Python-微信机器人
一、简介
wxpy基于itchat,使用了 Web 微信的通讯协议,,通过大量接口优化提升了模块的易用性,并进行丰富的功能扩展。实现了微信登录、收发消息、搜索好友、数据统计等功能。
总而言之,可用来实现各种微信个人号的自动化操作。
https://wxpy.readthedocs.io/zh/latest/index.html
安装:wxpy模块(支持 Python 3.4-3.+ 以及 2.7 版本):pip3 install wxpy
安装 pillow模块:pip3 install pillow
安装 pyecharts模块:pip3 install pyecharts==0.1.9.4
二、微信登录
from wxpy import * bot = Bot()
扫码登录微信,生成bot对象,对微信进行一系列操作
但是上面的代码有一个很明显的缺陷,就是你不管又没有修改你的程序,每次执行都需要重新登录你的微信,但是呢,里面有一个参数可以帮我们解决这个问题,
它可以通过缓存的形式将我们的登录信息保存下来,这个一来就不需要每次都进行扫码登录了。
from wxpy import * bot = Bot(cache_path=True)
三、微信好友男女比例
from wxpy import * import webbrowser from pyecharts import Pie bot = Bot(cache_path=True) # 弹出二维码登录微信,生成bot对象 friends = bot.friends() # 获取所有的微信好友信息 attr = ['男盆友','女盆友','其他'] # 设置男性/女性/未知性别好友名称 value = [0,0,0] # 初始化对象好友数量 # 遍历所有好友,判断该好友性别 for friend in friends: if friend.sex == 1: value[0] += 1 elif friend.sex == 2: value[1] += 1 else: value[2] += 1 pie = Pie("Sean的好朋友们") pie.add("",attr,value,is_label_show=True) pie.render("friends.html") webbrowser.open('friends.html')
四、微信好友地区分布
from wxpy import * from pyecharts import Map import webbrowser bot = Bot(cache_path=True) friends = bot.friends() area_dic = {} # 定义一个空字典,用于存放省市以及省市人数 for friend in friends: if friend.province not in area_dic: area_dic[friend.province] = 1 else: area_dic[friend.province] += 1 attr = area_dic.keys() value = area_dic.values() map = Map("Sean的好友地域分布",width=1200,height=600) map.add("好友地域分布",attr,value,maptype='china',is_visualmap=True) map.render("area.html") webbrowser.open("area.html")
五、微信聊天机器人
1、常用方法
from wxpy import * # bot = Bot(cache_path=True) # 给指定朋友发送消息 # friend = bot.friends().search("tank")[0] # friend.send("hello,达莎雕") # 获取所有好友 # my_friend = bot.friends(update=False) # print("sean的好友数:"+str(len(my_friend)-1)) # 获取所有微信群 # group = bot.groups(update=False) # print(group) # print("sean的微信群聊数:"+str(len(group))) # 获取所有关注的微信公众号 # mp = bot.mps(update=False) # print("sean关注的微信公众号" + str(len(mp)))
2、给文件传输助手发信息
这里的file_helper就是微信的文件传输助手,我们给文件传输助手发送一条消息,可以在手机端的文件传输助手中收到括号内的消息
bot.file_helper.send('hello')
3、收发消息@bot.register()
from wxpy import * bot = Bot(cache_path=True) friend = bot.friends().search('tank')[0] friend.send("hello 达莎雕") @bot.register(friend) def recv_send_msg(recv_msg): print('收到的消息:', recv_msg) return '自动回复:{}'.format(recv_msg)
# 进入Python命令行,让程序保持运行
embed()
4、自动给对象回复消息
当你在宿舍打着LOL,各种骚断腿的操作的时候,你对象让你出来陪她逛街、吃饭,你怎么办....
