本两周学习总结
这两周主要再看源码,所以没什么好写的,但是绝大大多源码或者记录一些我觉得有意思的代码,
主要记录的有
D3源码
,date-fns
,rx.js主要是需要后期看能不能看看源码
,numjs
,然后修改成两周更新一次,其他这样的更新其实也是保持新鲜感
this指向问题
function sumArgs() {
return Array.prototype.reduce.call(arguments, (acc, val) => {
return acc + val
}, 0)
}
console.log(sumArgs(1, 2, 3))
263 丑数
编写一个程序判断给定的数是否为丑数。
丑数就是只包含质因数 2, 3, 5 的正整数。思考
所以判断一个数是否为丑数只要将这个数不断与2,3,5相除,只要除的尽,并且最终结果为1,即为丑数。
const isUgly = num => { if (num < 1) { return false } while (num % 2 == 0) { num /= 2; } while (num % 3 == 0) { num /= 3; } while (num % 5 == 0) { num /= 5; } return num == 1; }
removeEventListener的用法
document.addEventListener('mousemove',removeMouse)
document.removeEventListener('mousemove',removeMouse);
function removeMouse(e){}
str.js
https://github.com/panzerdp/voca/blob/master/dist/voca.js#L1939
语音发声
const speechSynthesis = message => {
const msg = new SpeechSynthesisUtterance(message);
msg.voice = window.speechSynthesis.getVoices()[0];
window.speechSynthesis.speak(msg);
};
speechSynthesis('我是谁,你能听到我说话吗')
需要点击触发,浏览器不支持自动触发
30s
https://github.com/30-seconds/30-seconds-of-code/blob/master/dist/_30s.js#L14202
arr-union
const unions = (first, ...arr) => {
if (!Array.isArray(first)) {
throw new TypeError('first argument 应该是数组')
}
let i = 0
let len = arr.length
while (i < len) {
let item = arr[i]
if (!item) {
continue
}
if (!Array.isArray(item)) {
item = [item]
}
for (let j = 0; j < item.length; j++) {
if (first.includes(item[j])) {
continue
}
first.push(item[j])
}
i++
}
return first
}
console.log(unions([], 1, 2, 3, 4, 5, [1, 2, 3]))
// [ 1, 2, 3, 4, 5 ]
isInteger
const _isInteger=n=>Number.isInteger(n)|| n<<0 === n
async.js
https://github.com/caolan/async/blob/master/dist/async.js
node 相关,什么时候把node看得看不多就看看
有趣的代码
const add = (a, b) => {
return +a + +b;
}
Node.js
unlink 删除文件
unlinkSync 同步删除 应该放在try catch里面
rename 重命名
stat fstat 文件属性
两个的方法功能是一样的都是获取文件的状态信息
fs.open('./test46.js','r',(err,fd)=>{ if(err) throw err; fs.fstat(fd,(err,status)=>{ if(err) throw err; //文件描述 console.log(status) /*关闭文件空间*/ fs.close(fd,(err)=>{ if(err)throw err; }) }) }) fs.stat('./test46.js',(err,res)=>{ if(err) throw err; console.log(res) }) fs.stat 接受的第一个参数是一个文件路径字符串 fs.fstat 接收的是一个文件描述符
API
https://juejin.im/post/5d6e5a2851882554841c3f3b
