日志统计
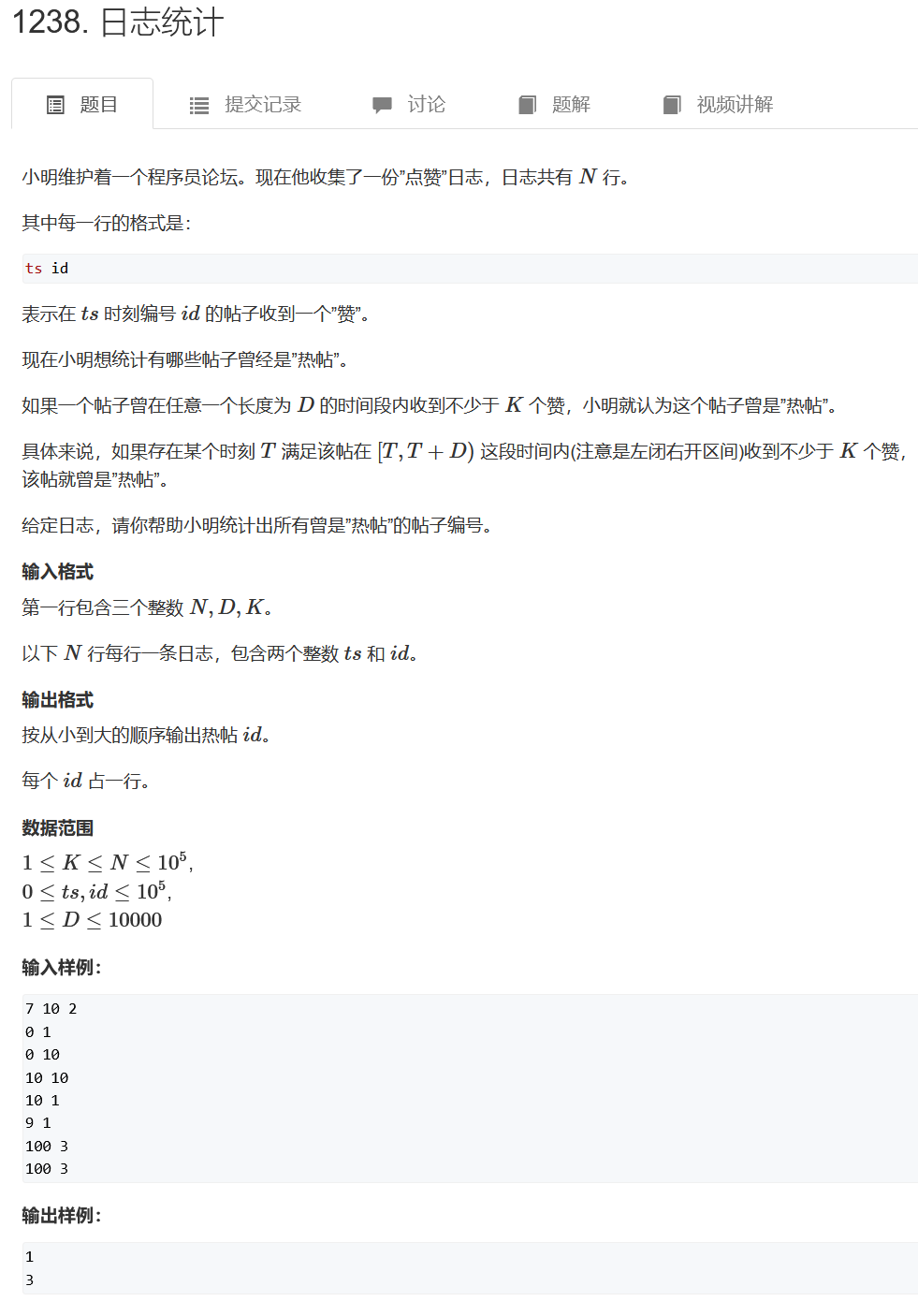
#include<iostream>
#include<algorithm>
using namespace std;
const int N=1e5+10;
#define x first
#define y second
typedef pair<int,int> PII;
int n,d,k;
PII logs[N];
bool st[N];
int cnt[N];
int main(){
scanf("%d%d%d",&n,&d,&k);
for(int i=0;i<n;i++)
scanf("%d%d",&logs[i].x,&logs[i].y);
sort(logs,logs+n);
for(int i=0,j=0;i<n;i++){
int id = logs[i].y;
cnt[id] ++;
// j在前
while(logs[i].x - logs[j].x>=d){
cnt[logs[j].y]--;
j ++;
}
if(cnt[id]>=k)st[id]=true;
}
for(int i=0;i<N;i++)
if(st[i])cout << i << endl;
return 0;
}
献给阿尔吉侬的花束
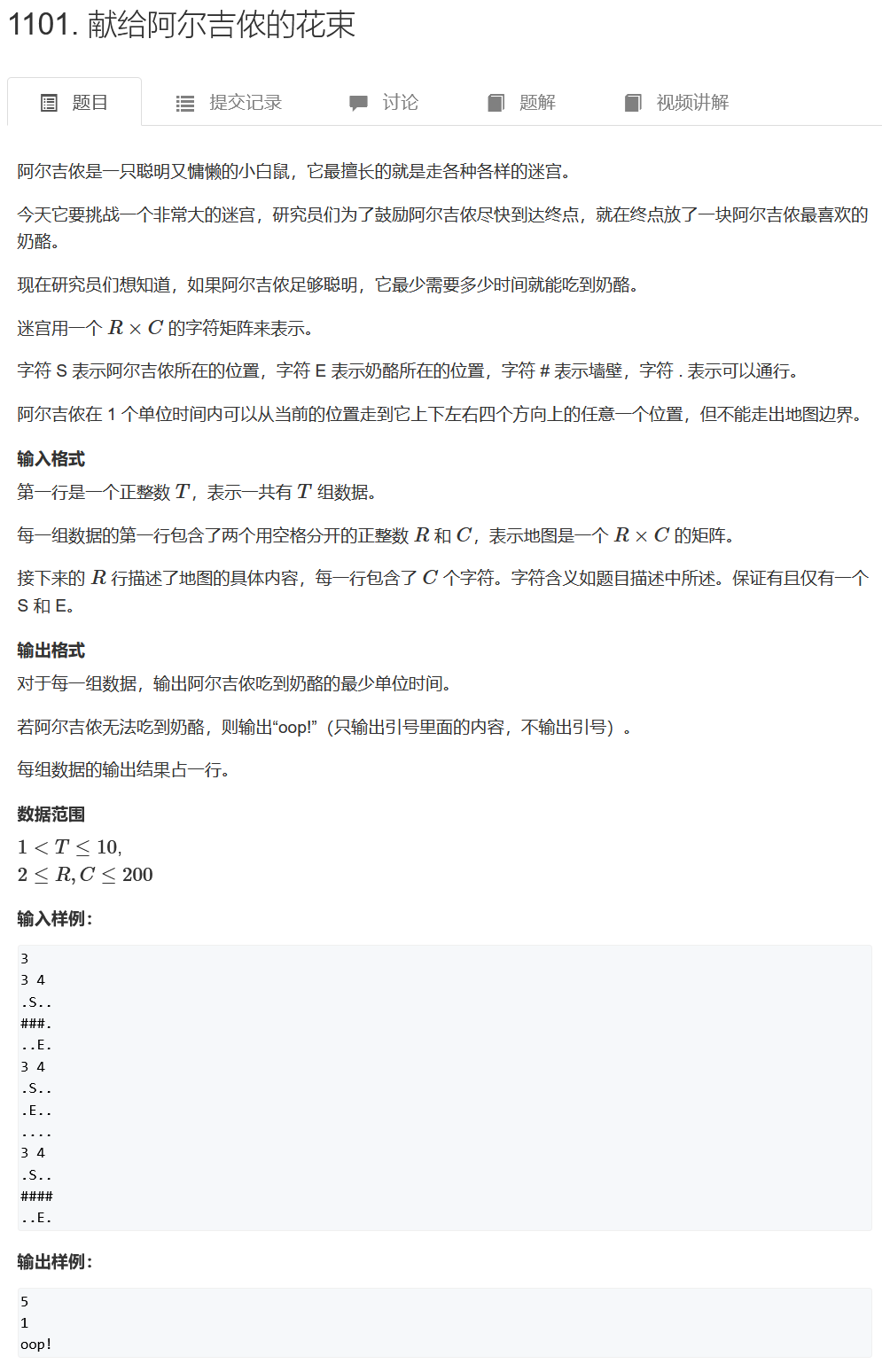
