实验6
task1_1
1 #include <stdio.h> 2 #include<stdlib.h> 3 #define N 4 4 int main() 5 { 6 int x[N] = {1, 9, 8, 4}; 7 int i; 8 int *p; 9 // 方式1:通过数组名和下标遍历输出数组元素 10 11 for(i=0; i<N; ++i) 12 printf("%d", x[i]); 13 printf("\n"); 14 // 方式2:通过指针变量遍历输出数组元素 (写法1) 15 16 for(p=x; p<x+N; ++p) 17 printf("%d", *p); 18 printf("\n"); 19 // 方式2:通过指针变量遍历输出数组元素(写法2) 20 p = x; 21 22 for(i=0; i<N; ++i) 23 printf("%d", *(p+i)); 24 printf("\n"); 25 // 方式2:通过指针变量遍历输出数组元素(写法3) 26 p = x; 27 28 for(i=0; i<N; ++i) 29 printf("%d", p[i]); 30 printf("\n"); 31 32 system("PAUSE"); 33 return 0; 34 }
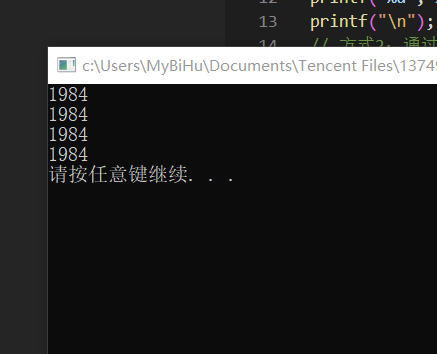
task1_2
1 #include <stdio.h> 2 #include<stdlib.h> 3 #define N 4 4 int main() 5 { 6 char x[N] = {'1', '9', '8', '4'}; 7 int i; 8 char *p; 9 10 // 方式1:通过数组名和下标遍历输出数组元素 11 for(i=0; i<N; ++i) 12 printf("%c", x[i]); 13 printf("\n"); 14 15 // 方式2:通过指针变量遍历输出数组元素 (写法1) 16 for(p=x; p<x+N; ++p) 17 printf("%c", *p); 18 printf("\n"); 19 20 // 方式2:通过指针变量遍历输出数组元素(写法2) 21 p = x; 22 for(i=0; i<N; ++i) 23 printf("%c", *(p+i)); 24 printf("\n"); 25 26 // 方式2:通过指针变量遍历输出数组元素(写法3) 27 p = x; 28 for(i=0; i<N; ++i) 29 printf("%c", p[i]); 30 printf("\n"); 31 32 system("PAUSE"); 33 return 0; 34 }
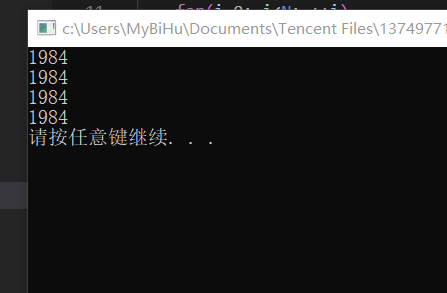
问题1:2004
问题2:2002
因为int型一个数据占用4个字节,而char型一个数据占用2个字节
task2_1
