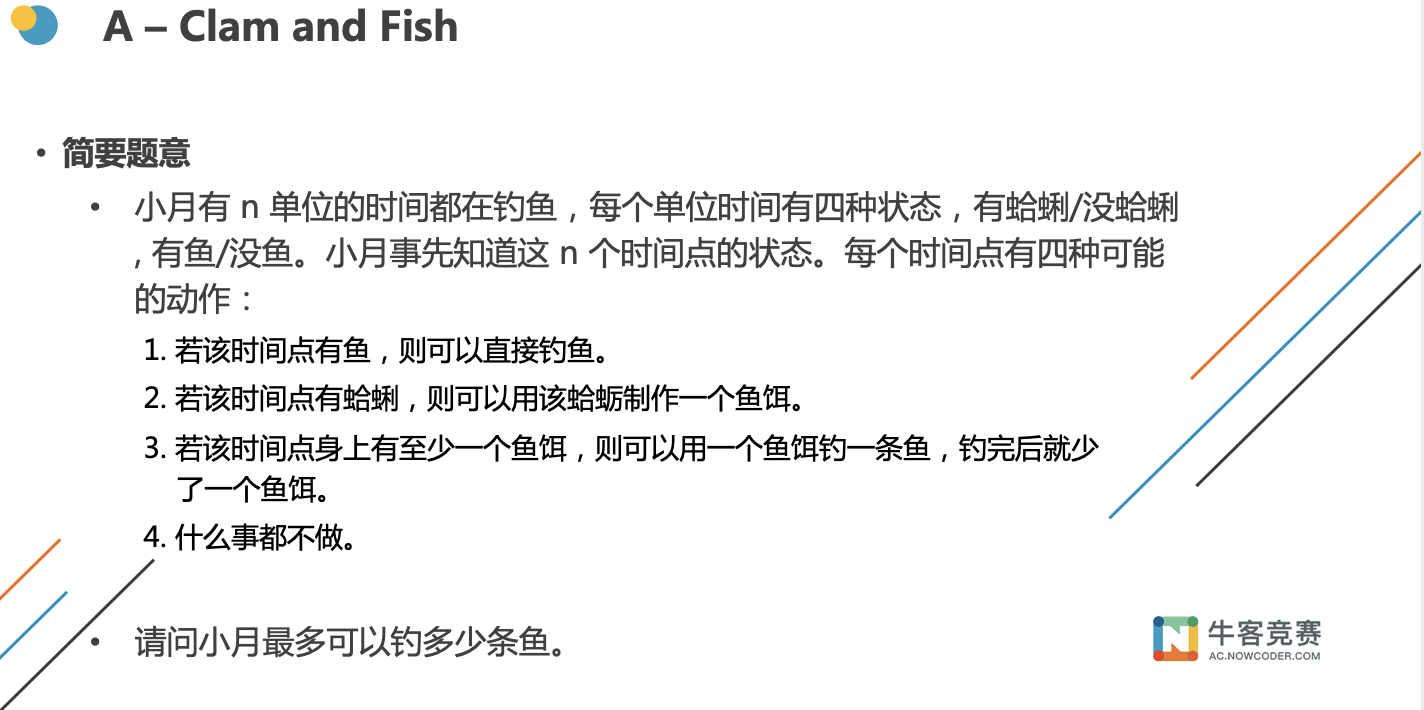
- 记录答案为ans,很明显当为3、4的时候,捕鱼即可,ans++,当为0的时候,
判断是否有做好的鱼饵,有鱼饵的话直接钓鱼,ans++,此时鱼饵数也对应减一,
为1时,有蛤,故做一个鱼饵,鱼饵数+1。
#include <stdio.h>
#include <string.h>
#include <iostream>
#include <algorithm>
#include <vector>
#include <queue>
#include <set>
#include <map>
#include <list>
#include <map>
#include <string>
#include <stack>
#include <cmath>
#include <stdlib.h>
#include <time.h>
#include <iomanip>
#define S(X) scanf("%d",&(X))
#define SS(X, Y) scanf("%d%d",&(X),&(Y))
#define SSS(X,Y,Z) scanf("%d%d%d",&(X),&(Y),&(Z))
#define SL(X) scanf("%lld",&(X))
#define SLL(X, Y) scanf("%lld%lld",&(X),&(Y))
using namespace std;
typedef double db;
typedef long long ll;
const int mod = 998244353;
const int maxn=2e6+100;
const double pi=acos(-1);
int main()
{
#ifdef ONLINE_JUDGE
#else
freopen(".vscode/in.txt","r",stdin);
#endif
int t;
S(t);
while(t--)
{
int n;
S(n);
char s[maxn];
scanf("%s",s);
int er=0,ans=0,make=0;;
for(int i=0;i<n;i++)
{
if(s[i]=='0')
{
if(make)
{
ans++;make--;
}
}
else if(s[i]=='1')
{
make++;
}
else
ans++;
// cout<<"i="<<i<<" er="<<er<<" make="<<make<<" ans="<<ans<<endl;
}
printf("%d\n",ans+make/2);
}
return 0;
}
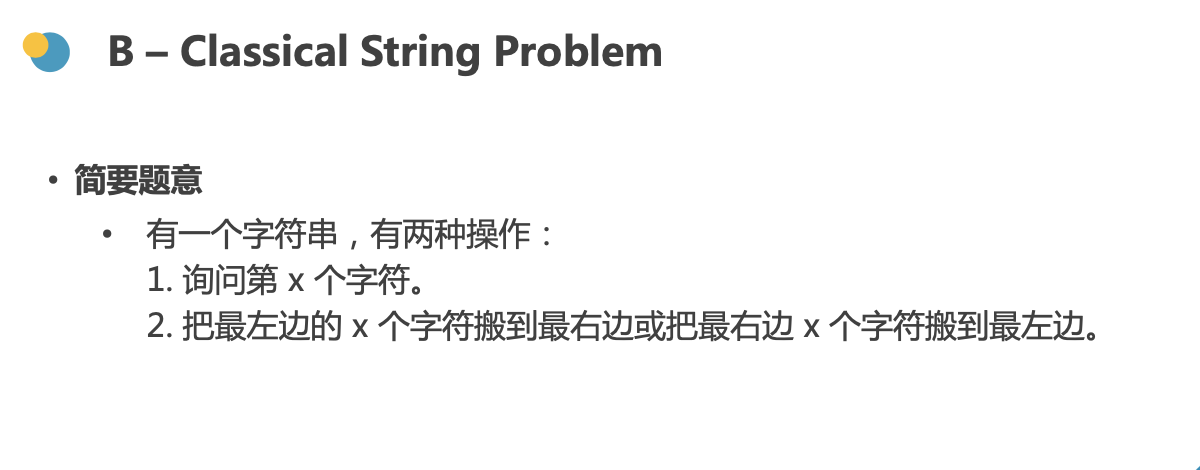
- 这个直接将字符串首尾相连组成一个环即可,我们可以定义一个指针ops,这个指针就是更改后的字符串的初始位置,令初始值为0,当我们查询字符串x位置的字符时输出str[(ops+x-1+len)%len]即可,当x>0时,我们将指针顺时针走x个,即将一开始的字符串的初始位置+x,这样前x个就到字符串后面去了,这个位置就是更改后的字符串位置,因为可能会超过len的长度,因此要(ops+x+len)%len,当x为负的时候,同理ops也要加上x,因为x是负的,直接加即可,又因为可能结果小于0因此(ops+x+len)%len,这两个直接合并就好。
#include <stdio.h>
#include <string.h>
#include <iostream>
#include <algorithm>
#include <vector>
#include <queue>
#include <set>
#include <map>
#include <list>
#include <map>
#include <string>
#include <stack>