#include<bits/stdc++.h>
using namespace std;
typedef pair<int,int> PII;
#define x first
#define y second
const int N=210;
char g[N][N];
int n,m;
int dist[N][N];
int dx[]={-1,0,1,0},dy[]={0,1,0,-1};
int bfs(int x,int y){
queue<PII> q;
q.push({x,y});
memset(dist,-1,sizeof dist);
dist[x][y]=0;
while(!q.empty()){
PII t = q.front();
q.pop();
if(g[t.x][t.y]=='E')
return dist[t.x][t.y];
for(int d=0;d<4;d++){
int tx=t.x+dx[d],ty=t.y+dy[d];
if(tx<0 || tx>=n || ty<0 || ty>=m || g[tx][ty]=='#' || dist[tx][ty]!=-1)
continue;
dist[tx][ty]=dist[t.x][t.y]+1;
q.push({tx,ty});
}
}
return -1;
}
int main(){
int T;
cin >> T;
while(T--){
cin >> n >> m;
for(int i=0;i<n;i++)
cin >> g[i];
int x,y;
for(int i=0;i<n;i++)
for(int j=0;j<m;j++)
if(g[i][j]=='S')
x=i,y=j;
int res = bfs(x,y);
if(res==-1)cout << "oop!" << endl;
else cout << res << endl;
}
return 0;
}
红与黑
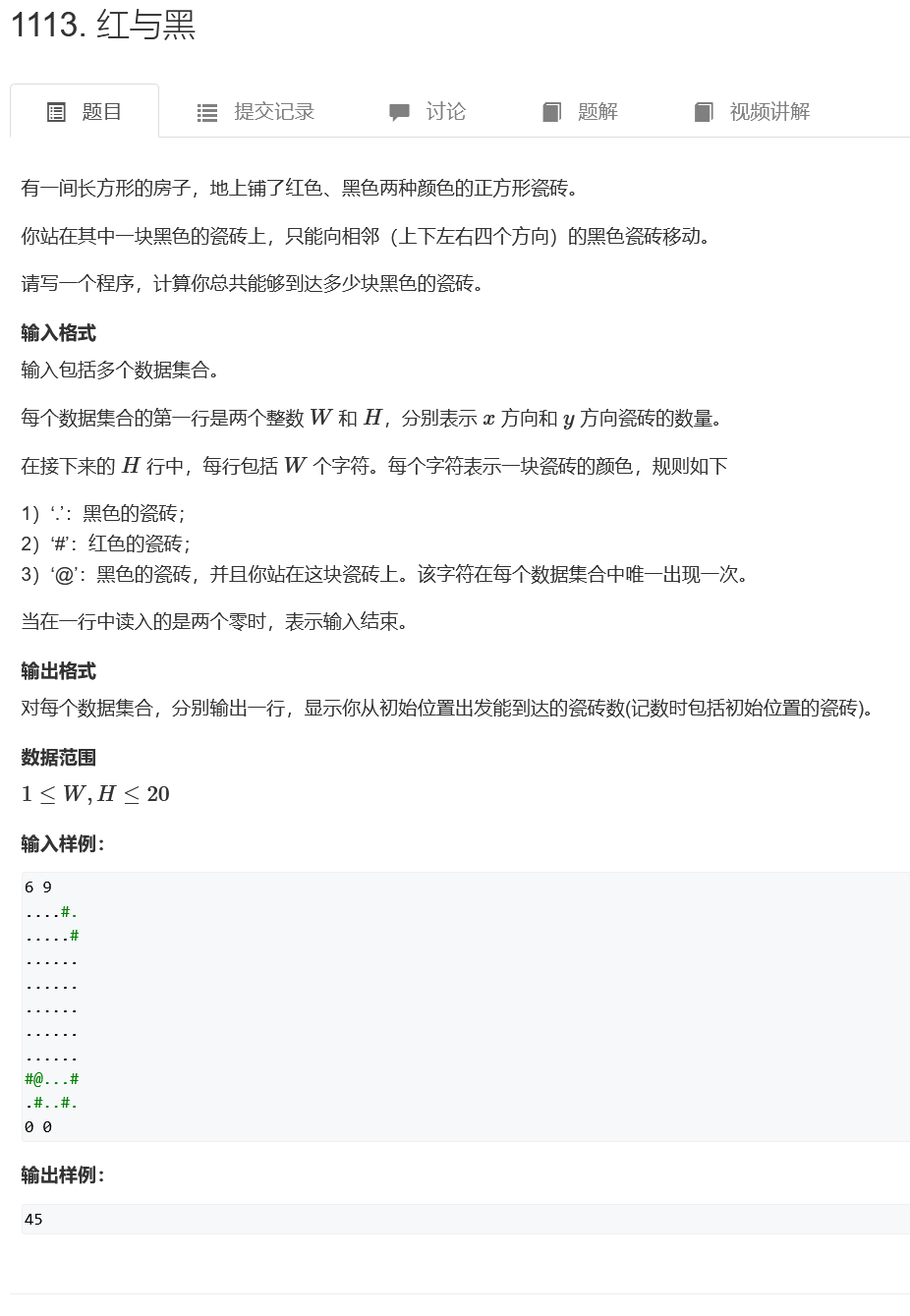
#include<bits/stdc++.h>
using namespace std;
typedef pair<int,int> PII;
#define x first
#define y second
const int N=30;
char g[N][N];
int h,w;
int dx[]={-1,0,1,0},dy[]={0,1,0,-1};
int dfs(int x,int y){
int res=0;
g[x][y]='#';
res ++;
for(int d=0;d<4;d++){
int tx=x+dx[d],ty=y+dy[d];
if(tx<0 || tx>=h || ty<0 || ty>=w || g[tx][ty]!='.')continue;