1 #include <stdio.h> 2 #include<stdlib.h> 3 4 int main() 5 { 6 int x[2][4] = { {1,9,8,4}, {2,0,2,2}} ; 7 int i, j; 8 int *p; // 指针变量,存放int类型数据的地址 9 int (*q)[4]; // 指针变量,指向包含4个int型元素的一维数组 10 11 // 使用数组名、下标访问二维数组元素 12 for(i=0; i<2; ++i) 13 { 14 for(j=0; j<4; ++j) 15 printf("%d", x[i][j]); 16 printf("\n"); 17 } 18 19 // 使用指针变量p间接访问二维数组元素 20 for(p = &x[0][0], i = 0; p < &x[0][0] + 8; ++p, ++i) 21 { 22 printf("%d", *p); 23 if( (i+1)%4 == 0) 24 printf("\n"); 25 } 26 27 // 使用指针变量q间接访问二维数组元素 28 for(q=x; q<x+2; ++q) 29 { 30 for(j=0; j<4; ++j) 31 printf("%d", *(*q+j)); 32 printf("\n"); 33 } 34 35 system("PAUSE"); 36 return 0; 37 }
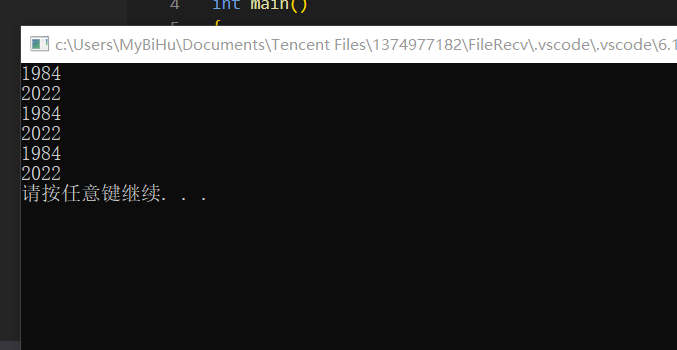
task2_2
1 #include <stdio.h> 2 #include<stdlib.h> 3 int main() 4 { 5 char x[2][4] = { {'1', '9', '8', '4'}, {'2', '0', '2', '2'} }; 6 int i, j; 7 char *p; // 指针变量,存放char类型数据的地址 8 char (*q)[4]; // 指针变量,指向包含4个char型元素的一维数组 9 10 // 使用数组名、下标访问二维数组元素 11 for(i=0; i<2; ++i) 12 { 13 for(j=0; j<4; ++j) 14 printf("%c", x[i][j]); 15 printf("\n"); 16 } 17 18 // 使用指针变量p间接访问二维数组元素 19 for(p = &x[0][0], i = 0; p < &x[0][0] + 8; ++p, ++i) 20 { 21 printf("%c", *p); 22 if( (i+1)%4 == 0) 23 printf("\n"); 24 } 25 26 // 使用指针变量q间接访问二维数组元素 27 for(q=x; q<x+2; ++q) 28 { 29 for(j=0; j<4; ++j) 30 printf("%c", *(*q+j)); 31 printf("\n"); 32 } 33 system("PAUSE"); 34 return 0; 35 }
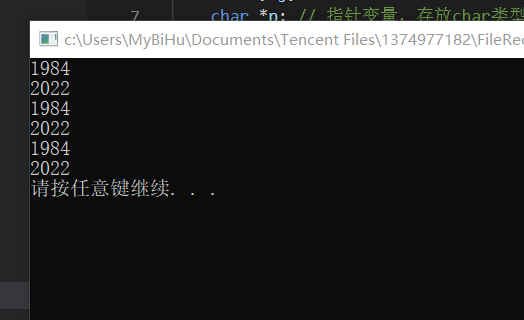
程序task2_1.c中: 假设指针变量p初始值是地址2000,则执行++p后,指针变量p中存放的地址值是多少?
2004
假设指针变量q初始值是地址2000,则执行++q后,指针变量q中存放的地址值是多少?
2016
程序task1_2.c中: 假设指针变量p初始值是地址2000,则执行++p后,指针变量p中存放的地址值是多少?
2002
假设指针变量q初始值是地址2000,则执行++q后,指针变量q中存放的地址值是多少?
2008
都是对指针变量做++操作,为什么结果有差别?
