Codeforces Round #339 (Div.2)
Programmer Rostislav got seriously interested in the Link/Cut Tree data structure, which is based on Splay trees. Specifically, he is now studying the expose procedure.
Unfortunately, Rostislav is unable to understand the definition of this procedure, so he decided to ask programmer Serezha to help him. Serezha agreed to help if Rostislav solves a simple task (and if he doesn't, then why would he need Splay trees anyway?)
Given integers l, r and k, you need to print all powers of number k within range from l to r inclusive. However, Rostislav doesn't want to spent time doing this, as he got interested in playing a network game called Agar with Gleb. Help him!
The first line of the input contains three space-separated integers l, r and k (1 ≤ l ≤ r ≤ 1018, 2 ≤ k ≤ 109).
Print all powers of number k, that lie within range from l to r in the increasing order. If there are no such numbers, print "-1" (without the quotes).
1 10 2
1 2 4 8
2 4 5
-1
Note to the first sample: numbers 20 = 1, 21 = 2, 22 = 4, 23 = 8 lie within the specified range. The number 24 = 16 is greater then 10, thus it shouldn't be printed.


1 #include <bits/stdc++.h> 2 using namespace std; 3 4 int main() 5 { 6 long long l,r,k,m; 7 scanf("%I64d %I64d %I64d",&l,&r,&k); 8 if(k==1) 9 { 10 if(k<=r && k>=l) 11 printf("1\n"); 12 else 13 printf("-1\n"); 14 } 15 else 16 { 17 m=1; 18 int flg=0; 19 while(m<=r) 20 { 21 if(m>=l) 22 { 23 if(flg==0) 24 printf("%I64d",m); 25 else 26 printf(" %I64d",m); 27 flg=1; 28 } 29 if(r/m<k) 30 break; 31 m=m*k; 32 } 33 if(flg==0) 34 printf("-1"); 35 printf("\n"); 36 } 37 }
It's the year 4527 and the tanks game that we all know and love still exists. There also exists Great Gena's code, written in 2016. The problem this code solves is: given the number of tanks that go into the battle from each country, find their product. If it is turns to be too large, then the servers might have not enough time to assign tanks into teams and the whole game will collapse!
There are exactly n distinct countries in the world and the i-th country added ai tanks to the game. As the developers of the game are perfectionists, the number of tanks from each country is beautiful. A beautiful number, according to the developers, is such number that its decimal representation consists only of digits '1' and '0', moreover it contains at most one digit '1'. However, due to complaints from players, some number of tanks of one country was removed from the game, hence the number of tanks of this country may not remain beautiful.
Your task is to write the program that solves exactly the same problem in order to verify Gena's code correctness. Just in case.
The first line of the input contains the number of countries n (1 ≤ n ≤ 100 000). The second line contains n non-negative integers aiwithout leading zeroes — the number of tanks of the i-th country.
It is guaranteed that the second line contains at least n - 1 beautiful numbers and the total length of all these number's representations doesn't exceed 100 000.
Print a single number without leading zeroes — the product of the number of tanks presented by each country.
3
5 10 1
50
4
1 1 10 11
110
5
0 3 1 100 1
0
In sample 1 numbers 10 and 1 are beautiful, number 5 is not not.
In sample 2 number 11 is not beautiful (contains two '1's), all others are beautiful.
In sample 3 number 3 is not beautiful, all others are beautiful.


1 #include <bits/stdc++.h> 2 using namespace std; 3 4 char a[100005],b[100005]; 5 int num; 6 7 int check() 8 { 9 int i,j,k=0; 10 for(i=0;i<strlen(a);i++) 11 { 12 if(a[i]=='0' || a[i]=='1') 13 { 14 if(a[i]=='1') 15 k++; 16 if(k>1) 17 return 1; 18 } 19 else 20 return 1; 21 } 22 return 0; 23 } 24 25 void cal() 26 { 27 num=num+strlen(a)-1; 28 return ; 29 } 30 31 int main() 32 { 33 int n,flg=1; 34 int i,j,k; 35 int ch=0; 36 num=0;b[0]='1'; 37 scanf("%d",&n); 38 for(i=1;i<=n;i++) 39 { 40 scanf("%s",a); 41 if(a[0]=='0') 42 { 43 flg=0; 44 } 45 if(flg==1) 46 { 47 if(ch==0) 48 { 49 if(check()==1) 50 { 51 strcpy(b,a); 52 ch=1; 53 continue; 54 } 55 } 56 cal(); 57 } 58 } 59 60 if(flg==0) 61 { 62 printf("0\n"); 63 return 0; 64 } 65 printf("%s",b); 66 for(i=1;i<=num;i++) 67 printf("0"); 68 printf("\n"); 69 return 0; 70 }
Peter got a new snow blower as a New Year present. Of course, Peter decided to try it immediately. After reading the instructions he realized that it does not work like regular snow blowing machines. In order to make it work, you need to tie it to some point that it does not cover, and then switch it on. As a result it will go along a circle around this point and will remove all the snow from its path.
