先看看程序界面:
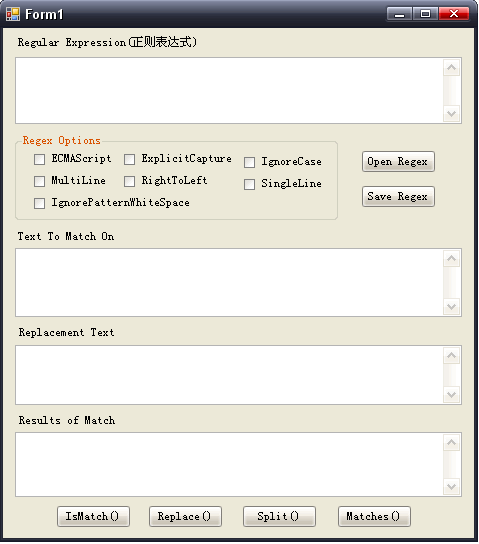
下面是程序代码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Text.RegularExpressions;
namespace Regex_DEMO
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void OpenRegexButton_Click(object sender, EventArgs e)
{
openFileDialog1.ShowDialog();
}
private void SaveRegexButton_Click(object sender, EventArgs e)
{
saveFileDialog1.ShowDialog();
}
private void saveFileDialog1_FileOk(object sender, CancelEventArgs e)
{
StreamWriter streamWriterRegex = File.CreateText(saveFileDialog1.FileName);
streamWriterRegex.Write(this.RegexTextBox.Text);
streamWriterRegex.Close();
}
private void openFileDialog1_FileOk(object sender, CancelEventArgs e)
{
StreamReader streamReaderRegex = File.OpenText(openFileDialog1.FileName);
this.RegexTextBox.Text = streamReaderRegex.ReadToEnd();
streamReaderRegex.Close();
}
private RegexOptions GetSelectedRegexOptions()
{
RegexOptions selectedRegexOptions = RegexOptions.None;
if (this.IgnoreCaseChkBox.Checked)
selectedRegexOptions |= RegexOptions.IgnoreCase;
if (this.ExplicitCaptureChkBox.Checked)
selectedRegexOptions |= RegexOptions.ExplicitCapture;
if (this.ECMAScriptchkBox.Checked)
selectedRegexOptions |= RegexOptions.ECMAScript;
if (this.IgnorePatternWhiteSpaceChkBox.Checked)
selectedRegexOptions |= RegexOptions.IgnorePatternWhitespace;
if (this.MultiLineChkBox.Checked)
selectedRegexOptions |= RegexOptions.Multiline;
if (this.RightToLeftChkBox.Checked)
selectedRegexOptions |= RegexOptions.RightToLeft;
if (this.SingleLineChkBox.Checked)
selectedRegexOptions |= RegexOptions.Singleline;
return selectedRegexOptions;
}
private void TestRegexButton_Click(object sender, EventArgs e)
{
try
{
RegexOptions selectedRegexOptions = this.GetSelectedRegexOptions();
Regex testRegex = new Regex(this.RegexTextBox.Text, selectedRegexOptions);
if (testRegex.IsMatch(this.InputTextBox.Text))
{
this.ResultsTextBox.ForeColor = Color.Black;
this.ResultsTextBox.Text = "MATCH FOUND";
}
else
{
this.ResultsTextBox.Text = "NO MATCH FOUND";
this.ResultsTextBox.ForeColor = Color.Red;
}
}
catch (ArgumentException ex)
{
this.ResultsTextBox.ForeColor = Color.Red;
this.ResultsTextBox.Text = "There was an error in your regular expression:\r\n" + ex.Message;
}
}
private void ReplaceButton_Click(object sender, EventArgs e)
{
try
{
RegexOptions selectedRegexOptions = this.GetSelectedRegexOptions();
Regex replaceRegex = new Regex(this.RegexTextBox.Text, selectedRegexOptions);
this.ResultsTextBox.ForeColor = Color.Black;
this.ResultsTextBox.Text = replaceRegex.Replace(this.InputTextBox.Text, this.ReplacementTextBox.Text);
}
catch (ArgumentException ex)
{
this.ResultsTextBox.ForeColor = Color.Red;
this.ResultsTextBox.Text = "There was an error in your regular expression;\r\n"+ex.Message;
}
}
private void SplitButton_Click(object sender, EventArgs e)
{
try
{
RegexOptions selectedRegexOptions = this.GetSelectedRegexOptions();
Regex splitRegex = new Regex(this.RegexTextBox.Text, selectedRegexOptions);
String[] splitResults;
splitResults = splitRegex.Split(this.InputTextBox.Text);
StringBuilder resultsString = new StringBuilder(this.InputTextBox.Text.Length);
foreach (String stringElement in splitResults)