#include <math.h>
#include <stdlib.h>
#include <time.h>
#define S(X) scanf("%d",&(X))
#define SS(X, Y) scanf("%d%d",&(X),&(Y))
#define SSS(X,Y,Z) scanf("%d%d%d",&(X),&(Y),&(Z))
#define SL(X) scanf("%lld",&(X))
#define SLL(X, Y) scanf("%lld%lld",&(X),&(Y))
using namespace std;
typedef double db;
typedef long long ll;
const int maxn=2e6+100;
const double eps=1e-5;
using namespace std;
int main()
{
#ifdef ONLINE_JUDGE
#else
freopen(".vscode/in.txt","r",stdin);
#endif
char str[maxn];
scanf("%s",str);
getchar();
int len=strlen(str);
int q;
S(q);
char ch[5];
int x;
int ops=0;
while(q--)
{
scanf("%s%d",ch,&x);
//cout<<ch<<" "<<x<<endl;
if(ch[0]=='A')
{
//cout<<"ops="<<ops<<" "<<(ops+x-1+len)%len<<endl;
printf("%c\n",str[(ops+x-1+len)%len]);
}
else
{
//if(x>0)
ops=(ops+x+len)%len;
//else
// ops=(ops-x+len)%len;
//cout<<"ops="<<ops<<endl;
}
}
return 0;
}
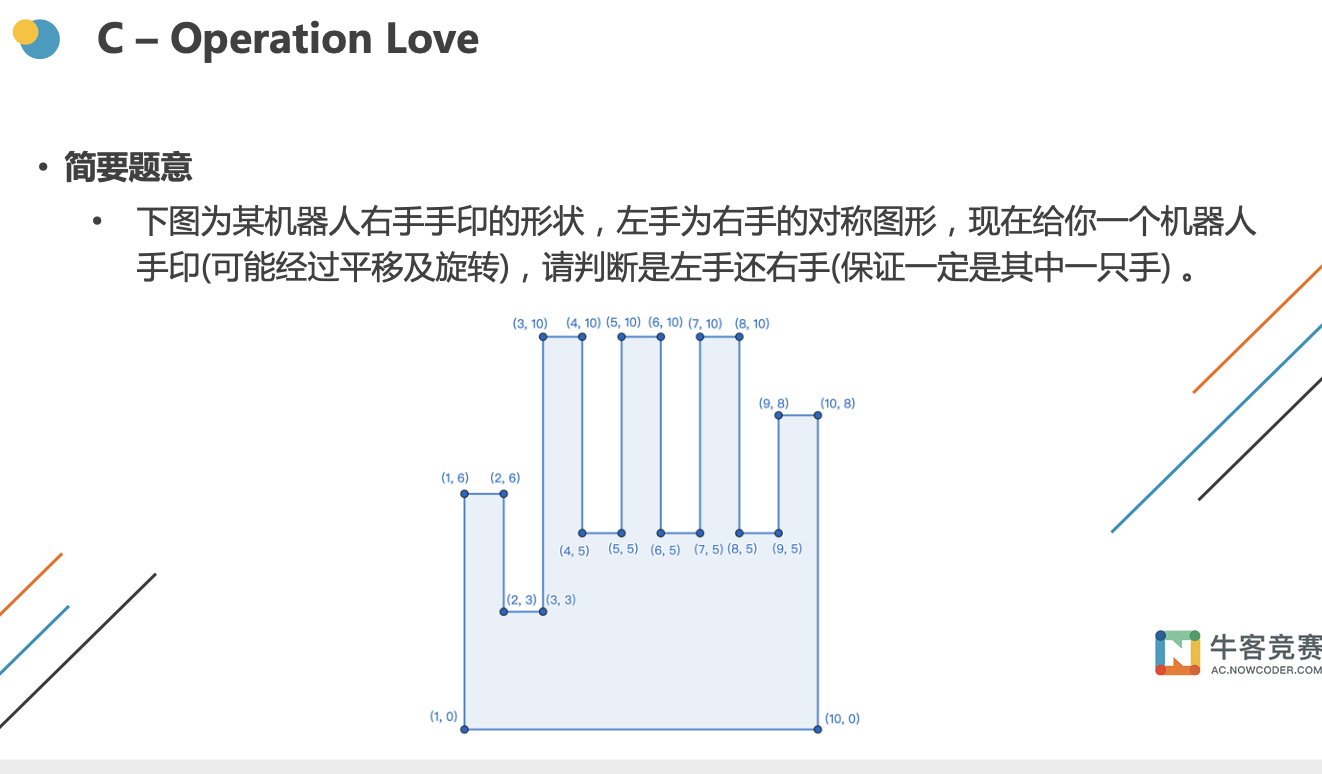
- 这题呢,找到长度为6和长度为9的两个相临边即可,记录这三个点组成的长度为6(端点为sp,op)和9(端点为op,ep)的两个边,然后按照sp,op,ep的顺序求叉乘即可,大于0就说明短边向长边逆时针旋转左手,小于0说明顺时针旋转右手。一开始设的eps为1e-8,然后改了各种方法结果发现是eps的问题,改了1e5就过了,血亏。。
#include <stdio.h>
#include <string.h>
#include <iostream>
#include <algorithm>
#include <vector>
#include <queue>
#include <set>
#include <map>
#include <list>
#include <map>
#include <string>
#include <stack>
#include <math.h>
#include <stdlib.h>
#include <time.h>
#define S(X) scanf("%d",&(X))
#define SS(X, Y) scanf("%d%d",&(X),&(Y))
#define SSS(X,Y,Z) scanf("%d%d%d",&(X),&(Y),&(Z))
#define SL(X) scanf("%lld",&(X))
#define SLL(X, Y) scanf("%lld%lld",&(X),&(Y))
using namespace std;
typedef double db;
typedef long long ll;
const int maxn=2e5+100;
const double eps=1e-5;
using namespace std;
struct Point{