Formally, we assume that Peter's machine is a polygon on a plane. Then, after the machine is switched on, it will make a circle around the point to which Peter tied it (this point lies strictly outside the polygon). That is, each of the points lying within or on the border of the polygon will move along the circular trajectory, with the center of the circle at the point to which Peter tied his machine.
Peter decided to tie his car to point P and now he is wondering what is the area of the region that will be cleared from snow. Help him.
The first line of the input contains three integers — the number of vertices of the polygon n (), and coordinates of point P.
Each of the next n lines contains two integers — coordinates of the vertices of the polygon in the clockwise or counterclockwise order. It is guaranteed that no three consecutive vertices lie on a common straight line.
All the numbers in the input are integers that do not exceed 1 000 000 in their absolute value.
Print a single real value number — the area of the region that will be cleared. Your answer will be considered correct if its absolute or relative error does not exceed 10 - 6.
Namely: let's assume that your answer is a, and the answer of the jury is b. The checker program will consider your answer correct, if .
3 0 0
0 1
-1 2
1 2
12.566370614359172464
4 1 -1
0 0
1 2
2 0
1 1
21.991148575128551812
In the first sample snow will be removed from that area:
Lesha plays the recently published new version of the legendary game hacknet. In this version character skill mechanism was introduced. Now, each player character has exactly n skills. Each skill is represented by a non-negative integer ai — the current skill level. All skills have the same maximum level A.
Along with the skills, global ranking of all players was added. Players are ranked according to the so-called Force. The Force of a player is the sum of the following values:
- The number of skills that a character has perfected (i.e., such that ai = A), multiplied by coefficient cf.
- The minimum skill level among all skills (min ai), multiplied by coefficient cm.
Now Lesha has m hacknetian currency units, which he is willing to spend. Each currency unit can increase the current level of any skill by 1 (if it's not equal to A yet). Help him spend his money in order to achieve the maximum possible value of the Force.
The first line of the input contains five space-separated integers n, A, cf, cm and m (1 ≤ n ≤ 100 000, 1 ≤ A ≤ 109, 0 ≤ cf, cm ≤ 1000,0 ≤ m ≤ 1015).
The second line contains exactly n integers ai (0 ≤ ai ≤ A), separated by spaces, — the current levels of skills.
On the first line print the maximum value of the Force that the character can achieve using no more than m currency units.
On the second line print n integers a'i (ai ≤ a'i ≤ A), skill levels which one must achieve in order to reach the specified value of the Force, while using no more than m currency units. Numbers should be separated by spaces.
3 5 10 1 5
1 3 1
12
2 5 2
3 5 10 1 339
1 3 1
35
5 5 5
In the first test the optimal strategy is to increase the second skill to its maximum, and increase the two others by 1.
In the second test one should increase all skills to maximum.


1 #include <bits/stdc++.h> 2 using namespace std; 3 4 struct Node 5 { 6 int v; 7 int id; 8 }a[100005]; 9 long long sum[100005]; 10 bool cmp(Node x,Node y) 11 { 12 return x.v<y.v; 13 } 14 15 bool anothercmp(Node x,Node y) 16 { 17 return x.id<y.id; 18 } 19 20 int main() 21 { 22 int i,j; 23 int n,Cf,Cm; 24 long long A,m; 25 while(~scanf("%d%I64d%d%d%I64d",&n,&A,&Cf,&Cm,&m)){ 26 for(i=1;i<=n;i++) 27 { 28 scanf("%d",&a[i].v); 29 a[i].id=i; 30 } 31 sort(a+1,a+n+1,cmp); 32 sum[0]=0; 33 for(i=1;i<=n;i++) 34 { 35 sum[i]=sum[i-1]+a[i].v; 36 } 37 long long ans=-1,ansi,ansj,ansmi; 38 long long cost,remin,tmi,force; 39 for(i=0,j=1;i<=n;i++) 40 { 41 cost=A*(n-i)-(sum[n]-sum[i]); 42 if(cost>m) 43 continue; 44 remin=m-cost; 45 while(j<=i && (1ll*a[j].v*j-sum[j])<=remin) 46 { 47 j++; 48 } 49 j--; 50 if(i==0) 51 { 52 tmi=A; 53 } 54 else 55 { 56 tmi=min((remin+sum[j])/j,A); 57 } 58 force=1ll*(n-i)*Cf+1ll*tmi*Cm; 59 if(force>ans) 60 { 61 ans=force; 62 ansi=i; 63 ansj=j; 64 ansmi=tmi; 65 } 66 } 67 68 for(i=1;i<=ansj;i++) 69 { 70 a[i].v=ansmi; 71 } 72 for(i=ansi+1;i<=n;i++) 73 { 74 a[i].v=A; 75 } 76 sort(a+1,a+n+1,anothercmp); 77 printf("%I64d\n",ans); 78 79 for(i=1;i<=n;i++) 80 { 81 printf("%d ",a[i].v); 82 } 83 printf("\n"); 84 85 } 86 return 0; 87 }
Ivan wants to make a necklace as a present to his beloved girl. A necklace is a cyclic sequence of beads of different colors. Ivan says that necklace is beautiful relative to the cut point between two adjacent beads, if the chain of beads remaining after this cut is a palindrome (reads the same forward and backward).
