[LeetCode] 2049. Count Nodes With the Highest Score
There is a binary tree rooted at 0
consisting of n
nodes. The nodes are labeled from 0
to n - 1
. You are given a 0-indexed integer array parents
representing the tree, where parents[i]
is the parent of node i
. Since node 0
is the root, parents[0] == -1
.
Each node has a score. To find the score of a node, consider if the node and the edges connected to it were removed. The tree would become one or more non-empty subtrees. The size of a subtree is the number of the nodes in it. The score of the node is the product of the sizes of all those subtrees.
Return the number of nodes that have the highest score.
Example 1:
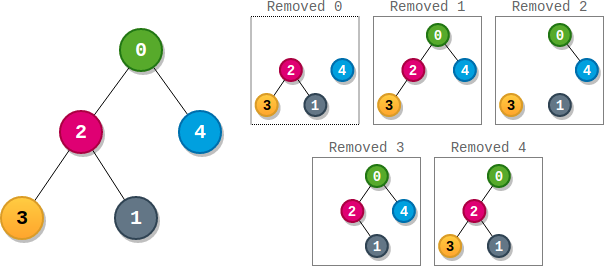
Input: parents = [-1,2,0,2,0] Output: 3 Explanation: - The score of node 0 is: 3 * 1 = 3 - The score of node 1 is: 4 = 4 - The score of node 2 is: 1 * 1 * 2 = 2 - The score of node 3 is: 4 = 4 - The score of node 4 is: 4 = 4 The highest score is 4, and three nodes (node 1, node 3, and node 4) have the highest score.
Example 2:
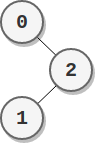
Input: parents = [-1,2,0] Output: 2 Explanation: - The score of node 0 is: 2 = 2 - The score of node 1 is: 2 = 2 - The score of node 2 is: 1 * 1 = 1 The highest score is 2, and two nodes (node 0 and node 1) have the highest score.
Constraints:
n == parents.length
2 <= n <= 105
parents[0] == -1
0 <= parents[i] <= n - 1
fori != 0
parents
represents a valid binary tree.
统计最高分的节点数目。
给你一棵根节点为 0 的 二叉树 ,它总共有 n 个节点,节点编号为 0 到 n - 1 。同时给你一个下标从 0 开始的整数数组 parents 表示这棵树,其中 parents[i] 是节点 i 的父节点。由于节点 0 是根,所以 parents[0] == -1 。
一个子树的 大小 为这个子树内节点的数目。每个节点都有一个与之关联的 分数 。求出某个节点分数的方法是,将这个节点和与它相连的边全部删除 ,剩余部分是若干个 非空 子树,这个节点的 分数 为所有这些子树大小的乘积 。
请你返回有 最高得分 节点的 数目 。
来源:力扣(LeetCode)
链接:https://leetcode.cn/problems/count-nodes-with-the-highest-score
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
这道题题意不难理解,题设类似1339题,但是实现起来难度比1339题大得多,个人觉得挺值得做的,因为思路不止一种,而且比较考验写代码熟练度。这里我提供一个思路,需要把树的结构构造出来。
因为树的构造需要自己做,所以我们在定义数据结构的时候,除了 node.left 和 node.right 定义树的左孩子和右孩子,我们还可以设置一个参数 count 记录以当前节点为根节点的子树有多少个节点。这样当我们计算某一个节点的分数的时候,我们就可以立马知道这个节点的左右子树的大小。对于一个在树中间任意位置的节点而言,他的分数 = 整棵树的节点数 total(记录在根节点 root.count)- left.count - right.count - 1。
时间O(n)
空间O(n)
Java实现
1 class Solution { 2 long highest = 0; 3 4 public int countHighestScoreNodes(int[] parents) { 5 int n = parents.length; 6 Node[] arr = new Node[n]; 7 // construct tree 8 for (int i = 0; i < n; i++) { 9 arr[i] = new Node(); 10 } 11 12 for (int i = 1; i < n; i++) { 13 // 当前节点的父节点 14 int parentId = parents[i]; 15 // 如果父节点的左孩子为空,则把当前节点接到父节点的左子树;否则就接到右子树上 16 if (arr[parentId].left == null) { 17 arr[parentId].left = arr[i]; 18 } else { 19 arr[parentId].right = arr[i]; 20 } 21 } 22 23 helper(arr[0]); 24 25 for (int i = 0; i < n; i++) { 26 long product = 1; 27 int left = arr[i].left == null ? 0 : arr[i].left.count; 28 int right = arr[i].right == null ? 0 : arr[i].right.count; 29 int rest = n - 1 - left - right; 30 if (left > 0) { 31 product *= left; 32 } 33 if (right > 0) { 34 product *= right; 35 } 36 if (rest > 0) { 37 product *= rest; 38 } 39 arr[i].product = product; 40 highest = Math.max(highest, product); 41 } 42 43 int res = 0; 44 for (int i = 0; i < n; i++) { 45 if (arr[i].product == highest) { 46 res++; 47 } 48 } 49 return res; 50 } 51 52 // find the size of current (sub)tree 53 private int helper(Node root) { 54 if (root == null) { 55 return 0; 56 } 57 int size = helper(root.left) + helper(root.right) + 1; 58 root.count = size; 59 return size; 60 } 61 62 class Node { 63 Node left; 64 Node right; 65 long product = 0L; 66 int count = 0; 67 public Node() {} 68 } 69 }
相关题目
1339. Maximum Product of Splitted Binary Tree