long double x,y;
Point(long double _x=0,long double _y=0){
x=_x;
y=_y;
}
Point operator-(const Point& p1)const{return Point(x-p1.x,y-p1.y);}
Point operator+(const Point& p1)const{return Point(x+p1.x,y+p1.y);}
bool friend operator<(const Point& p1,const Point& p2){
if(fabs(p1.x-p2.x)>eps)return p1.x<p2.x;return p1.y<p2.y;
}//map点的时候
bool friend operator==(const Point& p1,const Point& p2){
if(fabs(p1.x-p2.x)>eps)return 0;
if(fabs(p1.y-p2.y)>eps)return 0;
return 1;
}
long double friend operator^(const Point& p1,const Point& p2){return p1.x*p2.y-p2.x*p1.y;}//共线的时候
Point friend operator*(const Point& p1,const double& k){return Point(p1.x*k,p1.y*k);}
long double friend operator*(const Point& p1,const Point& p2){return p1.x*p2.x+p1.y*p2.y;}
};
long double dis(Point a,Point b)
{
return sqrt((a.x-b.x)*(a.x-b.x)+(a.y-b.y)*(a.y-b.y));
}
long double Cross(Point &sp, Point &ep, Point &op)
{
return ((sp.x-op.x)*(ep.y-op.y)-(ep.x-op.x)*(sp.y-op.y));
}
long double Cross1(Point A, Point B){
return (A.x*B.y-A.y*B.x);
}
long double Area(Point* p, int n){
long double s = 0;
for(int i = 1; i < n-1; ++i)
s += Cross1(p[i]-p[0], p[i+1]-p[0]);
return s;
}
int main()
{
#ifdef ONLINE_JUDGE
#else
freopen(".vscode/in.txt","r",stdin);
#endif
int n;
S(n);
while(n--)
{
Point p[100];
Point a,b,c,d;
Point sp,op,ep;
for(int i=0;i<20;i++)
{
scanf("%Lf%Lf",&p[i].x,&p[i].y);
if(i!=0)
{
long double len=dis(p[i],p[i-1]);
//cout<<"len="<<len<<endl;
if(fabs(len-9.0)<eps)
{
a=p[i];b=p[i-1];
}
else if(fabs(len-6.0)<eps)
{
c=p[i];
d=p[i-1];
}
}
}
long double len1=dis(p[0],p[19]);
// cout<<"len1="<<len1<<endl;
if(fabs(len1-9.0)<eps)
{
//cout<<"haha"<<endl;
a=p[0];
b=p[19];
}
else if(fabs(len1-6.0)<eps)
{
