jQuery 学习笔记(jQuery: The Return Flight)
第一课.
ajax:$.ajax(url[, settings])
练习代码:
$(document).ready(function() { $("#tour").on("click", "button", function() { $.ajax('/photos.html', { success: function(response) { $('.photos').html(response).fadeIn(); } }); }); });
<div id="tour" data-location="london"> <button>Get Photos</button> <ul class="photos"> </ul> </div>
ajax 调用简写:$.get(url, success)
$(document).ready(function() { $("#tour").on("click", "button", function() { $.get('/photos.html', function(response) { $('.photos').html(response).fadeIn(); }); }); });
ajax 传递参数:直接在 url 中拼接或使用 data 对象传递,data: { "confNum": 1234 }
练习代码:获取网页元素中的 data 属性值作为 ajax 参数值传递
$(document).ready(function() { $("#tour").on("click", "button", function() { $.ajax('/photos.html', { success: function(response) { $('.photos').html(response).fadeIn(); }, data: { "location": $("#tour").data("location") } }); }); });
<div id="tour" data-location="london"> <button>Get Photos</button> <ul class="photos"> </ul> </div>
第二课.
ajax 异常处理:error: function(request, errorType, errorMessage) { }
ajax 超时设定:timeout: 3000
ajax 调用前(beforeSend: function() {})完成后(complete: function() {})执行方法:
练习代码:
$(document).ready(function() { var el = $("#tour"); el.on("click", "button", function() { $.ajax('/photos.html', { data: {location: el.data('location')}, success: function(response) { $('.photos').html(response).fadeIn(); }, error: function(request, errorType, errorMessage) { //var errmsg = $("<li>Error: " + errorType + " with message: " + errorMessage + "</li>"); //$('.photos').append(errmsg); //alert('Error: ' + errorType + ' with message: ' + errorMessage); $('.photos').html('<li>There was a problem fetching the latest photos. Please try again.</li>'); }, timeout: 3000, beforeSend: function() { $("#tour").addClass("is-fetching") }, complete: function() { $("#tour").removeClass("is-fetching") } }); }); });
<div id="tour" data-location="london"> <button>Get Photos</button> <ul class="photos"> </ul> </div>
ajax 事件处理:处理 ajax 完成新增的标签元素的事件
练习代码:
$(document).ready(function() { function showPhotos() { $(this).find('span').slideToggle(); } $('.photos').on('mouseenter', 'li', showPhotos) .on('mouseleave', 'li', showPhotos); var el = $("#tour"); el.on("click", "button", function() { $.ajax('/photos.html', { data: {location: el.data('location')}, success: function(response) { $('.photos').html(response).fadeIn(); }, error: function() { $('.photos').html('<li>There was a problem fetching the latest photos. Please try again.</li>'); }, timeout: 3000, beforeSend: function() { $('#tour').addClass('is-fetching'); }, complete: function() { $('#tour').removeClass('is-fetching'); } }); }); });
HTML、AJAX Result
<div id="tour" data-location="london"> <button>Get Photos</button> <ul class="photos"> </ul> </div>
<li> <img src="/assets/photos/paris1.jpg"> <span style="display: none;">Arc de Triomphe</span> </li> <li> <img src="/assets/photos/paris2.jpg"> <span style="display: none;">The Eiffel Tower</span> </li> <li> <img src="/assets/photos/london.jpg"> <span style="display: none;">London</span> </li>
第三课.
JavaScript Objects:
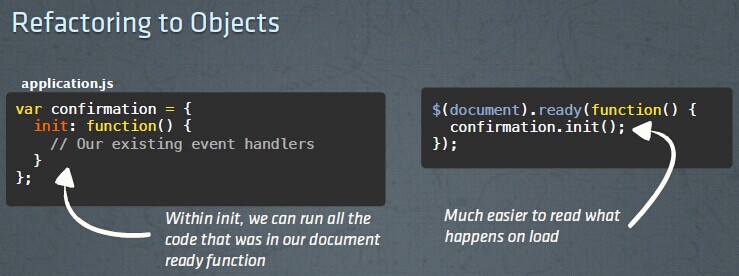
练习代码:
重构前:
$(document).ready(function() { $("#tour").on("click", "button", function() { $.ajax('/photos.html', { data: {location: $("#tour").data('location')}, success: function(response) { $('.photos').html(response).fadeIn(); }, error: function() { $('.photos').html('<li>There was a problem fetching the latest photos. Please try again.</li>'); }, timeout: 3000, beforeSend: function() { $('#tour').addClass('is-fetching'); }, complete: function() { $('#tour').removeClass('is-fetching'); } }); }); });
重构为 JavaScript 对象 tour 后:
var tour = { init: function() { $("#tour").on("click", "button", this.fetchPhotos); }, fetchPhotos: function() { $.ajax('/photos.html', { data: {location: $("#tour").data('location')}, success: function(response) { $('.photos').html(response).fadeIn(); }, error: function() { $('.photos').html('<li>There was a problem fetching the latest photos. Please try again.</li>'); }, timeout: 3000, beforeSend: function() { $('#tour').addClass('is-fetching'); }, complete: function() { $('#tour').removeClass('is-fetching'); } }) } } $(document).ready(function() { tour.init(); });
第四课.
