实验3
task1
1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <time.h> 4 #include <windows.h> 5 #define N 80 6 7 void printText(int line, int col, char text[]); // 函数声明 8 void printSpaces(int n); // 函数声明 9 void printBlankLines(int n); // 函数声明 10 11 int main() 12 { 13 int line, col, i; 14 char text[N] ="hi, May~"; 15 16 srand(time(0)); // 以当前系统时间作为随机种子 17 18 for(i=1; i<=10; ++i) 19 { 20 line=rand()%25; 21 col=rand()%80; 22 printText(line, col, text); 23 Sleep(1000); // 暂停1000ms 24 } 25 system("PAUSE"); 26 return 0; 27 } 28 29 // 打印n个空格 30 void printSpaces(int n) 31 { 32 int i; 33 34 for(i=1; i<= n; ++i) 35 printf(" "); 36 } 37 38 // 打印n行空白行 39 void printBlankLines(int n) 40 { 41 int i; 42 43 for(i=1; i<=n; ++i) 44 printf("\n"); 45 } 46 47 // 在第line行第col列打印一段文本 48 void printText(int line, int col, char text[]) 49 { 50 printBlankLines(line-1); // 打印n-1行空行 51 printSpaces(col-1);// 打印n-1列空格 52 printf("%s", text); 53 }
把某段文字如"hi, May~"打印在某行某列
task2_1
1 // 利用局部static变量的特性,计算阶乘 2 3 #include <stdio.h> 4 #include<stdlib.h> 5 long long fac(int n); // 函数声明 6 7 int main() 8 { 9 int i, n; 10 11 printf("Enter n: "); 12 scanf("%d", &n); 13 14 for (i=1; i<=n; ++i) 15 printf("%d! = %lld\n", i, fac(i)); 16 system("PAUSE"); 17 return 0; 18 } 19 20 // 函数定义 21 long long fac(int n) 22 { 23 static long long p = 1; 24 printf("p = %lld\n", p); 25 p = p * n; 26 27 return p; 28 }
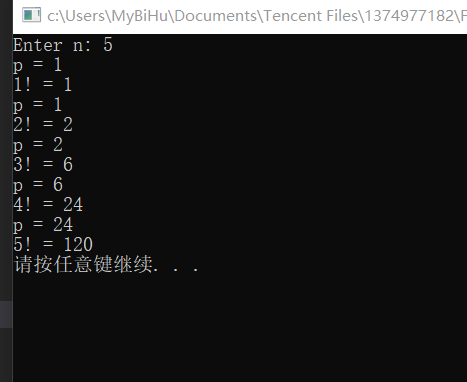
task2_2
1 // 练习:局部static变量特性 2 #include <stdio.h> 3 #include<stdlib.h> 4 int func(int, int);// 函数声明 5 int main() 6 { 7 int k = 4, m = 1, p1, p2; 8 p1 = func(k, m);// 函数调用 9 p2 = func(k, m);// 函数调用 10 printf("%d,%d\n", p1, p2); 11 system("PAUSE"); 12 return 0; 13 } 14 // 函数定义 15 int func(int a, int b) 16 { 17 static int m=0, i=2; 18 i += m + 1; 19 m = i + a + b; 20 return m; 21 } // static使得变量返回不再为初始值,即为变换后的值
task3
1 #include <stdio.h> 2 #include<stdlib.h> 3 long long fun(int n); // 函数声明 4 5 int main() 6 { 7 int n; 8 long long f; 9 while (scanf("%d", &n) !=EOF) 10 { 11 f = fun(n); // 函数调用 12 printf("n = %d, f = %lld\n", n, f); 13 } 14 system("PAUSE"); 15 return 0; 16 } 17 long long fun(int n)// 函数定义 18 { 19 int i; 20 long long x = 1; 21 if(n != 0) 22 { 23 for(i = 0; i < n; i++) 24 { 25 x *= 2; 26 27 } 28 } 29 x -= 1; 30 return x; 31 }