res += dfs(tx,ty);
}
return res;
}
int main(){
while(cin>>w>>h,w||h){
for(int i=0;i<h;i++)
cin >> g[i];
int x=0,y=0;
for(int i=0;i<h;i++)
for(int j=0;j<w;j++)
if(g[i][j]=='@')x=i,y=j;
cout << dfs(x,y) << endl;
}
return 0;
}
交换瓶子
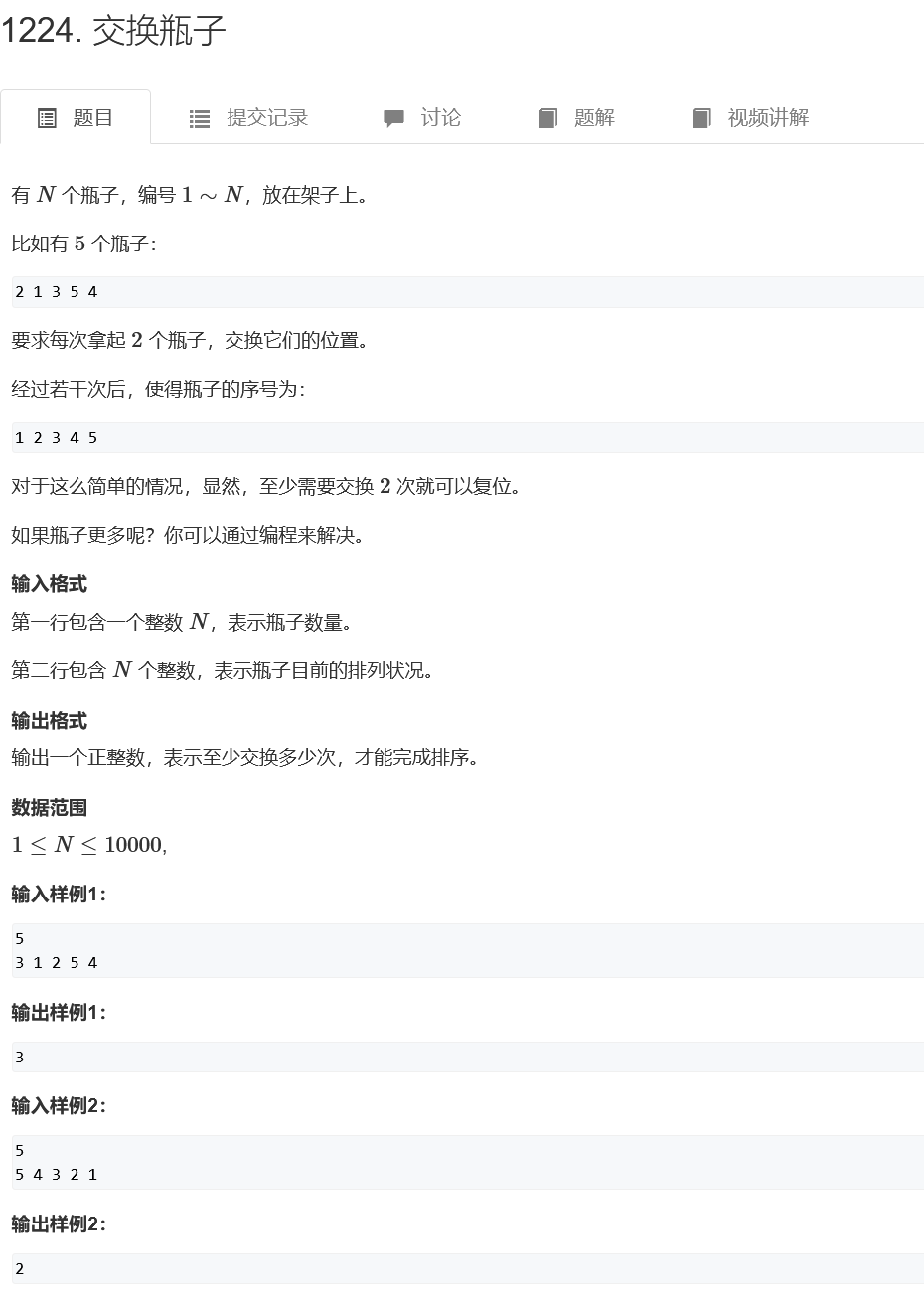
#include<iostream>
using namespace std;
const int N=1e4+10;
int q[N];
int n;
int cnt;
int pos(int x){
for(int i=1;i<=n;i++)
if(q[i]==x)return i;
return -1;
}
int main(){
cin >> n;
for(int i=1;i<=n;i++)
scanf("%d",&q[i]);
for(int i=1;i<=n;i++){
if(i!=q[i]){
swap(q[i],q[pos(i)]);
cnt ++;
}
}
cout << cnt << endl;
