Cocos2d-x 学习笔记(6) Sprite SpriteFrameCache Texture2D TextureCache
1. 概述
TextureCache是对Texture2D纹理的缓存,对图片文件的缓存。SpriteFrameCache是对SpriteFrame的缓存,是对纹理大图的矩形块的缓存,每个SpriteFrame是对Texture2D的封装,每个精灵帧都在同一个纹理大图中。
Sprite是对Texture2D和SpriteFrame的封装,可以实现一张图片在屏幕上的显示和变换。
2. Sprite
2.1 概述
Sprite是2d图像,是在屏幕上可变化的对象,受到控制。
通过对Sprite的操作,实现了一张图片在游戏屏幕上的各种变化。
Sprite继承自父类Node,属性包括位置、旋转、缩放、斜放、大小等。
2.2 创建方法
直接创建精灵,可选图片文件、矩形大小、多边形:
static Sprite* create(); static Sprite* create(const std::string& filename); static Sprite* create(const PolygonInfo& info); static Sprite* create(const std::string& filename, const Rect& rect);
从精灵帧创建精灵:
static Sprite* createWithSpriteFrame(SpriteFrame *spriteFrame); static Sprite* createWithSpriteFrameName(const std::string& spriteFrameName);
从Texture创建精灵:
TextureCache::getInstance()->addImage("pic.png"); auto texture=TextureCache::getInstance()->getTextureForKey("pic.png"); auto sprite = Sprite::createWithTexture(texture,Rect(0,0,150,200),true);
3. SpriteFrame SpriteFrameCache
3.1 概念
一张大图包含很多小图,一个精灵帧SpriteFrame对应一张小图,小图在大图中的位置大小等,在精灵帧中定义好。
很多的精灵帧封装成一个plist文件,和一张对应的大图。
精灵帧缓存SpriteFrameCache是对很多精灵帧的缓存,通过plist文件和大图获取每个帧的内容,进行缓存。
SpriteFrameCache类有Map类型成员_spriteFrames,key为帧名,value为精灵帧:
Map<std::string, SpriteFrame*> _spriteFrames;
plist文件对应了一张包含多个精灵图像的大图,plist文件包括了每个精灵在大图中的位置大小等信息。
plist内部包括:
- frames:精灵帧的字典,key是sprite name,value是精灵帧;
- 每一个精灵帧的数据,定位了一个精灵帧:offset、sourceize、rotated等;
- metadata:精灵图集的其他信息,包括纹理图像文件名、大小等。
3.2 方法
把精灵帧通过plist文件添加到精灵帧缓存中:
auto spriteFrameCache = SpriteFrameCache::getInstance(); spriteFrameCache->addSpriteFramesWithFile("sprites.plist");
addSpriteFramesWithFile(...) 方法的实现大致过程:
从plist添加精灵帧到精灵帧缓存最终调用的方法:
addSpriteFramesWithFile(const std::string& plist, Texture2D *texture)
从精灵帧缓存获取精灵帧:
getSpriteFrameByName(const std::string& name)
用帧创建sprite:
auto sprite2 = Sprite::createWithSpriteFrame(spriteFrame);
删除精灵帧:
通过遍历,从_spriteFrames中用指定的key删除精灵帧。
removeSpriteFrames()
removeUnusedSpriteFrames()
removeSpriteFramesFromTexture(Texture2D* texture)
removeSpriteFramesFromDictionary(ValueMap& dictionary)
4. TextureCache
4.1 概念
纹理缓存TextureCache是最基本的图片缓存,将纹理缓存在内存中,SpriteFrameCache以它为基础。
纹理缓存类存储Texture2D有Map类型成员_textures,key为纹理文件名,value为纹理Texture2D:
std::unordered_map<std::string, Texture2D*> _textures;
Texture2D类可从图像等数据创建OpenGL二维纹理。
4.2 纹理加入到纹理缓存
回顾Sprite从图片路径创建过程:
- create(...)方法:
Sprite* Sprite::create(const std::string& filename, const Rect& rect) { Sprite *sprite = new (std::nothrow) Sprite(); if (sprite && sprite->initWithFile(filename, rect)) // ...... }