resultsString.Append(stringElement + Environment.NewLine);
this.ResultsTextBox.ForeColor = Color.Black;
this.ResultsTextBox.Text = resultsString.ToString();
}
catch (ArgumentException ex)
{
this.ResultsTextBox.ForeColor = Color.Red;
this.ResultsTextBox.Text = "There was an error in your regular expression;\r\n"+ex.Message;
}
}
private void MatchesButton_Click(object sender, EventArgs e)
{
try
{
RegexOptions selectedRegexOptions = GetSelectedRegexOptions();
Regex matchesRegex = new Regex(this.RegexTextBox.Text, selectedRegexOptions);
MatchCollection matchesFound;
matchesFound = matchesRegex.Matches(this.InputTextBox.Text);
String nextMatch = "------ NEXT MATCH ------\r\n";
StringBuilder resultsString = new StringBuilder(64);
foreach (Match matchMade in matchesFound)
{
resultsString.Append(matchMade.Value + (Environment.NewLine + nextMatch));
}
this.ResultsTextBox.ForeColor = Color.Black;
this.ResultsTextBox.Text = resultsString.ToString();
}
catch (ArgumentException ex)
{
this.ResultsTextBox.ForeColor = Color.Red;
this.ResultsTextBox.Text = "There was an error in your regular expressions;\r\n"+ex.Message;
}
}
}
}
下面是窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要
/// 使用代码编辑器修改此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.RegexTextBox = new System.Windows.Forms.TextBox();
this.InputTextBox = new System.Windows.Forms.TextBox();
this.ReplacementTextBox = new System.Windows.Forms.TextBox();
this.ResultsTextBox = new System.Windows.Forms.TextBox();
this.OptionGroups = new System.Windows.Forms.GroupBox();
this.IgnorePatternWhiteSpaceChkBox = new System.Windows.Forms.CheckBox();
this.SingleLineChkBox = new System.Windows.Forms.CheckBox();
this.IgnoreCaseChkBox = new System.Windows.Forms.CheckBox();
this.RightToLeftChkBox = new System.Windows.Forms.CheckBox();
this.ExplicitCaptureChkBox = new System.Windows.Forms.CheckBox();
this.MultiLineChkBox = new System.Windows.Forms.CheckBox();
this.ECMAScriptchkBox = new System.Windows.Forms.CheckBox();
this.OpenRegexButton = new System.Windows.Forms.Button();
this.SaveRegexButton = new System.Windows.Forms.Button();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
this.TestRegexButton = new System.Windows.Forms.Button();
this.ReplaceButton = new System.Windows.Forms.Button();
this.SplitButton = new System.Windows.Forms.Button();
this.MatchesButton = new System.Windows.Forms.Button();
this.openFileDialog1 = new System.Windows.Forms.OpenFileDialog();
this.saveFileDialog1 = new System.Windows.Forms.SaveFileDialog();
this.OptionGroups.SuspendLayout();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(13, 8);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(185, 12);
this.label1.TabIndex = 0;
this.label1.Text = "Regular Expression(正则表达式)";
//
// RegexTextBox
//
this.RegexTextBox.Location = new System.Drawing.Point(12, 29);
this.RegexTextBox.Multiline = true;
this.RegexTextBox.Name = "RegexTextBox";
this.RegexTextBox.ScrollBars = System.Windows.Forms.ScrollBars.Vertical;
this.RegexTextBox.Size = new System.Drawing.Size(447, 67);
this.RegexTextBox.TabIndex = 1;
//
// InputTextBox
//
this.InputTextBox.Location = new System.Drawing.Point(12, 220);
this.InputTextBox.Multiline = true;
this.InputTextBox.Name = "InputTextBox";