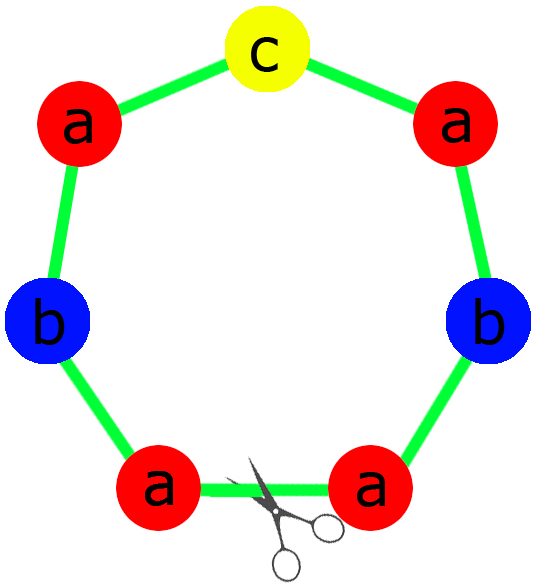
Ivan has beads of n colors. He wants to make a necklace, such that it's beautiful relative to as many cuts as possible. He certainly wants to use all the beads. Help him to make the most beautiful necklace.
The first line of the input contains a single number n (1 ≤ n ≤ 26) — the number of colors of beads. The second line contains after npositive integers ai — the quantity of beads of i-th color. It is guaranteed that the sum of ai is at least 2 and does not exceed 100 000.
In the first line print a single number — the maximum number of beautiful cuts that a necklace composed from given beads may have. In the second line print any example of such necklace.
Each color of the beads should be represented by the corresponding lowercase English letter (starting with a). As the necklace is cyclic, print it starting from any point.
3
4 2 1
1
abacaba
1
4
4
aaaa
2
1 1
0
ab
In the first sample a necklace can have at most one beautiful cut. The example of such a necklace is shown on the picture.
In the second sample there is only one way to compose a necklace.


1 #include <bits/stdc++.h> 2 using namespace std; 3 4 int gcd(int x,int y) 5 { 6 if(x<y) 7 return gcd(y,x); 8 else if(y==0) 9 return x; 10 else 11 return gcd(y,x%y); 12 } 13 14 int a[30]; 15 16 int main() 17 { 18 int n,g; 19 int i,j,k,oddnum=0; 20 scanf("%d",&n); 21 for(i=1;i<=n;i++) 22 { 23 scanf("%d",&a[i]); 24 if(a[i]%2!=0) 25 { 26 oddnum++; 27 k=i; 28 } 29 } 30 31 if(n==1) 32 { 33 printf("%d\n",a[1]); 34 while(a[1]--) printf("%c",96+1); 35 printf("\n"); 36 return 0; 37 } 38 if(oddnum>1) 39 { 40 printf("0\n"); 41 for(i=1;i<=n;i++) 42 { 43 for(j=1;j<=a[i];j++) 44 printf("%c",i+96); 45 } 46 printf("\n"); 47 } 48 else 49 { 50 g=a[1]; 51 for(i=1;i<=n;i++) 52 { 53 g=gcd(g,a[i]); 54 } 55 printf("%d\n",g);int h=g; 56 57 if(g%2==1) 58 { 59 while(h--) 60 { 61 for(i=1;i<=n;i++) 62 { 63 if(i!=k) 64 for(j=1;j<=a[i]/(g*2);j++) 65 { 66 printf("%c",i+96); 67 } 68 } 69 for(j=1;j<=a[k]/g;j++) 70 printf("%c",k+96); 71 for(i=n;i>=1;i--) 72 { 73 if(i!=k) 74 for(j=1;j<=a[i]/(g*2);j++) 75 { 76 printf("%c",i+96); 77 } 78 } 79 } 80 printf("\n"); 81 } 82 else 83 { 84 85 int flg=1; 86 while(h--) 87 { 88 if(flg==1) 89 { 90 flg=2; 91 for(i=1;i<=n;i++) 92 { 93 for(j=1;j<=a[i]/g;j++) 94 { 95 printf("%c",i+96); 96 } 97 } 98 } 99 else 100 { 101 flg=1; 102 for(i=n;i>=1;i--) 103 { 104 for(j=1;j<=a[i]/g;j++) 105 { 106 printf("%c",i+96); 107 } 108 } 109 } 110 } 111 printf("\n"); 112 } 113 } 114 }