《最新出炉》系列初窥篇-Python+Playwright自动化测试-20-处理鼠标拖拽-下篇
1.简介
上一篇中,宏哥说的宏哥在最后提到网站的反爬虫机制,那么宏哥在自己本地做一个网页,没有那个反爬虫的机制,谷歌浏览器是不是就可以验证成功了,宏哥就想验证一下自己想法,其次有人私信宏哥说是有那种类似拼图的验证码如何处理。于是写了这一篇文章,另外也是相对前边做一个简单的总结分享给小伙伴们或者童鞋们。废话不多数,直接进入今天的主题。
2.滑动验证码
2.1演示模拟验证码点击拖动场景
例如:演示模拟验证码点击拖动场景示例如下:
3.代码准备
3.1前端HTML代码
前端HTML代码如下:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>北京-宏哥 滑动条</title> <link rel="stylesheet" href="drag.css"> <script src="jquery-1.7.1.min.js"></script> <script src="drag.js"></script> <style type="text/css"> .slidetounlock{ font-size: 12px; background:-webkit-gradient(linear,left top,right top,color-stop(0,#4d4d4d),color-stop(.4,#4d4d4d),color-stop(.5,#fff),color-stop(.6,#4d4d4d),color-stop(1,#4d4d4d)); -webkit-background-clip:text; -webkit-text-fill-color:transparent; -webkit-animation:slidetounlock 3s infinite; -webkit-text-size-adjust:none } @-webkit-keyframes slidetounlock{0%{background-position:-200px 0} 100%{background-position:200px 0}} .button1 { background-color: #f44336; border: none; color: white; padding: 15px 32px; text-align: center; text-decoration: none; display: inline-block; font-size: 28px; margin-bottom: 100px; text-decoration:none; color: white; } #myAnchor { text-decoration:none; color: white; } </style> </head> <body> <div id="wrapper" style="position: relative;top: 300px;left:300px;"> <button class="button1"><a id="myAnchor" href="https://www.cnblogs.com/du-hong/">北京-宏哥</a></button></br> <div id="drag"> <div class="drag_bg"></div> <div class="drag_text slidetounlock" onselectstart="return false;" unselectable="on"> 请按住滑块,拖动到最右边 </div> <div class="handler handler_bg"></div> </div> </div> <!--<a href="#" class="img"><img src="img/Lighthouse.jpg"/></a>--> <script> $('#drag').drag(); </script> </body> </html>
3.2滑块CSS样式
HTML滑块CSS样式代码如下:
#drag{ position: relative; background-color: #e8e8e8; width: 300px; height: 34px; line-height: 34px; text-align: center; } #drag .handler{ position: absolute; top: 0px; left: 0px; width: 40px; height: 32px; border: 1px solid #ccc; cursor: move; } .handler_bg{ background: #fff url("../img/slider.png") no-repeat center; } .handler_ok_bg{ background: #fff url("../img/complet.png") no-repeat center; } #drag .drag_bg{ background-color: #7ac23c; height: 34px; width: 0px; } #drag .drag_text{ position: absolute; top: 0px; width: 300px; color:#9c9c9c; -moz-user-select: none; -webkit-user-select: none; user-select: none; -o-user-select:none; -ms-user-select:none; font-size: 12px; // add }
3.3滑块拖拽JS
滑块拖拽JS代码如下:
$.fn.drag = function(options) { var x, drag = this, isMove = false, defaults = { }; var options = $.extend(defaults, options); var handler = drag.find('.handler'); var drag_bg = drag.find('.drag_bg'); var text = drag.find('.drag_text'); var maxWidth = drag.width() - handler.width(); //能滑动的最大间距 //鼠标按下时候的x轴的位置 handler.mousedown(function(e) { isMove = true; x = e.pageX - parseInt(handler.css('left'), 10); }); //鼠标指针在上下文移动时,移动距离大于0小于最大间距,滑块x轴位置等于鼠标移动距离 $(document).mousemove(function(e) { var _x = e.pageX - x;// _x = e.pageX - (e.pageX - parseInt(handler.css('left'), 10)) = x if (isMove) { if (_x > 0 && _x <= maxWidth) { handler.css({'left': _x}); drag_bg.css({'width': _x}); } else if (_x > maxWidth) { //鼠标指针移动距离达到最大时清空事件 dragOk(); } } }).mouseup(function(e) { isMove = false; var _x = e.pageX - x; if (_x < maxWidth) { //鼠标松开时,如果没有达到最大距离位置,滑块就返回初始位置 handler.css({'left': 0}); drag_bg.css({'width': 0}); } }); //清空事件 function dragOk() { handler.removeClass('handler_bg').addClass('handler_ok_bg'); text.removeClass('slidetounlock').text('验证通过').css({'color':'#fff'}); //modify // drag.css({'color': '#fff !important'}); handler.css({'left': maxWidth}); // add drag_bg.css({'width': maxWidth}); // add handler.unbind('mousedown'); $(document).unbind('mousemove'); $(document).unbind('mouseup'); } };
3.4jquery-1.7.1.min.js下载地址
jquery-1.7.1.min.js下载链接:http://www.jqueryfuns.com/resource/2169
4.自动化代码实现
4.1代码设计
4.2参考代码
# coding=utf-8🔥 # 1.先设置编码,utf-8可支持中英文,如上,一般放在第一行 # 2.注释:包括记录创建时间,创建人,项目名称。 ''' Created on 2023-07-22 @author: 北京-宏哥 QQ交流群:705269076 公众号:北京宏哥 Project: 《最新出炉》系列初窥篇-Python+Playwright自动化测试-19-处理鼠标拖拽-下篇 ''' # 3.导入模块 from playwright.sync_api import Playwright, sync_playwright, expect def run(playwright: Playwright) -> None: browser = playwright.chromium.launch(headless=False) context = browser.new_context() page = context.new_page() page.goto("C:/Users/DELL/Desktop/test/MouseDrag/identifying_code.html") page.wait_for_timeout(2000) #获取拖动按钮位置并拖动 //*[@id="slider"]/div[1]/div[2] dropbutton=page.locator("//*[@id='drag']/div[3]") box=dropbutton.bounding_box() page.mouse.move(box['x']+box['width']/2,box['y']+box[ 'height']/2) page.mouse.down() mov_x=box['x']+box['width']/2+390 page.mouse.move(mov_x,box['y']+box[ 'height']/2) page.mouse.up() page.wait_for_timeout(3000) # page.pause() context.close() browser.close() with sync_playwright() as playwright: run(playwright)
4.3运行代码
1.运行代码,右键Run'Test',控制台输出,如下图所示:
2.运行代码后电脑端的浏览器的动作。如下图所示:
5.小结
好了,今天时间也不早了,宏哥就讲解和分享到这里,感谢您耐心的阅读,希望对您有所帮助。
欢迎各位体验腾讯云的Edge:https://cloud.tencent.com/developer/article/2346776
感谢您花时间阅读此篇文章,如果您觉得这篇文章你学到了东西也是为了犒劳下博主的码字不易不妨打赏一下吧,让博主能喝上一杯咖啡,在此谢过了!
如果您觉得阅读本文对您有帮助,请点一下左下角“推荐”按钮,您的
本文版权归作者和博客园共有,来源网址:https://www.cnblogs.com/du-hong 欢迎各位转载,但是未经作者本人同意,转载文章之后必须在文章页面明显位置给出作者和原文连接,否则保留追究法律责任的权利!
公众号(关注宏哥)                                                                                 客服微信
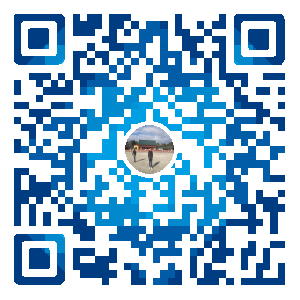
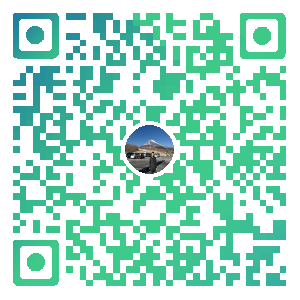