# Buffer类 原生没有,node引入Buffer类 Buffer.alloc(size[,fill[,encoding]]) //长度 值 编码方式 (默认utf8),长度必传 例如 //创建长度为5,填充12,默认utf8 console.log(Buffer.alloc(5,12)) <Buffer 0c 0c 0c 0c 0c> # Buffer.from(array) //接受一个*整数数组*,返回一个buffer对象 console.log(Buffer.from([1, 2, 3,13])) <Buffer 01 02 03 0d> //接受一个字符串 let a=Buffer.from('Hello World!') // <Buffer 48 65 6c 6c 6f 20 57 6f 72 6c 64 21> console.log(a.toString()) //Hello World! # Buffer.isEncoding(encoding) 判断是否支持某种编码 Buffer.isEncoding('utf8') //true # Buffer.isBuffer(obj) 判断是否为Buffer对象 console.log(Buffer.isBuffer(Buffer.alloc(3))) // 输出true # Buffer.byteLength(string) console.log(Buffer.byteLength('我是ss')) //3+3+2 # 合并传入的buffer数组 let a=Buffer.alloc(1,2) let b=Buffer.alloc(2,3) console.log(Buffer.concat([a, b])) //<Buffer 02 03 03> # buf.write const buf = Buffer.alloc(10); //写入string,索引位置,长度 buf.write('heelo', 1, 4) console.log(buf) // <Buffer 00 68 65 65 6c 00 00 00 00 00> console.log(buf.toString()) // heel #buf.slice([start[,end]]) //包左不包右 const buf1 = Buffer.from('Hello world'); const buf2=buf1.slice(0,4); console.log(buf2.toString()); // Hell # buf.toString([encoding[,start[,end]]]) 将二进制数据流转换成字符串,encoding是编码方式,start与end效果同slice const buf1 = Buffer.from('Hello world'); console.log(buf1.toString('utf8', 0,4 )) //Hell
path 操作路径对象
path.basename(path[,ext]) //获取文件名及文件后缀,ext是文件后缀 const path = require('path'); console.log(path.basename('/a/b/c/d/index.html')) //index.html console.log(path.basename('/a/b/c/d/index.html', '.html'))//index //获取不带文件名的文件路径 console.log(path.dirname('/a/b/c/d/index.html'))// /a/b/c/d //获取文件名后缀 console.log(path.extname('/a/b/c/index.html')) // .html //将路径字符串转换为路径对象 const pathObj = path.parse('E:\\a\\b\\c\\d\\index.html') console.log(pathObj) /* 输出 * { * root: 'E:\\', // 文件根目录 * dir: 'E:\\a\\b\\c\\d', // 不带文件名的文件路径 * base: 'index.html', // 文件名 * ext: '.html', // 文件后缀 * name: 'index' // 不带后缀的文件名 * } */ // 将路径对象转换为路径字符串 const pathObj = { root: 'E:\\', dir: 'E:\\a\\b\\c\\d', //必传 base: 'index.html',//必传 ext: '.html', name: 'index' } console.log(path.format(pathObj)) // 输出 E:\a\b\c\d\index.html // 注意:路径对象的每个属性不是必须的,提供了dir属性时会忽略root属性,提供了base属性时会忽略ext与name属性 //判断路径是否是绝对路径 console.log(path.isAbsolute('E:/a/b/c/d\index.html')) //true console.log(path.isAbsolute('./a/b/c/d\index.html')) //false
报错信息
TypeError 允许一个TypeError类型错误
