期末大作业
#1.回归模型预测波士顿房价 #导入load_boston数据 from sklearn.datasets import load_boston data = load_boston() #多元线性回归模型 from sklearn.model_selection import train_test_split # 训练集与测试集划分为7:3 x_train,x_test,y_train,y_test = train_test_split(data.data,data.target,test_size=0.3) print(x_train.shape,y_train.shape) #线性回归模型:建立13个变量与房价之间的预测模型,并检测模型好 #线性回归模型公式:y=^bx+^a from sklearn.linear_model import LinearRegression mlr = LinearRegression() mlr.fit(x_train,y_train) print('系数b',mlr.coef_,"\n截距a",mlr.intercept_) #检测模型的好坏 from sklearn.metrics import regression y_predict = mlr.predict(x_test) #计算模型的预测指标 print('线性回归模型判断指数') print("预测的均方误差:",regression.mean_squared_error(y_test,y_predict)) print("预测的平均绝对误差:",regression.mean_absolute_error(y_test,y_predict)) #打印模型分数 print("模型的分数:",mlr.score(x_test,y_test)) #多项式回归模型:建立13个变量与房价之间的预测模型,并检测模型好坏。 # 多项式回归模型公式y = a0 + a1 * x + a2 * (x**2) + ... + an * (x ** n) + e from sklearn.preprocessing import PolynomialFeatures #多项式的训练集与测试集 poly2 =PolynomialFeatures(degree=2) x_poly_train = poly2.fit_transform(x_train) x_poly_test = poly2.transform(x_test) #多项回归模型 mlrp=LinearRegression() mlrp.fit(x_poly_train,y_train) #预测值 y_predict2 = mlrp.predict(x_poly_test) #检测模型预测指数的好坏 print("多项式回归模型判断指数") print("预测的均方误差:",regression.mean_squared_error(y_test,y_predict2)) print("预测平均绝对误差:",regression.mean_absolute_error(y_test,y_predict2)) #打印模型分数 print("模型的分数:",mlrp.score(x_poly_test,y_test))
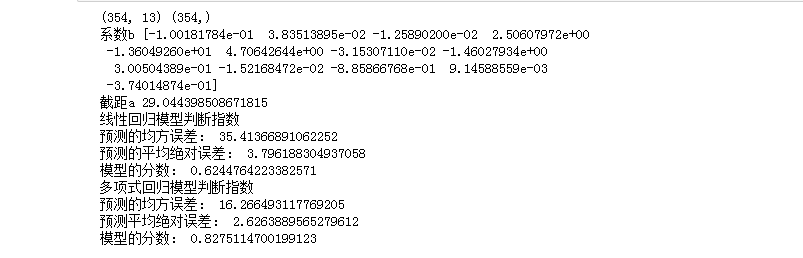
#新闻文本分类 import os import jieba #读取文件内容 content=[]#存放新闻的内容 label=[]#存放新闻的类别 def read_txt(path): folder_list=os.listdir(path)#遍历data下的文件名 for file in folder_list: new_path=os.path.join(path,file) #读取文件夹的名称,生成新的路径 files=os.listdir(new_path)#存放文件的内容 # i=1 #遍历每个txt文件 for f in files: # if i>50: # break with open(os.path.join(new_path,f),'r',encoding='UTF-8')as f: #打开txt文件 temp_file=f.read() content.append(processing(temp_file)) label.append(file) # i+=1 # print(content) # print(label) #对数据进行预处理 with open(r'C:\Users\ASUS\Desktop\stopsCN.txt', encoding='utf-8') as f: stopwords = f.read().split('\n') def processing(texts): # 去掉非法的字符 texts = "".join([char for char in texts if char.isalpha()]) # 用jieba分词 texts = [text for text in jieba.cut(texts,cut_all=True) if len(text) >=2] # 去掉停用词 texts = " ".join([text for text in texts if text not in stopwords]) return texts if __name__== '__main__': path=r'C:\Users\ASUS\Desktop\0369' read_txt(path) #划分训练集和测试,用TF-IDF算法进行单词权值的计算 from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.model_selection import train_test_split tfidf= TfidfVectorizer() x_train,x_test,y_train,y_test=train_test_split(content,label,test_size=0.2) X_train=tfidf.fit_transform(x_train) X_test=tfidf.transform(x_test) #构建贝叶斯模型 from sklearn.naive_bayes import MultinomialNB #用于离散特征分类,文本分类单词统计,以出现的次数作为特征值 mulp=MultinomialNB () mulp_NB=mulp.fit(X_train,y_train) #对模型进行预测 y_predict=mulp.predict(X_test) # # 从sklearn.metrics里导入classification_report做分类的性能报告 from sklearn.metrics import classification_report print('模型的准确率为:', mulp.score(X_test, y_test)) print('classification_report:\n',classification_report(y_test, y_predict))
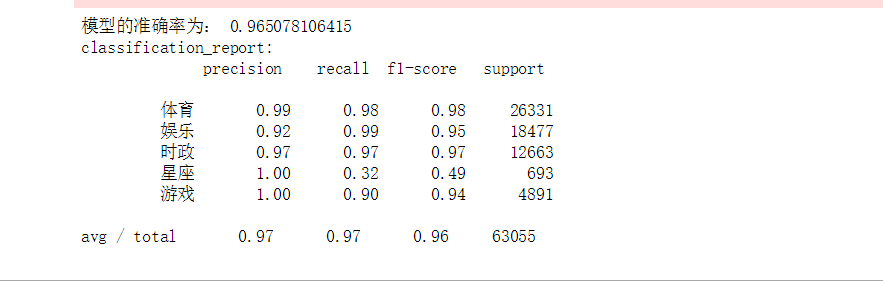
# 将预测结果和实际结果进行对比 import collections import matplotlib.pyplot as plt # 统计测试集和预测集的各类新闻个数 testCount = collections.Counter(y_test) predCount = collections.Counter(y_predict) print('实际:',testCount,'\n', '预测', predCount) # 建立标签列表,实际结果列表,预测结果列表, nameList = list(testCount.keys()) testList = list(testCount.values()) predictList = list(predCount.values()) x = list(range(len(nameList))) print("新闻类别:",nameList,'\n',"实际:",testList,'\n',"预测:",predictList)