Codeforces Round #356 (Div. 2)
A little bear Limak plays a game. He has five cards. There is one number written on each card. Each number is a positive integer.
Limak can discard (throw out) some cards. His goal is to minimize the sum of numbers written on remaining (not discarded) cards.
He is allowed to at most once discard two or three cards with the same number. Of course, he won't discard cards if it's impossible to choose two or three cards with the same number.
Given five numbers written on cards, cay you find the minimum sum of numbers on remaining cards?
The only line of the input contains five integers t1, t2, t3, t4 and t5 (1 ≤ ti ≤ 100) — numbers written on cards.
Print the minimum possible sum of numbers written on remaining cards.
7 3 7 3 20
26
7 9 3 1 8
28
10 10 10 10 10
20
In the first sample, Limak has cards with numbers 7, 3, 7, 3 and 20. Limak can do one of the following.
- Do nothing and the sum would be 7 + 3 + 7 + 3 + 20 = 40.
- Remove two cards with a number 7. The remaining sum would be 3 + 3 + 20 = 26.
- Remove two cards with a number 3. The remaining sum would be 7 + 7 + 20 = 34.
You are asked to minimize the sum so the answer is 26.
In the second sample, it's impossible to find two or three cards with the same number. Hence, Limak does nothing and the sum is7 + 9 + 1 + 3 + 8 = 28.
In the third sample, all cards have the same number. It's optimal to discard any three cards. The sum of two remaining numbers is10 + 10 = 20.
记录判断

1 #include <bits/stdc++.h> 2 using namespace std; 3 4 int main() 5 { 6 int i,j; 7 int n; 8 int a[10],b[105]; 9 memset(b,0,sizeof(b)); 10 11 int sum=0,ma=0; 12 for(i=1;i<=5;i++) 13 { 14 scanf("%d",&a[i]); 15 b[a[i]]++; 16 sum+=a[i]; 17 } 18 19 for(i=1;i<=5;i++) 20 { 21 if(b[a[i]]>=2 && a[i]*2>ma) 22 { 23 ma=a[i]*2; 24 } 25 if(b[a[i]]>=3 && a[i]*3>ma) 26 { 27 ma=a[i]*3; 28 } 29 } 30 31 printf("%d\n",sum-ma); 32 }
There are n cities in Bearland, numbered 1 through n. Cities are arranged in one long row. The distance between cities i and j is equal to |i - j|.
Limak is a police officer. He lives in a city a. His job is to catch criminals. It's hard because he doesn't know in which cities criminals are. Though, he knows that there is at most one criminal in each city.
Limak is going to use a BCD (Bear Criminal Detector). The BCD will tell Limak how many criminals there are for every distance from a citya. After that, Limak can catch a criminal in each city for which he is sure that there must be a criminal.
You know in which cities criminals are. Count the number of criminals Limak will catch, after he uses the BCD.
The first line of the input contains two integers n and a (1 ≤ a ≤ n ≤ 100) — the number of cities and the index of city where Limak lives.
The second line contains n integers t1, t2, ..., tn (0 ≤ ti ≤ 1). There are ti criminals in the i-th city.
Print the number of criminals Limak will catch.
6 3
1 1 1 0 1 0
3
5 2
0 0 0 1 0
1
In the first sample, there are six cities and Limak lives in the third one (blue arrow below). Criminals are in cities marked red.
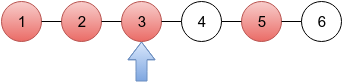
Using the BCD gives Limak the following information:
- There is one criminal at distance 0 from the third city — Limak is sure that this criminal is exactly in the third city.
- There is one criminal at distance 1 from the third city — Limak doesn't know if a criminal is in the second or fourth city.
- There are two criminals at distance 2 from the third city — Limak is sure that there is one criminal in the first city and one in the fifth city.
- There are zero criminals for every greater distance.
So, Limak will catch criminals in cities 1, 3 and 5, that is 3 criminals in total.