实验2(2022.4.12)
task1
1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <time.h> 4 5 #define N 5 6 7 int main() // 问题3: 随机列出5个学号,包括4个年级(2018 19 20 21)的学生 8 { 9 int grade, number; 10 int i; 11 12 srand(time(0)); // 以当前系统时间作为随机种子 13 14 for(i=0; i<N; ++i) 15 { 16 grade=rand()%4+2018; // 问题1:生成2018~2021之间的一个随机整数,其中4=(2021-2018+1) 17 number=rand()%999+1; // 问题2:生成1~999之间的一个随机整数 18 printf("%d8330%04d\n", grade, number); 19 } 20 21 system("PAUSE"); 22 return 0; 23 }
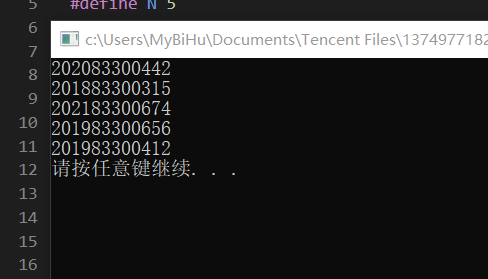
task2
1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <time.h> 4 5 #define N 1 6 7 int main() 8 { 9 int luckyday, date; 10 int i, a = 0; 11 12 srand(time(0)); // 以当前系统时间作为随机种子 13 14 printf("猜猜2022年4月哪一天会是你的lucky day\n"); 15 16 for(i=0; i<N; ++i) 17 { 18 luckyday=rand()%30+1; //生成1~30之间的一个随机整数 19 } 20 21 scanf("%d", &date); 22 printf("开始喽,你有三次机会,猜吧(1~30): %d\n", date); 23 24 25 if(date == luckyday) 26 printf("哇,猜中了:-)\n"); 27 if(date != luckyday) 28 { 29 do{ 30 if(date < luckyday) 31 printf("你猜的日期早了,你的lucky day还没到呢\n"); 32 else if(date > luckyday) 33 printf("你猜的日期晚了,你的lucky day已经过啦\n"); 34 35 scanf("%d", &date); 36 printf("再猜(1~30): %d\n", date); 37 ++a; 38 }while(a == 2 && date == luckyday); 39 if(date == luckyday) 40 printf("哇,猜中了:-)\n"); 41 else if(date != luckyday) 42 printf("次数用完啦.偷偷告诉你,4月,你的luckyday是%d号\n", luckyday); 43 } 44 system("PAUSE"); 45 return 0; 46 }