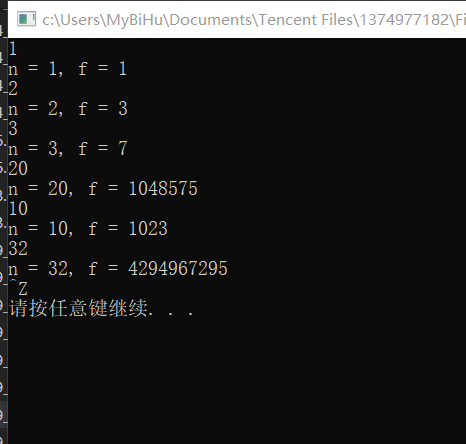
task4
1 #include<stdio.h> 2 #include<stdlib.h> 3 #include<math.h> 4 5 void hanoi(unsigned int n, char from, char temp, char to); 6 void moveplate(unsigned int n, char from, char to); 7 int times(int n); 8 9 int main() 10 { 11 unsigned int n; 12 while (scanf("%u", &n) !=EOF) 13 { 14 hanoi(n, 'A', 'B', 'C'); 15 printf("\n"); 16 printf("一共移动了%d次.", times(n)); 17 printf("\n"); 18 } 19 system("PAUSE"); 20 return 0; 21 } 22 void hanoi(unsigned int n, char from, char temp, char to) 23 { 24 if(n == 1) 25 moveplate(n, from, to); 26 else 27 { 28 hanoi(n - 1, from, to, temp); 29 moveplate(n, from, to); 30 hanoi(n - 1, temp, from, to); 31 32 } 33 } 34 void moveplate(unsigned int n, char from, char to) 35 { 36 printf("%u:%c-->%c\n", n, from, to); 37 } 38 int times(int n) 39 { 40 static int x = 1; 41 x += pow(2, n - 1); 42 return x; 43 }
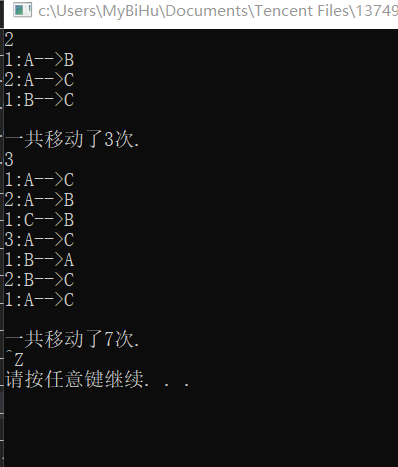
task5
1 #include<stdio.h> 2 #include<stdlib.h> 3 #include<math.h> 4 int is_prime(int x); 5 6 int main() 7 { 8 int i, j; 9 for(j = 4; j <= 20; j++) 10 { 11 if(j % 2 == 0) 12 for(i = 2; ;i++) 13 { 14 if(is_prime(i) && is_prime(j-i)) 15 { 16 printf("%d = %d + %d\n", j, i, j - i); 17 break; 18 } 19 } 20 } 21 22 system("PAUSE"); 23 return 0; 24 } 25 26 int is_prime(int x) //函数定义 27 { 28 int i; 29 for(i = 2; i <= sqrt(x); i++) 30 { 31 if(x % i == 0) 32 return 0; 33 } 34 return 1; 35 }
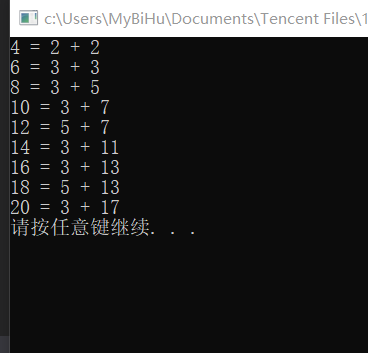
task6
1 #include <stdio.h> 2 #include<stdlib.h> 3 #include<math.h> 4 int length(long num); 5 long fun(long s); // 函数声明 6 7 int main() 8 { 9 long s, t; 10 printf("Enter a number: "); 11 while (scanf("%ld", &s) !=EOF) 12 { 13 t = fun(s); // 函数调用 14 printf("new number is: %ld\n\n", t); 15 printf("Enter a number: "); 16 } 17 system("PAUSE"); 18 return 0; 19 } 20 long fun(long s)// 函数定义 21 { 22 int i, a, b, j = 0; 23 long x = 0, t; 24 t = s; 25 b = length(s); 26 for(i = 0; i < b; i++) 27 { 28 a = t % 10; 29 if(a != 0) 30 { 31 if(a % 2 != 0) 32 { 33 x += a * pow(10, j); 34 j++; 35 } 36 37 } 38 t /= 10; 39 40 } 41 return x; 42 } 43 int length(long num) 44 { 45 int num1=0; 46 while(num>0) 47 { 48 num /= 10;//让num位数-1 49 num1++;//位数+1 50 } 51 return num1; 52 }
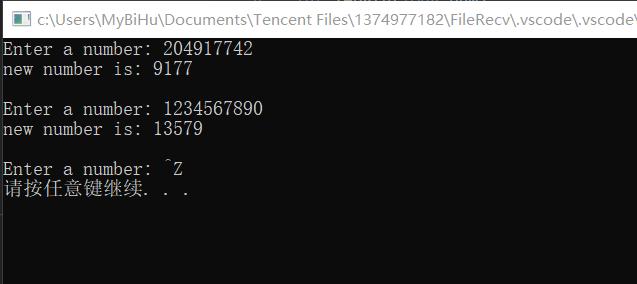