ASP.NET MVC3 AJAX 上传图片示例
最近在博问中看到一个问题,问在MVC中如何用AJAX方式上传图片,这里做了一个DEMO,详细解释一下。
本DEMO代码非常简单,就是一个页面上有一个上传图片按钮,点击后弹出一个层,在这个弹出层里上传图片,然后把图片地址更新到页面上。在获得上传的图片地址后你可以做其他处理(如插入到文本编辑器中)。
Controller
public class ImageController : Controller { public ActionResult Index() { return View(); } [HttpPost] public JsonResult Upload(HttpPostedFileBase upImg) { string fileName = System.IO.Path.GetFileName(upImg.FileName); string filePhysicalPath = Server.MapPath("~/upload/" + fileName); string pic="", error=""; try { upImg.SaveAs(filePhysicalPath); pic = "/upload/" + fileName; } catch (Exception ex) { error = ex.Message; } return Json(new { pic = pic, error = error }); } }
提示:这里上传到网站根目录的upload文件夹中,请根据自己的需要更改或添加这个目录。
View
Index.cshtml:
@{ ViewBag.Title = "Index"; Layout = "~/Views/Shared/_Layout.cshtml"; } @section HeadCss{ <style type="text/css"> form{ border:1px solid #CCC; border-radius:5px; padding:10px; margin:10px 0; width:400px; background:#EEE; } </style> } @section HeadScript{ <script src="/Scripts/jquery.form.js" type="text/javascript"></script> <script type="text/javascript"> $(function () { $("#btn_show").bind("click", function () { $("#form_upload").show(); var options = { success: function (responseText, statusText, xhr, $form) { var picPath = responseText.pic; if (picPath == "") { alert(responseText.error); } else { $("#form_upload").hide(); $("#result").attr("src", picPath).show(); } }, error: function (XMLHttpRequest, textStatus, errorThrown) { console.log(textStatus); console.log(errorThrown); } }; $("#form_upload").ajaxForm(options); }); }); </script> } <input type="button" id="btn_show" value="上传图片" /><br /> <form id="form_upload" style="padding:20px; display:none;" action="upload" method="post" enctype="multipart/form-data"> <input name="upImg" style="width:350px;height:25px;" size="38" type="file"/><input type="submit" value="上传"/> </form> <img alt="" style="display:none;" id="result" src="" />
提示:在options的success方法中获取到上传的图片地址,你可以根据需要进行后续处理
_Layout.cshtml:
<!DOCTYPE html>
<html>
<head>
<title>@ViewBag.Title</title>
<link href="@Url.Content("~/Content/Site.css")" rel="stylesheet" type="text/css" />
@RenderSection("HeadCss",required:false)
<script src="@Url.Content("~/Scripts/jquery-1.4.4.min.js")" type="text/javascript"></script>
@RenderSection("HeadScript",required:false)
</head>
<body>
@RenderBody()
</body>
</html>
引用的几个文件
Site.css跟jquery-1.4.4.min.js就不说了,用VS创建MVC项目默认就有
jquery.form.js,这是一个jquery Form 插件,地址:http://jquery.malsup.com/form/
效果
打开页面,点击“上传图片”后选择一张图片
上传后效果图
PS:本文只是简单的示例,很多细节没有处理,请使用者自己根据需要完善
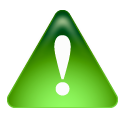