看下面这段LINQ to SQL示例代码
using System;
using System.Linq;
using System.Data.Linq;
using System.Data.Linq.Mapping;
class Program
{
static void Main(string[] args)
{
//直接传入数据库文件的路径,需要Express版本的数据库
var path = System.IO.Path.GetFullPath(@"..\..\..\Data\NORTHWND.mdf");
var db = new DataContext(path);
var customers =
from customer in db.GetTable<Customer>()
where customer.City == "London"
select customer;
foreach (var customer in customers)
Console.WriteLine("Contact: " + customer.Name);
}
}
//数据表映射类
[Table(Name = "Customers")]
class Customer
{
[Column(IsPrimaryKey = true)]
public string CustomerID { get; set; }
[Column(Name = "ContactName")]
public string Name { get; set; }
[Column]
public string City { get; set; }
}
LINQ to SQL自动实现的一些功能:
1 打开与数据库的连接;
2 生成SQL查询;
3 执行SQL查询;
4 将执行结果填充至对象中。
回想一下传统的ADO.NET需要用到的一些类:DbCommand、DbConnection、DbReader、DataSet、DataTable等,功能相似的ADO.NET代码要多少行
下面是LINQ to SQL和ADO.NET的对比:
传统的ADO.NET相对于LINQ to SQL的不足:
1 以字符串形式给出的SQL语句;
2 没有编译时检查;
3 松散绑定的参数;
4 弱类型的结果集;
5 代码冗长;
6 需要掌握更多技能。
LINQ to SQL对于ADO.NET更符合面向对象
如果上面的代码还不能说明LINQ所带来的优势,那下面这段同时操作数据库和XML的示例代码,会更加明显地说明问题
using System;
using System.Linq;
using System.Xml.Linq;
using System.Data.Linq;
using System.Data.Linq.Mapping;
class Program
{
static void Main(string[] args)
{
//直接传入数据库文件的路径,需要Express版本的数据库
var path = System.IO.Path.GetFullPath(@"..\..\..\Data\NORTHWND.mdf");
var db = new DataContext(path);
var customers =
from customer in db.GetTable<Customer>().Take(6)
select customer;
var xml = new XElement("Customers",
new XAttribute("Date", "20101101"),
new XElement("Customer",
new XElement("CustomerID", "Programmer"),
new XElement("Name", "薛江白"),
new XElement("City", "上海")
),
from customer in customers //查询表达式
orderby customer.City
select new XElement("Customer",
new XElement("CustomerID", customer.CustomerID),
new XElement("Name", customer.Name),
new XElement("City", customer.City)
)
);
Console.WriteLine(xml);
}
}
//数据表映射类
[Table(Name = "Customers")]
class Customer
{
[Column(IsPrimaryKey = true)]
public string CustomerID { get; set; }
[Column(Name = "ContactName")]
public string Name { get; set; }
[Column]
public string City { get; set; }
}
试想一下用传统的ADO.NET加System.Xml命名空间下的一些类:XmlDocument、XmlElement、XmlReader、XPathNavigator、XslTransform等,需要多少行代码,自己试一下就知道了
作者:Lucifer Xue
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。
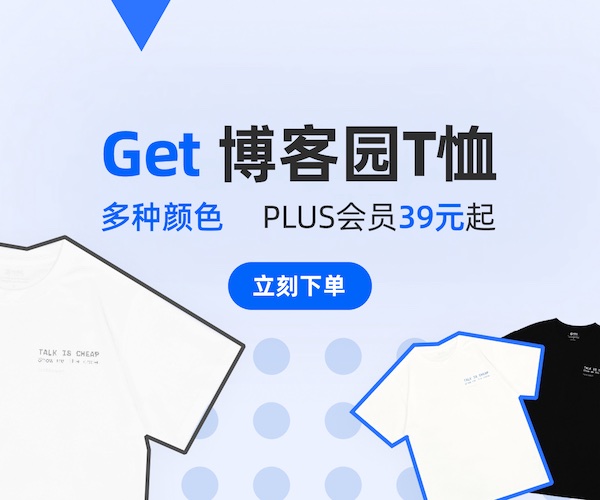