task3_1
1 #include <stdio.h> 2 #include <string.h> 3 #include<stdlib.h> 4 #define N 80 5 6 int main() 7 { 8 char s1[] = "C, I love u."; 9 char s2[] = "C, I hate u."; 10 char tmp[N]; 11 12 printf("sizeof(s1) vs. strlen(s1): \n"); 13 printf("sizeof(s1) = %d\n", sizeof(s1)); 14 printf("strlen(s1) = %d\n", strlen(s1)); 15 16 printf("\nbefore swap: \n"); 17 printf("s1: %s\n", s1); 18 printf("s2: %s\n", s2); 19 20 printf("\nswapping...\n"); 21 strcpy(tmp, s1); 22 strcpy(s1, s2); 23 strcpy(s2, tmp); 24 25 printf("\nafter swap: \n"); 26 printf("s1: %s\n", s1); 27 printf("s2: %s\n", s2); 28 29 system("PAUSE"); 30 return 0; 31 }
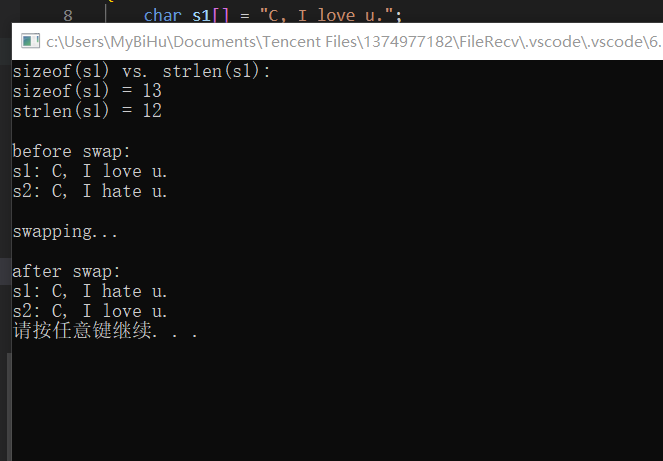
问题1:数组s1的大小是多少?sizeof(s1)计算的是什么?strlen(s1)统计的是什么?
12;数组总长度;数组除‘/0’以外的长度
问题2:line7代码,能否替换成以下写法?不能
问题3:line20-22执行后,字符数组s1和s2中的内容是否交换?
task3_2
1 #include <stdio.h> 2 #include <string.h> 3 #include<stdlib.h> 4 #define N 80 5 6 int main() 7 { 8 char *s1 = "C, I love u."; 9 char *s2 = "C, I hate u."; 10 char *tmp; 11 12 printf("sizeof(s1) vs. strlen(s1): \n"); 13 printf("sizeof(s1) = %d\n", sizeof(s1)); 14 printf("strlen(s1) = %d\n", strlen(s1)); 15 16 printf("\nbefore swap: \n"); 17 printf("s1: %s\n", s1); 18 printf("s2: %s\n", s2); 19 printf("\nswapping...\n"); 20 21 tmp = s1; 22 s1 = s2; 23 s2 = tmp; 24 25 printf("\nafter swap: \n"); 26 printf("s1: %s\n", s1); 27 printf("s2: %s\n", s2); 28 29 system("PAUSE"); 30 return 0; 31 }
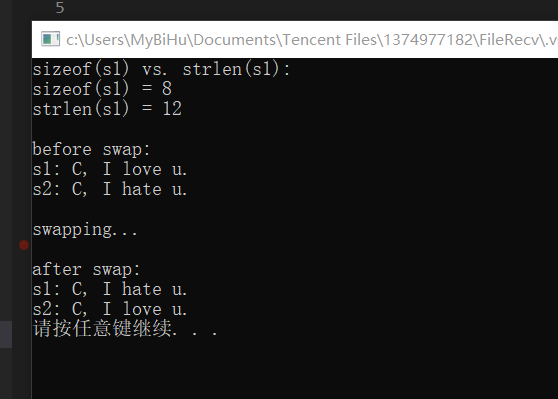
问题1:指针变量s1中存放的是什么?sizeof(s1)计算的是什么?strlen(s1)统计的是什么?
问题2:line7代码能否替换成下面的写法?
问题3:line20-line22,交换的是什么?字符串常量"C, I love u."和字符串常量"C, I hate u."在内存 存储单元中有没有交换?
