【一】序列化模块
【1】json模块
- 将python对象序列化成json字符串
- 将json字符串反序列化成python对象
import json
json.dump() # 写文件
json.dumps() # 转换字符串
json.load() # 读数据
json.loads() # 将字符串转回对象
【2】pickle模块
- 用于python特有的类型和 python的数据类型间进行转换
import pickle
# 序列化方法(dumps)
dic = {'k1': 'v1', 'k2': 'v2', 'k3': 'v3'}
str_dic = pickle.dumps(dic)
print(str_dic)
# b'\x80\x04\x95#\x00\x00\x00\x00\x00\x00\x00}\x94(\x8c\x02k1\x94\x8c\x02v1\x94\x8c\x02k2\x94\x8c\x02v2\x94\x8c\x02k3\x94\x8c\x02v3\x94u.'
print(type(str_dic))
# <class 'bytes'>
# 反序列化方法(loads)
dic2 = pickle.loads(str_dic)
print(dic2)
# {'k1': 'v1', 'k2': 'v2', 'k3': 'v3'}
print(type(dic2))
# <class 'dict'>
import pickle
user_data = {'username':'chosen'}
# 写入文件(dump)
def save_data():
with open('data', 'wb') as fp:
pickle.dump(obj=user_data, file=fp)
save_data() # 写入之后在文件夹里用记事本方式打开发现是乱码
# €? }攲username攲chosen攕.
# 读取文件数据(load)
def read_data():
with open('data', 'rb') as fp:
data = pickle.load(file=fp)
print(data)
read_data() #读取文件后 可以将乱码转化为写入前的正常文字
# {'username': 'chosen'}
import pickle
def save_data(data):
with open('data','wb') as fp:
pickle.dump(obj=data,file=fp)
def read_data():
with open('data','rb') as fp:
data=pickle.load(file=fp)
print(data(5,6))
def add(x,y):
print(x+y)
save_data(data=add) # €? ?__main__攲add敁?
read_data() # 11
【3】总结
- json是一种所有的语言都可以识别的数据结构
- 所以我们序列化的内容是列表或者字典,推荐使用json模块
- 如果出于某种原因不得不序列化其他的数据类型,或者反序列的话,可以使用pickle
【二】subprocess模块
【1】popen
import subprocess
res = subprocess.Popen('dir(这里放的是linux命令或者shell命令)', shell=True,
stdout=subprocess.PIPE, # 管道 负责存储正确的信息
stderr=subprocess.PIPE # 管道 负责存储错误信息
)
print(res) # <subprocess.Popen object at 0x000001ABB1970310>
# print(res.stdout.read().decode('gbk')) # tasklist执行之后的正确结果返回
print(res.stderr.read().decode('gbk'))
- subprocess模块首先推荐使用的是它的 run 方法
- 更高级的用法可以直接使用Popen接口
【2】run
def runcmd(command):
ret = subprocess.run(command, # 子进程要执行的命令
shell=True, # 执行的是shell的命令
stdout=subprocess.PIPE,
stderr=subprocess.PIPE,
# encoding="utf-8",
timeout=1
)
# print( ret.returncode)
# returncode属性是run()函数返回结果的状态。
if ret.returncode == 0:
print("success:", ret.stdout)
else:
print("error:", ret)
runcmd(["dir",'/b']) # 序列参数
# success: CompletedProcess(args=['dir', '/b'], returncode=0, stderr='')
# runcmd("exit 1") # 字符串参数
# error: CompletedProcess(args='exit 1', returncode=1, stdout='', stderr='')
【3】call
