task4
task.cpp
#include <iostream>
#include "Vector.hpp"
void test() {
using namespace std;
int n;
cin >> n;
Vector<double> x1(n);
for(auto i = 0; i < n; ++i)
x1.at(i) = i * 0.7;
output(x1);
Vector<int> x2(n, 42);
Vector<int> x3(x2);
output(x2);
output(x3);
x2.at(0) = 77;
output(x2);
x3[0] = 999;
output(x3);
}
int main() {
test();
}
vector.hpp
#include<bits/stdc++.h>
using namespace std;
template<typename T>
class Vector{
private:
int length;
T * base;
public:
Vector(const int & n, const T & x);
Vector(const int & n);
Vector(const Vector<T> & obj);
~ Vector();
T & at(const int & w) const ;
T & operator [] (const int & w) const ;
int get_size() const;
template<typename TT>
friend void output(const Vector<TT> & obj);
};
template<typename T>
Vector<T>::Vector(const int & n){
length = n;
base = new T[n];
}
template<typename T>
Vector<T>::Vector(const int & n, const T & x){
length = n;
base = new T[n];
for(int i = 0; i < n; i ++)
base[i] = x;
}
template<typename T>
Vector<T>::Vector(const Vector<T> & obj){
int n = obj.get_size();
length = n;
base = new T[n];
for(int i = 0; i < n; i ++)
base[i] = obj[i];
}
template<typename T>
T & Vector<T>::at(const int & w) const {
return base[w];
}
template<typename T>
T & Vector<T>::operator [] (const int & w) const {
return base[w];
}
template<typename T>
int Vector<T>::get_size() const {
return length;
}
template<typename T>
Vector<T>::~Vector(){
delete [] base;
}
template<typename TT>
void output(const Vector<TT> & obj){
for(int i = 0; i < obj.get_size(); i ++)
cout << obj[i] << " ";
cout << endl;
}
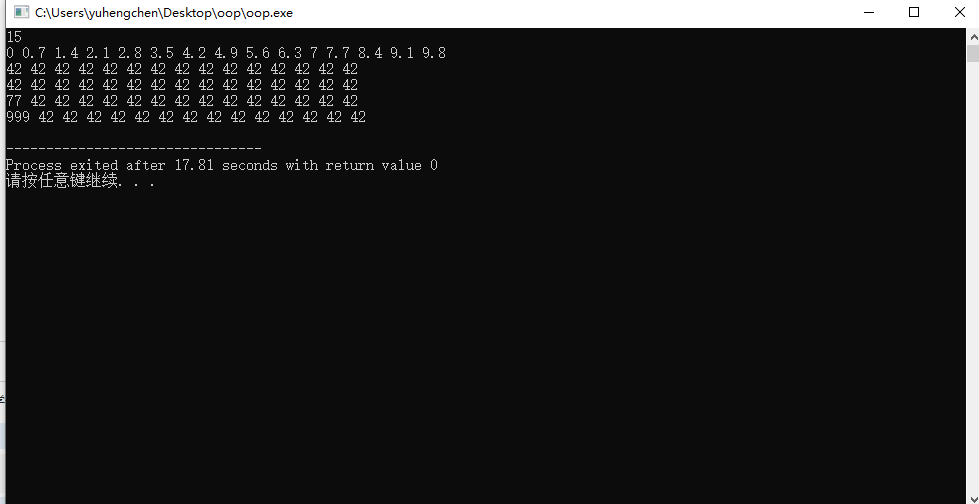
task5
#include<fstream>
#include<iostream>
#include<bits/stdc++.h>
using namespace std;
void output(ofstream &out, string To = "con"){
out.open(To);
if(!out.is_open()){
cout << "open fail!!\n";
return ;
}
out << " ";
for(char c = 'a'; c <= 'z'; c ++)
out << ' ' << c;
out << endl;
for(int i = 1; i <= 26; i ++){
out << setw(2) << right << i;
for(int drt = 1; drt <= 26; drt ++)
out << ' ' << (char)((drt + i - 1) % 26 + 'A');
out << endl;
}
out.close();
}
int main(){
ofstream out;
output(out);
output(out, "cipher_key.txt");
}
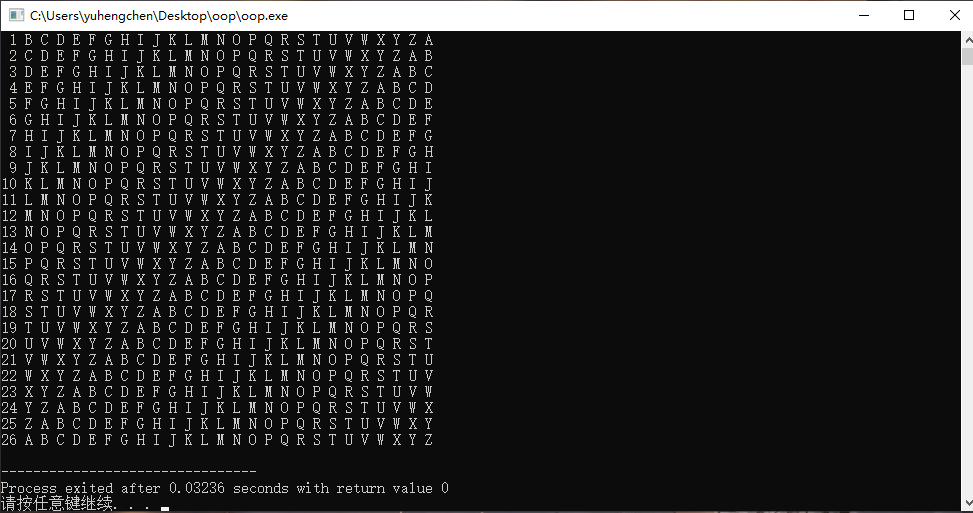