN
["1", "2", "3"].map(parseInt)进一步的理解
parseInt(val,radix)
(val,index)=>parseInt(val,index)
['1', '2', '3'].map((val, index) => parseInt(val, index))
parseInt('1',0) 1
parseInt('2',1) NaN
parseInt('3',2) NaN 二进制不会有3
优先级问题
** 优先级比 * 高
console.log(4*3*2) // 4*9 等于36
3 > 2 && 2 > 1
//true
let a = 1 + 2 && 3;
console.log(a);//3
let b = 1 || 1 ? 2 : 3;
console.log(b);//2
一段有趣的代码
var ary = [0,1,2];
ary[10] = 10;
console.log(ary.map(val => val * 10));
// [ 0, 10, 20, <7 empty items>, 100 ]
console.log(ary.filter(val=>val==='undefined'));
// []
不对空数组进行检测,直接跳过
switch 判断的是全等(===)
filter(滤镜)
https://www.bestagencies.com/tools/filter-effects-css-generator/
模糊
filter: blur(9px);
灰度
filter:grayscale(1)
亮度
filter: brightness(2.3);
对比度
filter: contrast(4.4);
饱和度
filter: saturate(3.6);
色相旋转
filter: hue-rotate(185deg);
反光
filter: invert(1);
阴影
filter: drop-shadow(0px 0px 5px #000);
透明度
filter: opacity(55%);
褐色
filter: sepia(0.77);
鼠标手势
渐变
判断对象
const isObject = val => typeof val === 'object' ? val !== null : typeof val === 'function';
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
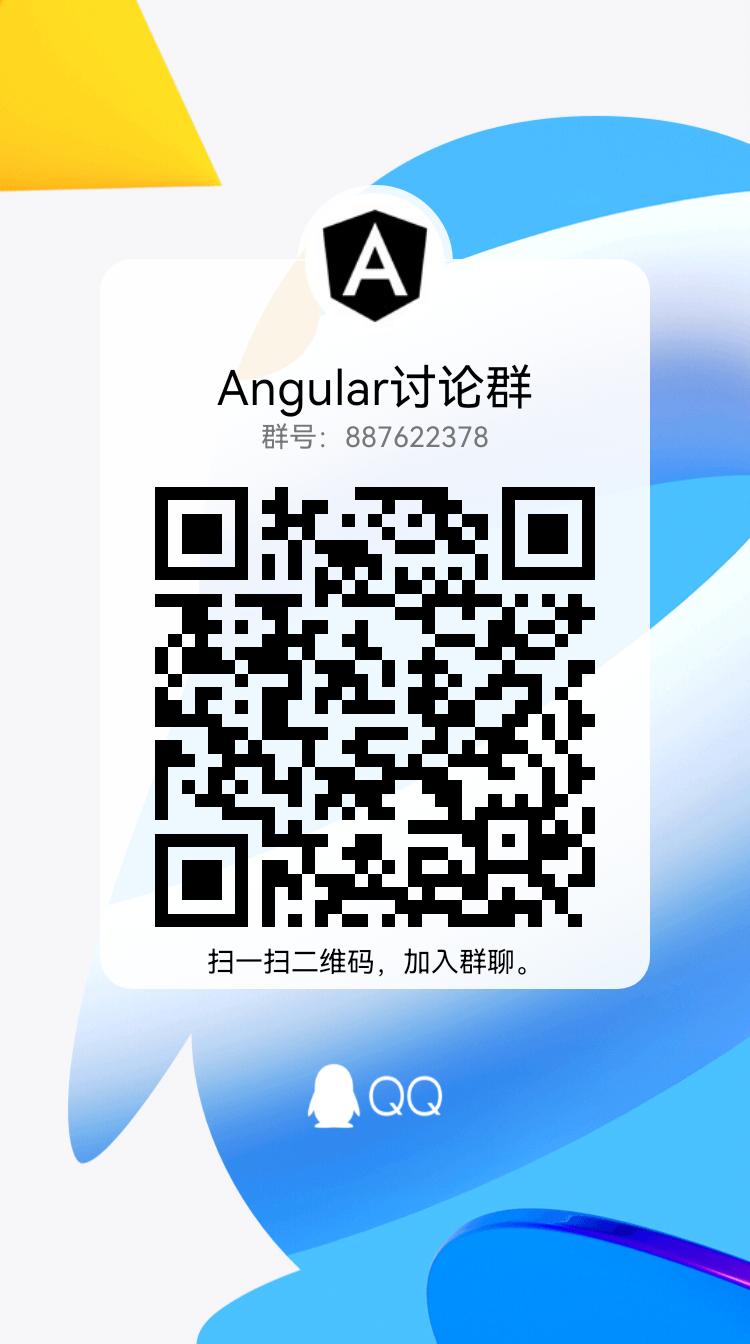