task4
1 #include <stdio.h> 2 #include <string.h> 3 #include<stdlib.h> 4 #define N 5 5 int check_id(char *str); // 函数声明 6 7 int main() 8 { 9 char *pid[N] = {"31010120000721656X", 10 "330106199609203301", 11 "53010220051126571", 12 "510104199211197977", 13 "53010220051126133Y"}; 14 int i; 15 for(i=0; i<N; ++i) 16 if( check_id(pid[i]) ) // 函数调用 17 printf("%s\tTrue\n", pid[i]); 18 else 19 printf("%s\tFalse\n", pid[i]); 20 system("PAUSE"); 21 return 0; 22 } 23 // 函数定义 24 // 功能: 检查指针str指向的身份证号码串形式上是否合法。 25 // 形式合法,返回1,否则,返回0 26 int check_id(char *str) 27 { 28 int a,b,i; 29 int *p; 30 31 if(strlen(str) == 18) 32 a = 1; 33 else a = 0; 34 35 for(i = 0; i < strlen(str); ++i) 36 { 37 if(*(str +i) >= '0' && *(str +i ) <= '9' || *(str +i) == 'X') 38 b=1; 39 else 40 b= 0;} 41 return a*b; 42 }
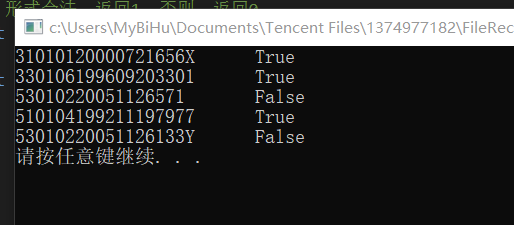
task5
1 #include <stdio.h> 2 #include <string.h> 3 #include<stdlib.h> 4 #define N 80 5 int is_palindrome(char *s); // 函数声明 6 7 int main() 8 { 9 char str[N]; 10 int flag; 11 printf("Enter a string:\n"); 12 gets(str); 13 flag = is_palindrome(str); // 函数调用 14 if (flag) 15 printf("YES\n"); 16 else 17 printf("NO\n"); 18 system("PAUSE"); 19 return 0; 20 } 21 // 函数定义 22 // 功能:判断s指向的字符串是否是回文串 23 // 如果是,返回1;否则,返回0 24 int is_palindrome(char *s) 25 { 26 int i, a; 27 a = strlen(s); 28 29 30 for(i = 0; i < a / 2; i++) 31 { 32 if(s[i] != s[a-1-i]) 33 return 0; 34 } 35 }
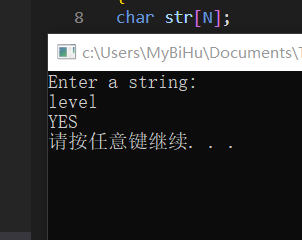
task6
1 #include <stdio.h> 2 #include<stdlib.h> 3 #define N 80 4 void encoder(char *s); // 函数声明 5 void decoder(char *s); // 函数声明 6 7 int main() 8 { 9 char words[N]; 10 printf("输入英文文本: "); 11 gets(words); 12 13 printf("编码后的英文文本: "); 14 encoder(words); // 函数调用 15 printf("%s\n", words); 16 17 printf("对编码后的英文文本解码: "); 18 decoder(words); // 函数调用 19 printf("%s\n", words); 20 21 system("PAUSE"); 22 return 0; 23 } 24 /*函数定义 25 功能:对s指向的字符串进行编码处理 26 编码规则: 27 对于a~z或A~Z之间的字母字符,用其后的字符替换; 其中,z用a替换,Z用A替换 28 其它非字母字符,保持不变 29 */ 30 void encoder(char *s) 31 { 32 int i,a=0; 33 while(s[a]) a++; 34 for(i = 0; i < a; i++) 35 { 36 if(s[i] == 'z') s[i] = 'a'; 37 if(s[i] == 'Z') s[i] = 'A'; 38 if((s[i] > 'a' && s[i] < 'z')||(s[i] > 'A' && s[i] < 'Z')) 39 s[i] = s[i] + 1; 40 41 } 42 } 43 /*函数定义 44 功能:对s指向的字符串进行解码处理 45 解码规则: 46 对于a~z或A~Z之间的字母字符,用其前面的字符替换; 其中,a用z替换,A用Z替换 47 其它非字母字符,保持不变 48 */ 49 void decoder(char *s) 50 { 51 int i, a = 0; 52 while(s[a]) a++; 53 54 for(i=0; i < a; i++) 55 { 56 if(s[i] == 'a') s[i] = 'z'; 57 if(s[i] == 'A') s[i] = 'Z'; 58 if((s[i] >= 'a'&&s[i] <= 'z')||(s[i] >= 'A'&&s[i] <= 'Z')) 59 s[i] = s[i] - 1; 60 } 61 }
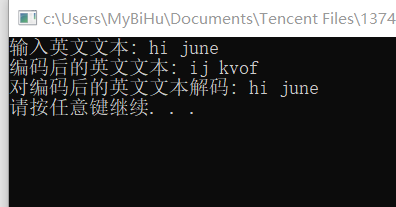