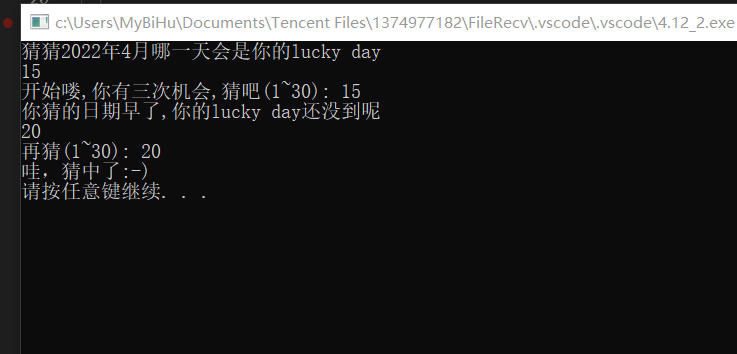
task3
1 #include<stdio.h> 2 #include<stdlib.h> 3 4 int main() 5 { 6 char color; 7 8 while(scanf("%c", &color) != EOF) 9 { 10 11 if(color == 114) 12 printf("stop!\n"); 13 14 if(color == 121) 15 printf("wait a minute\n"); 16 17 if(color == 103) 18 printf("go go go\n"); 19 20 if(color == 114 && color == 121 && color == 103) 21 printf("something must be wrong...\n"); 22 23 } 24 system("PAUSE"); 25 return 0; 26 27 }
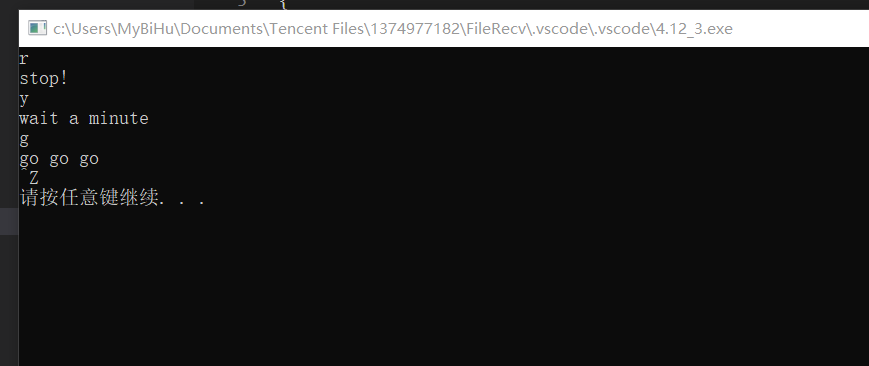
task4
1 #include<stdio.h> 2 #include<math.h> 3 #include<stdlib.h> 4 5 int main() 6 7 { 8 int n, a; 9 float c, i, b, s; 10 while(scanf("%d %d",&n, &a) != EOF) 11 { 12 s = 0; 13 for(i = 1; i <= n; ++i) 14 { 15 b = pow(a, i); 16 c = i / b; 17 s = s + c; 18 } 19 printf("n = %d, a = %d, s = %f\n", n, a, s); 20 } 21 22 system("PAUSE"); 23 return 0; 24 25 }
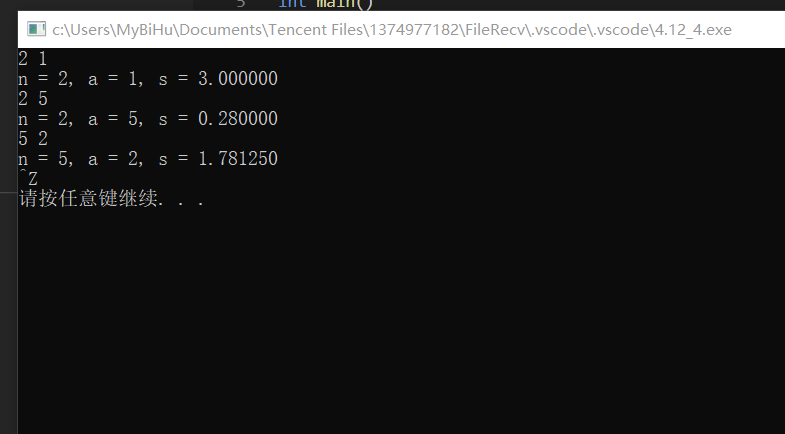
1 #include<stdio.h> 2 #include<stdlib.h> 3 4 int main() 5 { 6 int i, j, s; 7 for(i = 1; i <= 9; i++) 8 { 9 for(j = 1; j <= i; j++) 10 { 11 s = j * i; 12 if (s <= 9) 13 printf("%d×%d = %d ",j, i, s); 14 if (s > 9) 15 printf("%d×%d = %d ",j, i, s); 16 if(j == i) 17 printf("\n"); 18 } 19 } 20 system("PAUSE"); 21 return 0; 22 }
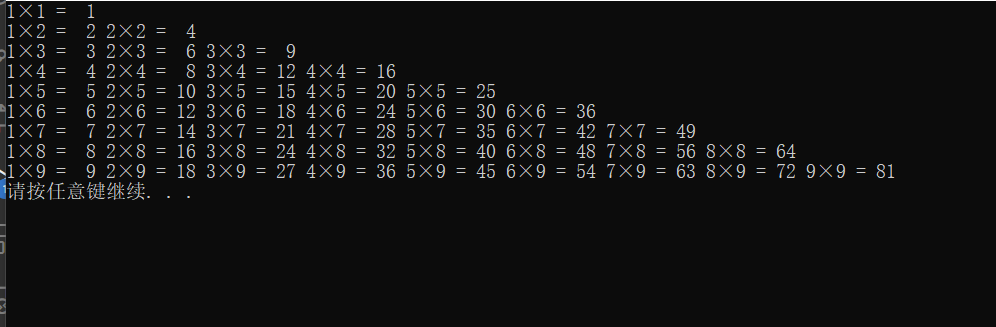
task6_1
1 #include<stdio.h> 2 #include<stdlib.h> 3 4 int main() 5 { 6 int n, j; 7 scanf("%d", &n); 8 printf("input n: %d\n", n); 9 10 for(j = 1; j <= n; j++) 11 { 12 printf(" O "); 13 printf("<H>"); 14 printf("I I"); 15 printf("\n"); 16 } 17 18 system("PAUSE"); 19 return 0; 20 }