subprocess.call(['python', '--version']) #查看当前python解释器版本
【三】re模块
- 正则:按照指定的匹配规则从字符串中匹配或者截取相应的内容
【1】引入
# 手机号码必须是 11 位,并且是数字 ,必须符合国家规范
import re
def check_phone_re(phone_number):
if re.match('^(13|14|15|18)[0-9]{9}$', phone_number):
print('是合法的手机号码')
else:
print('不是合法的手机号码')
phone = input("phone :>>>> ").strip()
check_phone_re(phone_number=phone)
【2】字符组
- 字符组 :[字符组] 在同一个位置可能出现的各种字符组成了一个字符组
- 在正则表达式中用[]表示
正则 |
待匹配字符 |
匹配结果 |
说明 |
[0123456789] |
8 |
True |
在一个字符组里枚举合法的所有字符,字符组里的任意一个字符和"待匹配字符"相同都视为可以匹配 |
[0123456789] |
a |
False |
由于字符组中没有"a"字符,所以不能匹配 |
[0-9] |
7 |
True |
也可以用-表示范围,[0-9]就和[0123456789]是一个意思 |
[a-z] |
s |
True |
同样的如果要匹配所有的小写字母,直接用[a-z]就可以表示 |
[A-Z] |
B |
True |
[A-Z]就表示所有的大写字母 |
[0-9a-fA-F] |
e |
True |
可以匹配数字,大小写形式的a~f,用来验证十六进制字符 |
# (1) 匹配 0 - 9 数字
import re
pattern = "[0123456789]"
res = re.findall(pattern, 'a b c d e f g h i j k 1 2 3 4 5')
print(res) # ['1', '2', '3', '4', '5']
pattern = "[0-9]"
res = re.findall(pattern, 'a b c d e f g h i j k 1 2 3 4 5')
print(res) # ['1', '2', '3', '4', '5']
# (2)匹配小写字母
pattern = "[abcd]"
res = re.findall(pattern, 'a b c d e f g h i j k 1 2 3 4 5')
print(res) # ['a', 'b', 'c', 'd']
pattern = "[a-z]"
res = re.findall(pattern, 'a b c d e f g h i j k A B C D E 1 2 3 4 5')
print(res) # ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k']
# (3)匹配大写字母字符组
pattern = "[ABC]"
res = re.findall(pattern, 'a b c d e f g h i j k A B C D E 1 2 3 4 5')
print(res) # ['A', 'B', 'C']
pattern = "[A-Z]"
res = re.findall(pattern, 'a b c d e f g h i j k A B C D E 1 2 3 4 5')
print(res) # ['A', 'B', 'C', 'D', 'E']
# (4)大小写字母 + 数字混合
pattern = "[0123abcdABC]"
res = re.findall(pattern, 'a b c d e f g h i j k A B C D E 1 2 3 4 5')
print(res) # ['a', 'b', 'c', 'd', 'A', 'B', 'C', '1', '2', '3']
pattern = "[0-9a-zA-Z]"
res = re.findall(pattern, 'a b c d e f g h i j k A B C D E 1 2 3 4 5')
print(res) # ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'A', 'B', 'C', 'D', 'E', '1', '2', '3', '4', '5']
【3】元字符
元字符 |
匹配内容 |
. |
匹配除换行符以外的任意字符 |
\w |
匹配字母或数字或下划线 |
\s |
匹配任意的空白符 |
\d |
匹配数字 |
\n |
匹配一个换行符 |
\t |
匹配一个制表符 |
\b |
匹配一个单词的结尾 |
^ |
匹配字符串的开始 |
$ |
匹配字符串的结尾 |
\W |
匹配非字母或数字或下划线 |
\D |
匹配非数字 |
\S |
匹配非空白符 |
a|b |
匹配字符a或字符b |
() |
匹配括号内的表达式,也表示一个组 |
[...] |
匹配字符组中的字符 |
[^...] |
匹配除了字符组中字符的所有字符 |
import re
# (1) . 代表匹配除换行符以外的任意字符
letter = 'abcdefghijkABCDE12345 , '
pattern = "."