- initWithFile(...) 方法:
bool Sprite::initWithFile(const std::string &filename, const Rect& rect) { // ...... Texture2D *texture = _director->getTextureCache()->addImage(filename); if (texture) { return initWithTexture(texture, rect); } // ...... }
从Director单例中获取TextureCache对象,添加图片文件,获得texture指针。
- addImage(...) 方法
如果文件路径名对应的纹理之前没有加载,则新建Texture2D对象并返回指针,否则返回先前加载的指针。 - initWithTexture(...) 方法:对Sprite对象初始化。
纹理加入到纹理缓存:
addImage(const std::string &path) addImageAsync(const std::string &path, const std::function<void(Texture2D*)>& callback, const std::string& callbackKey)
4.3 获取纹理
通过key在_textures中搜索对应的纹理。
Texture2D* TextureCache::getTextureForKey(const std::string &textureKeyName) const;
4.4 清理纹理
清理没有被使用的纹理:
_textures中所有其引用计数为1的对象执行release()方法。
void TextureCache::removeUnusedTextures();
通过指定key清理指定纹理:
void TextureCache::removeTextureForKey(const std::string &textureKeyName)
清理所有纹理缓存
_textures中所有texture对象执行release(),再对_textures执行clear()。
void TextureCache::removeAllTextures();
5. Texture2D
5.1 概念
Texture2D是一张二维图片,一个个像素拼接而成,存储了每个像素的信息。
5.2 成员属性
像素格式 enum PixelFormat
PixelFormat _pixelFormat
像素格式信息 struct PixelFormatInfo
map<PixelFormat, const PixelFormatInfo> PixelFormatInfoMap
struct _TexParams
/** pixel format of the texture */
Texture2D::PixelFormat _pixelFormat;
/** width in pixels */
int _pixelsWide;
/** height in pixels */
int _pixelsHigh;
/** texture name */
GLuint _name;
/** texture max S */
GLfloat _maxS;
/** texture max T */
GLfloat _maxT;
/** content size */
Size _contentSize;
/** whether or not the texture has their Alpha premultiplied */
bool _hasPremultipliedAlpha;
/** whether or not the texture has mip maps*/
bool _hasMipmaps;
/** shader program used by drawAtPoint and drawInRect */
GLProgram* _shaderProgram;\
5.3 应用
从精灵得到纹理:
auto sp = Sprite::create("pic.png");
auto texture=sp->getTexture();
auto sprite = Sprite::createWithTexture(texture);
用纹理创建精灵:
auto sprite = Sprite::createWithTexture(texture);
从精灵帧缓冲中得到纹理:
SpriteFrameCache::getInstance()->addSpriteFramesWithFile("sps.plist");
auto frame = SpriteFrameCache::getInstance()->getSpriteFrameByName("01.png");
auto texture = frame->getTexture();
用纹理创建精灵帧:
auto sf=SpriteFrame::createWithTexture(Texture2D*,const Rect&);
从纹理缓冲中得到纹理:
//第一种方式
auto texture = TextureCache::getInstance()->addImage("01.png");
//第二种方式
auto texture = TextureCache::getInstance()->getTextureForKey("01.png");
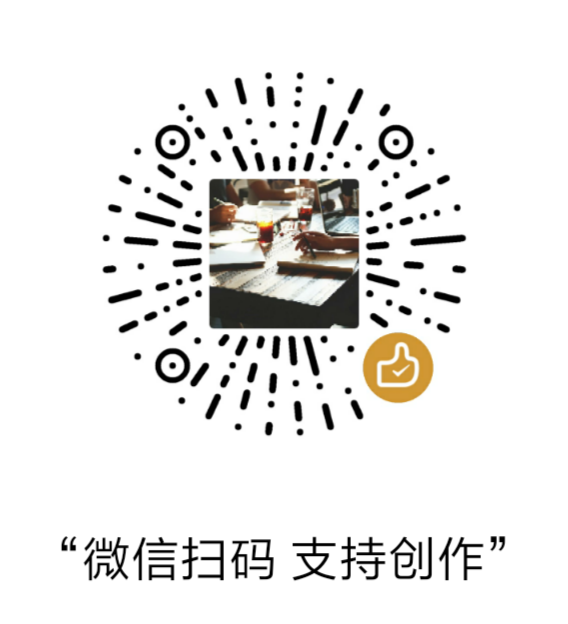