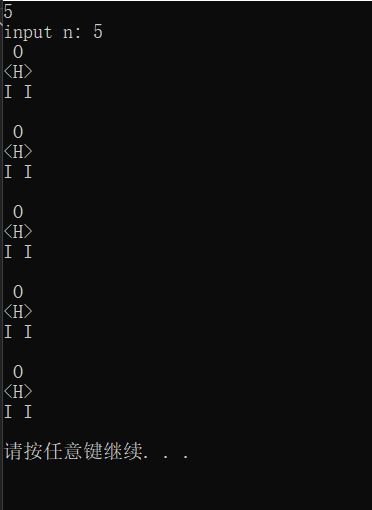
task6_2
1 #include<stdio.h> 2 #include<stdlib.h> 3 4 int main() 5 { 6 int n, m, a, j; 7 scanf("%d", &n); 8 printf("input n: %d\n", n); 9 10 for(j = 1; j <= n; j++) 11 { 12 printf(" O "); 13 } 14 printf("\n"); 15 for(m = 1; m <= n; m++) 16 { 17 printf("<H> "); 18 } 19 printf("\n"); 20 for(a = 1; a <= n; a++) 21 { 22 printf("I I "); 23 } 24 25 26 system("PAUSE"); 27 return 0; 28 }
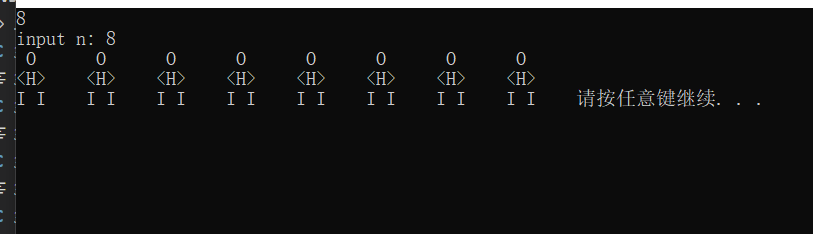
task6_3
1 #include<stdio.h> 2 #include<stdlib.h> 3 void blank(int x); 4 5 int main() 6 { 7 int n, m, a, j, line, b, s; 8 scanf("%d", &n); 9 printf("input n: %d\n", n); 10 s = n; 11 12 for(line = 1; line <= n; line++, s--) 13 { 14 15 blank(line); 16 b = 2 * s - 1; 17 for(j = 1; j <= b; j++) 18 { 19 printf(" O "); 20 } 21 printf("\n"); 22 blank(line); 23 for(m = 1; m <= b; m++) 24 { 25 printf("<H> "); 26 } 27 printf("\n"); 28 blank(line); 29 for(a = 1; a <= b; a++) 30 { 31 printf("I I "); 32 } 33 printf("\n"); 34 } 35 36 37 system("PAUSE"); 38 return 0; 39 } 40 void blank(int x) 41 { 42 int y; 43 for(y = 1; y <= x - 1; y++) 44 { 45 printf(" "); 46 } 47 48 }
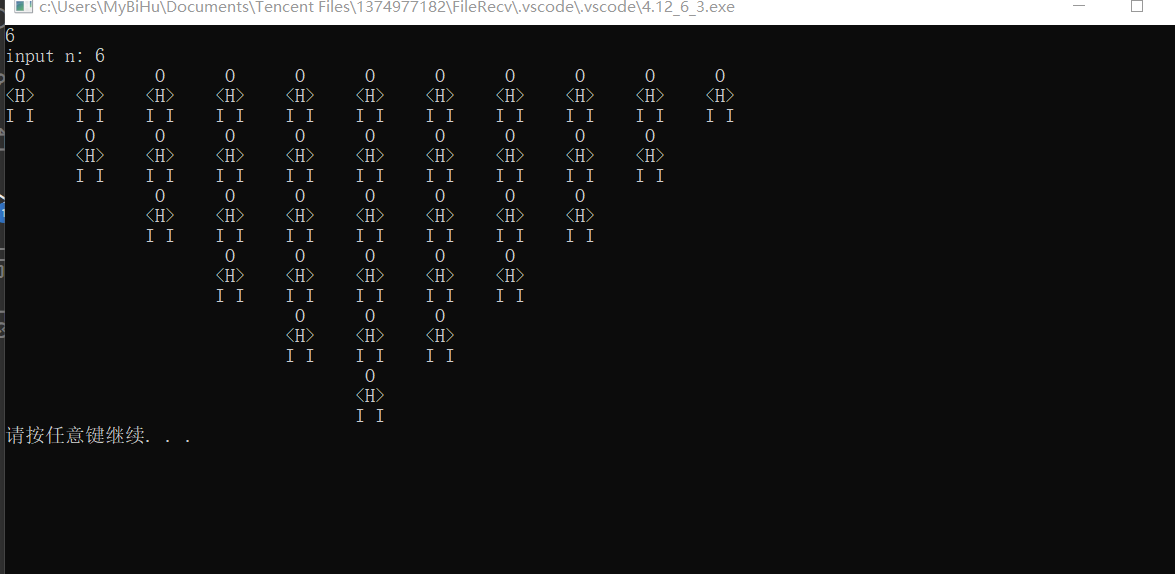
实验结论:
1.srand(time(0)),这个值是按照时间而变化的,所以,这个函数的作用,就是一个简单的设定随机数的种子方法。通过这个函数,可以得到每次都不一样的随机数;
2.函数的声明一般要放在头文件中;
3.使用while语句可实现多组输入。