this.InputTextBox.ScrollBars = System.Windows.Forms.ScrollBars.Vertical;
this.InputTextBox.Size = new System.Drawing.Size(447, 69);
this.InputTextBox.TabIndex = 2;
//
// ReplacementTextBox
//
this.ReplacementTextBox.Location = new System.Drawing.Point(12, 317);
this.ReplacementTextBox.Multiline = true;
this.ReplacementTextBox.Name = "ReplacementTextBox";
this.ReplacementTextBox.ScrollBars = System.Windows.Forms.ScrollBars.Vertical;
this.ReplacementTextBox.Size = new System.Drawing.Size(447, 60);
this.ReplacementTextBox.TabIndex = 3;
//
// ResultsTextBox
//
this.ResultsTextBox.Location = new System.Drawing.Point(12, 404);
this.ResultsTextBox.Multiline = true;
this.ResultsTextBox.Name = "ResultsTextBox";
this.ResultsTextBox.ScrollBars = System.Windows.Forms.ScrollBars.Vertical;
this.ResultsTextBox.Size = new System.Drawing.Size(447, 65);
this.ResultsTextBox.TabIndex = 4;
//
// OptionGroups
//
this.OptionGroups.Controls.Add(this.IgnorePatternWhiteSpaceChkBox);
this.OptionGroups.Controls.Add(this.SingleLineChkBox);
this.OptionGroups.Controls.Add(this.IgnoreCaseChkBox);
this.OptionGroups.Controls.Add(this.RightToLeftChkBox);
this.OptionGroups.Controls.Add(this.ExplicitCaptureChkBox);
this.OptionGroups.Controls.Add(this.MultiLineChkBox);
this.OptionGroups.Controls.Add(this.ECMAScriptchkBox);
this.OptionGroups.Location = new System.Drawing.Point(12, 106);
this.OptionGroups.Name = "OptionGroups";
this.OptionGroups.Size = new System.Drawing.Size(323, 86);
this.OptionGroups.TabIndex = 5;
this.OptionGroups.TabStop = false;
this.OptionGroups.Text = "Regex Options";
//
// IgnorePatternWhiteSpaceChkBox
//
this.IgnorePatternWhiteSpaceChkBox.AutoSize = true;
this.IgnorePatternWhiteSpaceChkBox.Location = new System.Drawing.Point(19, 61);
this.IgnorePatternWhiteSpaceChkBox.Name = "IgnorePatternWhiteSpaceChkBox";
this.IgnorePatternWhiteSpaceChkBox.Size = new System.Drawing.Size(162, 16);
this.IgnorePatternWhiteSpaceChkBox.TabIndex = 6;
this.IgnorePatternWhiteSpaceChkBox.Text = "IgnorePatternWhiteSpace";
this.IgnorePatternWhiteSpaceChkBox.UseVisualStyleBackColor = true;
//
// SingleLineChkBox
//
this.SingleLineChkBox.AutoSize = true;
this.SingleLineChkBox.Location = new System.Drawing.Point(229, 42);
this.SingleLineChkBox.Name = "SingleLineChkBox";
this.SingleLineChkBox.Size = new System.Drawing.Size(84, 16);
this.SingleLineChkBox.TabIndex = 5;
this.SingleLineChkBox.Text = "SingleLine";
this.SingleLineChkBox.UseVisualStyleBackColor = true;
//
// IgnoreCaseChkBox
//
this.IgnoreCaseChkBox.AutoSize = true;
this.IgnoreCaseChkBox.Location = new System.Drawing.Point(229, 20);
this.IgnoreCaseChkBox.Name = "IgnoreCaseChkBox";
this.IgnoreCaseChkBox.Size = new System.Drawing.Size(84, 16);
this.IgnoreCaseChkBox.TabIndex = 4;
this.IgnoreCaseChkBox.Text = "IgnoreCase";
this.IgnoreCaseChkBox.UseVisualStyleBackColor = true;
//
// RightToLeftChkBox
//
this.RightToLeftChkBox.AutoSize = true;
this.RightToLeftChkBox.Location = new System.Drawing.Point(109, 39);
this.RightToLeftChkBox.Name = "RightToLeftChkBox";
this.RightToLeftChkBox.Size = new System.Drawing.Size(90, 16);
this.RightToLeftChkBox.TabIndex = 3;
this.RightToLeftChkBox.Text = "RightToLeft";
this.RightToLeftChkBox.UseVisualStyleBackColor = true;