URIError URL错误
ReferenceError 一个不存在的变量
RangeError 当一个值不在其所允许的范围或者集合中。
数据结构
https://github.com/mgechev/javascript-algorithms
算法
https://github.com/jeantimex/javascript-problems-and-solutions
优化滚动条样式
*::-webkit-scrollbar {
/*滚动条整体样式*/
width: 8px;/*定义纵向滚动条宽度*/
height: 8px;/*定义横向滚动条高度*/
}
*::-webkit-scrollbar-thumb {
/*滚动条内部滑块*/
border-radius: 8px;
background-color: hsla(220, 4%, 58%, 0.3);
transition: background-color 0.3s;
}
*::-webkit-scrollbar-thumb:hover {
/*鼠标悬停滚动条内部滑块*/
background: #bbb;
}
*::-webkit-scrollbar-track {
/*滚动条内部轨道*/
background: #ededed;
}
改成less模式
* {
&::-webkit-scrollbar {
width: 8px;
height: 8px;
}
&::-webkit-scrollbar-thumb {
border-radius: 8px;
background-color: hsla(220, 4%, 58%, 0.3);
transition: background-color 0.3s;
&:hover {
background: #bbb;
}
}
&::-webkit-scrollbar-track {
background: #ededed;
}
}
禁止选中
.aaa{
user-select:none;
}
删选数组
var origin = [ 1 , 2 , 'a' , 3 , 1 , 'b' , 'a' ];
console.log(origin.filter((val, index) => origin.indexOf(val) === index))
//[ 1, 2, 'a', 3, 'b' ]
随机值
let a=(1 + Math.random()).toString(36);
console.log(a) // 1.cn52taii2g
有意思的代码
let slice = Function.call.bind(Array.prototype.slice);
let concat = Function.call.bind(Array.prototype.concat);
let forEach = Function.call.bind(Array.prototype.forEach);
let map = Function.call.bind(Array.prototype.map);
console.log(map([1, 2, 3, 4, 5], (val, index) => [val * 2, index]))
// [ [ 2, 0 ], [ 4, 1 ], [ 6, 2 ], [ 8, 3 ], [ 10, 4 ] ]
时间转化
var s = 1000;
var m = s * 60;
var h = m * 60;
var d = h * 24;
var w = d * 7;
var y = d * 365.25;
function fmtShort(ms) {
var msAbs = Math.abs(ms);
if (msAbs >= d) {
return Math.round(ms / d) + 'd';
}
if (msAbs >= h) {
return Math.round(ms / h) + 'h';
}
if (msAbs >= m) {
return Math.round(ms / m) + 'm';
}
if (msAbs >= s) {
return Math.round(ms / s) + 's';
}
return ms + 'ms';
}
你们知道为啥在源码看总是有xxx!=null
undefined==null //true
null==null //true
//把其他类型转成数字类型
const number = (x) => x === null ? NaN : +x;
复制剪贴板
https://clipboardjs.com/
物理引擎
https://github.com/schteppe/p2.js
获取当前时间
let str=new Date(+new Date() + 8 * 3600 * 1000).toISOString().split(/[T.]/g).slice(0,2)
let newDate=`${str[0]} ${str[1]}`
console.log(newDate)
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
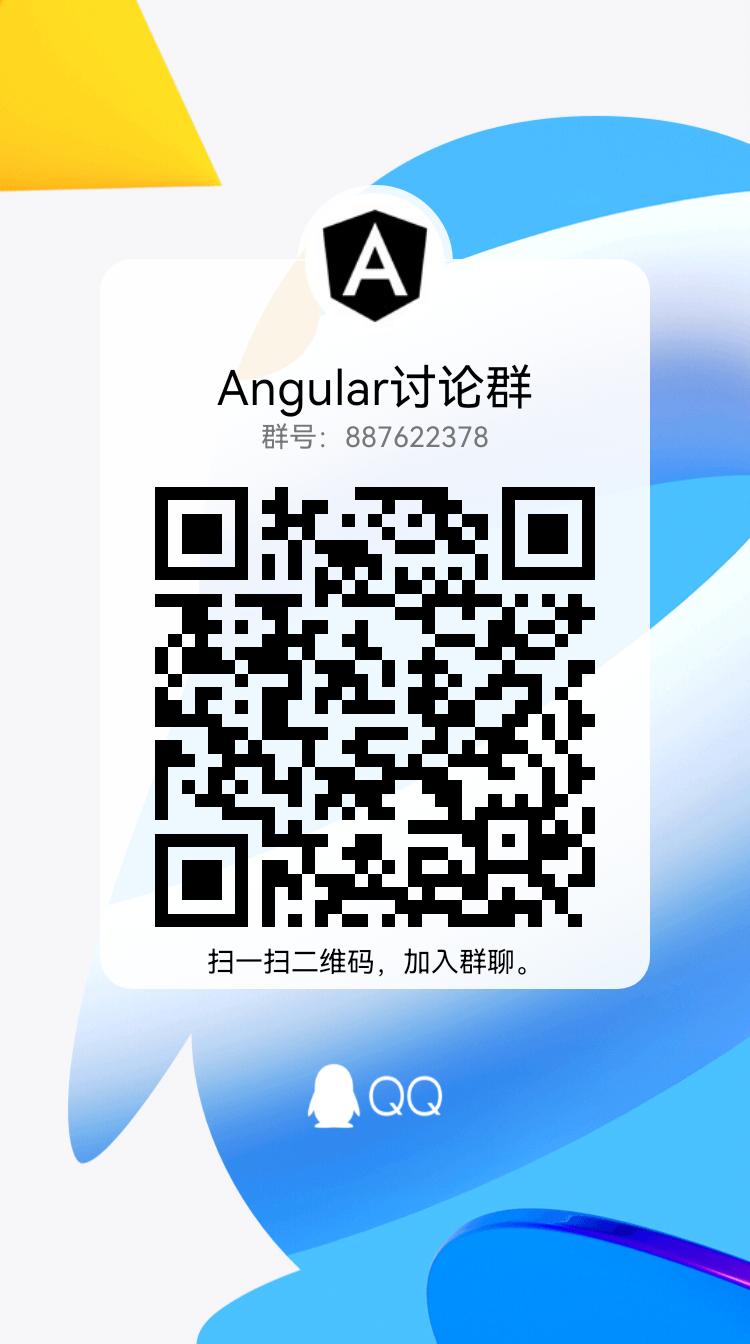