res = re.findall(pattern, letter)
print(res)
# ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'A', 'B', 'C', 'D', 'E', '1', '2', '3', '4', '5', ' ', ',', ' ']
# (2) \w 代表匹配数字或者字母或下划线
letter = 'a b c d e f g h i j k A B C D E 1 2 3 4 5 , _ . ; % '
pattern = "\w"
res = re.findall(pattern, letter)
print(res)
# ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'A', 'B', 'C', 'D', 'E', '1', '2', '3', '4', '5', '_']
# (3) \s 代表匹配任意的空白符 ---> 空格
letter = 'a b c d e f g h i j k A B C D E 1 2 3 4 5 , _ . ; % '
pattern = "\s"
res = re.findall(pattern, letter)
print(res)
# [' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ']
# (4) \d 只能匹配数字
letter = 'a b c d e f g h i j k A B C D E 1 2 3 4 5 , _ . ; % '
pattern = "\d"
res = re.findall(pattern, letter)
print(res) # ['1', '2', '3', '4', '5']
# (5) \W 匹配除了字母 或数字 或下滑线以外的任意字符
letter = 'a b c d e f g h i j k A B C D E 1 2 3 4 5 , _ . ; % '
pattern = "\W"
res = re.findall(pattern, letter)
print(res)
# [' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ',', ' ', ' ', '.', ' ', ';', ' ', '%', ' ']
# (6) \n 只能匹配换行符
letter = '''
' a
b c
d e f g h i j k A B C D E
1 2 3 4 5 , _ . ; %
'''
pattern = "\n"
res = re.findall(pattern, letter)
print(res)
# ['\n', '\n', '\n', '\n', '\n']
# (7) \t 制表符
msg="""h e\tll\n\no 123_ (0
\t1
"""
print(re.findall('\t',msg)) # ['\t', '\t']
# (8) \b 匹配一个单词的结尾
# (9) ^字符 匹配以某个字符开头
letter = '''a b c d e f g h i j k A B C D E 1 2 3 4 5 , _ . ; %'''
pattern = "^a"
res = re.findall(pattern, letter)
print(res) # ['a']
# (10) 字符$ 匹配以某个字符结尾
letter = '''a b c d e f g h i j k A B C D E 1 2 3 4 5 , _ . ; %'''
pattern = "%$"
res = re.findall(pattern, letter)
print(res) # ['%']
# (11) \D 匹配除数字以外的任意字符
letter = '''a b c d e B C D E 1 2 3 4 5 , _ . ; %'''
pattern = "\D"
res = re.findall(pattern, letter)
print(res)
# ['a', ' ', ' ', 'b', ' ', 'c', ' ', 'd', ' ', 'e', ' ', 'B', ' ', 'C', ' ', 'D', ' ', 'E', ' ', ' ', ' ', ' ', ' ', ' ', ',', ' ', '_', ' ', '.', ' ', ';', ' ', '%']
# (12) \S 匹配出了空格以外的所有字符
letter = '''a b c d e f g h
i j k A B C D E 1 2 3 4 5 , _ . ; %'''
pattern = "\S"
res = re.findall(pattern, letter)
print(res)
# ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'A', 'B', 'C', 'D', 'E', '1', '2', '3', '4', '5', ',', '_', '.', ';', '%']
# (13) 字符|字符 匹配任意一个字符
letter = '''a b c d e f g h
i j k A B C D E 1 2 3 4 5 , _ . ; %'''
pattern = "a|b|1|%"
res = re.findall(pattern, letter)