//
// ExplicitCaptureChkBox
//
this.ExplicitCaptureChkBox.AutoSize = true;
this.ExplicitCaptureChkBox.Location = new System.Drawing.Point(109, 17);
this.ExplicitCaptureChkBox.Name = "ExplicitCaptureChkBox";
this.ExplicitCaptureChkBox.Size = new System.Drawing.Size(114, 16);
this.ExplicitCaptureChkBox.TabIndex = 2;
this.ExplicitCaptureChkBox.Text = "ExplicitCapture";
this.ExplicitCaptureChkBox.UseVisualStyleBackColor = true;
//
// MultiLineChkBox
//
this.MultiLineChkBox.AutoSize = true;
this.MultiLineChkBox.Location = new System.Drawing.Point(19, 39);
this.MultiLineChkBox.Name = "MultiLineChkBox";
this.MultiLineChkBox.Size = new System.Drawing.Size(78, 16);
this.MultiLineChkBox.TabIndex = 1;
this.MultiLineChkBox.Text = "MultiLine";
this.MultiLineChkBox.UseVisualStyleBackColor = true;
//
// ECMAScriptchkBox
//
this.ECMAScriptchkBox.AutoSize = true;
this.ECMAScriptchkBox.Location = new System.Drawing.Point(19, 17);
this.ECMAScriptchkBox.Name = "ECMAScriptchkBox";
this.ECMAScriptchkBox.Size = new System.Drawing.Size(84, 16);
this.ECMAScriptchkBox.TabIndex = 0;
this.ECMAScriptchkBox.Text = "ECMAScript";
this.ECMAScriptchkBox.UseVisualStyleBackColor = true;
//
// OpenRegexButton
//
this.OpenRegexButton.Location = new System.Drawing.Point(358, 122);
this.OpenRegexButton.Name = "OpenRegexButton";
this.OpenRegexButton.Size = new System.Drawing.Size(75, 23);
this.OpenRegexButton.TabIndex = 6;
this.OpenRegexButton.Text = "Open Regex";
this.OpenRegexButton.UseVisualStyleBackColor = true;
this.OpenRegexButton.Click += new System.EventHandler(this.OpenRegexButton_Click);
//
// SaveRegexButton
//
this.SaveRegexButton.Location = new System.Drawing.Point(358, 157);
this.SaveRegexButton.Name = "SaveRegexButton";
this.SaveRegexButton.Size = new System.Drawing.Size(75, 23);
this.SaveRegexButton.TabIndex = 7;
this.SaveRegexButton.Text = "Save Regex";
this.SaveRegexButton.UseVisualStyleBackColor = true;
this.SaveRegexButton.Click += new System.EventHandler(this.SaveRegexButton_Click);
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(13, 202);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(101, 12);
this.label2.TabIndex = 8;
this.label2.Text = "Text To Match On";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(14, 298);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(101, 12);
this.label3.TabIndex = 9;
this.label3.Text = "Replacement Text";
//
// label4
//
this.label4.AutoSize = true;
this.label4.Location = new System.Drawing.Point(14, 386);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(101, 12);
this.label4.TabIndex = 10;
this.label4.Text = "Results of Match";
//
// TestRegexButton
//
this.TestRegexButton.Location = new System.Drawing.Point(53, 477);
this.TestRegexButton.Name = "TestRegexButton";
this.TestRegexButton.Size = new System.Drawing.Size(75, 23);
this.TestRegexButton.TabIndex = 11;
this.TestRegexButton.Text = "IsMatch()";
this.TestRegexButton.UseVisualStyleBackColor = true;
this.TestRegexButton.Click += new System.EventHandler(this.TestRegexButton_Click);
//
// ReplaceButton
//
this.ReplaceButton.Location = new System.Drawing.Point(145, 477);
this.ReplaceButton.Name = "ReplaceButton";
this.ReplaceButton.Size = new System.Drawing.Size(75, 23);