return 0;
}
完全二叉树的权值
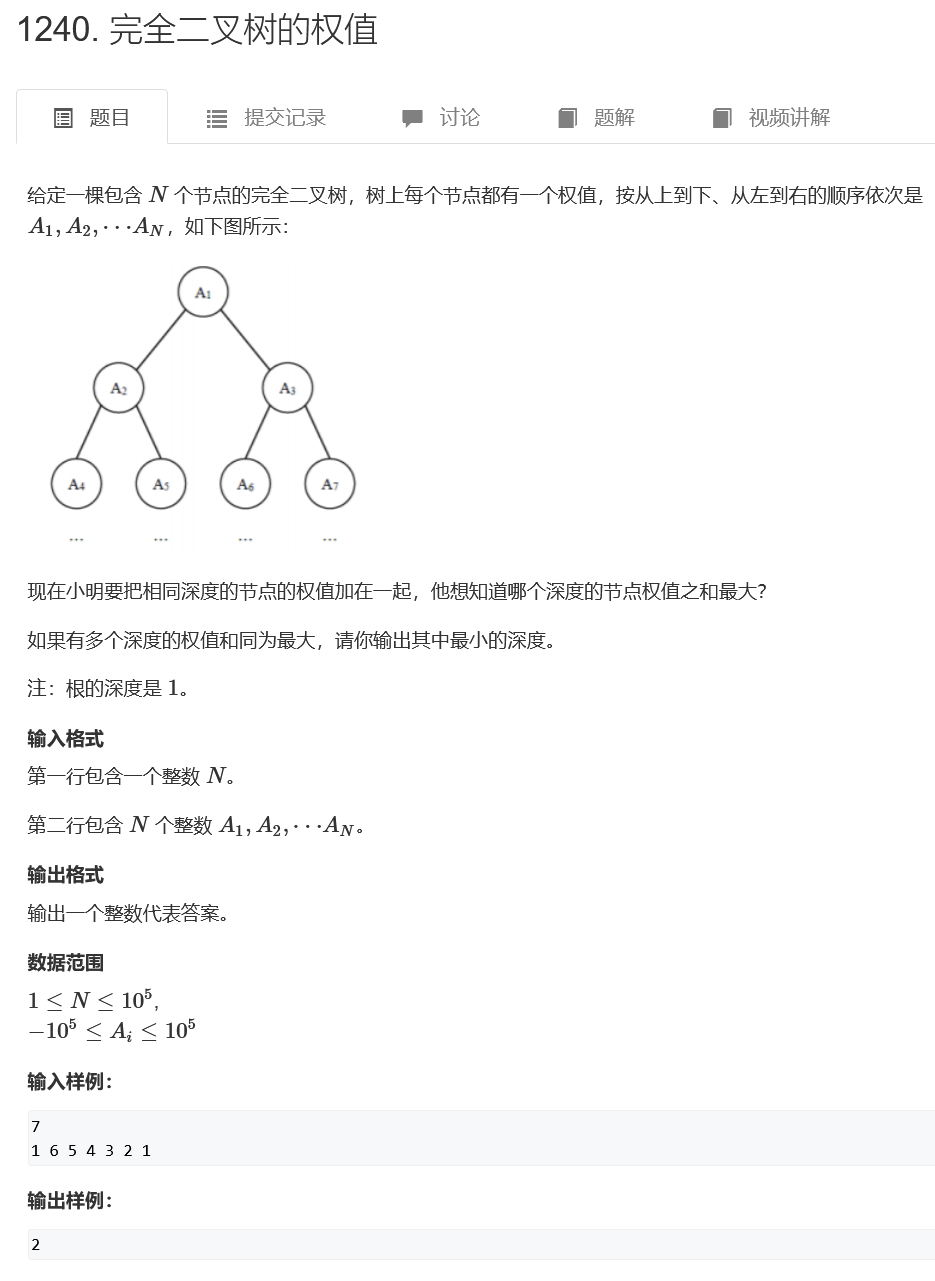
#include<bits/stdc++.h>
using namespace std;
typedef long long LL;
const int N=1e5+10,INF=1<<30;
int q[N],n;
int main(){
cin >> n;
for(int i=1;i<=n;i++)
scanf("%d",&q[i]);
LL maxs=-INF;
int depth=0;
for(int i=1,d=1;i<=n;i*=2,d++){
LL s=0;
for(int j=i;j<i+(1<<d-1) && j<=n;j++)
s+=q[j];
if(maxs<s){
maxs=s;
depth=d;
}
}
cout << depth << endl;
return 0;
}
地牢大师
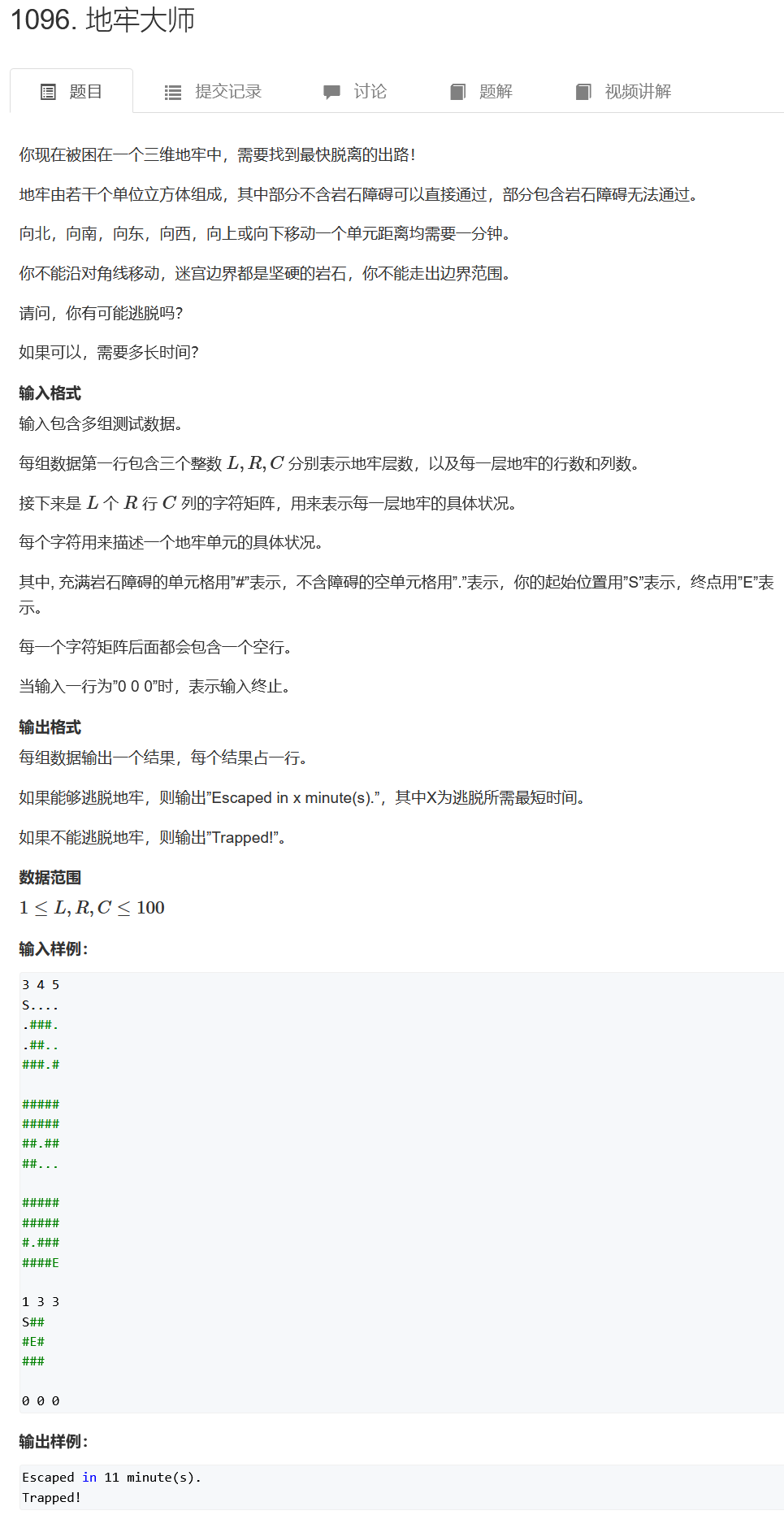
#include<iostream>
#include<cstring>
#include<algorithm>
#include<queue>
using namespace std;
const int N=110;
struct PIII{
int x,y,z;
};
