Dubbo系列之服务注册与发现
开发工具idea
1.首先启动zookeeper服务端
(1)先打包jar包(打开命令窗口cmd进入项目中mvn clean package )
(2)接着运行jar(打开项目进入到target里找到jar,然后cmd运行 java -jar dubbo-admin-0.0.1-SNAPSHOT.jar)
这样 zookeeper就运行成功了
2.启动dubbo-admin管理员
运行成功之后,访问: http://127.0.0.1:7001,输入默认的账号密码root/root,登录成功
3.Dubbo服务注册与发现
业务场景
4.api工程创建
api模块:dubbo-mall-interface
用户地址DTO类:
package com.ckf.mall.entity;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.io.Serializable;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class UserAddress implements Serializable {
private Integer id;
private String userAddress; //用户地址
private String userId; //用户id
private String consignee; //收货人
private String phoneNum; //电话号码
private String isDefault; //是否为默认地址 Y-是 N-否
}
用户信息服务接口:
package com.ckf.mall.service;
import com.ckf.mall.entity.UserAddress;
import java.util.List;
/**
* 用户服务接口
*/
public interface UserService {
/**
* 按照用户id返回所有的收货地址
* @param userId
* @return
*/
public List<UserAddress> getUserAddressList(String userId);
}
订单信息服务接口:
package com.ckf.mall.service;
import com.ckf.mall.entity.UserAddress;
import java.util.List;
/**
* 订单服务接口
*/
public interface OrdersService {
/**
* 初始化订单
* @param userId
*/
public List<UserAddress> initOrder(String userId);
}
这样创api工程建好了
5.服务提供者工程
创建一个user-service-provider模块:
maven配置:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>dubbo</artifactId>
<version>2.6.2</version>
</dependency>
<!-- 注册中心使用的是zookeeper,引入操作zookeeper的客户端端 -->
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-framework</artifactId>
<version>2.12.0</version>
</dependency>
<dependency>
<groupId>org.example</groupId>
<artifactId>dubbo-mall-interface</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
resources加一个提供者的配置文件provider.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://dubbo.apache.org/schema/dubbo http://dubbo.apache.org/schema/dubbo/dubbo.xsd
http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<!-- 1、指定当前服务/应用的名字(同样的服务名字相同,不要和别的服务同名) -->
<dubbo:application name="user-service-provider"></dubbo:application>
<!-- 2、指定注册中心的位置 -->
<!-- <dubbo:registry address="zookeeper://127.0.0.1:2181"></dubbo:registry> -->
<dubbo:registry protocol="zookeeper" address="127.0.0.1:2181"></dubbo:registry>
<!-- 3、指定通信规则(通信协议 通信端口) -->
<dubbo:protocol name="dubbo" port="20882"></dubbo:protocol>
<!-- 4、暴露服务 ref:指向服务的真正的实现对象 -->
<dubbo:service interface="com.ckf.mall.service.UserService"
ref="userServiceImpl01" timeout="1000" version="1.0.0">
<dubbo:method name="getUserAddressList" timeout="1000"></dubbo:method>
</dubbo:service>
<!--统一设置服务提供方的规则 -->
<dubbo:provider timeout="1000"></dubbo:provider>
<!-- 服务的实现 -->
<bean id="userServiceImpl01" class="com.ckf.mall.service.impl.UserServiceImpl"></bean>
<!-- 连接监控中心 -->
<dubbo:monitor protocol="registry"></dubbo:monitor>
</beans>
业务实现类:
package com.ckf.mall.service.impl;
import com.alibaba.dubbo.config.annotation.Service;
import com.ckf.mall.entity.UserAddress;
import com.ckf.mall.service.UserService;
import org.springframework.stereotype.Component;
import java.util.Arrays;
import java.util.List;
/**
* 服务提供者,需要用dubbo中的@Service来暴露服务
*/
public class UserServiceImpl implements UserService {
@Override
public List<UserAddress> getUserAddressList(String userId) {
System.out.println("UserServiceImpl..3.....");
UserAddress address1 = new UserAddress(1, "北京市昌平区宏福科技园综合楼3层", "1", "李老师", "010-56253825", "Y");
UserAddress address2 = new UserAddress(2, "深圳市宝安区西部硅谷大厦B座3层(深圳分校)", "1", "王老师", "010-56253825", "N");
UserAddress address3 = new UserAddress(3, "深圳市宝安区西部硅谷大厦B座8层(湛江分校)", "1", "陈老师", "010-56253825", "N");
return Arrays.asList(address1,address2,address3);