print(res) # ['a', 'b', '1', '%']
# (14) () 声明优先级
letter = '''a b c d e f g hi j k A B C D E 12 aa 34 5a , _ . ; %'''
pattern = "\d(\w)"
res = re.findall(pattern, letter)
print(res) # ['2', '4', 'a']
# (15)字符组
letter = '''a b c d e f g hi j k A B C D E 12 aa 34 5a , _ . ; %'''
pattern = "[a-z0-9A-Z][0-9]"
# pattern = "[a-z0-9A-Z][a-z]"
res = re.findall(pattern, letter)
print(res) # ['12', '34']
letter = '''a b c d e $f _g hi j k A B C D E 12 aa 34 5a , _ . ; %'''
# 匹配除了字符组中字符的所有字符
# $f _g hi 12 aa 34 5a
pattern = "[^a-z0-9A-Z][0-9]"
res = re.findall(pattern, letter)
print(res) # [' 1', ' 3', ' 5']
pattern = "[a-z0-9A-Z][0-^9]"
res = re.findall(pattern, letter)
print(res) # ['12', '34']
【4】量词
量词 |
用法说明 |
* |
重复零次或更多次 |
+ |
重复一次或更多次 |
? |
重复零次或一次 |
{n} |
重复n次 |
{n,} |
重复n次或更多次 |
{n,m} |
重复n到m次 |
import re
# (1) * 代表当前字符重读零次或更多次
pattern = "[0-9][a-z]*" #
letter = '''a b c d e $f _g hi j k A B C D E 12 aa 34 5a 111 6cs 9nb 6aaaa , _ . ; %'''
res = re.findall(pattern, letter)
print(res) # ['1', '2', '3', '4', '5a', '1', '1', '1', '6cs', '9nb', '6aaaa']
# (2) + 代表当前字符重读一次或更多次
pattern = "[0-9][a-z]+" #
letter = '''a b c d e $f _g hi j k A B C D E 12 aa 34 5a 111 6cs 9nb 6aaaa , _ . ; %'''
res = re.findall(pattern, letter)
print(res) # ['5a', '6cs', '9nb', '6aaaa']
# (3)? 重复零次或一次
pattern = "[0-9][a-z]?" #
letter = '''a b c d e $f _g hi j k A B C D E 12 aa 34 5a 111 6cs 9nb 6aaaa , _ . ; %'''
res = re.findall(pattern, letter)
print(res) # ['1', '2', '3', '4', '5a', '1', '1', '1', '6c', '9n', '6a']
# (4){n} 重复n次
pattern = "[0-9][a-z]{2}" #
letter = '''a b c d e $f _g hi j k A B C D E 12 aa 34 5a 111 6cs 9nb 6aaaa , _ . ; %'''
res = re.findall(pattern, letter)
print(res) # ['6cs', '9nb', '6aa']
# (5){n,m} 重复至少n次 至多m次
pattern = "[0-9][a-z]{2,3}" #
letter = '''a b c d e $f _g hi j k A B C D E 12 aa 34 5a 111 6cs 9nb 6aaaa , _ . ; %'''
res = re.findall(pattern, letter)
print(res) # ['6cs', '9nb', '6aaa']
【5】位置元字符
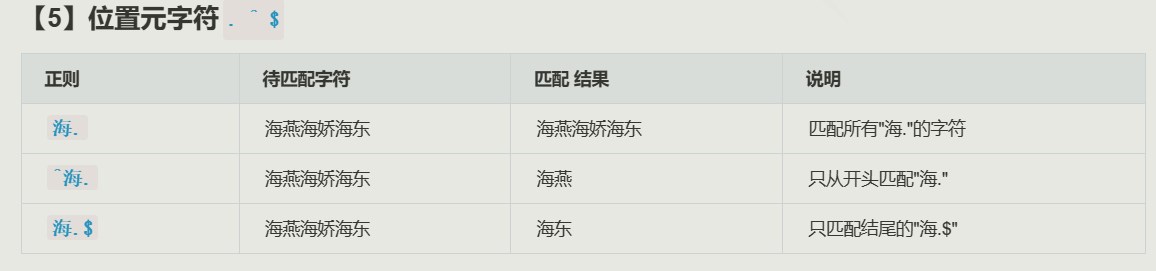
# . 代表任意字符
pattern = "海."
letter = '''海燕海娇海东海冬梅'''
res = re.findall(pattern, letter)
print(res) # ['海燕', '海娇', '海东', '海冬']
# . 代表任意字符
# ^ 以 ... 开头
pattern = "^海."
letter = '''海燕海娇海东海冬梅'''
res = re.findall(pattern, letter)
print(res) # ['海燕']
# . 代表任意字符
# $ 以 ... 结尾
pattern = "海.$"
letter = '''海燕海娇海东海冬梅'''
res = re.findall(pattern, letter)
print(res) # ['海冬']
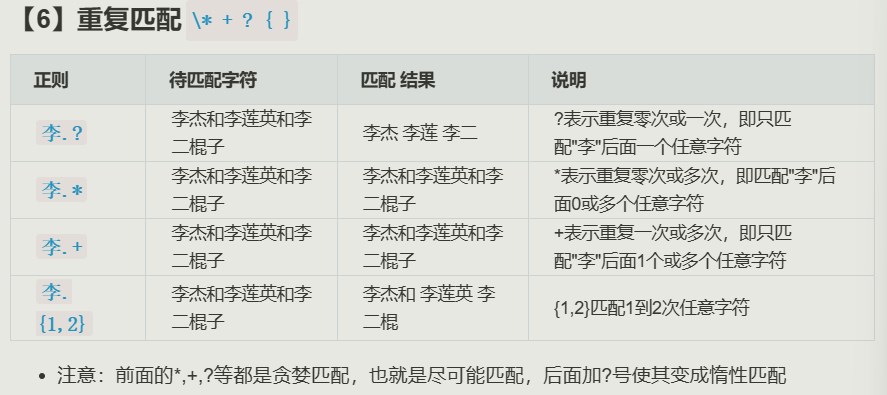
# . 代表任意字符
# ? 重复零次或一次
pattern = "李.?"