this.ReplaceButton.TabIndex = 12;
this.ReplaceButton.Text = "Replace()";
this.ReplaceButton.UseVisualStyleBackColor = true;
this.ReplaceButton.Click += new System.EventHandler(this.ReplaceButton_Click);
//
// SplitButton
//
this.SplitButton.Location = new System.Drawing.Point(239, 477);
this.SplitButton.Name = "SplitButton";
this.SplitButton.Size = new System.Drawing.Size(75, 23);
this.SplitButton.TabIndex = 13;
this.SplitButton.Text = "Split()";
this.SplitButton.UseVisualStyleBackColor = true;
this.SplitButton.Click += new System.EventHandler(this.SplitButton_Click);
//
// MatchesButton
//
this.MatchesButton.Location = new System.Drawing.Point(334, 477);
this.MatchesButton.Name = "MatchesButton";
this.MatchesButton.Size = new System.Drawing.Size(75, 23);
this.MatchesButton.TabIndex = 14;
this.MatchesButton.Text = "Matches()";
this.MatchesButton.UseVisualStyleBackColor = true;
this.MatchesButton.Click += new System.EventHandler(this.MatchesButton_Click);
//
// openFileDialog1
//
this.openFileDialog1.FileName = "openFileDialog1";
this.openFileDialog1.FileOk += new System.ComponentModel.CancelEventHandler(this.openFileDialog1_FileOk);
//
// saveFileDialog1
//
this.saveFileDialog1.FileOk += new System.ComponentModel.CancelEventHandler(this.saveFileDialog1_FileOk);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(471, 510);
this.Controls.Add(this.MatchesButton);
this.Controls.Add(this.SplitButton);
this.Controls.Add(this.ReplaceButton);
this.Controls.Add(this.TestRegexButton);
this.Controls.Add(this.label4);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.SaveRegexButton);
this.Controls.Add(this.OpenRegexButton);
this.Controls.Add(this.OptionGroups);
this.Controls.Add(this.ResultsTextBox);
this.Controls.Add(this.ReplacementTextBox);
this.Controls.Add(this.InputTextBox);
this.Controls.Add(this.RegexTextBox);
this.Controls.Add(this.label1);
this.Name = "Form1";
this.Text = "Form1";
this.Load += new System.EventHandler(this.Form1_Load);
this.OptionGroups.ResumeLayout(false);
this.OptionGroups.PerformLayout();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TextBox RegexTextBox;
private System.Windows.Forms.TextBox InputTextBox;
private System.Windows.Forms.TextBox ReplacementTextBox;
private System.Windows.Forms.TextBox ResultsTextBox;
private System.Windows.Forms.GroupBox OptionGroups;
private System.Windows.Forms.CheckBox IgnoreCaseChkBox;
private System.Windows.Forms.CheckBox RightToLeftChkBox;
private System.Windows.Forms.CheckBox ExplicitCaptureChkBox;
private System.Windows.Forms.CheckBox MultiLineChkBox;
private System.Windows.Forms.CheckBox ECMAScriptchkBox;
private System.Windows.Forms.CheckBox IgnorePatternWhiteSpaceChkBox;
private System.Windows.Forms.CheckBox SingleLineChkBox;
private System.Windows.Forms.Button OpenRegexButton;
private System.Windows.Forms.Button SaveRegexButton;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Button TestRegexButton;
private System.Windows.Forms.Button ReplaceButton;
private System.Windows.Forms.Button SplitButton;
private System.Windows.Forms.Button MatchesButton;
private System.Windows.Forms.OpenFileDialog openFileDialog1;
private System.Windows.Forms.SaveFileDialog saveFileDialog1;