}
}
启动服务提供者,注册到Zookeeper:
package com.ckf.mall;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.io.IOException;
public class MainApplication {
public static void main(String[] args) throws IOException {
ClassPathXmlApplicationContext ioc = new ClassPathXmlApplicationContext("provider.xml");
ioc.start();
System.in.read();
}
}
服务注册成功,可以去dubbo-admin看
6.服务消费者模块
服务已经注册了,现在创建一个消费者工程order-service-comsumer
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.example</groupId>
<artifactId>dubbo-mall-interface</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.alibaba/dubbo -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>dubbo</artifactId>
<version>2.6.2</version>
</dependency>
<!-- 注册中心使用的是zookeeper,引入操作zookeeper的客户端端 -->
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-framework</artifactId>
<version>2.12.0</version>
</dependency>
消费者配置文件
consumer.xml 文件如下:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://dubbo.apache.org/schema/dubbo"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://dubbo.apache.org/schema/dubbo http://dubbo.apache.org/schema/dubbo/dubbo.xsd
http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<context:component-scan base-package="com.ckf.mall.service.impl"></context:component-scan>
<dubbo:application name="orders-service-consumer"></dubbo:application>
<dubbo:registry address="zookeeper://127.0.0.1:2181"></dubbo:registry>
<!-- 配置本地存根-->
<!--声明需要调用的远程服务的接口;生成远程服务代理 -->
<!--
1)、精确优先 (方法级优先,接口级次之,全局配置再次之)
2)、消费者设置优先(如果级别一样,则消费方优先,提供方次之)
-->
<!-- timeout="0" 默认是1000ms-->
<!-- retries="":重试次数,不包含第一次调用,0代表不重试-->
<!-- 幂等(设置重试次数)【查询、删除、修改】、非幂等(不能设置重试次数)【新增】 -->
<dubbo:reference interface="com.ckf.mall.service.UserService"
id="userService" timeout="5000" retries="3" version="*">
<!-- <dubbo:method name="getUserAddressList" timeout="1000"></dubbo:method> -->
</dubbo:reference>
<!-- 配置当前消费者的统一规则:所有的服务都不检查 -->
<dubbo:consumer check="false" timeout="5000"></dubbo:consumer>
<dubbo:monitor protocol="registry"></dubbo:monitor>
<!-- <dubbo:monitor address="127.0.0.1:7070"></dubbo:monitor> -->
</beans>
订单服务类:
package com.ckf.mall.service.impl;
import com.ckf.mall.entity.UserAddress;
import com.ckf.mall.service.OrdersService;
import com.ckf.mall.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class OrdersServiceImpl implements OrdersService {
@Autowired
UserService userService;
@Override
public List<UserAddress> initOrder(String userId) {
System.out.println("用户id:"+userId);
/**
* 查询用户的收货地址
*/
List<UserAddress> addressList = userService.getUserAddressList(userId);
for (UserAddress userAddress : addressList) {
System.out.println(userAddress.getUserAddress());
}
return addressList;
}
}
消费者启动类:
package com.ckf.mall;
import com.ckf.mall.service.OrdersService;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.io.IOException;
public class MainApplication {
@SuppressWarnings("resource")
public static void main(String[] args) throws IOException {
ClassPathXmlApplicationContext applicationContext = new ClassPathXmlApplicationContext("consumer.xml");
OrdersService orderService = applicationContext.getBean(OrdersService.class);
orderService.initOrder("1");
System.out.println("调用成功....");
System.in.read();
}
}
调用成功,控制台打印信息如下:
dubbo-admin查询消费者效果图如下:
首页效果如下:
还有一种方法使用注解的方式暴露服务
需要加入依赖如下:
<dependency>
<groupId>com.alibaba.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>0.2.0</version>
</dependency>
在服务供应者的实现类上加入@sevice(注意导入的是阿里巴巴的而不是spring的)
服务供应者类:
然后在启动类加上注解如下:
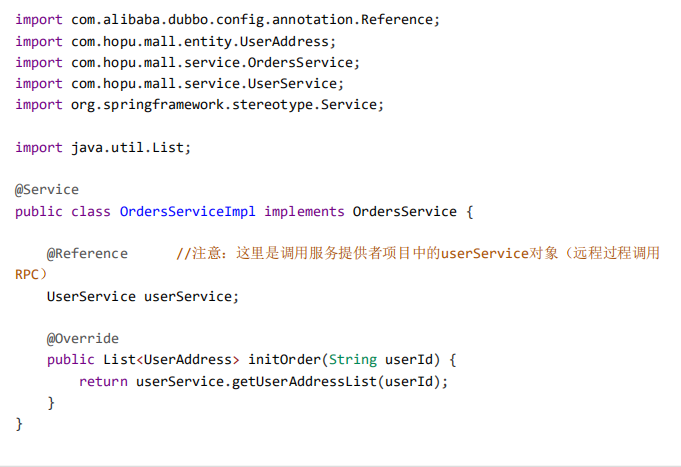
码云地址:https://gitee.com/ckfeng/dubbo-mall.git
第二种方法实现的地址:https://gitee.com/ckfeng/ckf-mall-dobbo.git