int L,R,C;
char g[N][N][N];
int dist[N][N][N];
int dx[6] = {1, -1, 0, 0, 0, 0};
int dy[6] = {0, 0, 1, -1, 0, 0};
int dz[6] = {0, 0, 0, 0, 1, -1};
int bfs(PIII start,PIII end){
queue<PIII> q;
memset(dist,-1,sizeof dist);
q.push(start);
dist[start.x][start.y][start.z]=0;
while(!q.empty()){
PIII t=q.front();q.pop();
if(t.x==end.x && t.y==end.y && t.z==end.z)
return dist[t.x][t.y][t.z];
for(int i=0;i<6;i++){
int tx=t.x+dx[i],ty=t.y+dy[i],tz=t.z+dz[i];
if(tx<0 || tx>=L || ty<0 || ty>=R || tz<0 || tz>=C)continue;
if(dist[tx][ty][tz]!=-1 || g[tx][ty][tz]=='#')continue;
dist[tx][ty][tz]=dist[t.x][t.y][t.z]+1;
q.push({tx,ty,tz});
}
}
return -1;
}
int main(){
while(cin>>L>>R>>C, L||R||C){
PIII start,end;
for(int i=0;i<L;i++){
for(int j=0;j<R;j++){
cin >> g[i][j];
for(int k=0;k<C;k++){
if(g[i][j][k]=='S'){
start = {i,j,k};
}
if(g[i][j][k]=='E'){
end = {i,j,k};
}
}
}
}
int ret = bfs(start,end);
if(ret==-1)puts("Trapped!");
else printf("Escaped in %d minute(s).\n",ret);
}
return 0;
}
全球变暖
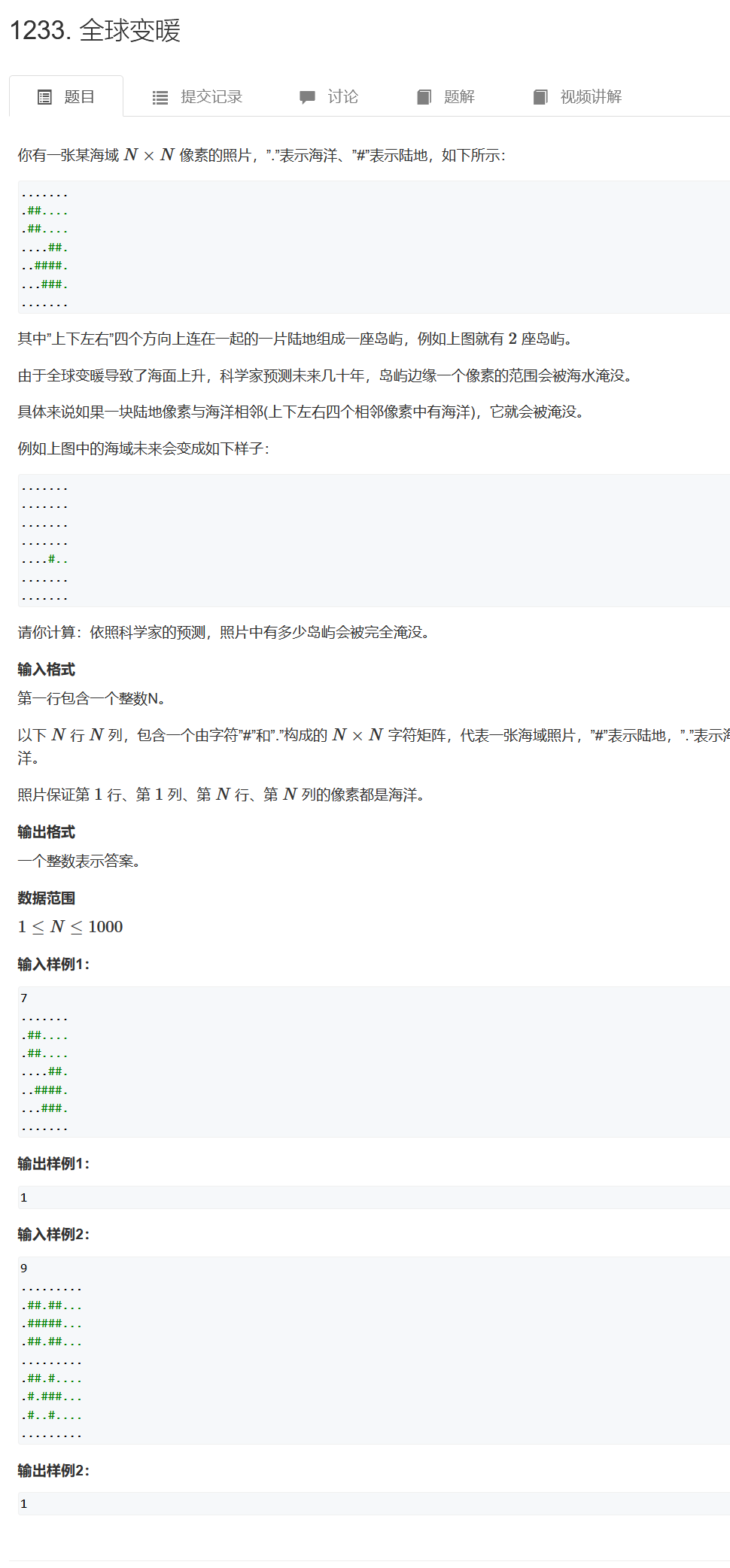
#include<bits/stdc++.h>
using namespace std;
const int N=1010;
int n;
char g[N][N];
int cnt; // 淹没岛屿的数量
bool st[N][N];
int dx[]={-1,0,1,0},dy[]={0,1,0,-1};
bool check(int x,int y){
for(int i=0;i<4;i++){
int tx=x+dx[i],ty=y+dy[i];
if(tx>=0 && tx<n && ty>=0 && ty<n && g[tx][ty]=='.')
return true;
}
return false;
}
void dfs(int x,int y,int& total,int &bound){
st[x][y]=true;
total++;
if(check(x,y))bound++;
for(int i=0;i<4;i++){
int tx=x+dx[i],ty=y+dy[i];
if(tx<0 || tx>=n || ty<0 || ty>=n || g[tx][ty]!='#' || st[tx][ty])continue;
dfs(tx,ty,total,bound);
}
}
int main(){
cin >> n;
for(int i=0;i<n;i++)
cin >> g[i];
for(int i=0;i<n;i++){
for(int j=0;j<n;j++){
if(g[i][j]=='#' && !st[i][j]){
int total=0,bound=0;
dfs(i,j,total,bound);
if(total==bound)cnt++;
}
}
}
cout << cnt << endl;
return 0;
}