letter = '李杰李莲英和李二棍子'
res = re.findall(pattern, letter)
print(res) # ['李杰', '李莲', '李二']
# . 代表任意字符
# * 重复零次或更多次
pattern = "李.*"
letter = '李杰李莲英和李二棍子'
res = re.findall(pattern, letter)
print(res) # ['李杰李莲英和李二棍子']
# . 代表任意字符
# + 重复一次或更多次
pattern = "李.+"
letter = '李杰李莲英和李二棍子'
res = re.findall(pattern, letter)
print(res) # ['李杰李莲英和李二棍子']
# . 代表任意字符
# {m,n} 重复一次或更多次
pattern = "李.{2,3}"
letter = '李杰李莲英和李二棍子'
res = re.findall(pattern, letter)
print(res) # ['李杰李莲', '李二棍子']
# . 代表任意字符
# [] 字符组中的任意一个
pattern = "李[杰莲英二棍子]"
letter = '李杰李莲英和李二棍子'
res = re.findall(pattern, letter)
print(res) # ['李杰', '李莲', '李二']
# . 代表任意字符
# [] 字符组中的任意一个
# ^和 除了和
# * 任意
pattern = "李[^和]*"
letter = '李杰李莲英和李二棍子'
res = re.findall(pattern, letter)
print(res) # ['李杰李莲英', '李二棍子']
【6】分组匹配
- 身份证号码是一个长度为15或18个字符的字符串
- 如果是15位则全部🈶️数字组成,首位不能为0;
- 如果是18位,则前17位全部是数字,末位可能是数字或x,
- 下面我们尝试用正则来表示:
正则 |
待匹配字符 |
匹配 结果 |
说明 |
^[1-9]\d{13,16}[0-9x]$ |
110101198001017032 |
110101198001017032 |
表示可以匹配一个正确的身份证号 |
^[1-9]\d{13,16}[0-9x]$ |
1101011980010170 |
1101011980010170 |
表示也可以匹配这串数字,但这并不是一个正确的身份证号码,它是一个16位的数字 |
^[1-9]\d{14}(\d{2}[0-9x])?$ |
1101011980010170 |
False |
现在不会匹配错误的身份证号了 ()表示分组,将\d{2}[0-9x]分成一组,就可以整体约束他们出现的次数为0-1次 |
`^([1-9]\d{16}[0-9x] |
[1-9]\d{14})$` |
110105199812067023 |
110105199812067023 |
【7】贪婪匹配
- 贪婪匹配:在满足匹配时,匹配尽可能长的字符串,默认情况下,采用贪婪匹配
- 非贪婪匹配:在满足匹配时,匹配尽可能短的字符串
- 几个常用的非贪婪匹配,Pattern
- *? 重复任意次,但尽可能少重复
- +? 重复1次或更多次,但尽可能少重复
- ?? 重复0次或1次,但尽可能少重复
- {n,m}? 重复n到m次,但尽可能少重复
- {n,}? 重复n次以上,但尽可能少重复
import re
pattern = "李.*?"
pattern = "李.+?"
pattern = "李.??"
pattern = "李.{2,3}?"