//cout<<"haha"<<endl;
c=p[0];
d=p[19];
}
// cout<<"a= ";
// cout<<a.x<<" "<<a.y<<endl;
// cout<<"b= ";
// cout<<b.x<<" "<<b.y<<endl;
// cout<<"c= ";
// cout<<c.x<<" "<<c.y<<endl;
// cout<<"d= ";
// cout<<d.x<<" "<<d.y<<endl;
if(a==c)
{
op=a;
sp=d;
ep=b;
}
else if(a==d)
{
op=a;
sp=c;
ep=b;
}
else if(b==c)
{
op=b;
sp=d;
ep=a;
}
else if(b==d)
{
op=b;
sp=c;
ep=a;
}
//cout<<sp.x<<" "<<sp.y<<endl;
//cout<<op.x<<" "<<op.y<<endl;
// cout<<ep.x<<" "<<ep.y<<endl;
// cout<<"Cross=";
// cout<<Cross(sp,ep,op)<<endl;
// Point pp[4];
// pp[0]=sp,pp[1]=op,pp[2]=ep;
// cout<<"Area="<<Area(pp,3)<<endl;
if(Cross(sp,ep,op)>0)
printf("left\n");
else
printf("right\n");
}
return 0;
}

- 签到题,设定一个字符串ans="lovely",然后只要比较前6个字符即可,是否为ans[i]或者toupper(ans[i])即可。
#include <stdio.h>
#include <string.h>
#include <iostream>
#include <algorithm>
#include <vector>
#include <queue>
#include <set>
#include <map>
#include <list>
#include <map>
#include <string>
#include <stack>
#include <cmath>
#include <stdlib.h>
#include <time.h>
#include <iomanip>
#define S(X) scanf("%d",&(X))
#define SS(X, Y) scanf("%d%d",&(X),&(Y))
#define SSS(X,Y,Z) scanf("%d%d%d",&(X),&(Y),&(Z))
#define SL(X) scanf("%lld",&(X))
#define SLL(X, Y) scanf("%lld%lld",&(X),&(Y))
using namespace std;
typedef double db;
typedef long long ll;
const int mod = 998244353;
const int maxn=5e5+100;
const double pi=acos(-1);
int main()
{
#ifdef ONLINE_JUDGE
#else
freopen(".vscode/in.txt","r",stdin);
#endif
string s;
cin>>s;
int len=s.length();
string ss="lovely";
bool flag=false;
for(int i=0;i<6;i++)
{
if((s[i]==ss[i])||(s[i]==toupper(ss[i])))
continue;
else
{
flag=true;
break;
}
}
if(flag)
printf("ugly\n");
else
{
printf("lovely\n");
}
return 0;
}