from wxpy import * bot = Bot(cache_path=True) girl_friend = bot.friends().search('girl_friend')[0] @bot.register(friend) def recv_send_friend(recv_msg): print("[接收]" + str(recv_msg)) if recv_msg.type != 'Text': ret = "我想陪你看遍世间美景,然后告诉你,它们都不及你万分之一。" elif "这是什么" in str(recv_msg): ret = "这是我的微信机器人" else: ret = "今晚月色真美" print('[发送]' + str(ret)) return ret embed()
5、定位微信群好友自动回复消息
from wxpy import * bot = Bot(cache_path=True) company_group = bot.groups()[3] # 获取指定群 no = company_group.members # 获取群内所有成员信息 @bot.register(chats=company_group) #接收从指定群发来的消息,发送者即recv_msg.sender为组 def recv_send_msg(recv_msg): print('收到的消息:',recv_msg.text) if recv_msg.member == no[0]: #这里不用recv_msg.render 因为render是群的名字 recv_msg.forward(bot.file_helper,prefix='测试发言: ') return '你是达莎雕?' # elif recv_msg.member == elif recv_msg.member == no[2]: return '鬼刀一开看不见' embed()
6、聊天机器人
第一种方式:
1、指定好友回复
from wxpy import * import requests import json # 调用图灵机器人API,发送消息并且获得机器人的回复 def auto_reply(text): url = 'http://openapi.tuling123.com/openapi/api/v2' api_key = '51a9bec5710941dda6ef836e164a4585' payload = { "reqType": 0, "perception": { "inputText": { "text": text }, "inputImage": { "url": "imageUrl" }, "selfInfo": { "location": { "city": "上海", "province": "上海", "street": "御青路" } } }, "userInfo": { "apiKey": api_key, "userId": "123456" } } re = requests.post(url, data=json.dumps(payload)) result = json.loads(re.content) return "[来自sean的智能机器人]:" + result['results'][0]['values']['text'] bot = Bot(cache_path=True) friend = bot.friends().search('好友名称')[0] @bot.register(friend) def forward_msg(msg): return auto_reply(msg.text) embed()
2、指定群回复
import json import requests from wxpy import * bot = Bot(cache_path=False) group=bot.groups().search('群名字')[0] print(group) # 调用图灵机器人API,发送消息并且获得机器人的回复 def auto_reply(text): url = 'http://openapi.tuling123.com/openapi/api/v2' api_key = '51a9bec5710941dda6ef836e164a4585' payload = { "reqType": 0, "perception": { "inputText": { "text": text }, "inputImage": { "url": "imageUrl" }, "selfInfo": { "location": { "city": "上海", "province": "上海", "street": "御青路" } } }, "userInfo": { "apiKey": api_key, "userId": "123456" } } re = requests.post(url, data=json.dumps(payload)) result = json.loads(re.content) return "[来自sean的智能机器人]:" + result['results'][0]['values']['text'] @bot.register(chats=group) def forward_message(msg): return auto_reply(msg.text) embed()
3、所有人自动回复(慎用)
import json import requests from wxpy import * bot = Bot(cache_path=True) # 调用图灵机器人API,发送消息并且获得机器人的回复 def auto_reply(text): url = 'http://openapi.tuling123.com/openapi/api/v2' api_key = '51a9bec5710941dda6ef836e164a4585' payload = { "reqType": 0, "perception": { "inputText": { "text": text }, "inputImage": { "url": "imageUrl" }, "selfInfo": { "location": { "city": "上海", "province": "上海", "street": "御青路" } } }, "userInfo": { "apiKey": api_key, "userId": "123456" } } re = requests.post(url, data=json.dumps(payload)) result = json.loads(re.content) return "[来自sean的智能机器人]:" + result['results'][0]['values']['text'] @bot.register() def forward_message(msg): return auto_reply(msg.text) embed()
第二种方式:
from wxpy import * bot = Bot(cache_path=True) friend = bot.friends().search('魏倩倩Bella')[0] print(friend) # wxpy自动集成了图灵机器人的接口,我们只需要调用即可 tuling = Tuling(api_key='a2064e6849a64c16aaf29452842f40f7') @bot.register(friend) def forward_msg(msg): tuling.do_reply(msg) embed()
作者信息
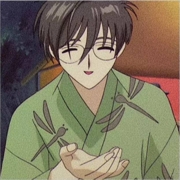
Sean
Stay hungry,Stay foolish.