组合模式(Composite Pattern)
组合模式主要处理的是客户在使用组合对象时对其结构的依赖。组合对象内部解决自身的复杂结构,使客户代码就像使用简单对象一样使用组合对象。
组合模式又分为安全式的组合模式和透明式的组合模式。安全式的组合模式要求管理聚集的方法只出现在组合对象中,而不出现在简单对象中。透明式的组合模式要求所有的对象,不论组合对象还是简单对象,均符合一个固定的接口。
下例中模拟ASP.NET中的button和panel控件,使用的是安全式的组合模式,类与结构图的对应关系是:
IControl = Component
Button = Leaf
Panel = Composite
图1:安全式的组合模式
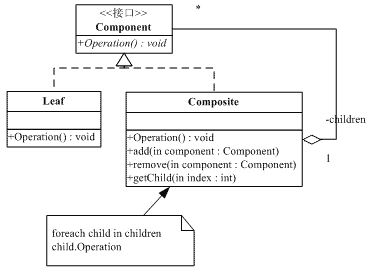
图2:透明式的组合模式
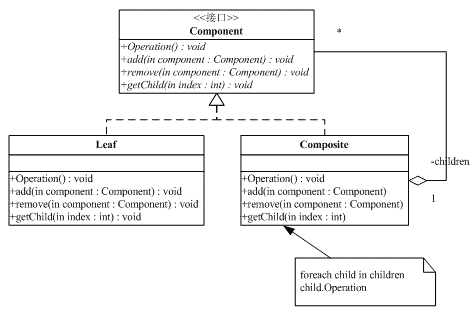
组合模式又分为安全式的组合模式和透明式的组合模式。安全式的组合模式要求管理聚集的方法只出现在组合对象中,而不出现在简单对象中。透明式的组合模式要求所有的对象,不论组合对象还是简单对象,均符合一个固定的接口。
下例中模拟ASP.NET中的button和panel控件,使用的是安全式的组合模式,类与结构图的对应关系是:
IControl = Component
Button = Leaf
Panel = Composite
图1:安全式的组合模式
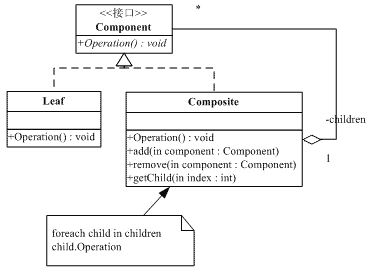
图2:透明式的组合模式
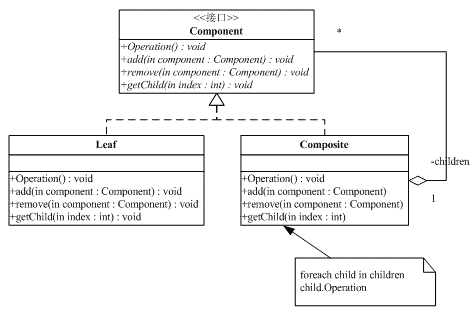
public class App
{
public static void Main()
{
IControl button1 = new Button();
Panel panel1 = new Panel();
panel1.Add(button1);
Panel panel2 = new Panel();
panel2.Add(panel1);
//如果没有组合模式,这里就要很繁琐的(遍历每个控件)调用Initialize方法了
panel2.Initialize();
Console.ReadLine();
}
}
public interface IControl
{
void Initialize();
}
public class Button : IControl
{
public void Initialize()
{
Console.WriteLine("初始化Button控件");
}
}
public class Panel : IControl
{
List<IControl> controlList;
public Panel()
{
controlList = new List<IControl>();
}
public void Add(IControl control)
{
controlList.Add(control);
}
public void Remove(IControl control)
{
if (controlList == null)
{
throw new InvalidOperationException("控件列表为空");
}
controlList.Remove(control);
}
public void Initialize()
{
Console.WriteLine("初始化Panel控件");
foreach (var control in controlList)
{
control.Initialize();
}
}
}
{
public static void Main()
{
IControl button1 = new Button();
Panel panel1 = new Panel();
panel1.Add(button1);
Panel panel2 = new Panel();
panel2.Add(panel1);
//如果没有组合模式,这里就要很繁琐的(遍历每个控件)调用Initialize方法了
panel2.Initialize();
Console.ReadLine();
}
}
public interface IControl
{
void Initialize();
}
public class Button : IControl
{
public void Initialize()
{
Console.WriteLine("初始化Button控件");
}
}
public class Panel : IControl
{
List<IControl> controlList;
public Panel()
{
controlList = new List<IControl>();
}
public void Add(IControl control)
{
controlList.Add(control);
}
public void Remove(IControl control)
{
if (controlList == null)
{
throw new InvalidOperationException("控件列表为空");
}
controlList.Remove(control);
}
public void Initialize()
{
Console.WriteLine("初始化Panel控件");
foreach (var control in controlList)
{
control.Initialize();
}
}
}