JavaScript Functions:重点理解 this 变量的作用域
ajax 回调函数中应用调用函数变量 this 时, 需要首先在 ajax 的 context 参数中传入调用函数的 this 变量:
练习代码:
function Tour(el) { var tour = this; this.el = el; this.photos = this.el.find('.photos'); this.fetchPhotos = function() { $.ajax('/photos.html', { data: {location: tour.el.data('location')}, context: tour, success: function(response) { this.photos.html(response).fadeIn(); }, error: function() { this.photos.html('<li>There was a problem fetching the latest photos. Please try again.</li>'); }, timeout: 3000, beforeSend: function() { this.el.addClass('is-fetching'); }, complete: function() { this.el.removeClass('is-fetching'); } }); } this.el.on('click', 'button', this.fetchPhotos); } $(document).ready(function() { var paris = new Tour($('#paris')); var london = new Tour($('#london')); });
<div id="paris" data-location="paris"> <button>Get Paris Photos</button> <ul class="photos"> </ul> </div> <div id="london" data-location="london"> <button>Get London Photos</button> <ul class="photos"> </ul> </div>
第五课.
Ajax Forms
$('form').on('submit', function(event) {}); //表单提交事件
event.preventDefault(); //阻止浏览器的默认表单提交行为
type: 'POST' // POST 方式提交
data:{ "destination": $('#destination').val(), "quantity": $('#quantity').val() } //获取表单中的元素值提交数据
data: $('form').serialize() //通过表单序列化,提交整张表单中的数据
练习代码:
$(document).ready(function() { $('form').on('submit', function(event) { event.preventDefault(); $.ajax('/book', { type: 'POST', data: $('form').serialize(), success: function(response){ $('.tour').html(response); } }); }); });
<div class="tour" data-daily-price="357"> <h2>Paris, France Tour</h2> <p>$<span id="total">2,499</span> for <span id="nights-count">7</span> Nights</p> <form action="/book" method="POST"> <p> <label for="nights">Number of Nights</label> </p> <p> <input type="number" name="nights" id="nights" value="7"> </p> <input type="submit" value="book"> </form> </div>
第六课.
Ajax with JSON
dataType: 'json' // 通知服务器提交的数据类型为 json
contentType: 'application/json' //要求服务器返回的数据类型为 json
attr(<attribute>) //获取 html 对象的属性
attr(<attribute>, <value>) //给 html 对象的属性赋值
练习代码:
$(document).ready(function() { $('form').on('submit', function(event) { event.preventDefault(); $.ajax($('form').attr('action'), { type: $('form').attr('method'), data: $('form').serialize(), dataType: 'json', success: function(response) { $('.tour').html('<p></p>') .find('p') .append('Trip to ' + response.description) .append(' at $' + response.price) .append(' for ' + response.nights + ' nights') .append('. Confirmation: ' + response.confirmation); } }); }); });
<div class="tour" data-daily-price="357"> <h2>Paris, France Tour</h2> <p>$<span id="total">2,499</span> for <span id="nights-count">7</span> Nights</p> <form action="/book" method="POST"> <p> <label for="nights">Number of Nights</label> </p> <p> <input type="number" name="nights" id="nights" value="7"> </p> <input type="submit" value="book"> </form> </div>
第七课.
Utility Methods
$.each(collection, function(<index>, <object>) {}) //iterate through the array
练习代码:
$('button').on('click', function() { $.ajax('/cities/deals', { contentType: 'application/json', dataType: 'json', success: function(result) { $.each(result, function(index, dealItem) { var dealElement = $('.deal-' + index); dealElement.find('.name').html(dealItem.name); dealElement.find('.price').html(dealItem.price); }); } }); });
$.getJSON(url, success); //result will be an array of avaScript Objects
练习代码:
$('button').on('click', function() { $.getJSON('/cities/deals', function(result) { $.each(result, function(index, dealItem) { var dealElement = $('.deal-' + index); dealElement.find('.name').html(dealItem.name); dealElement.find('.price').html(dealItem.price); }); }); });
$.map(collection, function(<item>, <index>){});
练习代码:
$('.update-available-flights').on('click', function() { $.getJSON('/flights/late', function(result) { var flightElements = $.map(result, function(flightItem, index){ var liItem = $('<li></li>'); liItem.append('<p>'+flightItem.flightNumber+'</p>'); liItem.append('<p>'+flightItem.time+'</p>'); return liItem; }); $('.flight-times').html(flightElements); }); });
$.each vs $.map
.detach() //.detach() removes an element from the DOM, preserving all data and events.
detach() 方法移除被选元素,包括所有文本和子节点。
这个方法会保留 jQuery 对象中的匹配的元素,因而可以在将来再使用这些匹配的元素。
detach() 会保留所有绑定的事件、附加的数据,这一点与 remove() 不同。
练习代码:
$('.update-available-flights').on('click', function() { $.getJSON('/flights/late', function(result) { var flightElements = $.map(result, function(flightItem, index){ var flightEl = $('<li>'+flightItem.flightNumber+'-'+flightItem.time+'</li>'); return flightEl; }); $('.flight-times').detach().html(flightElements).appendTo('.flights'); }); });
第八课.
Managing Events:同一个元素同一个事件挂接多个事件处理程序,按顺序执行
off(<event name>) //停止事件的监听,同时停止当前元素上指定事件的所有监听,如:$('button').off('click');
Namespacing Events:给事件监听程序命名,用于同一个元素,相同事件多个监听程序时的区分和禁用、还原等操作
trigger(<event name>):触发被选元素的指定事件类型
create a custom event:创建自定义事件后,可以通过代码触发的方式同时触发多种页面元素的监听的相同的自定义事件。
$(<dom element>).on("<event>.<namespace>", <method>)