letter = '李杰李莲英和李二棍子'
res = re.findall(pattern, letter)
print(res)
# ['李杰李', '李二棍']
【8】方法
(1)findall
name_str = "Chosen yuan Justin"
pattern = 'a'
result = re.findall(pattern, name_str)
print(result)
# 结果 : ['a']
(2)search
# 函数会在字符串内查找模式匹配,只到找到第一个匹配然后返回一个包含匹配信息的对象,该对象可以
# 通过调用group()方法得到匹配的字符串,如果字符串没有匹配,则返回None。
name_str = "Chosen yuan Justin"
pattern = 'a'
result = re.search(pattern, name_str)
print(result) # <re.Match object; span=(9, 10), match='a'>
result = re.search(pattern, name_str).group()
print(result) # 结果 : a
(3)match
name_str = 'abcaaaaaa'
# 同search,不过尽在字符串开始处进行匹配
pattern = 'a'
result = re.match(pattern, 'abc')
print(result) # <re.Match object; span=(0, 1), match='a'>
result = re.match(pattern, 'abc').group()
print(result)
# 结果 : 'a'
(4)split
- 先按'a'分割得到''和'bcd',在对''和'bcd'分别按'b'分割
name_str = 'abcd'
# 先按'a'分割得到''和'bcd',在对''和'bcd'分别按'b'分割
pattern = '[ab]'
result = re.split(pattern, name_str)
print(result) # ['', '', 'cd']
(5)sub
- 默认替换所有匹配到的字符 可以用 count 控制替换次数
name_str = 'eva3egon4yuan4'
# 将数字替换成'H',参数1表示只替换1个
# 默认替换所有匹配到的字符
pattern = '\d'
result = re.sub(pattern, 'H', name_str)
result = re.sub(pattern, 'H', name_str, count=2)
print(result) # evaHegonHyuan4
(6)subn
- 替换匹配到的所有字符并返回 替换成功的字符和替换次数
name_str = 'eva3egon4yuan4'
# 将数字替换成'H',返回元组(替换的结果,替换了多少次)
result = re.subn('\d', 'H', 'eva3egon4yuan4')
print(result) # ('evaHegonHyuanH', 3)
(7)compile
# 将正则表达式编译成为一个 正则表达式对象,规则要匹配的是3个数字
obj = re.compile('\d{3}')
print(obj)
# 正则表达式对象调用search,参数为待匹配的字符串
ret = obj.search('abc1234eeee')
print(ret.group()) # 结果 : 123
print(re.findall(obj, 'abc1234eeee'))
【9】正则方法之优先级
(1)findall的优先级查询
pattern = 'www.(baidu|oldboy).com'
pattern = re.compile(pattern)
data = 'www.oldboy.com'
ret = re.findall(pattern, data)
# 这是因为findall会优先把匹配结果组里内容返回,如果想要匹配结果,取消权限即可
print(ret) # ['oldboy']
ret = re.findall('www.(?:baidu|oldboy).com', 'www.baidu.com')
print(ret) # ['www.oldboy.com']
data= "<dd>弱者声嘶力竭,亦无人在乎,强者轻声细语,却能深入人心。一棵熊熊燃烧的天赋树,每一片叶子都承载着不同的灵纹,宗门被灭,沦为矿奴的陆叶凭此成为修士,搅动九州风云......</dd>"
pattern = '<dd>(.*)</dd>'
pattern = re.compile(pattern)
print(re.findall(pattern,data)) # ['弱者声嘶力竭,亦无人在乎,强者轻声细语,却能深入人心。一棵熊熊燃烧的天赋树,每一片叶子都承载着不同的灵纹,宗门被灭,沦为矿奴的陆叶凭此成为修士,搅动九州风云......']
(2)split的优先级查询
ret=re.split("\d+","eva3egon4yuan")
print(ret) #结果 : ['eva', 'egon', 'yuan']
ret=re.split("(\d+)","eva3egon4yuan")
print(ret) #结果 : ['eva', '3', 'egon', '4', 'yuan']
- 在匹配部分加上()之后所切出的结果是不同的,
- 没有()的没有保留所匹配的项,但是有()的却能够保留了匹配的项,
- 这个在某些需要保留匹配部分的使用过程是非常重要的。
【10】常用正则表达式
场景 |
正则表达式 |
用户名 |
^[a-z0-9_-]{3,16}$ |
密码 |
^[a-z0-9_-]{6,18}$ |
手机号码 |
^(?:\+86)?1[3-9]\d{9}$ |
颜色的十六进制值 |
^#?([a-f0-9] |
电子邮箱 |
^[a-z\d]+(\.[a-z\d]+)*@([\da-z](-[\da-z])?)+\.[a-z]+$ |
URL |
^(?:https:// |
IP 地址 |
((2[0-4]\d |
HTML 标签 |
^<([a-z]+)([^<]+)*(?:>(.*)<\/\1> |
utf-8编码下的汉字范围 |
^[\u2E80-\u9FFF]+$ |